In C++, `static_assert` is a compile-time assertion that checks a condition and produces a compilation error if the condition is false, ensuring that certain requirements are met before the program is compiled.
Here’s a simple example:
static_assert(sizeof(int) == 4, "This code requires an integer size of 4 bytes.");
What is `static_assert`?
`static_assert` is a powerful feature in C++ that allows developers to perform compile-time assertions. This means that certain conditions can be checked when the code is compiled instead of at runtime, which can lead to safer and more reliable code.
Syntax: The basic syntax of `static_assert` is as follows:
static_assert(condition, "Error message");
The `condition` must be a constant expression that evaluates to either `true` or `false`. If the condition is `false`, the compiler generates an error and outputs the provided error message.

How Does `static_assert` Work?
Compile-Time Checking
The main advantage of using `static_assert` is that it checks conditions during compilation, which helps prevent potential runtime errors. This mechanism allows you to enforce constraints on template parameters, types, and conditions that must be satisfied before the program can be successfully compiled.
Error Messages
Using informative error messages in `static_assert` enhances the clarity of the code and helps others understand the issue when an assertion fails. A well-structured error message provides context, making debugging significantly easier. For example, consider the following code:
static_assert(sizeof(int) == 4, "This compiler must support 4-byte integers.");
If executed in a compiler that does not comply with this requirement, the developer will receive a clear and precise error message indicating the nature of the problem.
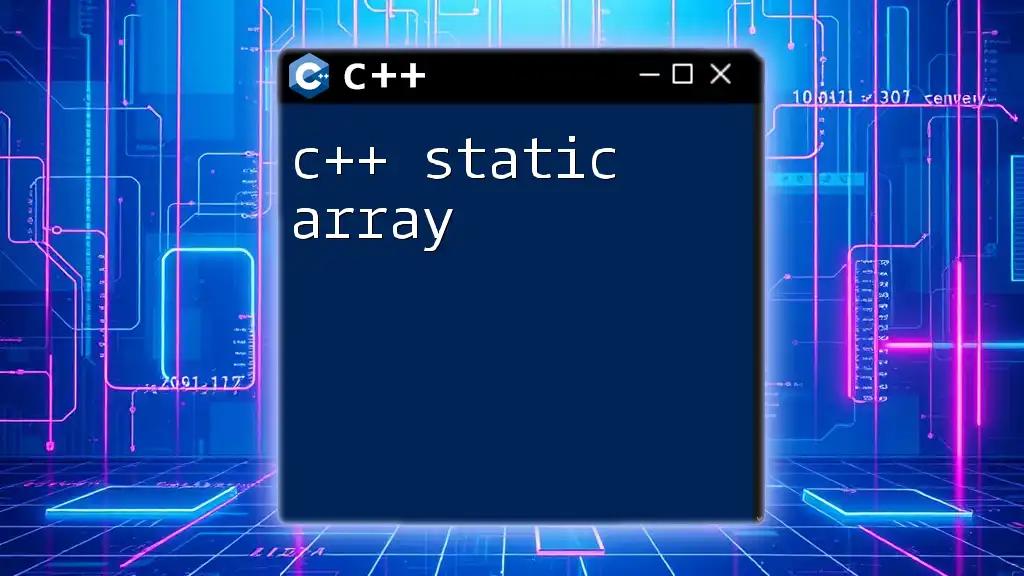
Benefits of Using `static_assert`
Early Error Detection
Detecting errors during compilation rather than at runtime is one of the most significant benefits of using `static_assert`. This early detection fosters a more robust development process, allowing developers to ensure the correctness of pre-conditions before the code is even executed.
Improved Code Safety
By incorporating `static_assert`, developers can enforce specific conditions for data types and template parameters, ultimately enhancing the safety of their code. The compiler effectively becomes an ally in catching unexpected scenarios before they lead to catastrophic failures.
Documentation
Using `static_assert` can also serve as a form of documentation within the codebase. Assertions outline important constraints and expectations for the types being used, providing clarity for future maintainers or for developers who may not be familiar with the original code.

Common Use Cases
Type Traits
`static_assert` is commonly used in conjunction with type traits from the Standard Library, which provide meta-information about data types. For instance, if you want to ensure that a given template parameter is an integral type, you can do so as follows:
static_assert(std::is_integral<T>::value, "T must be an integral type");
This assertion guarantees that `T` will always be an integral type, enhancing the correctness of functions and templates that rely on such assumptions.
Template Programming
In template programming, `static_assert` can enforce specific constraints on template parameters. For example, you may want to restrict the size of a given type. Implementing it can look like this:
template<typename T>
void func() {
static_assert(sizeof(T) <= 8, "Type size must be 8 bytes or less");
}
This kind of constraint ensures that templates only accept types that fit within particular size requirements, safeguarding against potential issues arising from type misusage.
Class Design
When designing classes, you might want to restrict types that can be used as template parameters. For instance, consider the following class definition:
template<typename T>
class Array {
static_assert(std::is_pod<T>::value, "Array can only hold POD types");
};
In this example, the `Array` class can only accept Plain Old Data (POD) types, thus preventing the potential inclusion of complex types that could lead to undefined behavior.

Limitations of `static_assert`
Scope of Use
While `static_assert` offers immense utility, it does have limitations. It can only be used in contexts where the assertion can be evaluated at compile-time; thus, it cannot be used for conditions that depend on runtime data, such as dynamically allocated memory or user inputs.
Complex Conditions
Another constraint of `static_assert` is its limited ability to express complex conditions. It is better suited for simpler boolean expressions and type traits rather than intricate logical evaluations, which may necessitate alternative programming approaches.
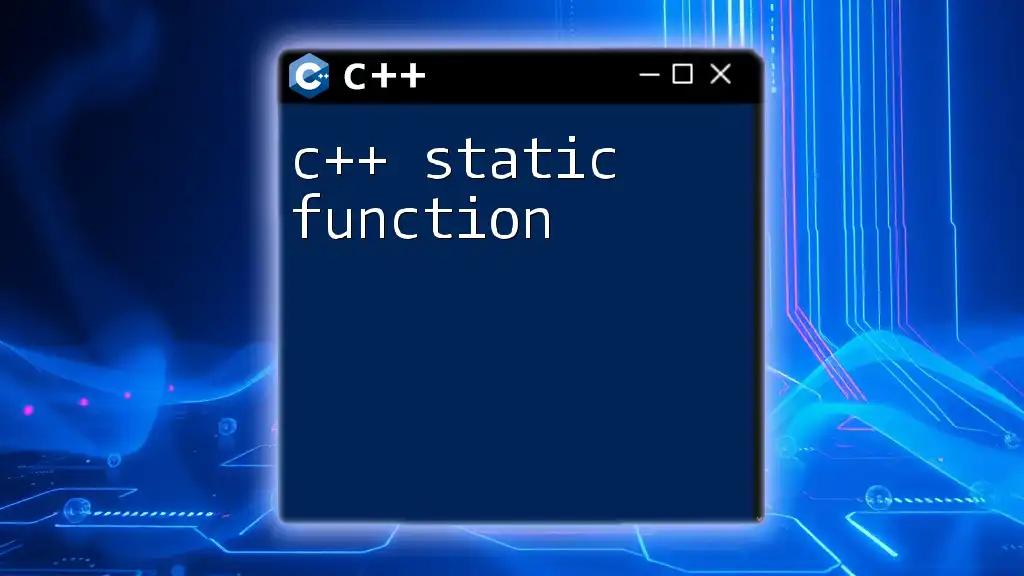
Error Messages in `static_assert`
Formatting and Clarity
A clear and informative error message is one of the most important aspects of `static_assert`. Well-structured messages assist developers in quickly identifying the source of the problem when an assertion fails. Following best practices, you might write:
static_assert(sizeof(T) == 8, "Error: T should be 8 bytes long. Check data type.");
This error message specifies exactly what is wrong, making it easier to troubleshoot.

Example Scenarios
Enforcing Constraints on Templates
Consider a practical example where you use `static_assert` to enforce constraints on templates that require only floating-point types.
template<typename T>
void check_type() {
static_assert(std::is_floating_point<T>::value, "Only floating point types are allowed");
}
In this example, if a non-floating-point type is passed to `check_type`, the compilation will fail, providing the caller with an informative error message.
Constrain Class Sizes
Another scenario involves enforcing size constraints on classes. Here's an example that demonstrates how to use `static_assert` to ensure a class meets a specific size requirement:
class LargeObject {
char data[100];
};
static_assert(sizeof(LargeObject) == 100, "LargeObject must be exactly 100 bytes.");
This assertion guarantees that the `LargeObject` class is always 100 bytes in size, preventing unintended changes that could violate this requirement.
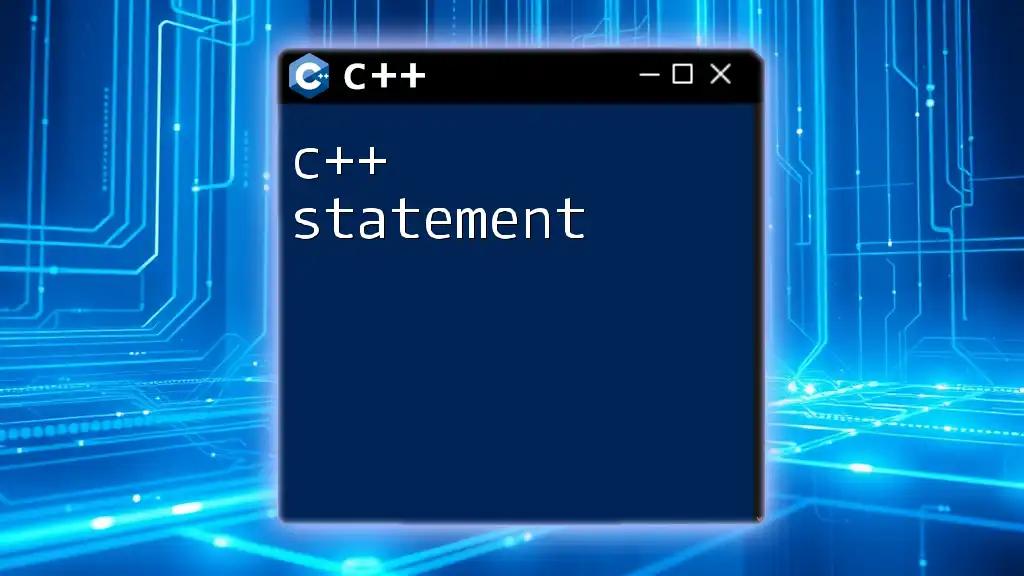
Conclusion
In summary, C++ static assert serves as a vital tool for developers looking to create robust and maintainable code. By providing compile-time checks, it enhances early error detection, improves code safety, and acts as a form of in-code documentation. Understanding and utilizing `static_assert` effectively can significantly elevate your programming practices, ensuring your code is not only functional but also adheres to the intended constraints and requirements.
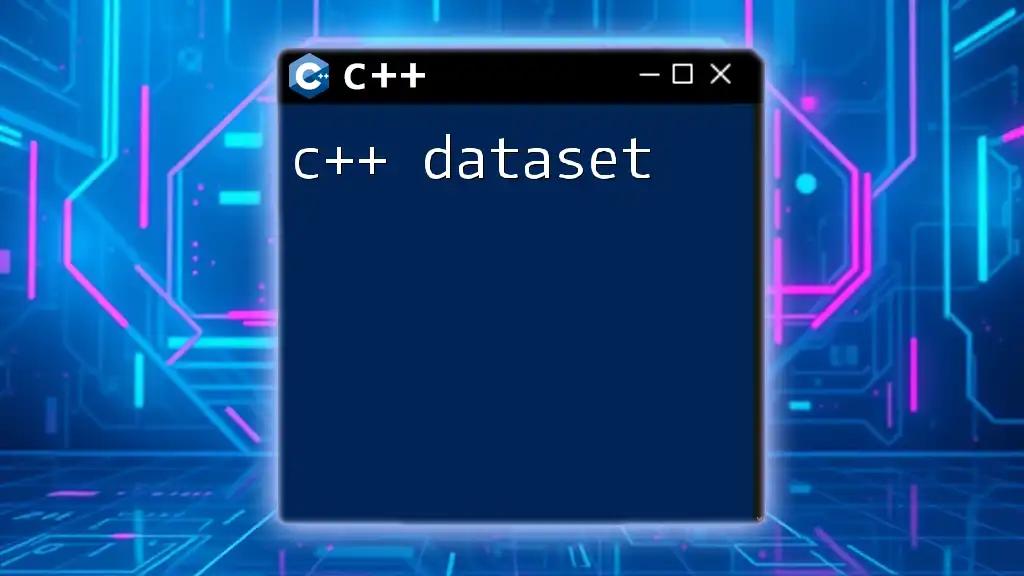
Additional Resources
For further exploration of `static_assert` and its applications, C++ standard libraries, templates, and type traits, consider diving into comprehensive C++ documentation or advanced programming texts. These resources will expand your knowledge and equip you with the necessary skills to leverage the full capabilities of the C++ language.

Call to Action
I encourage you to implement `static_assert` in your code and experience its benefits firsthand. Share your experiences or any queries you might have related to `static_assert` in the comments section below! Your insights can help foster a community of learning and programming excellence.