A C++ dataset typically refers to a collection of structured data that can be used for analysis or processing within a C++ program, often represented through arrays or custom data structures.
Here's a simple example of how to define and initialize a dataset using an array in C++:
#include <iostream>
int main() {
int dataset[] = {10, 20, 30, 40, 50}; // An example dataset
int size = sizeof(dataset) / sizeof(dataset[0]);
std::cout << "Dataset elements:" << std::endl;
for (int i = 0; i < size; i++) {
std::cout << dataset[i] << " ";
}
return 0;
}
Understanding C++ and Datasets
C++ Overview
C++ is a powerful object-oriented programming language renowned for its performance and versatility. While many use C++ for applications, system software, and game development, it is also well-suited for managing datasets. Understanding how to effectively work with datasets in C++ can empower developers to handle data processing with agility and precision.
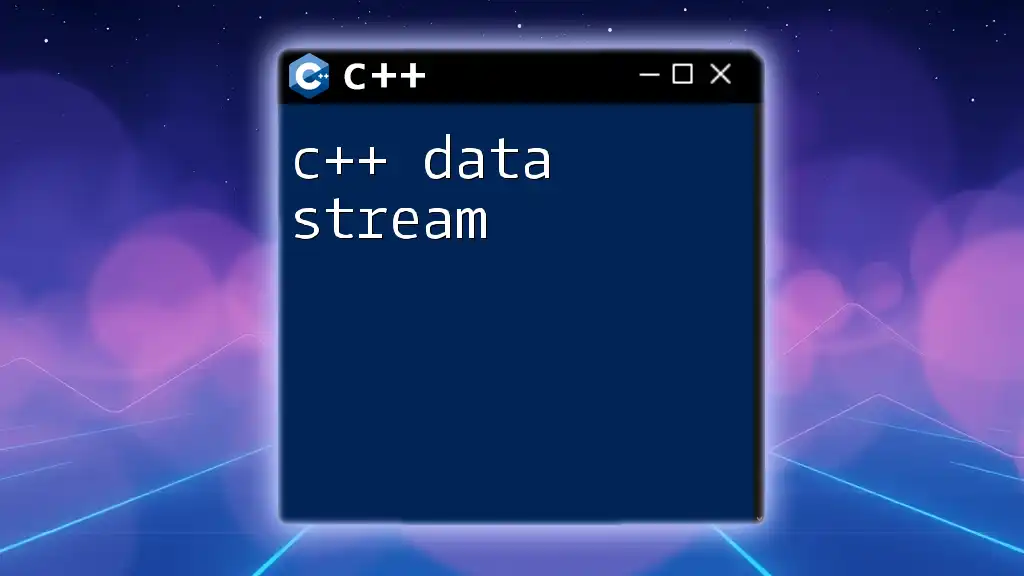
Types of Datasets in C++
Flat Files
Definition and Characteristics
Flat files are a universal way to store tabular data in a plain text format. They can either be text files or binary files, making them a simple yet effective means of handling datasets in C++. Being human-readable, text files are particularly easy to manage, while binary files offer compactness and speed.
Example Code for Reading/Writing Flat Files
Here's a basic C++ example that demonstrates how to write to and read from a flat file:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// Writing to a flat file
ofstream outfile("data.txt");
outfile << "Hello, World!" << endl;
outfile.close();
// Reading from a flat file
string line;
ifstream infile("data.txt");
while (getline(infile, line)) {
cout << line << endl;
}
infile.close();
return 0;
}
Databases
Using SQLite with C++
Databases are complex systems that allow for easy storage and retrieval of data. By using SQLite, a popular embedded database, developers can create datasets that are robust and easily queryable.
To connect to a SQLite database in C++, you can use the SQLite library. Once linked, performing simple queries becomes straightforward.
Example of Connecting and Querying a SQLite Database
Here's a scaffolded version of how you might set up a SQLite connection:
#include <sqlite3.h>
// Code for initializing SQLite, opening a database connection, and performing queries will go here
In-Memory Structures
Vectors and Arrays
C++ offers several ways to handle data in memory, with vectors and arrays being the most common. Vectors offer dynamic sizing and are part of the Standard Template Library (STL), while arrays need to be statically defined.
With vectors, you can manage datasets efficiently, dynamically adjusting to the size of the data you're processing.
Example of Using a Vector for Datasets
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> dataset = {1, 2, 3, 4, 5};
for (int data : dataset) {
cout << data << " ";
}
return 0;
}
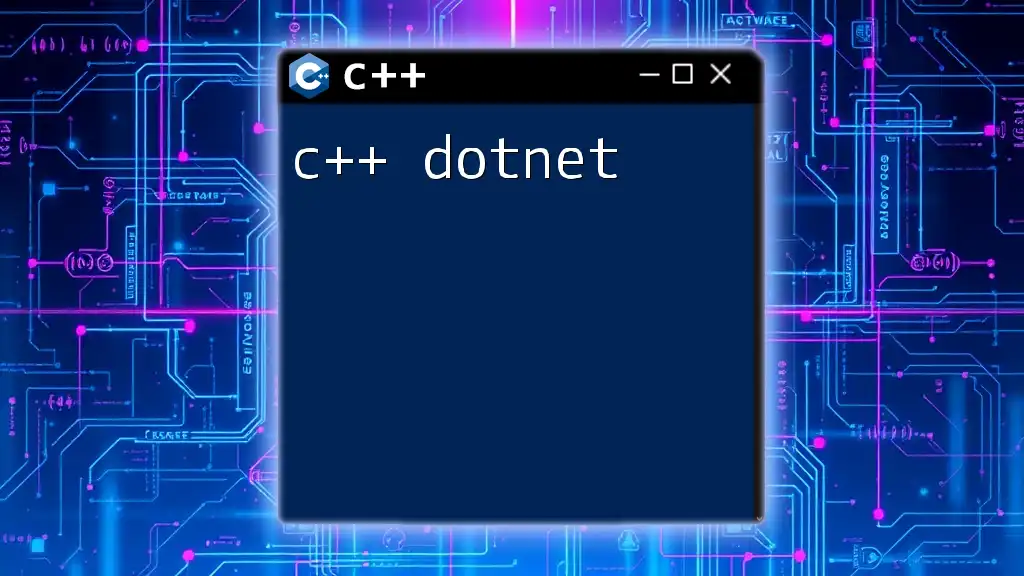
Working with Datasets in C++
Reading Data
Reading data can be accomplished through various file formats, with CSV (Comma-Separated Values) being one of the most prevalent. Efficient techniques for reading including file streams are imperative for performance, especially when dealing with large datasets.
When reading CSV data into C++, you can create a function to parse each line and extract the necessary fields.
Example Code to Read a CSV File
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
using namespace std;
void readCSV(const string& filename) {
ifstream file(filename);
string line;
while (getline(file, line)) {
stringstream ss(line);
string item;
while (getline(ss, item, ',')) {
cout << item << " ";
}
cout << endl;
}
}
int main() {
readCSV("data.csv");
return 0;
}
Data Manipulation
Sorting and Filtering
Sorting and filtering are core aspects of data management. C++ provides a variety of algorithms in the `<algorithm>` library, making it convenient to handle such operations.
For example, you can use the `std::sort` function to sort a dataset efficiently. This capability is crucial when looking to derive meaningful insights from your datasets.
Example of Sorting a Dataset
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> dataset = {5, 1, 4, 2, 3};
sort(dataset.begin(), dataset.end());
for (int data : dataset) {
cout << data << " ";
}
return 0;
}
Aggregation
Data aggregation is the process of summarizing detailed data into a more concise format. Common forms of aggregation include sums, averages, and counts.
Example of Calculating the Average of a Dataset
#include <iostream>
#include <vector>
using namespace std;
double calculateAverage(const vector<int>& data) {
double sum = 0;
for (int num : data) {
sum += num;
}
return sum / data.size();
}
int main() {
vector<int> dataset = {10, 20, 30, 40, 50};
cout << "Average: " << calculateAverage(dataset) << endl;
return 0;
}
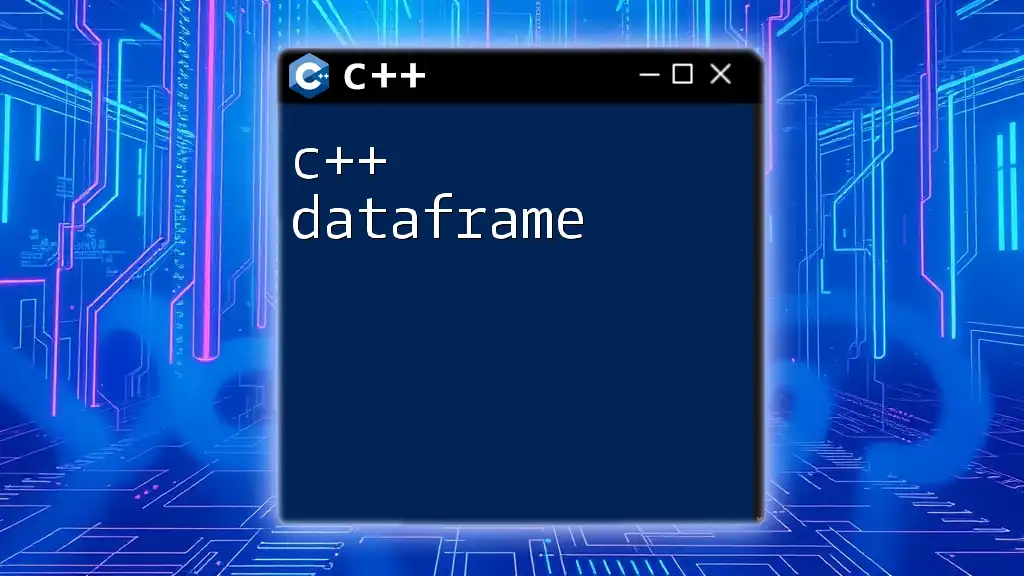
Advanced C++ Dataset Techniques
Templates and Data Structures
Templates in C++ allow for more flexible code, enabling the creation of functions and classes that can operate with any data type. This flexibility is particularly useful when creating versatile data handling structures that can adapt to the type of dataset being processed.
Example of Using Templates for Different Data Types
#include <iostream>
#include <vector>
using namespace std;
template<typename T>
void printDataset(const vector<T>& dataset) {
for (const auto& data : dataset) {
cout << data << " ";
}
cout << endl;
}
int main() {
vector<int> intDataset = {1, 2, 3};
vector<string> strDataset = {"Hello", "World"};
printDataset(intDataset);
printDataset(strDataset);
return 0;
}
External Libraries for Data Handling
Boost C++ Libraries
Boost is a set of C++ libraries that extend the functionality of C++. Several libraries within Boost cater to data manipulation and management, making it an excellent choice for anyone working with complex datasets.
Pandas for C++
While Pandas is primarily known for its Python implementation, there are C++ libraries inspired by it that facilitate data frame-like structures. These libraries enable users to manage and analyze datasets in a manner similar to how they would in Python.
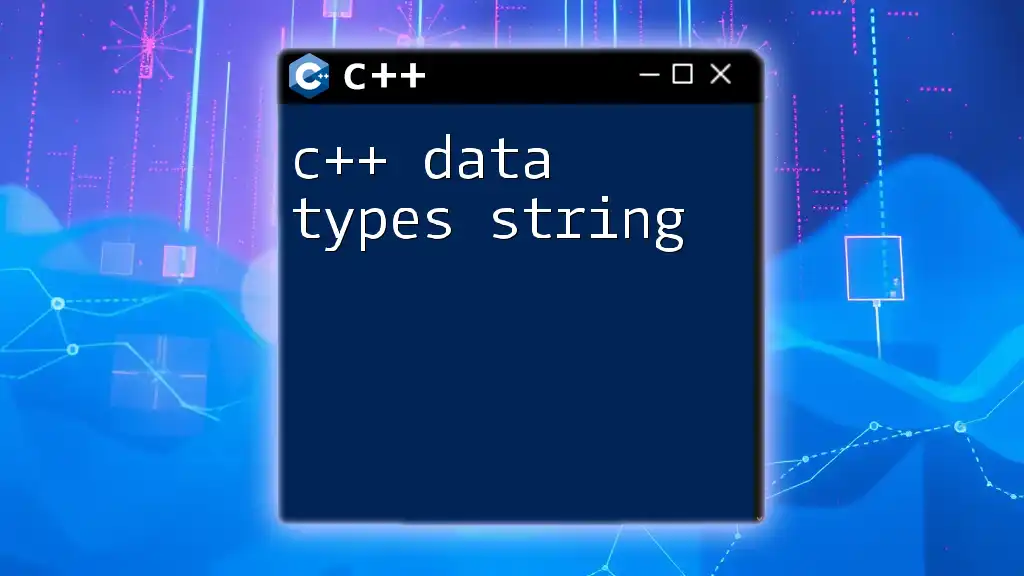
Practical Applications of Datasets in C++
Machine Learning with C++
Machine Learning (ML) is revolutionizing how datasets are used across various domains. C++ can be harnessed to build models that process large datasets efficiently. Many ML libraries, such as TensorFlow or Dlib, can be utilized to deploy sophisticated algorithmic solutions using C++.
Data Visualization
While C++ is generally not the primary language for data visualization, various libraries can be used to create visual representations of datasets. Integrating libraries like Matplotlib C++ can enable graphical outputs that help to interpret the underlying data better.
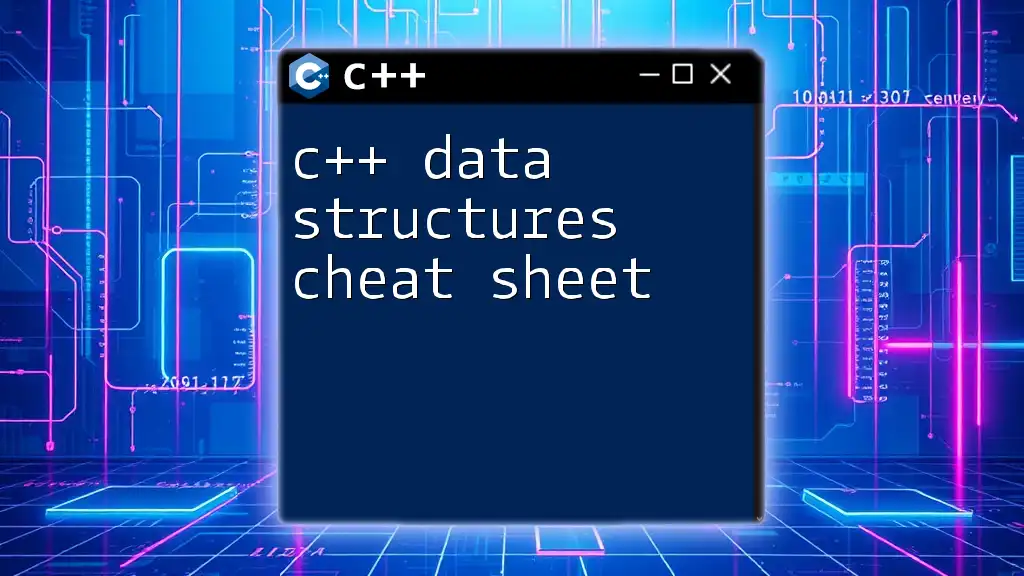
Conclusion
In this guide, we've explored the foundation of working with a C++ dataset, from understanding different types of datasets to practical applications and advanced techniques. C++ offers robust capabilities for managing datasets, and by experimenting with the concepts and examples provided, you can enhance your ability to work with data effectively.