C++ gtest, or Google Test, is a popular framework for writing unit tests in C++, allowing developers to create and run tests easily to ensure code reliability.
Here's a simple example of a C++ gtest:
#include <gtest/gtest.h>
// Function to be tested
int Add(int a, int b) {
return a + b;
}
// Test case for the Add function
TEST(AddTest, PositiveNumbers) {
EXPECT_EQ(Add(2, 3), 5);
EXPECT_EQ(Add(10, 20), 30);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
What is Google Test?
Google Test, commonly referred to as gtest, is a popular unit testing framework for C++. It provides a rich set of features that helps developers write effective and efficient unit tests. Unit testing is a critical part of the software development process, allowing developers to verify that individual parts of their code are functioning correctly. With Google Test, you can automate this process, making it easier to catch bugs and ensure code quality throughout the development lifecycle.
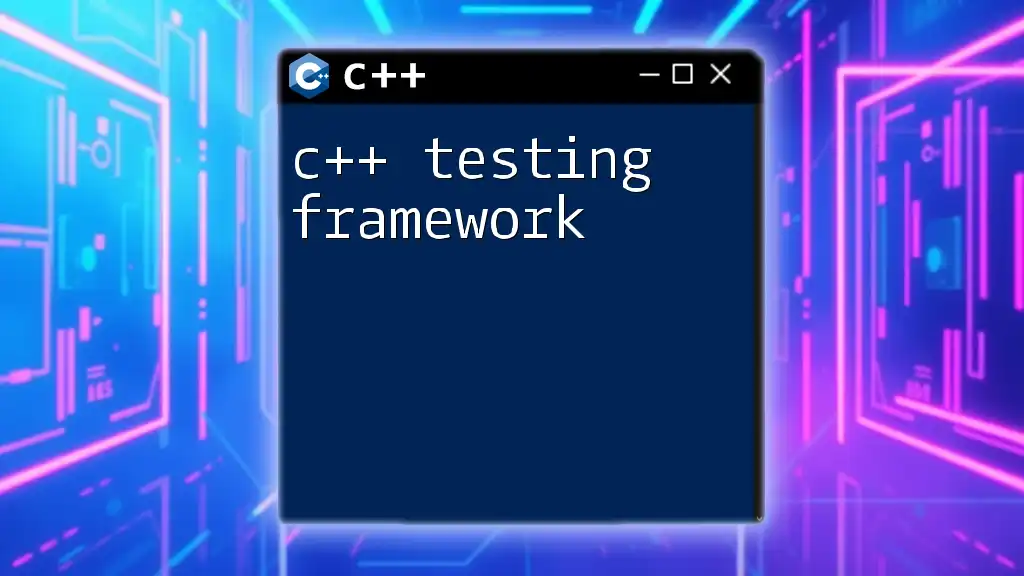
Why Use GTest C++?
Choosing Google Test over other testing frameworks comes with several significant benefits:
- Rich Assertions: Google Test comes with a wide array of assertion macros that help you validate the behavior of your code through readable and expressive syntax.
- Test Fixtures: This feature allows you to define reusable setups for your tests, providing a cleaner and more manageable way to share test-related logic.
- Death Tests: GTest enables you to verify that your code behaves as expected when it encounters fatal failures, ensuring robustness in your applications.
- Parameterized Tests: GTest supports writing tests that can be run with different sets of parameters, allowing for comprehensive testing scenarios without code duplication.
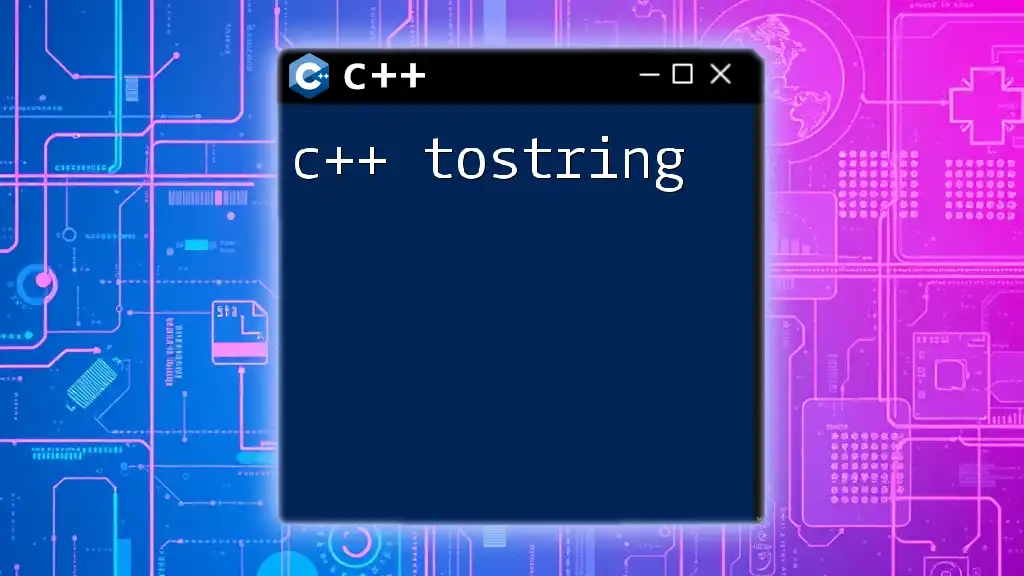
Setting Up Google Test
Installation of Google Test
Installing Google Test is straightforward. You can either build it from source or use a package manager to install it directly:
- Building from Source: You can clone the Google Test repository from GitHub, then compile it using CMake.
git clone https://github.com/google/googletest.git
cd googletest
mkdir build && cd build
cmake ..
make
- Using Package Managers: Many package managers allow you to install Google Test easily. For example, on Ubuntu, you can use:
sudo apt-get install libgtest-dev
Configuring Your Environment
To integrate Google Test into your project, you’ll typically use CMake. Here’s an example CMake configuration for a project that includes Google Test:
# Sample CMakeLists.txt configuration
cmake_minimum_required(VERSION 3.10)
project(MyTestProject)
# Fetch GoogleTest from GitHub
include(FetchContent)
FetchContent_Declare(
gtest
GITHUB_REPOSITORY google/googletest
GITHUB_TAG release-1.11.0
)
FetchContent_MakeAvailable(gtest)
# Create test executable
add_executable(my_tests test.cpp)
target_link_libraries(my_tests gtest gtest_main)
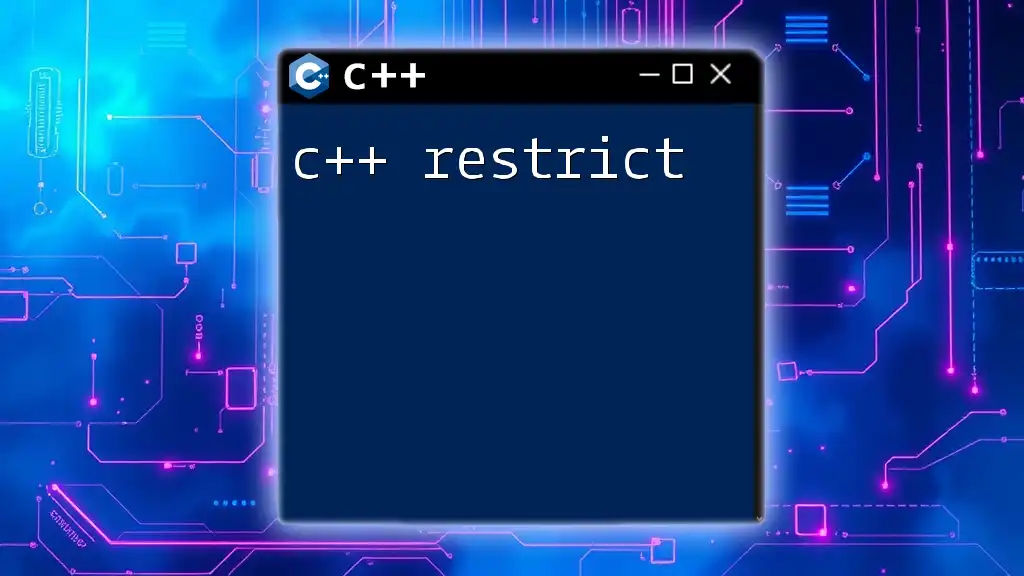
Writing Tests with GTest
Structure of a Google Test Case
The basic structure of a test case in Google Test utilizes the `TEST` macro. Each test consists of a name that includes the test suite name and the test name.
#include <gtest/gtest.h>
// Sample test case
TEST(SampleTest, PositiveTest) {
EXPECT_EQ(1, 1);
}
In the above code, `SampleTest` is the suite name, and `PositiveTest` is the specific test case. This clear structure helps in organizing tests and understanding their purpose at a glance.
Test Assertions in Google Test
Assertions are the backbone of unit tests as they determine whether a test has passed or failed. Google Test offers various assertions, such as:
- `EXPECT_EQ`: Checks if two values are equal.
- `ASSERT_TRUE`: Verifies that a condition is true, aborting the test if it isn’t.
Using both `EXPECT` and `ASSERT` is crucial; the key difference is that `EXPECT` allows the test to continue running even if it fails, while `ASSERT` will stop further execution of that test.
Grouping Tests: Test Suites
A test suite groups related tests together, making it easier to manage and run sets of tests. Google Test empowers you to define test suites using the `TEST` macro as well.
TEST(SampleSuite, FirstTest) {
EXPECT_TRUE(true);
}
TEST(SampleSuite, SecondTest) {
EXPECT_EQ(4, 2 * 2);
}
In this example, both tests are part of the `SampleSuite`, allowing for organized execution and reporting.
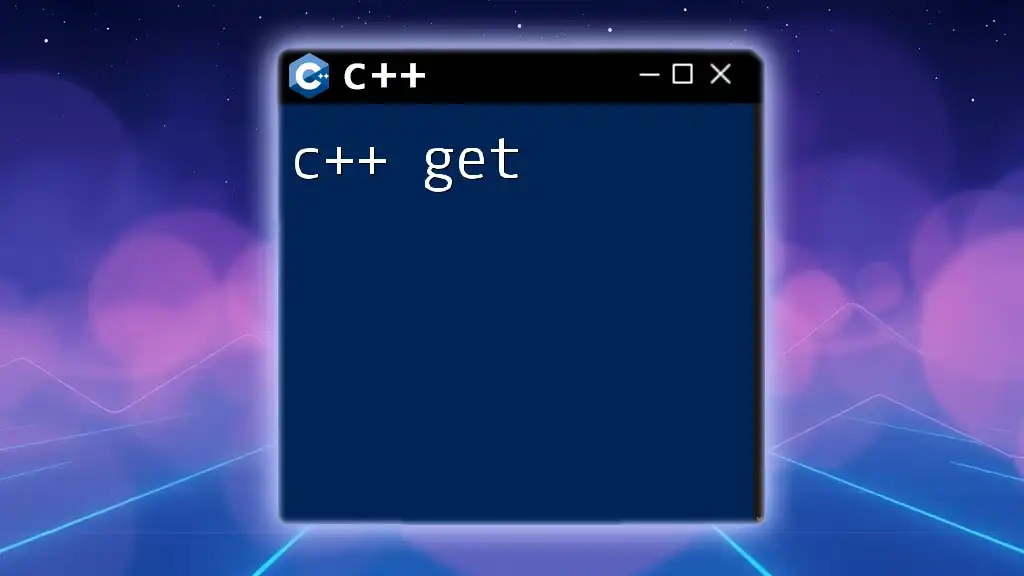
Advanced Testing Features
Test Fixtures in Google Test
Test fixtures enable you to set up a common environment for a series of tests. Google Test provides `SetUp` and `TearDown` methods for this purpose.
class MyTestFixture : public ::testing::Test {
protected:
void SetUp() override {
// Code to set up test environment
}
void TearDown() override {
// Code to clean up after tests
}
};
TEST_F(MyTestFixture, FixtureTest) {
EXPECT_EQ(1, 1);
}
Using fixtures promotes reuse of setup code during testing, reducing redundancy and enhancing maintainability.
Parameterized Tests
Parameterized tests are useful for running the same test logic with different input values. This method helps ensure that your code behaves correctly under various conditions.
class MyParamTest : public ::testing::TestWithParam<int> {};
TEST_P(MyParamTest, TestParameter) {
int param = GetParam();
EXPECT_GT(param, 0);
}
INSTANTIATE_TEST_SUITE_P(MyParameters, MyParamTest, ::testing::Values(1, 2, 3));
This structure avoids repeating the test code while ensuring comprehensive coverage across multiple scenarios.
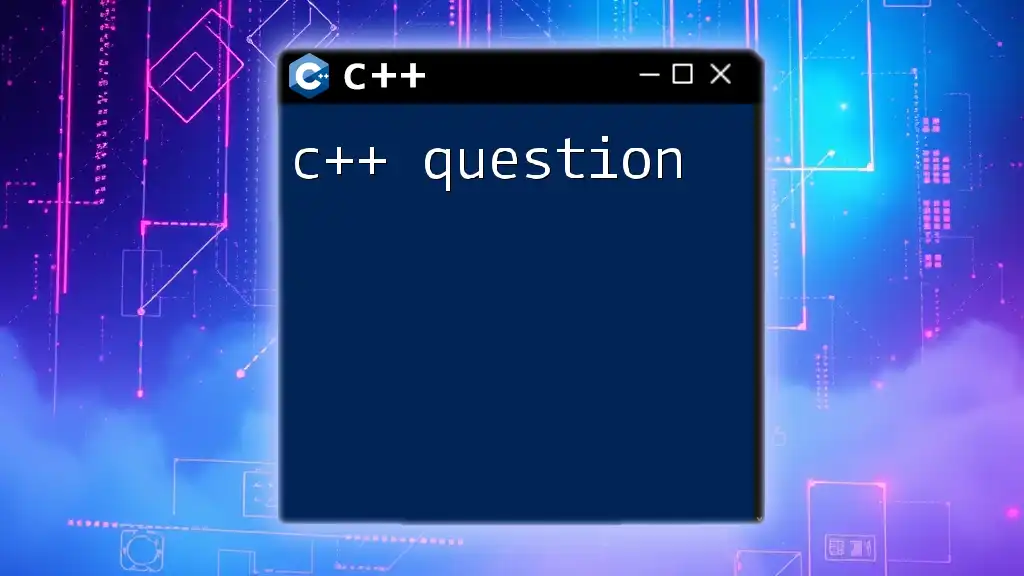
Running and Debugging Tests
Compiling and Running Your Tests
To compile and run the tests you've written, you can use `g++`:
g++ -o my_tests test.cpp -lgtest -lgtest_main -pthread
./my_tests
Incorporating flags like `-g` can help generate debug information, useful for diagnosing issues if a test fails.
Analyzing Test Results
Google Test’s output provides detailed summaries indicating passed and failed tests, including assertion messages. For cleaner logs, consider compiling with additional flags to enhance output readability.
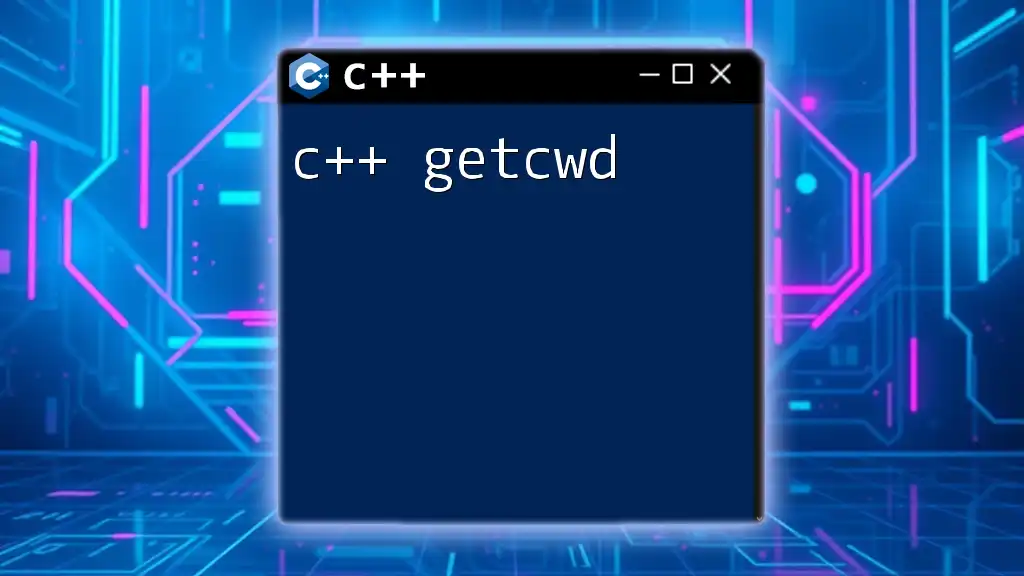
Best Practices for Using GTest
Writing Effective Unit Tests
When writing tests, keep the following tips in mind:
- Clear Names: Use descriptive names for tests to reflect their intentions, which enhances readability and maintainability.
- Isolate Tests: Each test should verify a single functionality without dependencies on other tests.
Organizing Your Test Code
Maintain a clear directory structure to manage your test files effectively. A good practice is to create a separate directory for tests, mirroring the source folder structure to make navigation seamless. For larger projects, consider organizing tests by feature or module to enhance manageability.
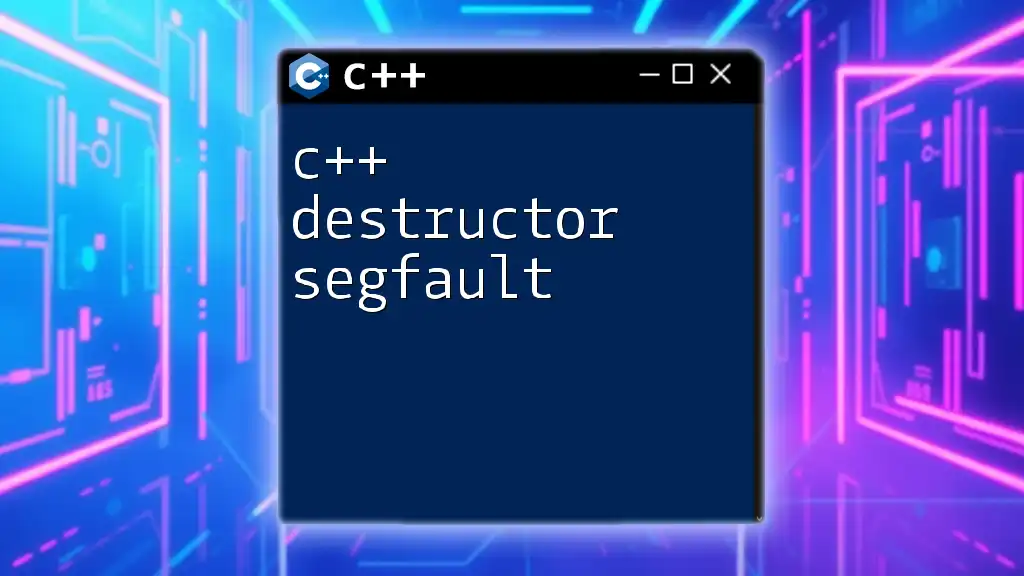
Conclusion
Using Google Test for C++ projects significantly boosts your ability to maintain high code quality through structured and automated testing. By integrating gtest into your development lifecycle, you ensure that potential issues are caught early and that your codebase remains robust and reliable. Start incorporating unit tests into your projects today to improve your software delivery process!
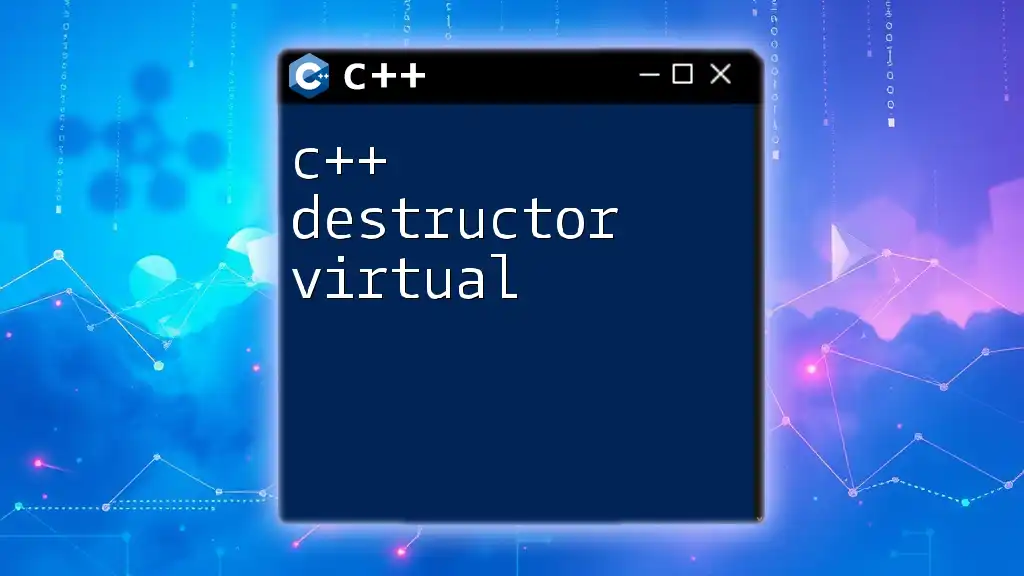
Further Resources
To delve deeper into unit testing with Google Test, consider exploring the official Google Test documentation or seeking out books and online courses focused on unit testing in C++. Engaging with these resources will enhance your understanding and proficiency in writing effective tests, making you a more capable C++ developer.