In C++, the `get` function is often used to read a single character or input from the standard input stream, commonly utilized with `std::cin` for user interaction.
Here’s a simple example using `std::cin.get()`:
#include <iostream>
int main() {
char ch;
std::cout << "Enter a character: ";
ch = std::cin.get(); // Reads a single character from input
std::cout << "You entered: " << ch << std::endl;
return 0;
}
What is "Get" in C++?
In C++, the term "get" is commonly associated with functions that retrieve or access data within classes, often referred to as getter functions. These functions play a critical role in controlling access to class properties, thereby facilitating encapsulation. A well-implemented "get" method ensures that the internal representation of the data remains protected while providing a clear interface for accessing it.

Understanding Getters
What are Getters?
Getters, or accessor functions, are special member functions that allow external code to obtain the values of private member variables. In the realm of Object-Oriented Programming (OOP), encapsulation is a principle that underlies the importance of getters. This approach enhances data integrity by preventing unauthorized access or modification of class members.
How to Define a Getter in C++
Defining a getter in C++ is straightforward. The syntax generally consists of declaring a public member function that returns the appropriate type. Here’s a simple example:
class Person {
private:
std::string name;
public:
Person(std::string n) : name(n) {}
std::string getName() const {
return name;
}
};
In this example, the `getName` function allows the `name` variable to be accessed without modifying it, as indicated by the `const` keyword following the function signature.
Benefits of Using Getters
Utilizing getters provides several benefits:
- Protecting Class Invariants: Getters ensure that the internal state of an object isn't altered inappropriately.
- Enhancing Code Maintainability: By centralizing access to class properties, changes can be made in one location without affecting other code that relies on those properties.
- Providing a Controlled Interface: Getters enable developers to manage how properties are accessed and allow for additional logic when fetching values, such as validation or logging.

C++ Standard Library: Getters in Context
Using std::getline
One practical application of the concept of "get" can be seen in the C++ Standard Library with the `std::getline` function. This function is used to read a line of text from an input stream into a string variable.
Here's a basic example:
std::string input;
std::cout << "Enter your name: ";
std::getline(std::cin, input);
std::cout << "Hello, " << input << "!";
In this code, `std::getline` captures the entire line entered by the user, demonstrating how "get" can be applied outside of getter functions.
The std::get Function Template
Another example related to "get" is the `std::get` function template, which is useful for accessing elements within tuples or pairs. Here’s how you can utilize it:
#include <tuple>
#include <iostream>
int main() {
std::tuple<int, std::string> person(1, "Alice");
std::cout << "ID: " << std::get<0>(person) << ", Name: " << std::get<1>(person) << std::endl;
return 0;
}
In this snippet, `std::get` is employed to extract individual elements from a tuple, showcasing the versatility of the "get" concept beyond class members.

Advanced "Get" Techniques in C++
Using Getters with Const-Correctness
Const-correctness is crucial in C++ to ensure that the function does not modify the object it is called on. In getter functions, marking them as `const` provides a guarantee that the function will only read data and not alter any state.
Here’s an example illustrating this concept:
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getRadius() const {
return radius;
}
};
The `getRadius` function returns the radius of the circle without modifying its state, which is vital for functions that are intended purely for data retrieval.
Chaining Getters
Chaining getters is a technique that enables seamless access to multiple properties of an object. This approach enhances code clarity and can significantly improve the conciseness and readability of your code.
Consider the following example:
class Car {
private:
std::string brand;
int year;
public:
Car(std::string b, int y) : brand(b), year(y) {}
std::string getBrand() const { return brand; }
int getYear() const { return year; }
};
Car myCar("Toyota", 2021);
std::cout << "Brand: " << myCar.getBrand() << ", Year: " << myCar.getYear() << std::endl;
In this code snippet, by calling `getBrand` and `getYear` on the `myCar` object, we demonstrate how chaining can simplify access to multiple properties.
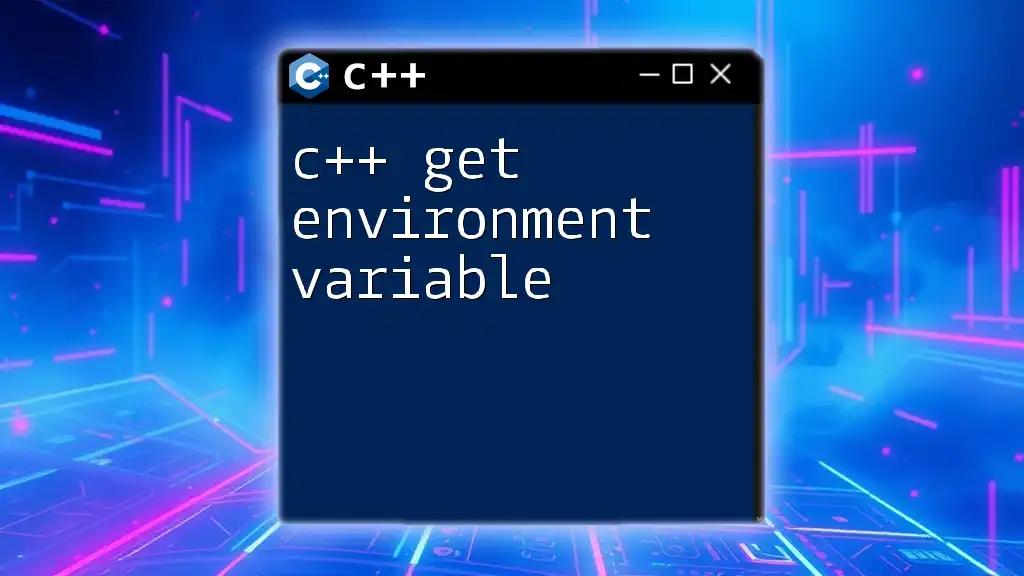
Best Practices for Using "Get" in C++
When implementing getter functions in C++, consider the following best practices:
- Keep Getters Simple: A getter should solely retrieve the value without performing complex operations.
- Avoid Modifying State: Ensure that getter functions do not alter the object's state. Use `const` to enforce this.
- Document Your Getters: Writing clear documentation helps other developers understand the intent and usage of your getters.
Getters have an intrinsic value in establishing a robust and maintainable codebase, particularly in applications where OOP principles are paramount.

Conclusion
The concept of "c++ get" encapsulates essential practices in object-oriented programming, focusing on data retrieval, encapsulation, and maintainability. Mastering getter functions enhances your ability to write efficient and clean code. As you continue exploring C++, remember that implementation practice reinforces understanding, so delve deeper into other C++ functionalities as you advance in your journey.

Additional Resources
While this guide has provided a solid foundation, further resources, including documentation and tutorials, can deepen your understanding of C++ and its powerful features.