To get the length of a vector in C++, you can use the `size()` member function, which returns the number of elements in the vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Length of the vector: " << vec.size() << std::endl;
return 0;
}
What is a Vector in C++?
A vector in C++ is a dynamic array provided by the Standard Template Library (STL). Vectors are part of the C++ Standard Library, and they automatically manage their memory, growing in size as needed. This makes them extremely versatile for storing collections of data.
Vectors have several advantages over traditional arrays:
- Dynamic Size: Unlike static arrays, vectors can grow and shrink as needed. This gives developers the flexibility to manage memory more efficiently and simplifies data management within applications.
- Easy Memory Management: Vectors handle memory allocation and deallocation automatically, reducing the risk of memory leaks.
- Built-in Functions: C++ vectors come equipped with various member functions that simplify common operations, such as adding, removing, and accessing elements.
To declare a vector, you typically use the following syntax:
#include <vector>
std::vector<int> myVector; // A vector of integers
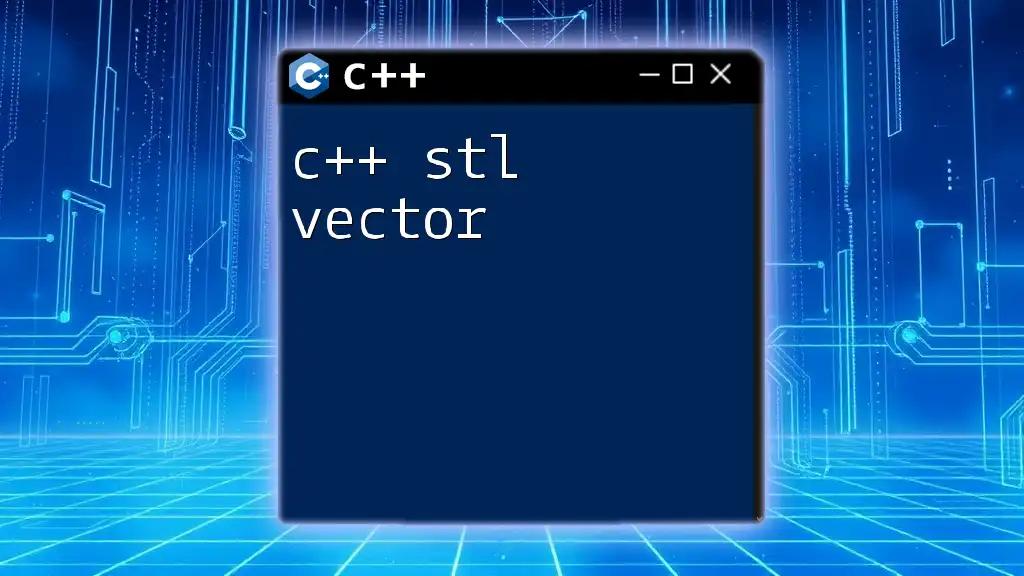
Understanding Vector Size and Length
When working with vectors in C++, it's essential to understand the concepts of size and length. In C++, the terms are often used interchangeably, but they have specific meanings.
- Size refers to the number of elements currently stored in the vector. You can obtain the size of a vector using the `size()` method.
- Length may refer to the same concept in everyday language, but in programming contexts, it often relates to the amount of memory allocated (capacity) as opposed to what is currently in use.
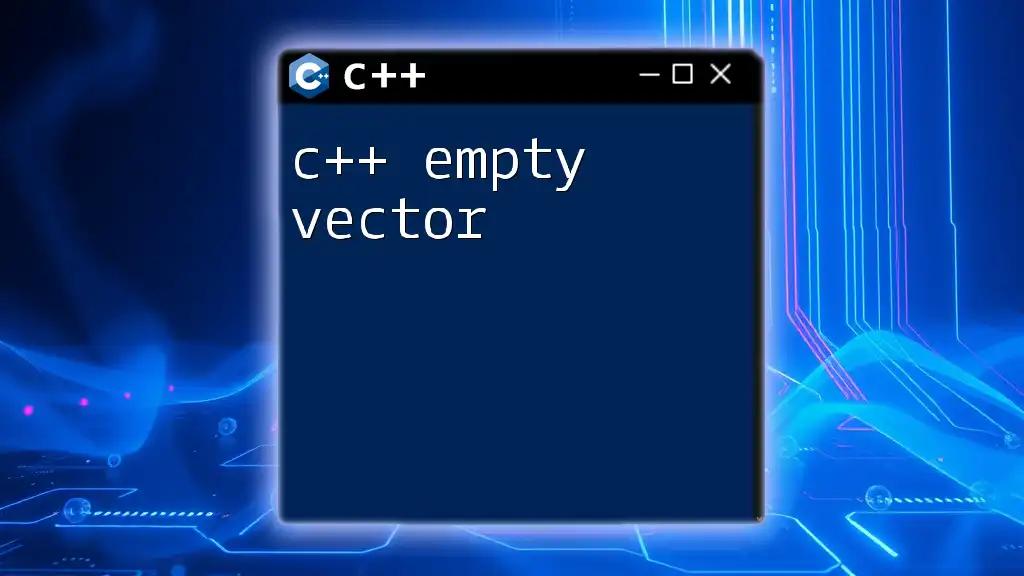
How to Get Size of Vector in C++
To get the size of a vector in C++, developers most commonly use the `size()` method, which returns the number of elements held in the vector.
Using the `size()` Method
Here’s a basic example of how to get the size of a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::cout << "Size of vector: " << myVector.size() << std::endl;
return 0;
}
In this example, the `size()` method will output `5` since there are five integers in `myVector`. This demonstrates the straightforward approach to determine how many elements your vector contains.
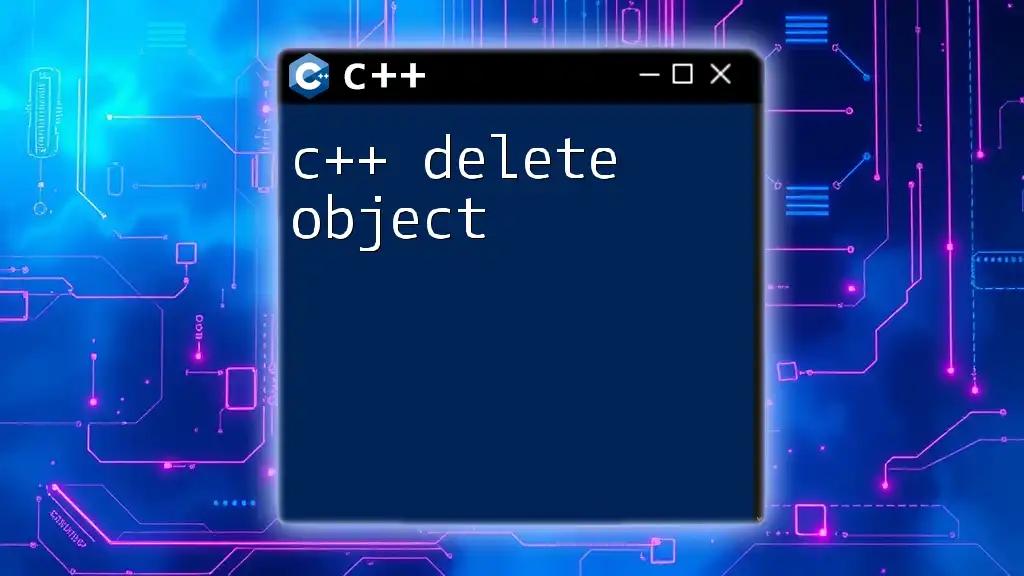
Length of Vector C++
Using the `length()` Method
It’s important to note that while some languages provide a `length()` method, C++ vectors do not. Instead, the proper way to get the size or length of a vector is to always use the `size()` method. Thus, you won't use `length()` with vectors in C++, as it is not a standard member function.

Other Ways to Get Size of a Vector C++
Accessing the Capacity
While the size represents how many elements are stored in the vector, the capacity signifies how much memory is allocated for the vector's future growth. To view a vector's capacity, you can use the `capacity()` method:
std::cout << "Capacity of vector: " << myVector.capacity() << std::endl;
Understanding both size and capacity allows for better memory management, especially in performance-critical applications.
Using Iterators
Another method to calculate the size of a vector involves iterators and the `distance()` function from the `<iterator>` header. This approach demonstrates the versatility of C++ STL:
#include <iterator>
// ... inside main
std::cout << "Size using iterators: " << std::distance(myVector.begin(), myVector.end()) << std::endl;
This code will yield the same result, showing the number of elements in the vector, and showcases an alternative method for determining the size.
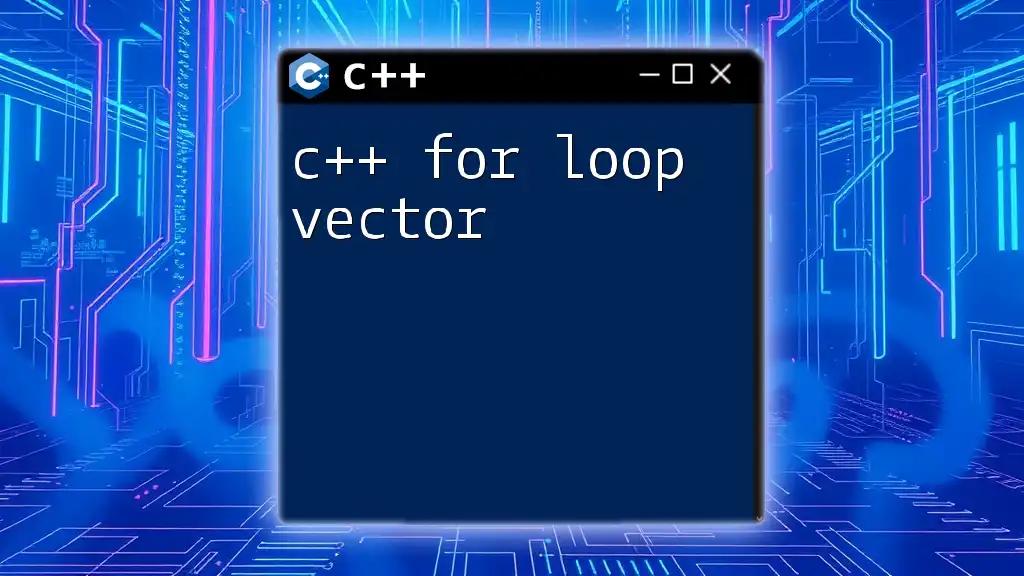
How to Get the Length of a Vector in C++
Stepping Through Elements
Although it's not common practice, one could technically determine the length of a vector by manually counting the elements. However, this is generally unnecessary, as using the `size()` method is more efficient. Here’s a simple illustration of manually counting elements:
int count = 0;
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
count++;
}
std::cout << "Manual count of vector elements: " << count << std::endl;
This method runs through each element, increasing the count, but it is less efficient and should be avoided when more straightforward methods are available.
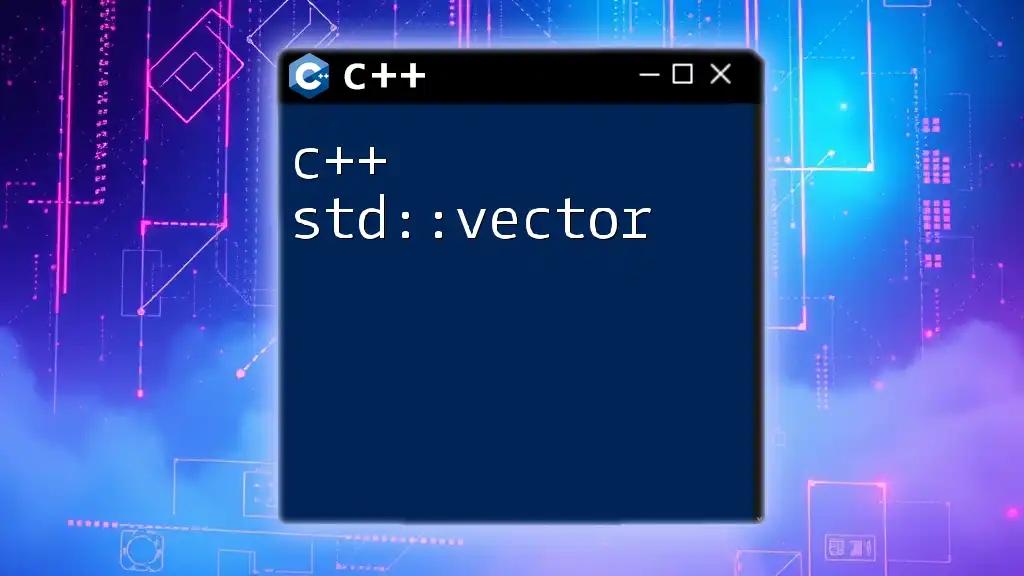
Common Mistakes to Avoid
While working with vectors, several common pitfalls can be encountered:
- Confusing `size()` with `capacity()`: Developers often misunderstand these two concepts. Remember, `size()` tells you how many elements are stored, while `capacity()` indicates how much space has been reserved.
- Forgetting to include necessary headers: When using vectors, always ensure to include `<vector>` and other relevant headers to avoid compilation errors.
- Improper initialization: Failing to initialize a vector or initializing it incorrectly can lead to unexpected results or crashes.
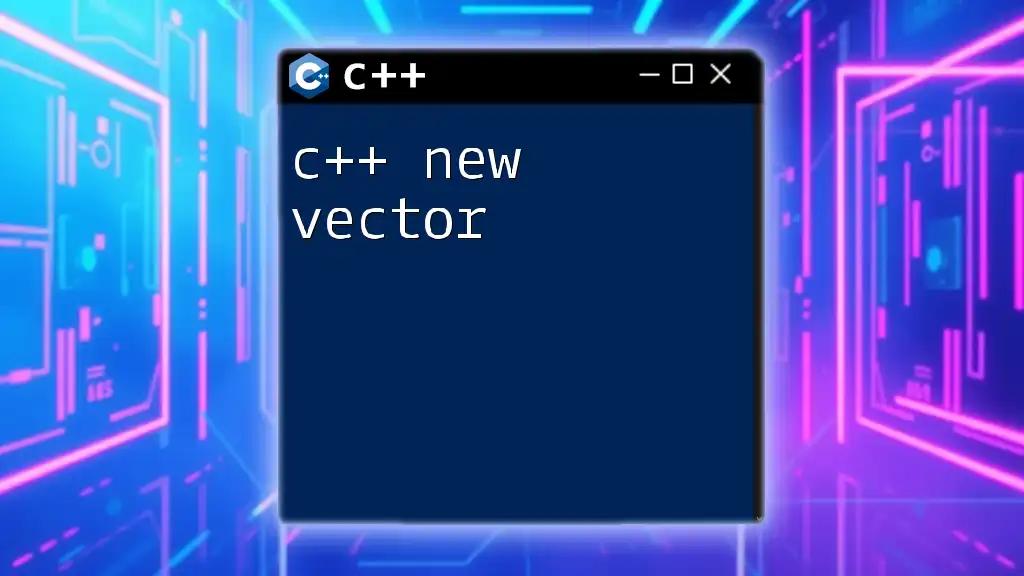
Practical Applications of Getting the Length of a Vector
Iterating Through Vectors
Knowing the vector's size is crucial when iterating through its elements. For loops often use the size to avoid out-of-bounds errors:
for(int i = 0; i < myVector.size(); i++) {
std::cout << "Element at index " << i << ": " << myVector[i] << std::endl;
}
This ensures that all elements are accessed safely within the vector's bounds.
Dynamic Operations
The length of a vector directly influences various functionalities. For instance, when implementing sorting algorithms, searching, or even basic data manipulation, knowing the vector's size allows for optimal logic and flow control in your programs.
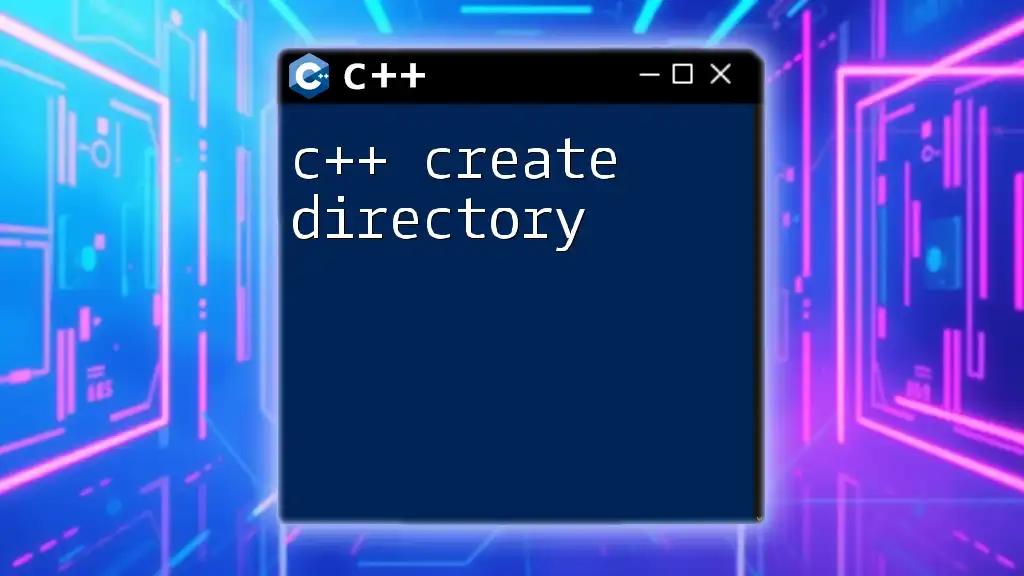
Conclusion
In summary, c++ get length of vector revolves around using the `size()` method to determine how many elements reside within a vector. Understanding the difference between size and capacity enhances your ability to manage memory efficiently. Mastering these concepts is vital for beginners and experienced developers alike, providing a foundation for efficient programming in C++. By understanding and leveraging the size of vectors, you can write concise, efficient, and effective C++ code.

FAQs
What is the difference between `size()` and `capacity()`?
The `size()` method returns the number of elements currently in the vector, while `capacity()` returns the total number of elements the vector can hold before needing to allocate more memory.
Can I use an array-like syntax with vectors?
Yes, vectors support array-like access using the subscript operator (`[]`), which allows you to get or set elements by their index.
How do vectors compare with other data structures in C++?
Vectors are dynamic and provide ease of use with built-in functions. They are often compared with arrays, lists, and deques, each having unique characteristics suited for specific use cases.