In C++, you can retrieve the last character of a string using the `back()` method or by accessing the last index directly. Here’s a code snippet demonstrating both approaches:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char lastChar1 = str.back(); // Using back() method
char lastChar2 = str[str.length() - 1]; // Accessing by index
std::cout << "Last character using back(): " << lastChar1 << std::endl;
std::cout << "Last character using index: " << lastChar2 << std::endl;
return 0;
}
Understanding C++ Strings
What is a String in C++?
In C++, a string is a sequence of characters represented as an object of the `std::string` class from the `<string>` header. Unlike traditional C-style strings, which are character arrays terminated by a null character, C++ strings provide a more convenient and flexible way to handle text. They manage memory automatically and offer a variety of functions for string manipulation.
Common String Operations
C++ strings support several common operations, including:
- Concatenation: Joining two strings together using the `+` operator.
- Length: Using the `length()` method to find the number of characters in a string.
For example, consider the following snippet that demonstrates string concatenation and length:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = " World!";
std::string result = str1 + str2;
std::cout << "Concatenated string: " << result << std::endl;
std::cout << "Length of the string: " << result.length() << std::endl;
return 0;
}

Accessing the Last Character of a String
The Basics of String Indexing
C++ uses zero-based indexing for strings, meaning the first character is at index 0, the second at index 1, and so on. This indexing system allows easy access to any character within the string, including the last character.
Using the `back()` Method
One of the simplest ways to get the last character of a string in C++ is by using the `back()` method. This method returns a reference to the last character of the string. Here's how it works:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char lastChar = str.back();
std::cout << "The last character is: " << lastChar << std::endl;
return 0;
}
In this example, the `back()` method retrieves the last character, which is `'!'`. This is an efficient and straightforward way to access the last character, leveraging the capabilities of the C++ Standard Library.
Using Indexing to Access the Last Character
Alternatively, you can access the last character of a string using indexing. To do this, you need to calculate the last index by subtracting one from the string's length, as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char lastChar = str[str.length() - 1];
std::cout << "The last character using indexing is: " << lastChar << std::endl;
return 0;
}
Here, `str.length() - 1` gives the index of the last character, ensuring accurate access to the desired element. This method can be particularly useful if you wish to manipulate or check the last character conditionally.
Checking If the String is Not Empty
Before accessing the last character, it’s crucial to ensure the string is not empty. Accessing the last character of an empty string using either `back()` or indexing may lead to undefined behavior or runtime errors. You can check if a string is empty using the `empty()` method, as demonstrated below:
#include <iostream>
#include <string>
int main() {
std::string str;
std::cout << "Enter a string: ";
std::getline(std::cin, str); // To include spaces
if (!str.empty()) {
char lastChar = str.back();
std::cout << "The last character is: " << lastChar << std::endl;
} else {
std::cout << "The string is empty." << std::endl;
}
return 0;
}
In this example, we first check if the string is empty before attempting to retrieve the last character. This practice enhances the robustness of your C++ code.
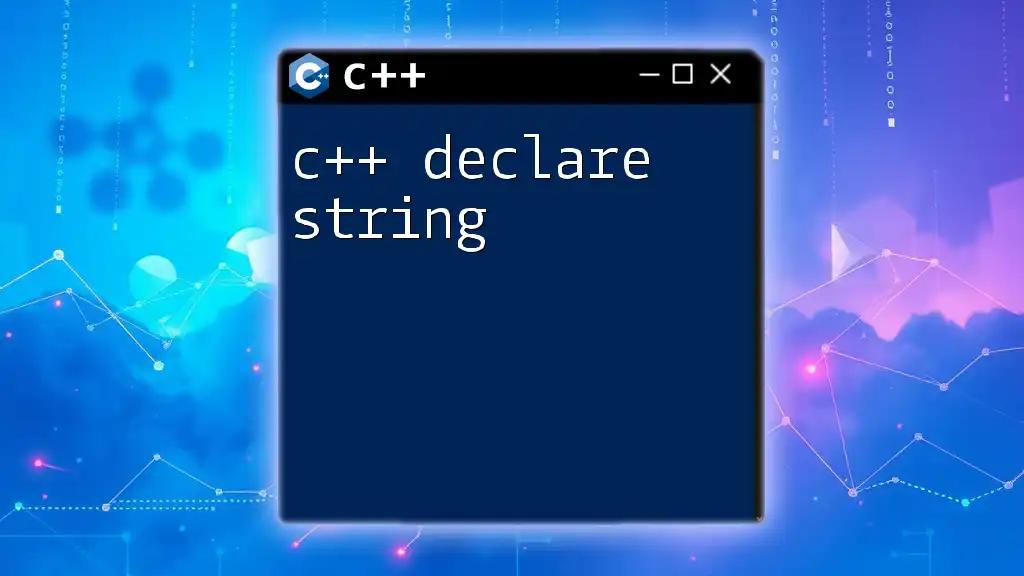
Additional Considerations
Handling Different String Types
It’s important to recognize the difference between C-style strings and C++ strings when accessing the last character. C-style strings are arrays of characters, and their last character is accessed differently. For example:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello, World!";
char lastChar = str[strlen(str) - 1];
std::cout << "The last character of the C-style string is: " << lastChar << std::endl;
return 0;
}
In this C-style string example, we use `strlen()` to find the length and then access the last character using indexing. This method is less safe than the C++ string methods since it requires careful management of memory and null terminators.
Performance Considerations
When deciding between the `back()` method and indexing to access the last character, consider your specific needs. The `back()` method is generally more readable and expressive, making it clear that you're accessing the last character. Conversely, indexing might be slightly more efficient in terms of performance in certain scenarios. Nonetheless, the difference is often negligible, so prioritize readability and maintainability in your code.
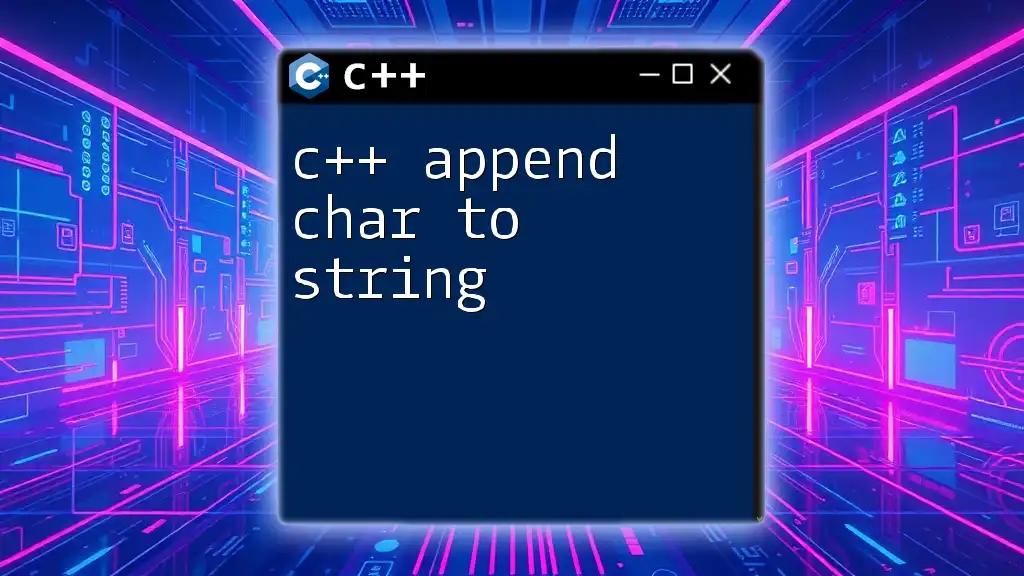
Summary
In summary, accessing the last character of a string in C++ can be efficiently achieved using the `back()` method or by calculating the last index. Both methods are effective; however, always ensure that the string is not empty to prevent errors. Mastering this fundamental string manipulation technique is essential for writing robust and effective C++ programs.
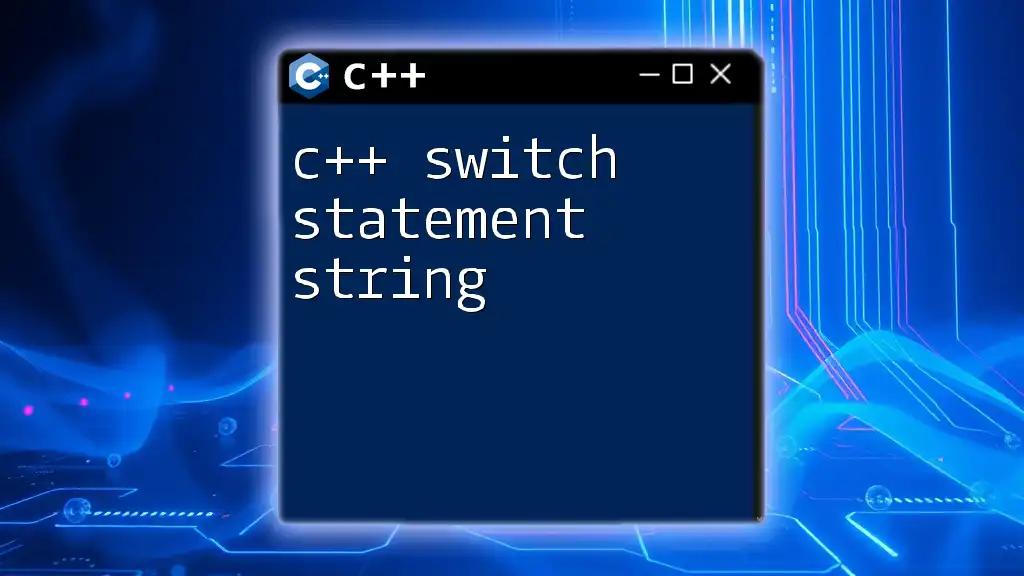
FAQs
Can I use the `back()` method on an empty string?
Attempting to use the `back()` method on an empty string results in undefined behavior. Always check if the string is empty using the `empty()` method before calling `back()`.
Is there any alternative to using `str[str.length() - 1]`?
Yes, using the `back()` method is a more concise and expressive alternative to access the last character directly, improving code readability.
What if my string contains multiple characters?
Both methods work seamlessly regardless of the number of characters in the string. They are designed to handle strings of varying lengths, provided you manage empty strings appropriately.