In C++, you can extract a substring from a string using the `substr()` method, specifying the starting position and length of the substring.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = str.substr(7, 5); // Extracts "World"
std::cout << subStr << std::endl;
return 0;
}
Understanding Substrings in C++
What is a Substring?
A substring is simply a subset of characters taken from a larger string. For instance, the word "apple" can have substrings like "app", "ple", or "p". Understanding how to manipulate substrings is crucial for various programming tasks, such as data parsing, text processing, and string comparison.
Overview of Strings in C++
In C++, strings are primarily represented by the `std::string` class, which is part of the C++ Standard Library. This class allows for dynamic string manipulation, which includes concatenation, slicing, and transformation. C++ strings simplify how we handle text, making operations like substring extraction straightforward and efficient.
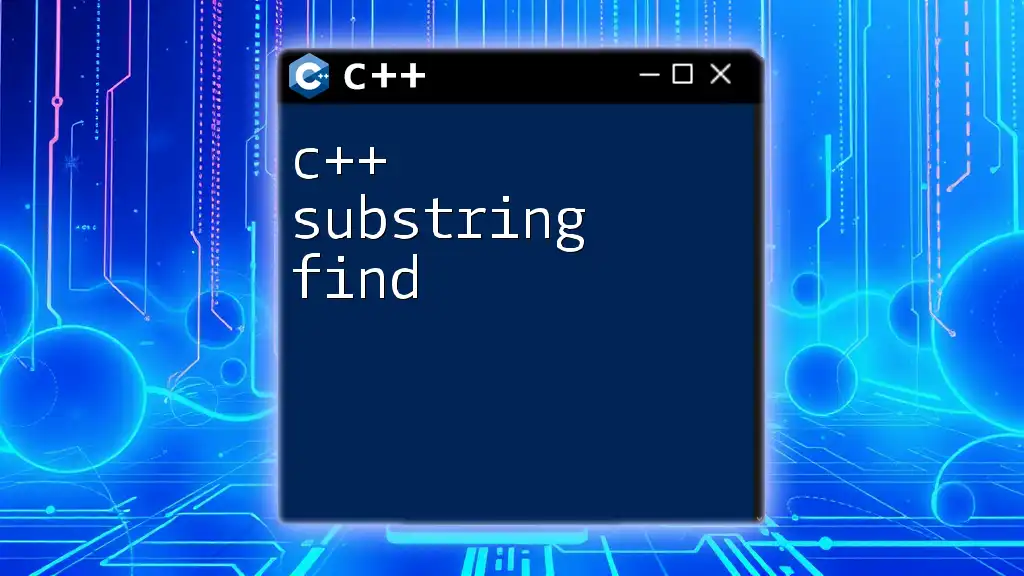
The C++ Substring Function
Introducing the `substr` Function
The `substr` function is a built-in method in the `std::string` class that enables developers to extract a portion of a string easily. Its basic syntax is as follows:
std::string substr(size_t pos = 0, size_t len = npos);
- `pos` is the starting position from which the substring extraction begins.
- `len` defines the length of the substring to be extracted. If omitted, it takes the substring from `pos` to the end of the string.
Parameters of the `substr` Function
Position Parameter
The `pos` parameter is critical as it lets you specify where to start extracting the substring. Remember that C++ uses zero-based indexing, meaning that the first character of the string is at index 0.
For example, consider the following code snippet:
std::string str = "Hello, World!";
std::string sub = str.substr(7); // Output: World!
Here, the `substr(7)` call instructs C++ to start extraction from the 8th character, resulting in "World!".
Length Parameter
The `len` parameter allows you to specify the length of the substring. For instance, if you want to extract only 5 characters starting from index 7, you would do:
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // Output: World
If you specify a length larger than what remains in the string, the function will simply return all characters from the specified position to the end of the string.
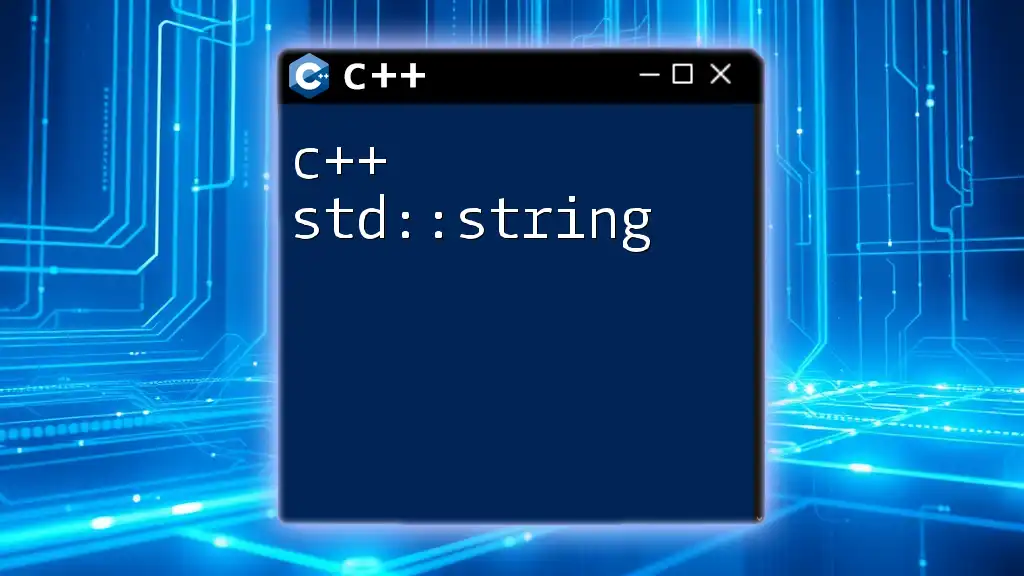
Practical Usage of Substrings in C++
Extracting Substrings from a String
The primary use case for the `substr` function is to extract fixed-length substrings. Here’s an example:
std::string str = "Hello, World!";
std::string sub = str.substr(0, 5); // Output: Hello
In this scenario, the function grabs the first 5 characters starting from the beginning of the string.
Dynamic Substring Extraction
A dynamic approach to extracting substrings allows user input to define the starting position and, optionally, the length. This flexibility makes your code more interactive. Here's a simple illustration:
std::string str = "Learning C++";
size_t pos;
std::cout << "Enter start position: ";
std::cin >> pos;
std::string sub = str.substr(pos);
This prompt enables the user to determine where to start extracting the substring.
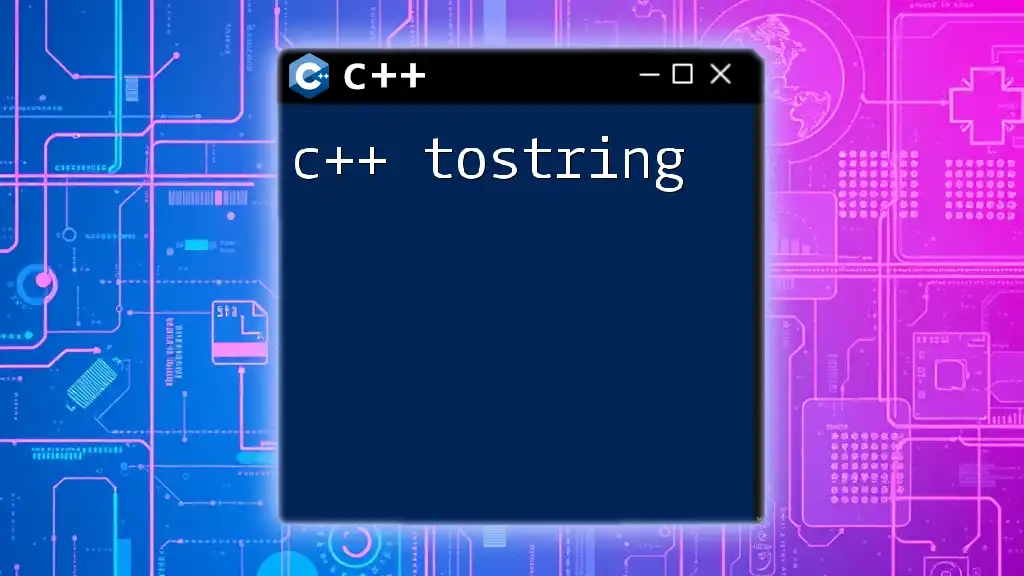
Advanced Substring Techniques
Finding Substrings Before Using `substr`
Before extracting a substring, it’s often useful to locate a specific substring. This can be achieved through the `find` method of the `std::string` class. Here’s how you can find a substring within a string and then extract it:
std::string str = "I love programming in C++!";
size_t pos = str.find("C++");
std::string sub = str.substr(pos, 3); // Output: C++
In this example, `str.find("C++")` returns the starting index of "C++", and then `substr` extracts it.
Using Ranges in Substring Extraction
Another advanced technique involves using iterators to extract a substring. This method allows for more flexible string manipulation. An example is shown below:
std::string str = "C++ Programming";
std::string sub(str.begin() + 0, str.begin() + 3); // Output: C++
This code extracts a substring using iterators, demonstrating the versatility of substring operations in C++.
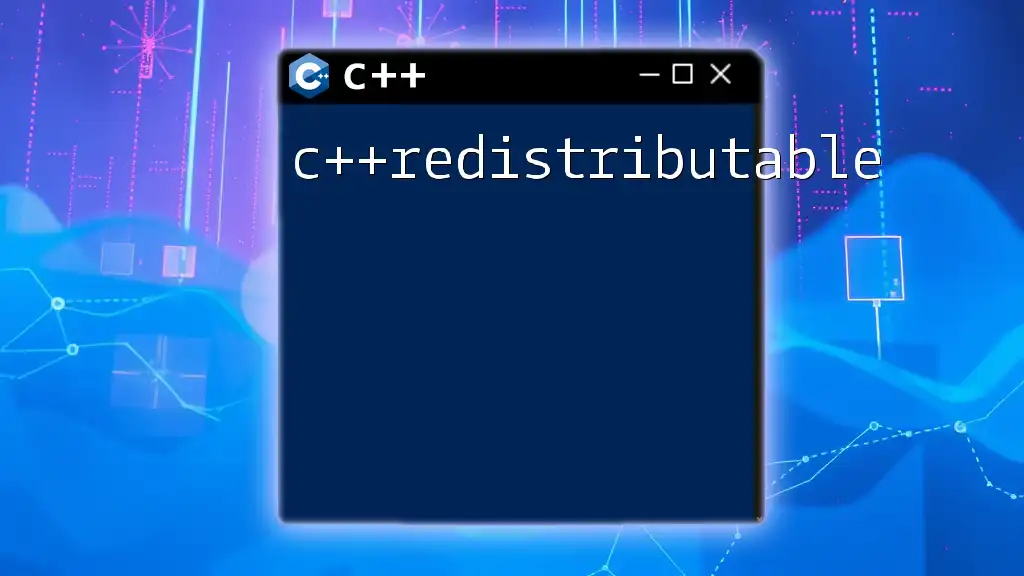
Common Use Cases for Substrings in C++
Text Parsing
Substrings are incredibly useful in text processing, especially when parsing structured data. For example, if you are working with CSV data, you can utilize substring operations to fetch specific fields:
std::string csvLine = "John,Doe,30";
std::string firstName = csvLine.substr(0, 4); // Output: John
String Comparison
Substrings also play a vital role in string comparison tasks. Here is an illustration where you compare the first few characters of two strings:
std::string str1 = "Hello, World!";
std::string str2 = "Hello, C++!";
if (str1.substr(0, 5) == str2.substr(0, 5)) {
std::cout << "Both strings start with Hello";
}
In this instance, the condition evaluates to true because both substrings match.
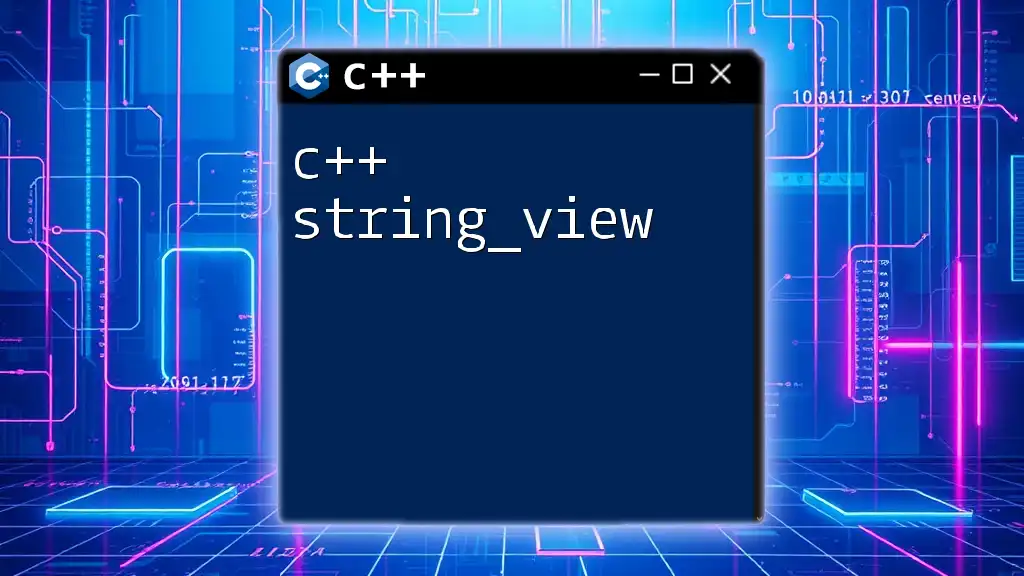
Benefits of Using the `substr` Function
Memory Management and Performance
Utilizing the `substr` function is not only efficient but also memory-friendly. The C++ `std::string` class handles memory management internally, reducing the risk of buffer overflows that are common with C-style strings. This makes your application safer and more robust.
Handling Edge Cases
One essential aspect to understand is how the `substr` function deals with out-of-bounds requests. If you attempt to access a position that doesn't exist, the function will throw an exception, allowing you to catch potential errors easily. Here’s an example of avoiding pitfalls:
std::string str = "Hello!";
try {
std::string sub = str.substr(10); // Out of bounds
} catch (const std::out_of_range& e) {
std::cout << "Requested substring out of range.";
}
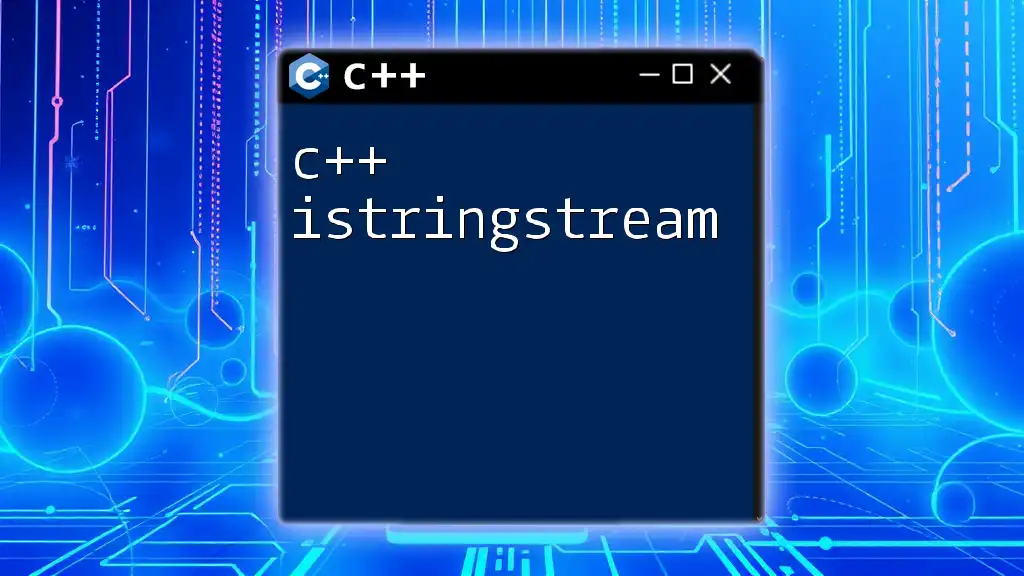
Conclusion
Recap of Key Points
Throughout this guide, we've explored the powerful `substr` function, its parameters, and its practical applications. From basic extraction to more advanced techniques involving searching and iterators, you've gained insight into how to effectively use C++ to manipulate strings.
Encouragement to Practice
To solidify your understanding, I encourage you to practice with different strings, length values, and positions. Experiment with extracting substrings from various data, and consider implementing these methods in your projects.
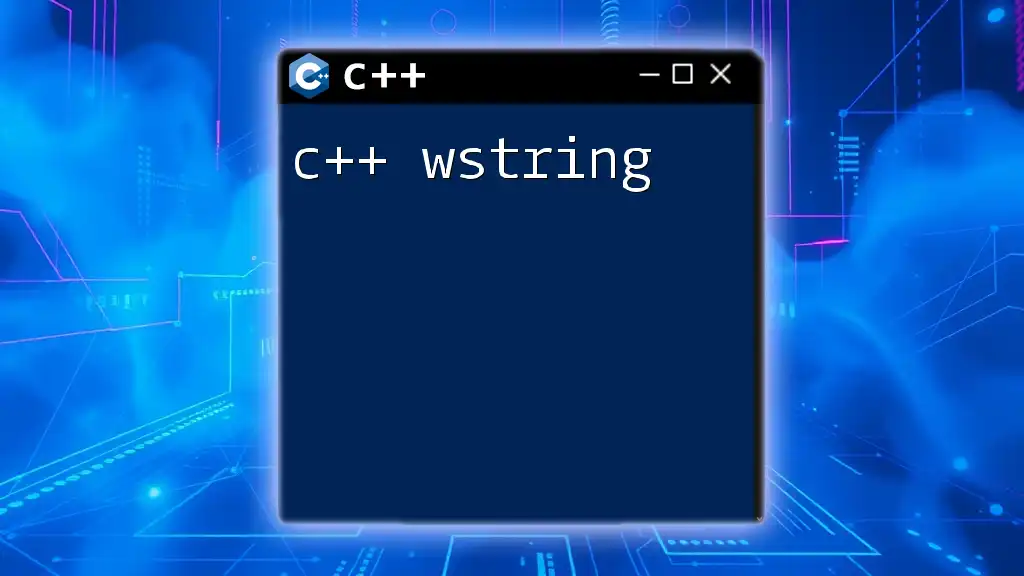
FAQs About C++ Substrings
Some common questions surrounding substring manipulations include how to efficiently extract multiple substrings or how to integrate them with other string functions. Remember that thorough practice and exploration are key to mastering substring operations in C++.
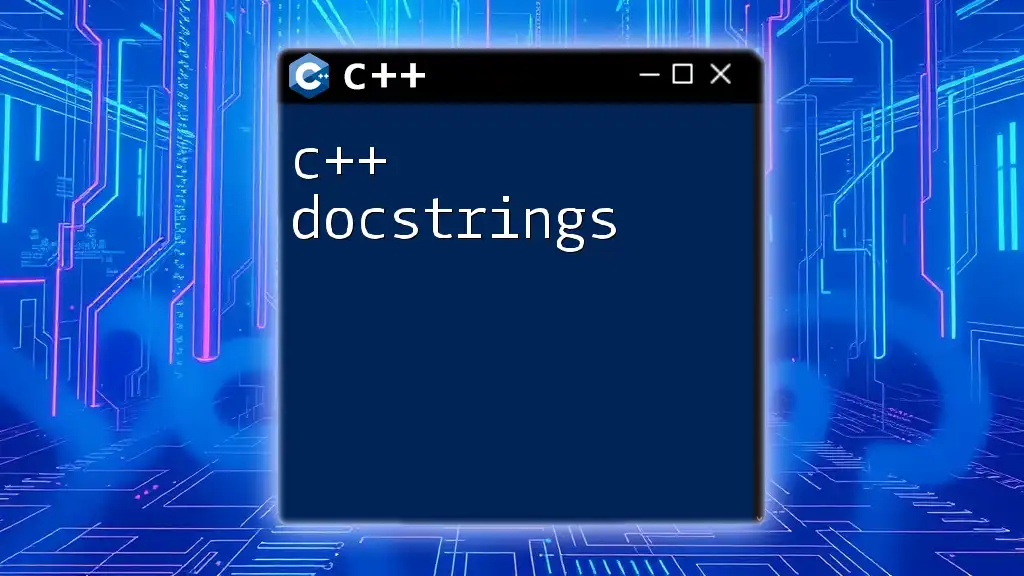
Code Snippet Gallery
The concluding section presents a collection of useful snippets for various substring operations, showcasing real-world applications that further enhance your understanding and proficiency in using the `substr` function in C++.