In C++, squaring a number can be easily accomplished by multiplying the number by itself. Here's a simple code snippet demonstrating how to square a number:
#include <iostream>
int main() {
int number = 5;
int squared = number * number;
std::cout << "The square of " << number << " is " << squared << std::endl;
return 0;
}
Understanding Squaring
Squaring is a fundamental mathematical operation that involves multiplying a number by itself. In programming, squaring a number is everywhere – from basic calculations to algorithms in computer graphics and data analysis.
For instance, squaring is applied in physics to compute the area, in finance for calculating interest, and often appears in statistical formulas. In C++, understanding how to square a number efficiently can greatly improve your coding skill set.
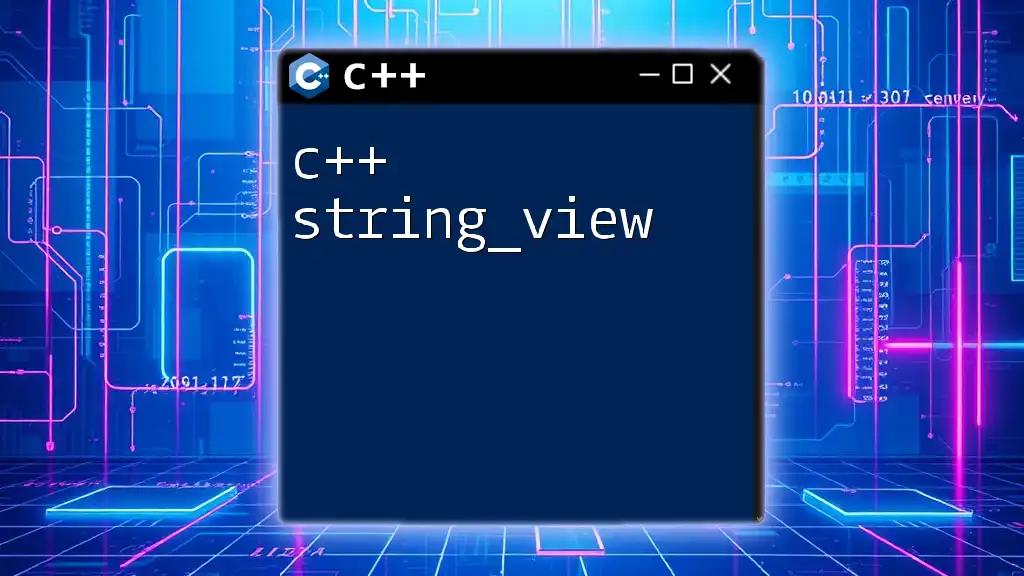
C++ Basics for Squaring
Syntax Essentials
Before diving into the concept of squaring, it’s essential to grasp the basics of C++ syntax. C++ is a statically typed, compiled programming language, meaning the type of a variable must be declared before use. Common data types include:
- int: Used for integers.
- float: Used for floating-point numbers, allowing for decimals.
- double: Offers double precision for floating-point representation.
Input and Output in C++
A significant aspect of programming involves interacting with the user. In C++, this is typically done using `cin` for input and `cout` for output. Understanding these commands lays the foundation for the rest of the article.
Here's a simple example of how to get user input and display output:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "You entered: " << num << endl;
return 0;
}
This snippet demonstrates taking an integer input and displaying it back to the user.

Methods to Square a Number in C++
Using Multiplication
The most straightforward way to square a number in C++ is by using multiplication. This method is not only the simplest but also the most efficient in terms of execution time for squaring a number.
Consider this example:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
int squared = num * num;
cout << "The square of " << num << " is " << squared << endl;
return 0;
}
In this code, the program prompts the user to enter a number. It then computes the square by multiplying the number by itself and displays the result. This approach is optimal for quick calculations, especially in scenarios where performance is crucial.
Using the `pow()` Function
C++ offers a built-in function in the `<cmath>` library called `pow()`, which allows for raising numbers to a power. To square a number, simply raise it to the power of two.
Here’s how to use it:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
int squared = pow(num, 2);
cout << "The square of " << num << " is " << squared << endl;
return 0;
}
While the `pow()` function is versatile (you can use it for any exponent), it comes with slight overhead compared to straightforward multiplication. It excels only in scenarios where ease of use and flexibility outweighs performance concerns.
Creating a Squaring Function
For better code organization and reusability, creating a dedicated function for squaring a number is highly recommended. Functions encapsulate logic and improve the maintainability of your code.
Consider this example:
#include <iostream>
using namespace std;
int square(int num) {
return num * num;
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "The square of " << num << " is " << square(num) << endl;
return 0;
}
In this example, the `square(int num)` function takes an integer as input and returns its square. The main function calls this squaring function, which simplifies future adjustments or modifications.
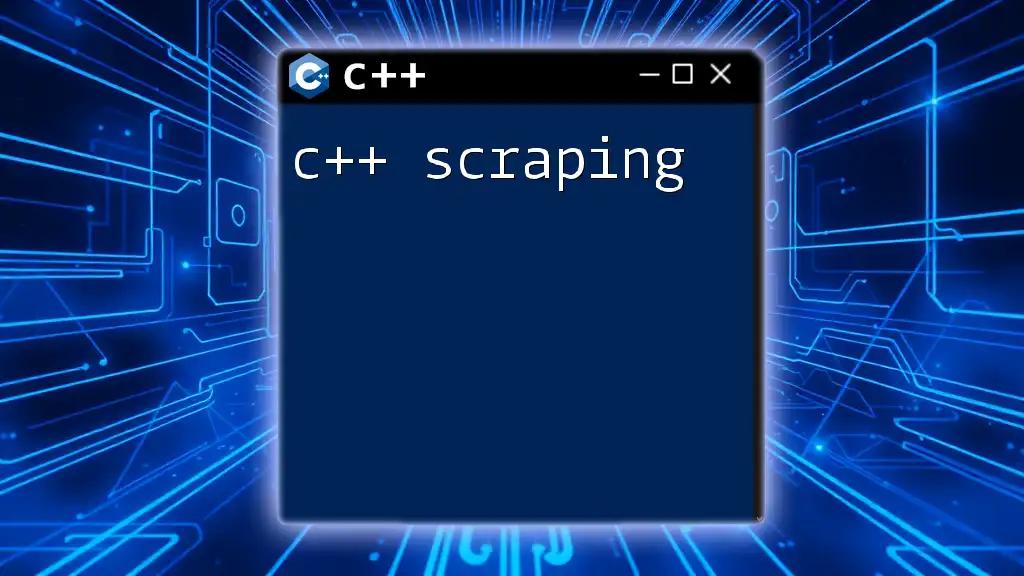
Optimizing Your Code
Using Inline Functions
For performance-critical applications, inline functions can provide significant speed advantages since they suggest to the compiler to insert the function's body directly into the calling code. This minimizes function call overhead.
Here’s how to create an inline squaring function:
#include <iostream>
using namespace std;
inline int square(int num) {
return num * num;
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "The square of " << num << " is " << square(num) << endl;
return 0;
}
By defining `square` as an inline function, you often gain performance benefits, especially in applications where this operation is called frequently.
Template Function for Squaring
Another advanced technique is using templates, which allow you to create a single function to handle multiple data types effortlessly. This versatility makes your code cleaner and more adaptable.
Here’s an example of a template function for squaring:
#include <iostream>
using namespace std;
template <typename T>
T square(T num) {
return num * num;
}
int main() {
int num_int = 3;
double num_double = 3.5;
cout << "The square of " << num_int << " is " << square(num_int) << endl;
cout << "The square of " << num_double << " is " << square(num_double) << endl;
return 0;
}
In this template function, you can square both integers and floating-point numbers without writing separate functions for each type. This is particularly useful in larger programs where code efficiency and clarity are paramount.
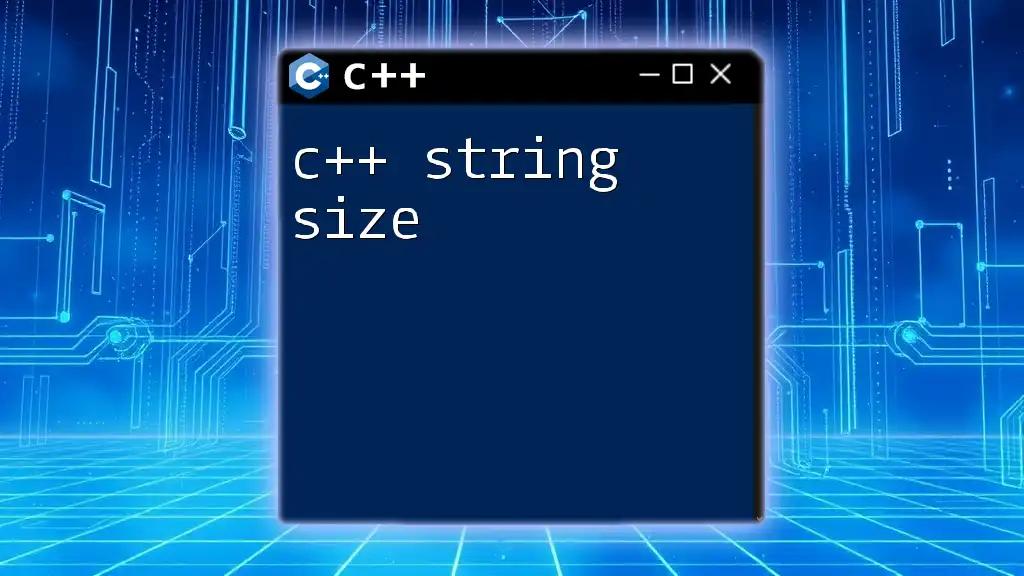
Common Errors and Debugging
Type Mismatches
One common mistake when squaring numbers in C++ relates to datatype mismatches. If an integer is expected, and a float is provided (or vice versa), you may encounter unexpected behavior or errors. Always ensure that the data types are compatible.
Using Libraries Incorrectly
Another common issue arises from incorrect usage of libraries. For instance, forgetting to include `<cmath>` when using the `pow()` function will lead to compilation errors. Here’s what you want to avoid:
// Incorrect without including <cmath>
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
int squared = pow(num, 2); // This will fail without <cmath>
cout << "The square of " << num << " is " << squared << endl;
return 0;
}
Ensure you include required headers to avoid such pitfalls.

Conclusion
In this guide on C++ squaring, we've explored various methods to square numbers effectively: simple multiplication, the `pow()` function, custom functions, inline, and template functions. Each method has its trade-offs in terms of performance and complexity.
As you tackle more advanced programming tasks, practicing these techniques will undoubtedly enhance your coding proficiency and prepare you for broader challenges within the C++ programming language.
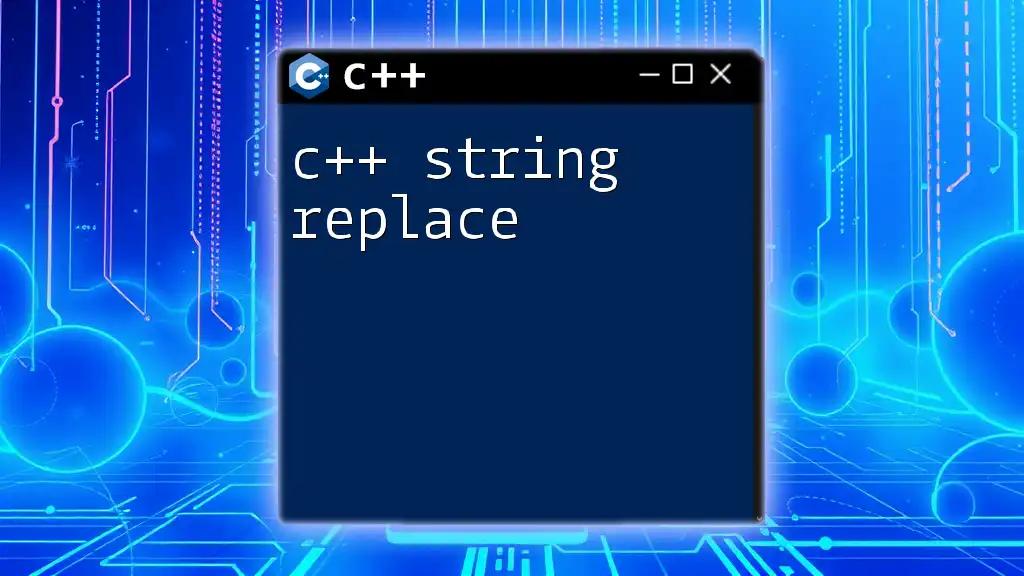
Additional Resources
For those looking to dive deeper into C++, we recommend exploring:
- Books on C++ fundamentals and advanced topics for a thorough grounding.
- Online coding platforms that offer interactive C++ challenges and exercises.
- The official C++ documentation for up-to-date information on libraries and features.

Call to Action
We invite you to subscribe to our newsletter for more concise and effective C++ tutorials. Share your insights and coding experiences with squaring numbers in C++, and let’s learn together!