In C++, you can append a character to a string using the `push_back()` method or the `+=` operator, allowing for dynamic string manipulation.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello";
// Using push_back
myString.push_back('!');
// Alternatively, using += operator
myString += '?';
std::cout << myString << std::endl; // Output: Hello!?
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string refers to a sequence of characters. The most common string type is `std::string`, which is part of the C++ Standard Library. Unlike C-style strings, which are arrays of characters terminated by a null character (`'\0'`), `std::string` provides a more flexible and powerful way to manipulate strings. Using `std::string` simplifies many tasks related to string handling, such as memory management and manipulation.
Why You Might Need to Append Characters
Appending characters to strings is a fundamental operation in programming, enabling developers to dynamically build strings based on user input, concatenate messages, or format output. For example, when creating user-friendly messages or logging information, you may need to construct strings on the fly, which often involves appending characters or other strings.
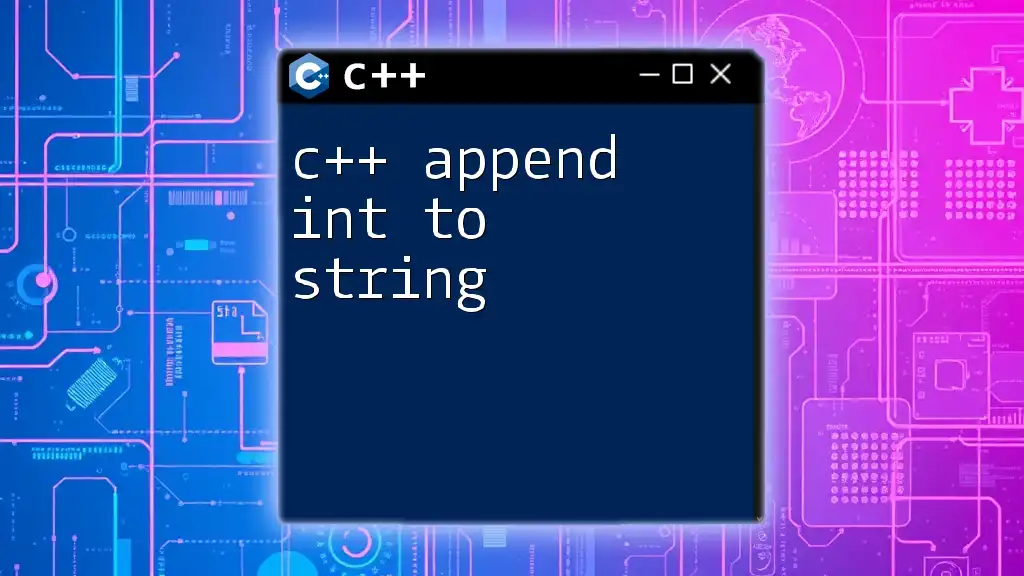
Basics of Appending Characters
Using the `+` Operator
One of the most straightforward ways to append characters to a string in C++ is by utilizing the `+` operator. This operator creates a new string that combines the original string with the character being added.
Example:
std::string str = "Hello";
str = str + '!'; // str now contains "Hello!"
This method is simple but creates a new string every time, which can be less efficient in performance-sensitive applications.
Using the `+=` Operator
The `+=` operator behaves similarly to the `+` operator but is often preferred for its simplicity and clarity when appending characters directly to an existing string.
Example:
std::string str = "World";
str += '!'; // str now contains "World!"
Using `+=`, you can easily append characters without reassigning the variable, making your code cleaner and more readable.
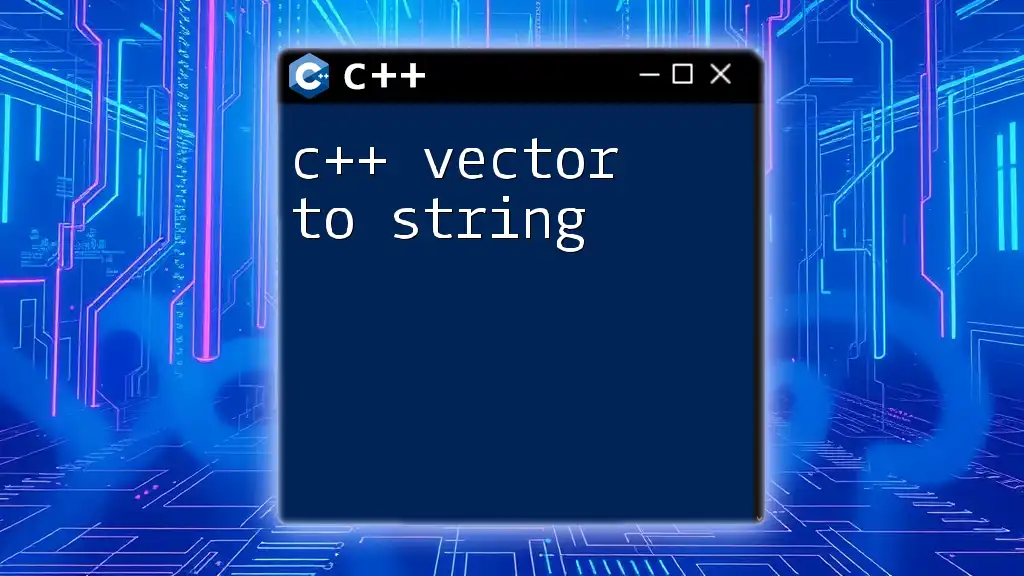
Methods to Add Char to String in C++
Using `std::string::push_back()`
The `push_back()` method is a member function of the `std::string` class designed specifically for adding a single character at the end of the string. This method is particularly efficient because it doesn’t create a new string; instead, it directly modifies the existing one.
Example:
std::string str = "Happy";
str.push_back(' '); // str now contains "Happy "
str.push_back('C'); // str now contains "Happy C"
str.push_back('+'); // str now contains "Happy C+"
str.push_back('+'); // str now contains "Happy C++"
This method is ideal for scenarios where you need to add single characters repeatedly.
Using `std::string::append()`
The `append()` method provides further flexibility by allowing you to add multiple characters at once or append an entire string. With this method, you can specify how many copies of the character you want to append.
Example:
std::string str = "Goodbye";
str.append(1, '!'); // str now contains "Goodbye!"
In this example, `append(1, '!')` adds a single exclamation mark to the end of the existing string.
Using the `insert()` Method
If you want to insert a character at a specific position rather than just appending it to the end, you can utilize the `insert()` method. This design gives you more control over where the new character will appear within the string.
Example:
std::string str = "C++ Programming";
str.insert(3, 1, '!'); // str now contains "C!++ Programming"
Here, `insert(3, 1, '!')` adds an exclamation mark at index 3 of the string.
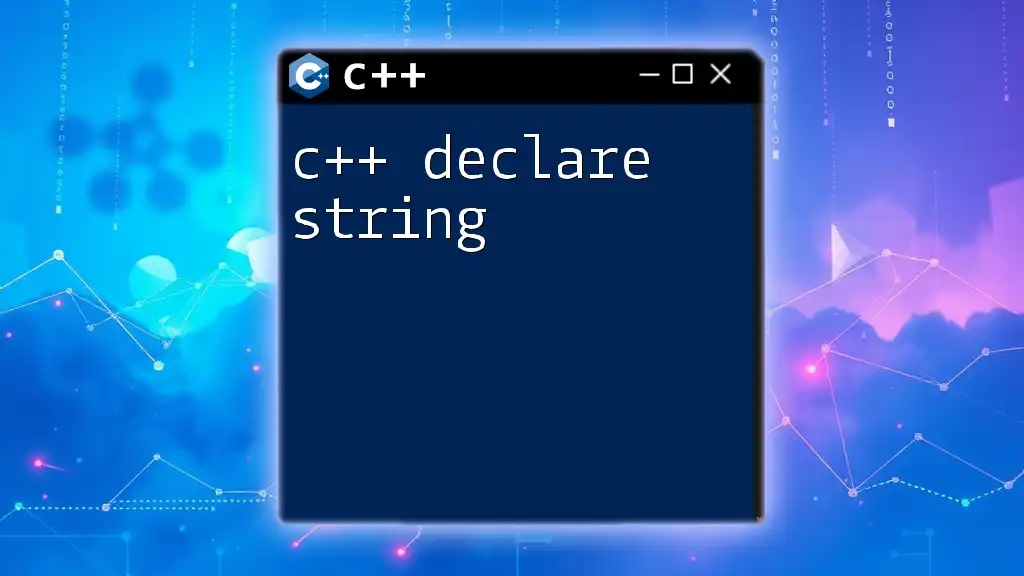
Combining Methods for Complex Scenarios
Appending Multiple Characters
You can combine methods to append multiple characters efficiently. The `append()` method can also be utilized to add multiple instances of a character in a single call.
Example:
std::string str = "C++";
str.append(3, '!'); // str now contains "C+++!!!"
This demonstrates that you can append three exclamation marks at once with a single function call.
Appending a Character Array
`std::string` can also handle entire character arrays or string literals, making it easy to build more complex strings.
Example:
std::string str = "Code";
str.append(" is fun!"); // str now contains "Code is fun!"
This method allows you to quickly concatenate longer strings without needing to manipulate them character by character.
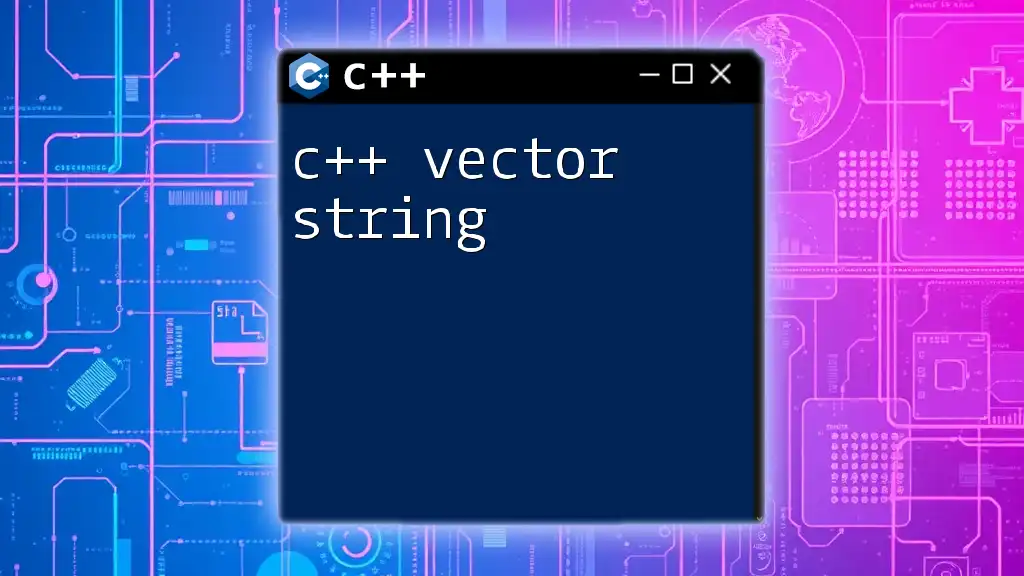
Performance Considerations
Understanding Memory Management
C++ strings manage memory dynamically, automatically resizing as needed when characters are appended. However, frequent appending can lead to performance overhead due to memory reallocations. It is essential to keep in mind how the standard library optimizes these operations.
Alternative Data Structures for Performance
In performance-critical applications, you might consider using `std::vector<char>` or other data structures instead of `std::string`. While `std::string` is generally more convenient, using a vector can avoid some overhead associated with constant resizing, especially when the total size is known in advance.
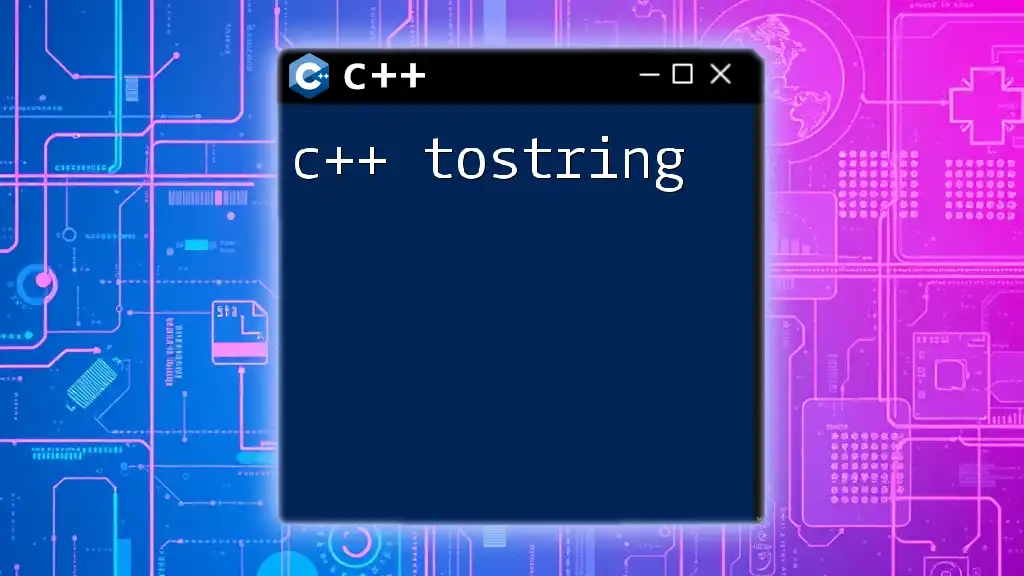
Common Pitfalls to Avoid
Null Character Issues
When working with C-style strings or character arrays, be cautious of null characters (`'\0'`). These can unintentionally terminate your strings if not handled properly. In C++, however, `std::string` eliminates these concerns by managing termination automatically.
Memory Allocation Errors
Improper handling of string operations can lead to memory allocation errors. If you frequently append characters, be mindful of the string's capacity and potential memory reallocations; this ensures that your program runs efficiently without exhausting resources.
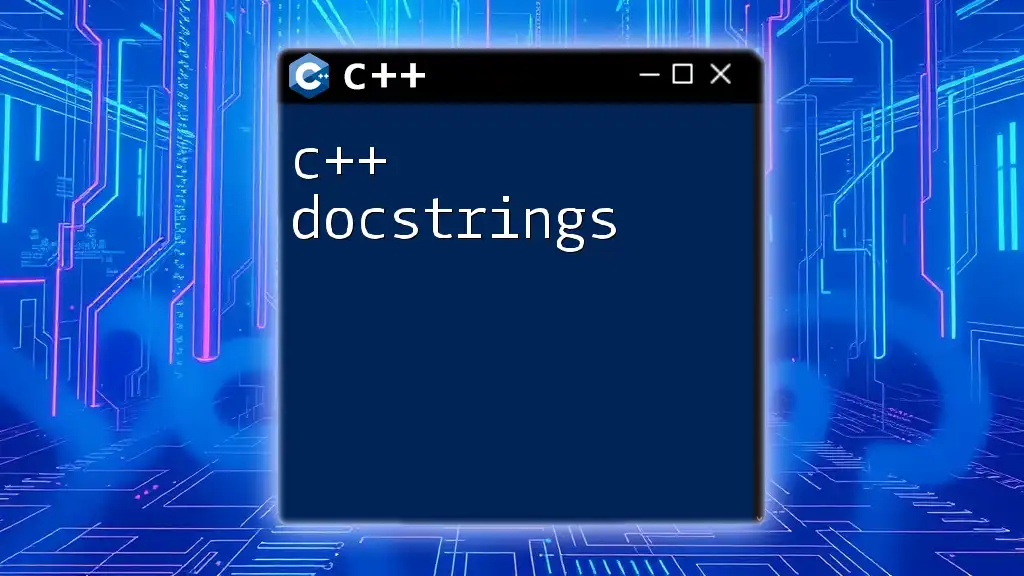
Conclusion
In this guide, we've explored various methods for appending characters to strings in C++. From simple methods like `push_back()` and `+=` to more complex techniques using `insert()` and `append()`, C++ offers robust tools for string manipulation.
Through practice and experimentation with these techniques, you can enhance your programming skills and develop efficient code that handles strings with ease.
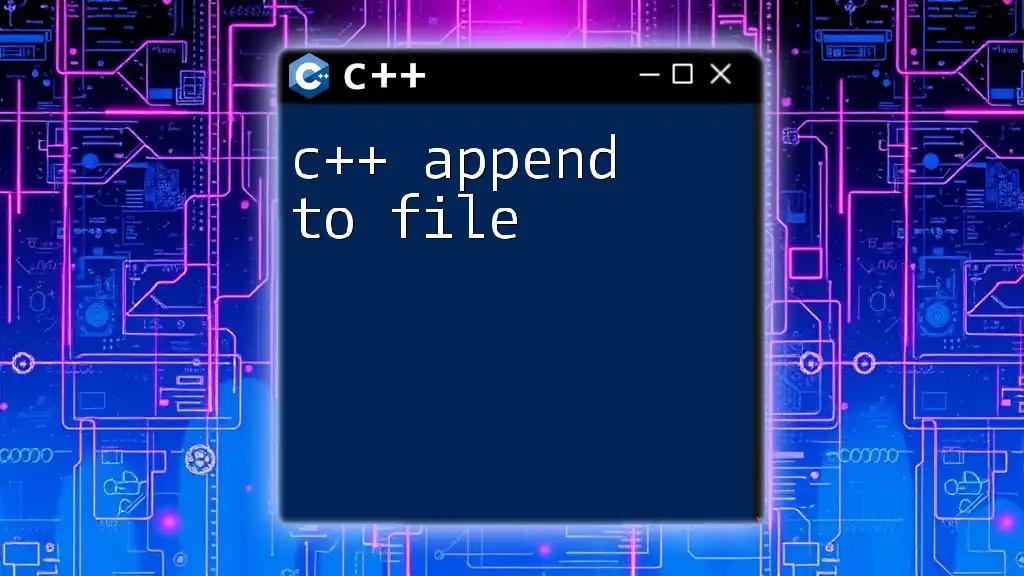
Further Resources
Recommended Readings and References
- C++ Standard Library documentation on `std::string`
- Books on C++ programming, such as "C++ Primer" by Lippman, Lajoie, and Moo
- Online tutorials to strengthen your understanding of C++ string manipulation techniques
Engage with Your Audience
Feel free to leave comments, questions, or share your experiences with string manipulation in C++. For more tutorials and programming tips, follow our company for the latest content and updates!