In C++, the percent sign `%` is used as the modulus operator, which returns the remainder of a division operation between two integers.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int remainder = a % b;
std::cout << "The remainder of " << a << " divided by " << b << " is " << remainder << std::endl;
return 0;
}
Understanding the Percent Sign in C++
The Role of the Percent Sign
In C++, the percent sign ("%") is a versatile symbol used in various contexts. Its significance can range from indicating mathematical operations to serving as a tool for formatting outputs. This multi-faceted nature makes it essential for programmers to grasp its multiple uses effectively.
Overview of Different Usages
Mathematical Operations
One of the most common uses of the percent sign in C++ is as the modulo operator. This operator enables you to perform division and obtain the remainder, providing an effective means of working with integers.
Formatting Output
In formatted output functions, such as `printf`, the percent sign serves as a placeholder for variables. It allows developers to dynamically insert values into strings which improves readability and functionality.
Preprocessor Directives
In preprocessor directives, especially when using `#define`, the percent sign can also be part of macros, allowing for more concise code and clearer logic.
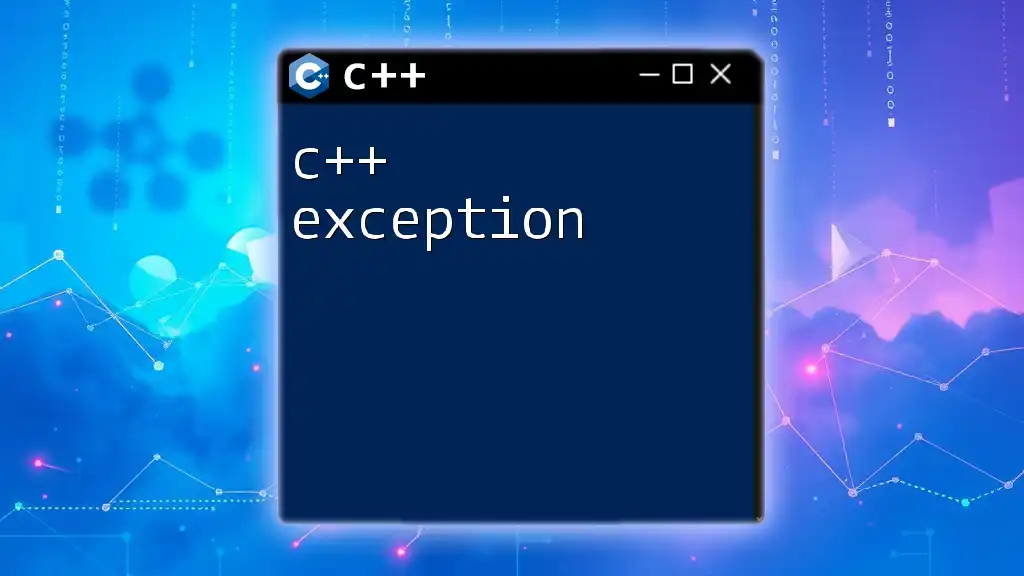
The Modulo Operator: `%`
What is the Modulo Operator?
The modulo operator (%) calculates the remainder of division between two integers. In a broader sense, it tells us how much is left after dividing up one quantity into equal parts. Understanding this operator is crucial, especially in loops and conditional statements.
How to Use the Modulo Operator
The syntax for using the modulo operator involves placing it between two integers. Here’s a simple example:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b; // result is 1
std::cout << "10 % 3 = " << result << std::endl;
return 0;
}
In this example, when 10 is divided by 3, the quotient is 3 with a remainder of 1. Hence, `10 % 3` returns 1, and this is displayed in the output.
Common Use Cases for Modulo
Even or Odd Checking
A prevalent use of the modulo operator is to determine whether a number is even or odd. An even number will yield a remainder of 0 when divided by 2, while an odd number will not.
#include <iostream>
int main() {
int num = 7;
if (num % 2 == 0) {
std::cout << num << " is even." << std::endl;
} else {
std::cout << num << " is odd." << std::endl; // This will be executed
}
return 0;
}
In this example, because 7 divided by 2 results in a remainder of 1, the output correctly indicates that 7 is odd.
Cycles and Patterns
The modulo operator is also beneficial for cycling through arrays or lists. For instance, you can loop through an array of fixed size using modulo to reset the index.
#include <iostream>
int main() {
const int size = 3;
int numbers[size] = {10, 20, 30};
for (int i = 0; i < 10; ++i) {
std::cout << numbers[i % size] << std::endl; // Cycles through 10 times
}
return 0;
}
This code outputs the elements 10, 20, and 30 repeatedly, demonstrating how to use the percent sign for controlled iteration.
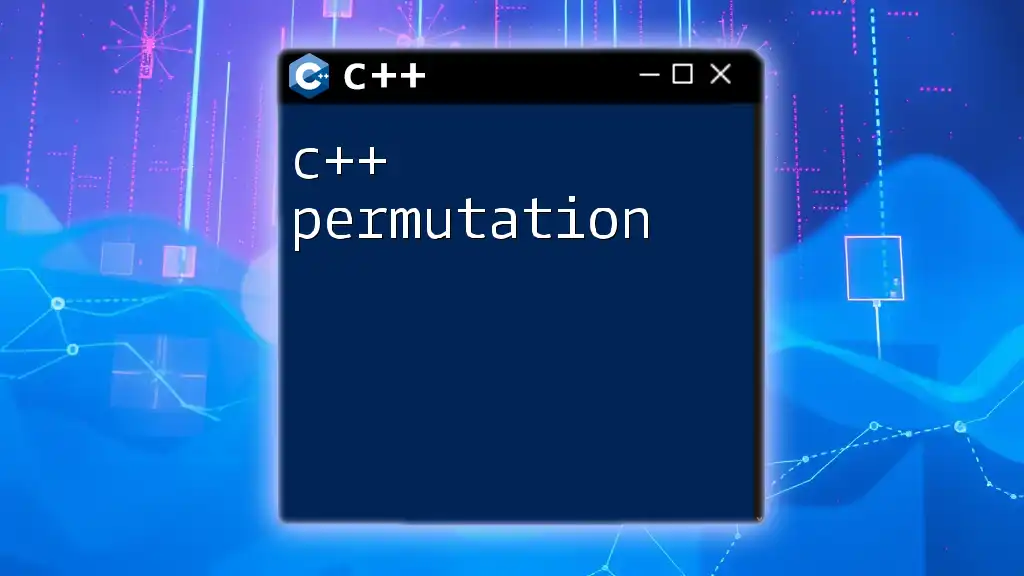
Formatting Output with Percent Sign
Introduction to `printf` and Percent Sign Usage
C++ includes various ways to output formatted text, and one notable method is through the `printf` function from the C standard library. The percent sign acts as a crucial marker for format specifiers, enabling the inclusion of variable data directly into strings.
Common Format Specifiers
Understanding the mapping of format specifiers is essential. The percent sign is used as follows:
- `%d` for printing integers.
- `%f` for floating-point numbers.
- `%%` to print the percent sign itself.
Example Code Snippet
Here’s a code snippet illustrating how to use the percent sign in formatted output:
#include <cstdio>
int main() {
int age = 25;
float score = 85.5;
printf("Age: %d, Score: %.1f%%\n", age, score); // Prints: Age: 25, Score: 85.5%
return 0;
}
In this example, the output clearly indicates age and score, utilizing the correct format specifiers. Note how `%.1f%%` prints out the score as a floating-point number followed by a percent sign.
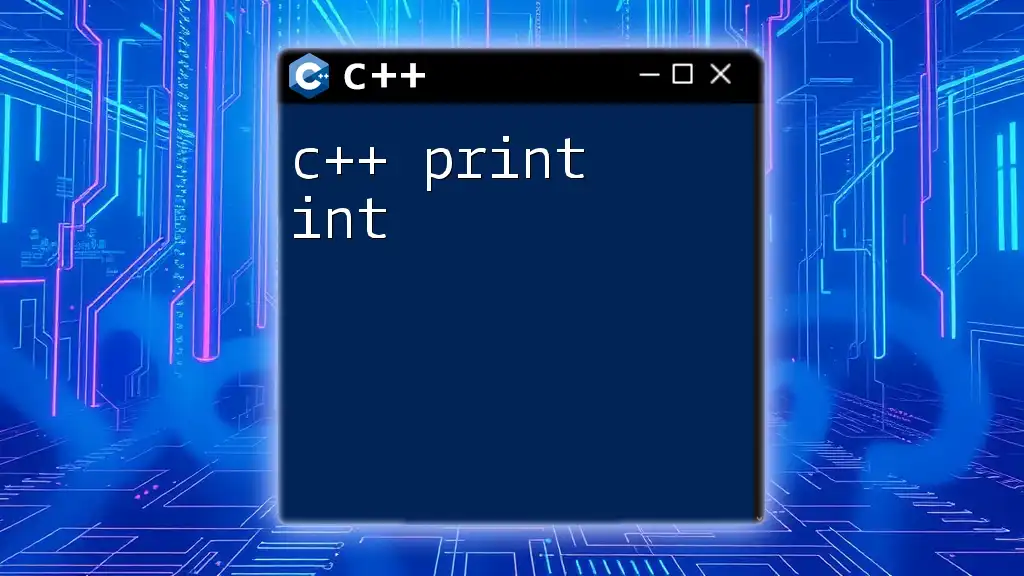
Preprocessor Directives: `#define` and Macros
Introduction to Macros in C++
Macros in C++ are defined using the `#define` directive, allowing for code substitution before compilation. This feature enables programmers to write more concise and understandable code, often improving maintainability.
The Percent Sign in Macro Definitions
The percent sign can be part of macro definitions, enabling you to create reusable logic more easily. For example, you can create a macro to calculate percentages.
Example Code Snippet for Macros
Here is an example that defines a couple of useful macros:
#include <iostream>
#define SQUARE(x) (x * x)
#define PERCENT(x, y) ((x) / (y) * 100)
int main() {
std::cout << "Square of 5: " << SQUARE(5) << std::endl; // Square of 5: 25
std::cout << "Percentage: " << PERCENT(30, 50) << "%" << std::endl; // Percentage: 60%
return 0;
}
In this snippet, `SQUARE(5)` results in 25 and `PERCENT(30, 50)` outputs 60%, effectively showcasing how the percent sign works within macros.
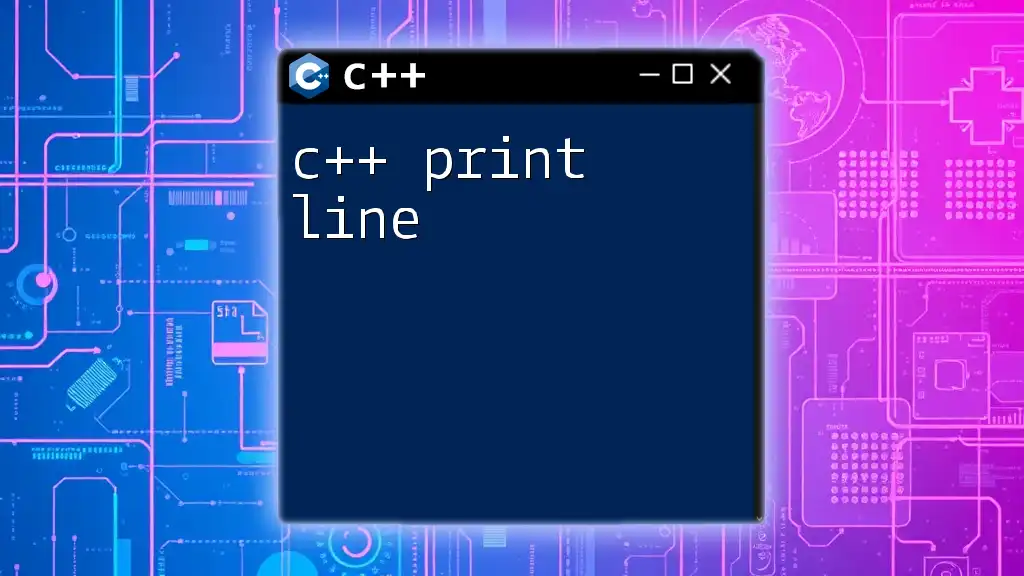
Common Pitfalls and Best Practices
Mistakes to Avoid with the Percent Sign
When using the modulo operator, one common pitfall is misunderstanding how it behaves with negative numbers. For instance, `-1 % 2` results in -1, not 1. Such behaviors can lead to unexpected results in code design, especially in loops.
When utilizing formatted output, forgetfulness in using the correct format specifier can lead to compilation errors or unintended outputs.
Best Practices
To avoid confusion or errors, always use parentheses around expressions within macro definitions to ensure correct evaluation order. This clarity can prevent bugs from creeping into your code. Furthermore, give meaningful names to your macros to enhance readability and maintainability.
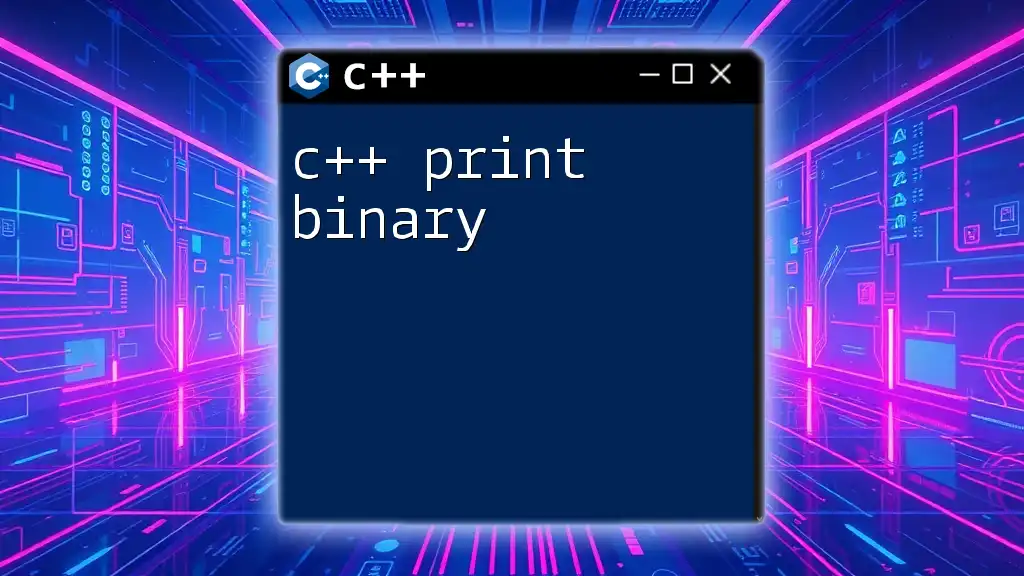
Conclusion
The C++ percent sign is more than just a symbol; it serves crucial roles in mathematical operations, formatted outputs, and code definitions. Mastering its various applications not only enhances coding efficiency but also fosters clearer logic within your programs. As you become familiar with these concepts, you’ll appreciate the versatility and power inherent in C++. Whether you're using it to determine patterns, format data, or simplify your code, the percent sign will invariably prove to be an invaluable ally in your programming toolkit.
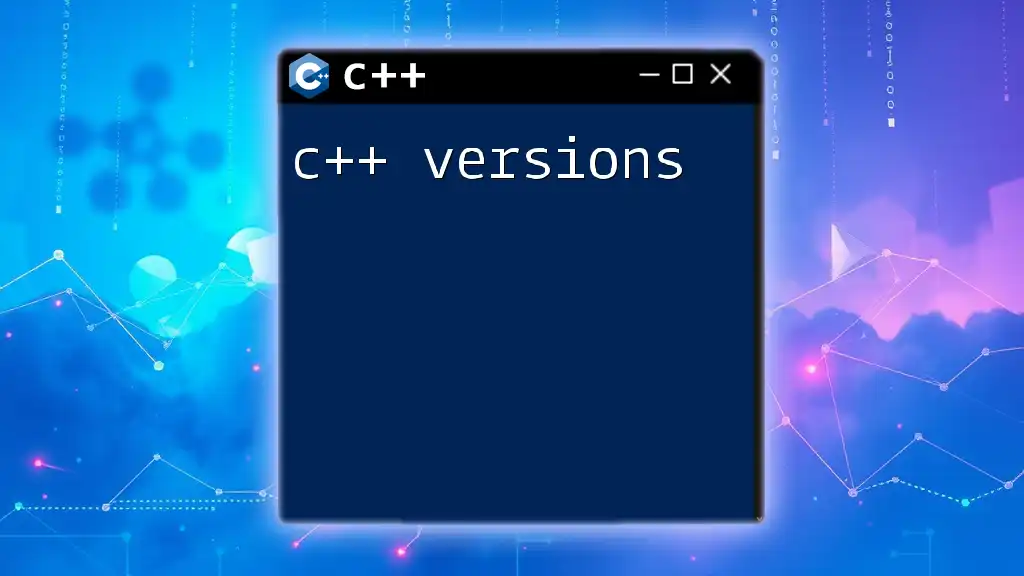
Additional Resources
For further understanding of the C++ percent sign and its applications, refer to the official C++ documentation and explore supplementary reading materials and practice exercises available online. This continuing exploration will help cement your skills in C++ programming, particularly around the effective use of operators and macros.