In C++, you can use the `std::cout` statement to print a line of text to the console, as shown in the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Output Streams in C++
What is an Output Stream?
In C++, an output stream is a sequence of characters sent to a destination, usually the console. This concept is fundamental as it enables programmers to communicate results, statuses, and other information to users. Output streams encapsulate the complexity of managing output, giving developers a simple interface to interact with.
The Standard Output Stream
The standard output stream is represented by the `cout` object found in the `<iostream>` library. It serves as the default channel for output, typically visible in the console or terminal. Understanding the distinction between standard output and error output (accessible via `cerr`) is crucial for effective debugging and logging in C++ applications.
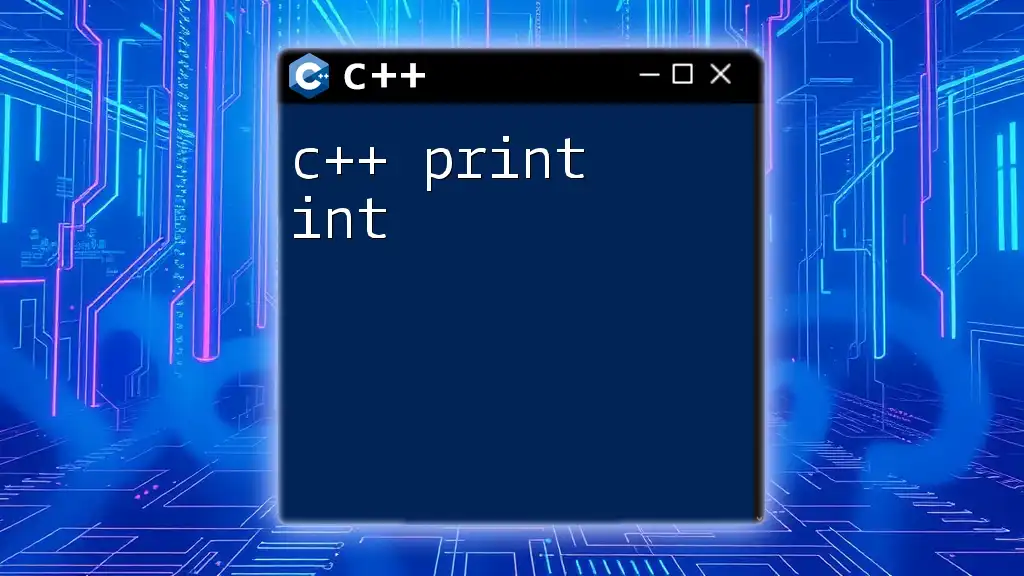
Basic Syntax of `cout`
Using the `<<` Operator
The heart of the C++ print line functionality lies in the syntax of the `cout` stream. The `<<` operator, known as the stream insertion operator, is used to send data to the output stream. It allows you to chain multiple outputs seamlessly.
Example Code
Here’s a simple example that demonstrates the use of `cout`:
#include <iostream>
int main() {
std::cout << "Hello, C++ World!" << std::endl;
return 0;
}
Explanation of Example:
- `#include <iostream>` includes the necessary library.
- `std::cout` is the standard output stream.
- The message "Hello, C++ World!" is printed to the console, followed by a line break due to `std::endl`.
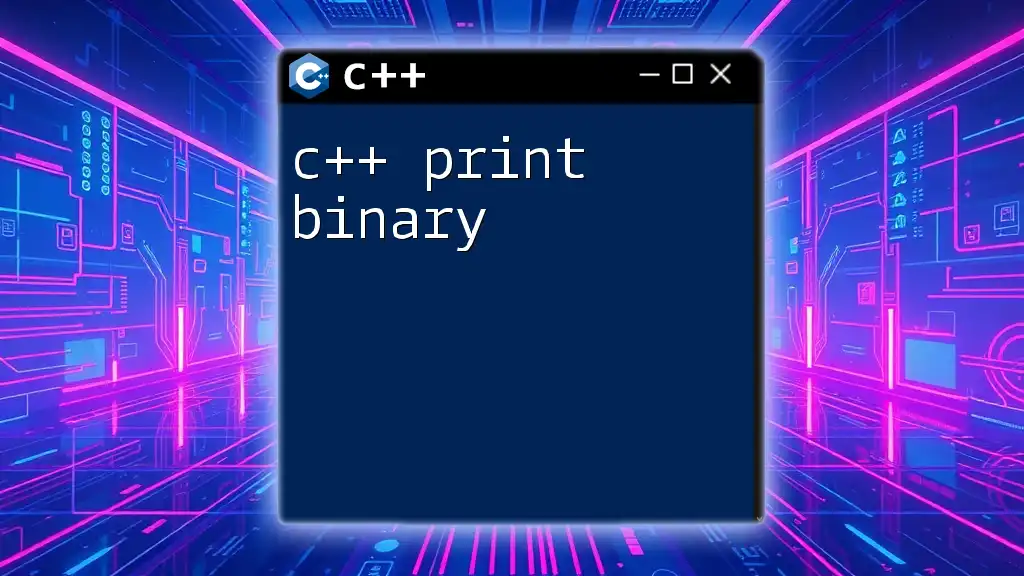
Formatting Output
Line Breaks with `std::endl`
The `std::endl` manipulator plays a vital role by inserting a newline character and flushing the output buffer. This means that the content is immediately sent to the output device, which can be useful for real-time user feedback.
Consider this example:
std::cout << "First line" << std::endl;
std::cout << "Second line\n";
In this case:
- The first line utilizes `std::endl`, ensuring that "First line" is fully flushed to the output.
- The second line uses `\n`, which introduces a newline without flushing the buffer.
Using `\n` for Simplicity
While `std::endl` is useful, using `\n` can be more efficient when line flushing is unnecessary. For example:
std::cout << "Line one\nLine two\nLine three\n";
This prints the lines without flushing the output buffer after each line, which can enhance performance in many situations.
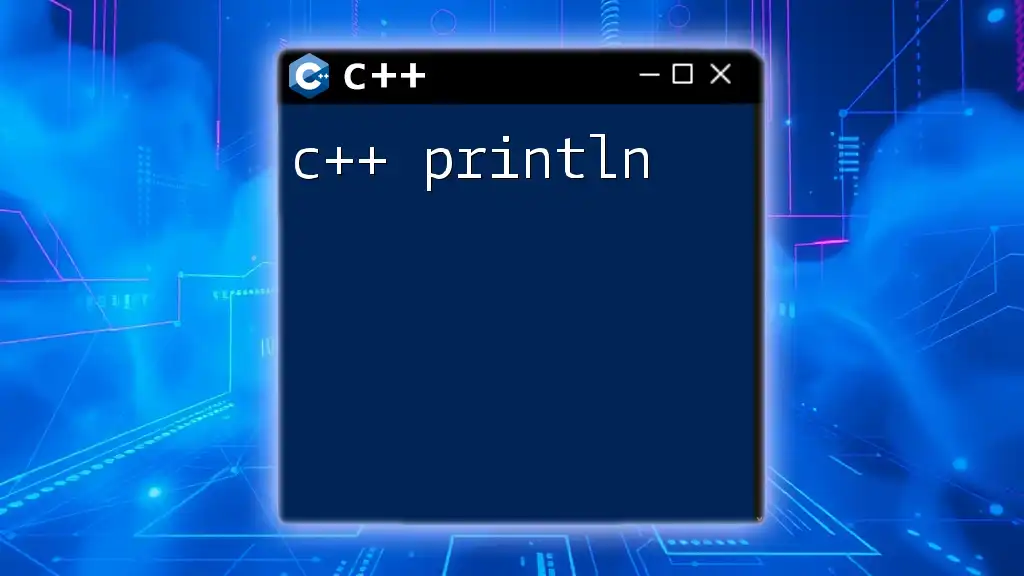
Printing Different Data Types
C++ allows for the seamless printing of various data types with the same `cout` statement.
Printing Integers
Here’s how you can effortlessly output an integer:
int num = 42;
std::cout << "The number is: " << num << std::endl;
The above code results in "The number is: 42" being displayed on the console.
Printing Floats and Doubles
C++ manages floating-point numbers slightly differently, particularly with regard to precision. It’s essential to be mindful of formatting:
double pi = 3.14159;
std::cout << "Value of Pi: " << pi << std::endl;
While the code outputs "Value of Pi: 3.14159", formatting control may be necessary for precise requirements.
Printing Strings
Both C-style strings and C++ strings can be printed using `cout`. A demonstration includes:
const char* cStr = "Hello, C-String!";
std::string cppStr = "Hello, C++ String!";
std::cout << cStr << std::endl;
std::cout << cppStr << std::endl;
This code outputs both string types correctly, showcasing the flexibility of `cout`.
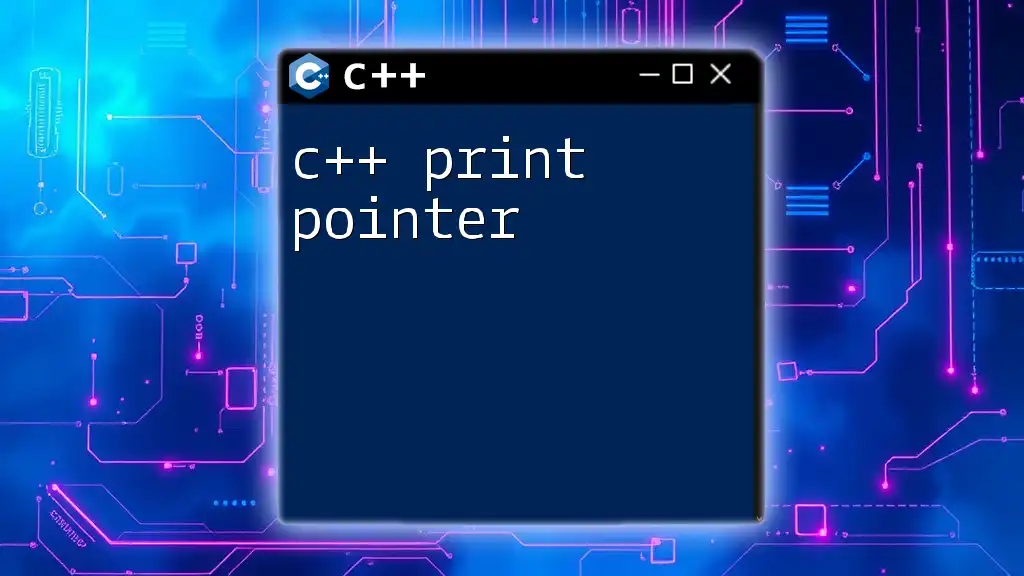
Advanced `cout` Features
Manipulators for Formatting
C++ offers manipulators that allow you to control the appearance of output. Some common examples are `std::setw` for setting width and `std::setprecision` for controlling decimal places.
Here’s how to use `std::setw` for aligned output:
#include <iomanip>
std::cout << std::setw(10) << "Column1" << std::setw(10) << "Column2" << std::endl;
Chaining Output Statements
A novel feature of `cout` is the ability to chain multiple output statements, thus enhancing readability:
std::cout << "Hello" << ", " << "World!" << std::endl;
This method of concatenation allows for compact and straightforward code, making it easier to maintain.
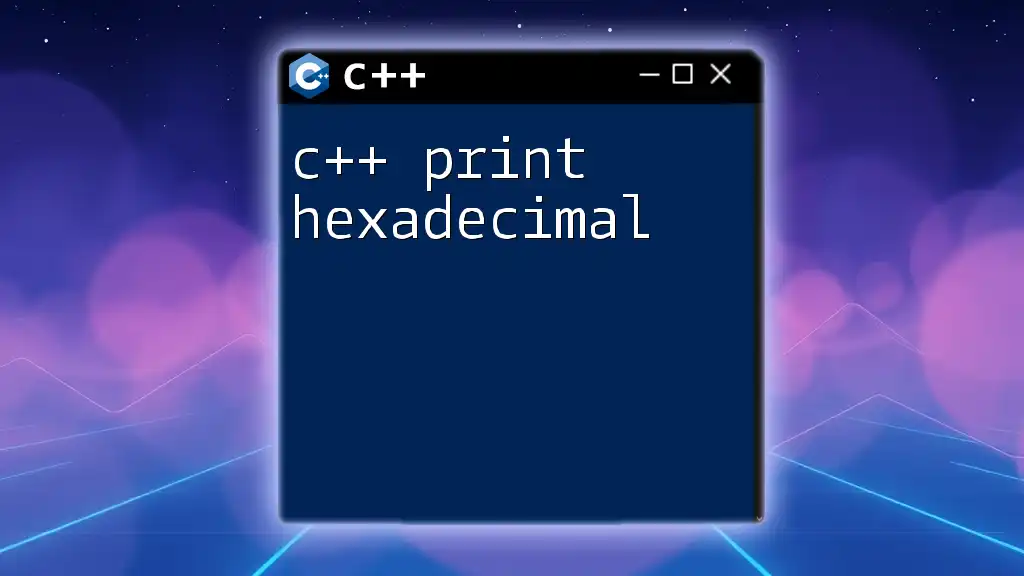
Common Errors and Debugging Tips
Common Mistakes with `cout`
One of the most frequent errors novices encounter is failing to include the necessary header file. Omitting `#include <iostream>` leads to compilation failure. Always ensure that you include the required libraries to avoid this pitfall.
Best Practices for Effective Output
When working with `cout`, following best practices can significantly improve the quality of your output:
- Use consistent formatting: Maintain a uniform style across your output for clarity.
- Comment your code: Clear annotations explaining what each part does can be beneficial for future reference.
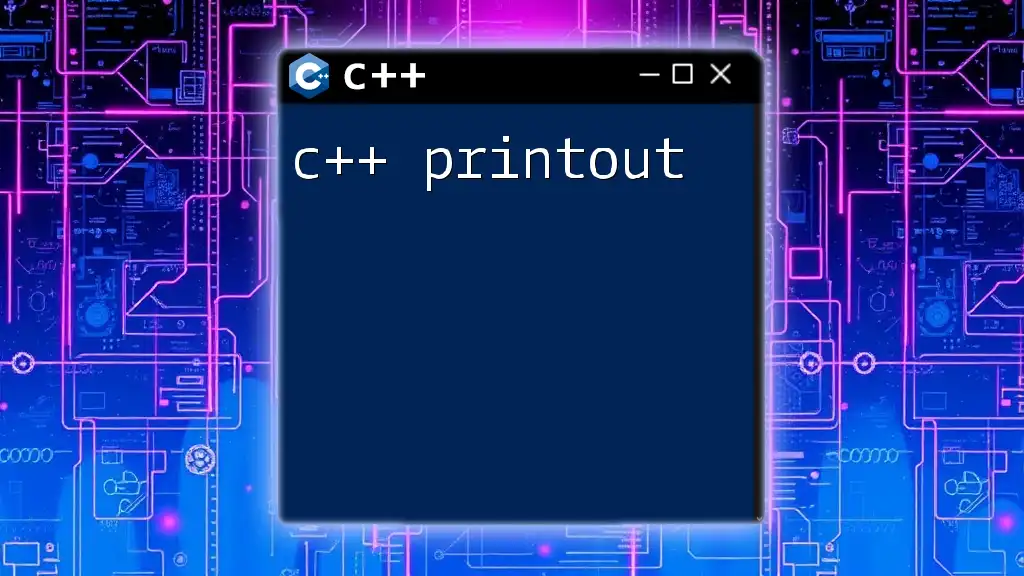
Conclusion
In this guide to C++ print line, we delved into the workings of `cout`, exploring its syntactic structure, formatting options, and functionalities with various data types. The versatility and simplicity of `cout` make it indispensable for effective communication in C++ programming. As you continue to practice, you will discover a deeper understanding and appreciation for C++ output streams.