C++ inline assembly allows you to insert assembly language instructions directly within C++ code for performance optimization and low-level hardware control.
Here's a simple example of inline assembly in C++:
#include <iostream>
int main() {
int a = 5, b = 10, result;
asm("addl %1, %2; movl %2, %0"
: "=r"(result) // output
: "r"(a), "r"(b) // inputs
);
std::cout << "The sum of " << a << " and " << b << " is " << result << std::endl;
return 0;
}
Understanding C++ Inline Assembly Syntax
C++ inline asm is a powerful tool that allows programmers to embed assembly language code directly within their C++ code. This integration provides close control over the hardware and can optimize performance for critical applications.
Basic Syntax Structure
The syntax for inline assembly is straightforward but requires attention to detail. The general structure of an inline ASM statement looks like this:
asm (assembly code : output operands : input operands : clobbered registers);
In this syntax:
- Assembly Code is where you write the actual assembly instructions.
- Output Operands specify what values the assembly code will produce.
- Input Operands indicate what values the assembly code should take in.
- Clobbered Registers list registers that may have been altered.
The Role of the `asm` Keyword
The `asm` keyword is essential in defining the boundaries of inline assembly in your C++ code. Here's how it works:
asm ("assembly instructions");
This tells the compiler that the following string contains assembly code. Understanding how the compiler interprets this code is crucial for mixing C++ and assembly effectively.
Writing Your First Inline ASM Statement
Setting up your development environment is the first step towards leveraging C++ inline asm. Ensure you have a suitable C++ compiler, such as GCC or Clang, that supports inline assembly.
Example of a Simple Inline ASM Statement
Consider the following example where we add two integers using C++ inline asm:
int add(int a, int b) {
int result;
asm ("addl %%ebx, %%eax;"
: "=a"(result)
: "a"(a), "b"(b));
return result;
}
Here, the `addl` instruction adds the values in registers `eax` and `ebx`. The `result` variable is defined as an output operand, and `a` and `b` are passed as input operands. Understanding how each part of the syntax contributes to the performance and functionality is vital for effective usage.
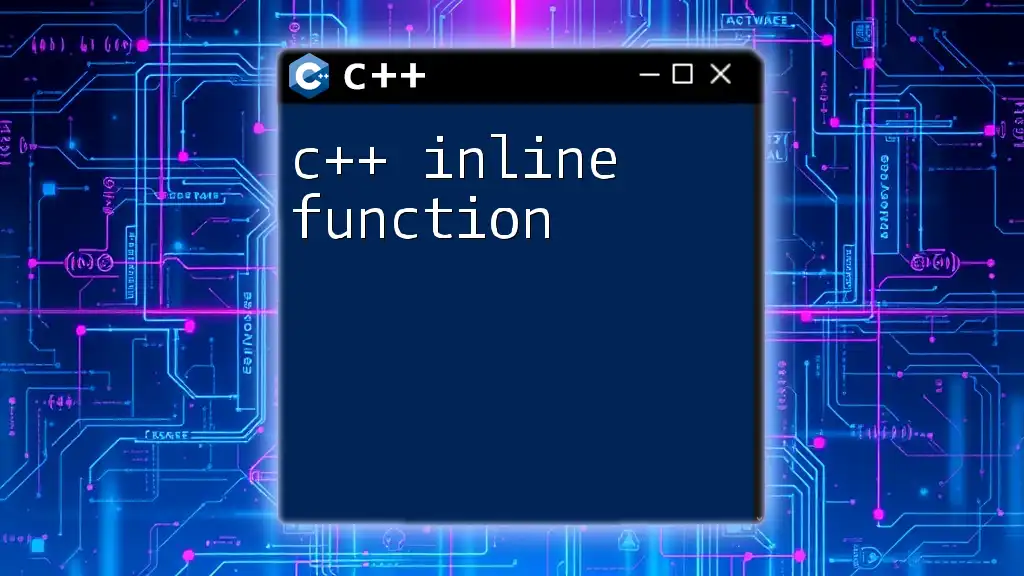
Integrating Inline Assembly with C++ Code
Accessing C++ Variables in Inline ASM
To access C++ variables within inline assembly, you must declare them accordingly. Here is an example showcasing how you can use local variables in inline ASM:
void multiply(int x, int y) {
int result;
asm ("imul %%ebx, %%eax;"
: "=a"(result)
: "a"(x), "b"(y));
// result now holds the product of x and y
}
In this code, the `imul` instruction performs multiplication on `x` and `y`, storing the product in `result`.
Returning Values from Inline ASM to C++
To return values from inline asm to C++, you should use output operands correctly. The previous examples show how to return values effectively.
int calculate_square(int number) {
int square;
asm ("imul %%eax, %%eax;"
: "=a"(square)
: "a"(number));
return square;
}
In this instance, the square of `number` is stored in `square`, which is then returned to the caller.
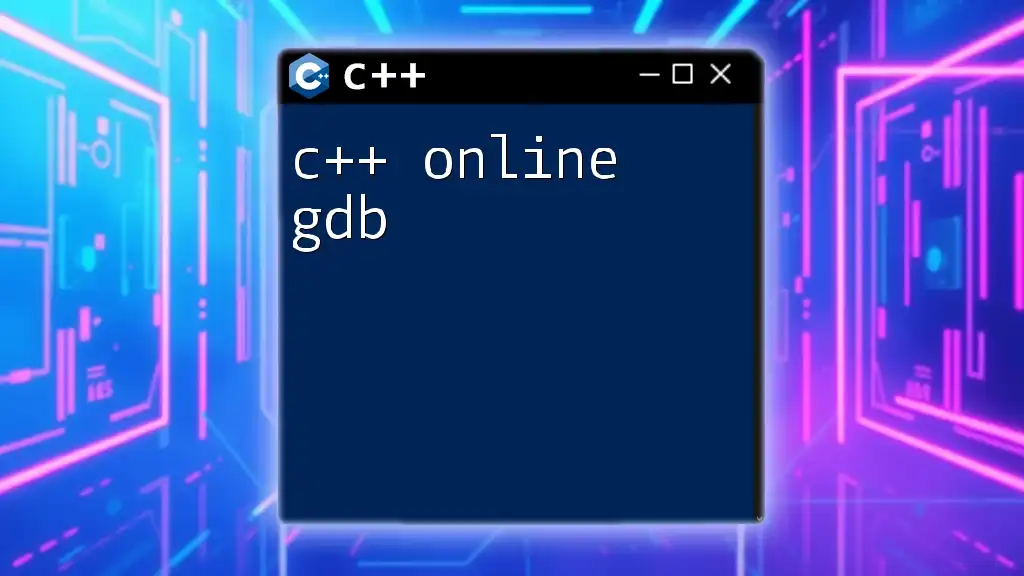
Advanced Inline Assembly Techniques
Using Constraints with Inline ASM
Constraints are fundamental in managing the interaction between C++ and inline assembly. They dictate how data is passed in and out of the assembly code. Here’s an example using different constraints:
int bitwise_and(int a, int b) {
int result;
asm ("andl %%ebx, %%eax;"
: "=a"(result)
: "a"(a), "b"(b)
: "cc"); // Clobber condition codes
return result;
}
In this example, the `andl` instruction performs a bitwise AND operation. It uses `cc` to specify that condition codes (flags) may be altered during execution, emphasizing the importance of understanding these constraints for correct program behavior.
Conditional Execution in Inline ASM
Inline ASM can include conditional execution as well. Here’s how to implement conditions:
void check_zero(int value) {
asm ("cmp $0, %%eax;"
"je zero_case;"
"jmp end_case;"
"zero_case: ... ;"
"end_case: ;"
:
: "a"(value));
}
This snippet compares `value` with zero, jumping to `zero_case` if they are equal. This level of flexibility shows how powerful C++ inline asm can be when integrated judiciously.
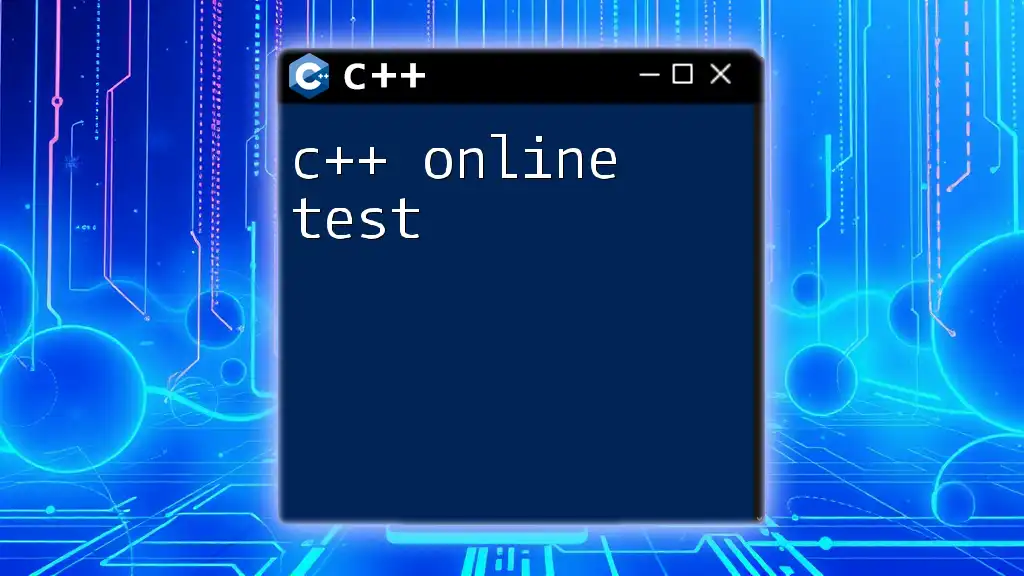
Debugging Inline Assembly Code
Common Pitfalls and Errors
Inline assembly can introduce several challenges, especially regarding data types, register usage, and constraints. Common issues include:
- Data Type Mismatches: Ensure that the types in C++ match those expected in assembly.
- Fouled Registers: Leaving registers dirty that are used later can lead to unpredictable behavior.
Tools for Debugging Inline ASM
To debug inline assembly effectively, utilize debugging tools like GDB. Compile your code with debug information, enabling inspection of registers and memory states to troubleshoot issues within the inline code.
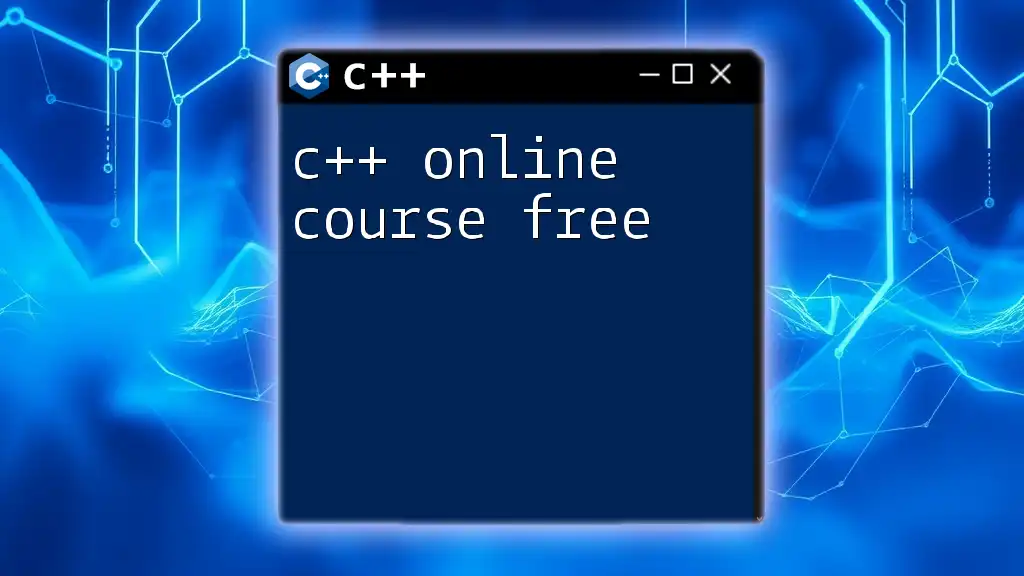
Performance Considerations
When to Use Inline ASM
Choosing to use inline asm should be a calculated decision. Use it when:
- Performance is a critical factor in specific parts of your application.
- You need to access hardware features that are not available through C++ APIs.
However, remember that excessive use can lead to decreased readability and maintainability.
Benchmarking Inline ASM vs C++
Conducting performance tests is essential to evaluate the efficiency of inline assembly versus C++. You can use tools like `gprof` to measure execution time and compare different implementations:
#include <chrono>
#include <iostream>
// Benchmark function with inline asm
void benchmark_function() {
// Inline ASM call
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
benchmark_function();
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Execution Time: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end - start).count()
<< "µs" << std::endl;
return 0;
}
This approach helps in establishing whether the performance gains from using inline assembly justify its complexity.
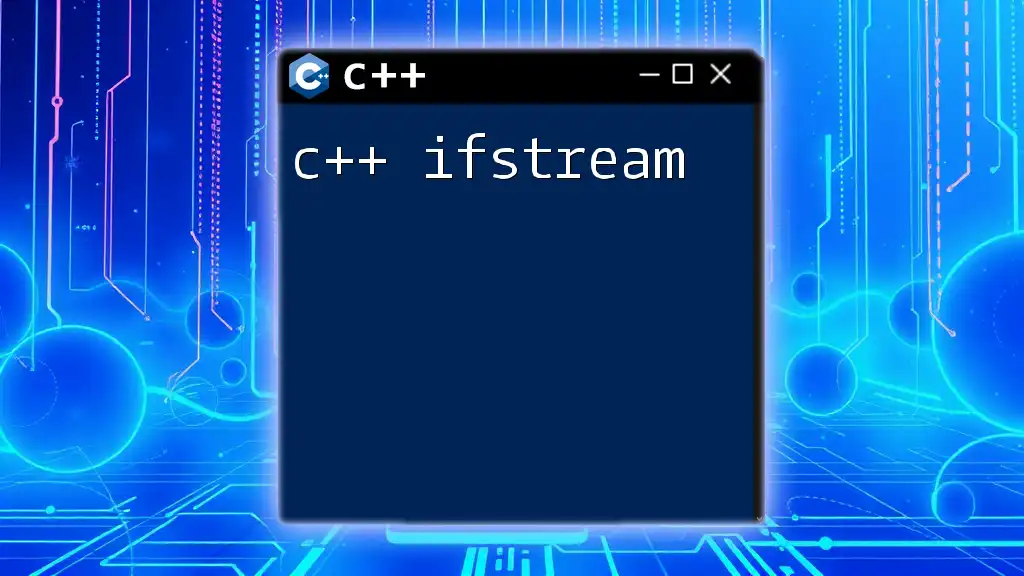
Best Practices for Using Inline Assembly
Keeping Code Readable and Maintainable
While inline ASM is powerful, it often sacrifices readability. To mitigate this, always:
- Include comments that clarify the purpose of assembly blocks.
- Isolate complex assembly code in functions to improve clarity.
Ensuring Portability
When using C++ inline asm, be aware that assembly code is often platform-specific. To maintain portability:
- Emphasize the use of abstraction layers when feasible.
- Identify the specific registers and instructions supported by your target architecture.
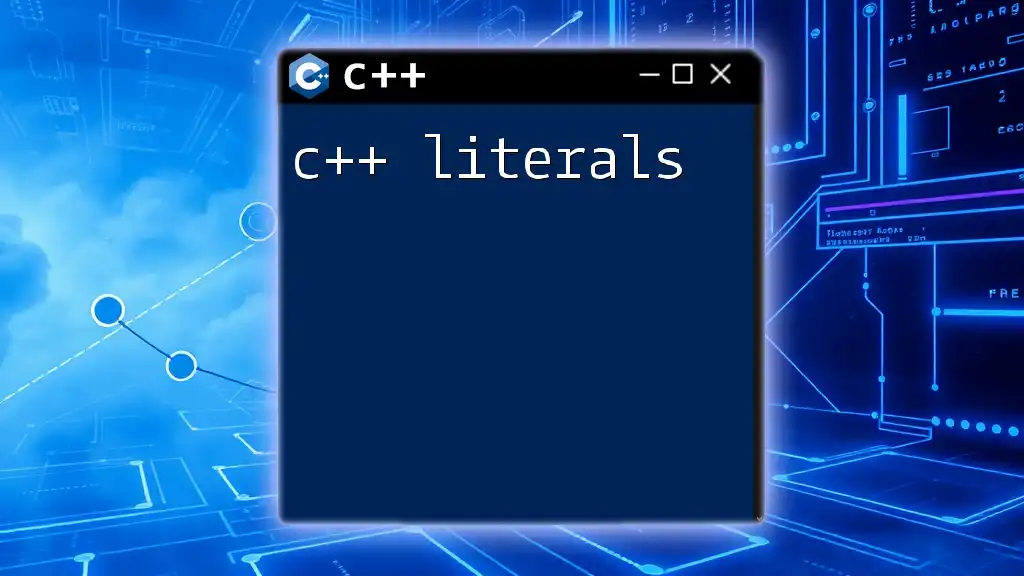
Conclusion
Utilizing C++ inline asm can unlock performance benefits and hardware control crucial for certain applications. However, it requires a good understanding of its syntax, constraints, and debugging techniques. By following best practices, you can write efficient, maintainable code that leverages the strengths of both C++ and assembly language.
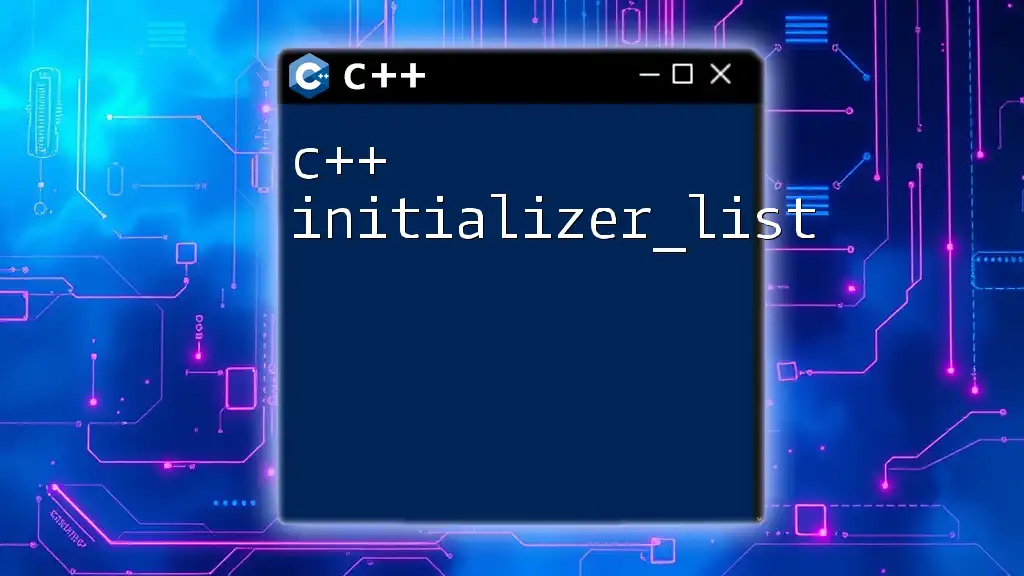
Additional Resources
For those eager to delve deeper into the world of C++ inline asm, consider exploring additional reading materials, tutorials, and community forums to engage with fellow learners and experts.