Discover our free online C++ course, designed to teach you essential C++ commands quickly and effectively, enabling you to master the language in no time.
Here's a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Applications
What is C++?
C++ is a high-level programming language developed by Bjarne Stroustrup in 1979. It is an extension of the C programming language, adding object-oriented features among other enhancements. One of the key features of C++ is its ability to combine both high-level and low-level programming, allowing developers to manage system resources efficiently while also utilizing powerful abstractions.
Why Learn C++?
Learning C++ is incredibly valuable due to its widespread application across various industries. From game development to systems programming and embedded systems, C++ serves as a cornerstone for many applications. It is in high demand, with numerous job openings requiring knowledge of C++. With C++, you gain not only a robust programming language but also an in-depth understanding of programming concepts that are applicable to other languages, giving you an edge in the tech industry.

Finding Free C++ Online Courses
Advantages of Online Learning
Opting for online courses allows you to learn about C++ in a flexible and convenient manner. The ability to access diverse materials means you can choose how you wish to learn—whether through video lectures, interactive exercises, or written tutorials. You can also learn at your own pace, making it easier to balance your studies with other commitments.
Popular Platforms Offering Free C++ Courses
Coursera offers a wide range of courses, including an excellent option called "C++ for C Programmers." This course dives deeper into advanced concepts for those with prior C knowledge.
edX hosts several C++ courses such as "Introduction to C++," which is perfect for beginners wanting to grasp the fundamentals.
Codecademy focuses on interactive learning, where students can dive into hands-on coding challenges to solidify their understanding of C++.
Udemy features many free C++ courses. A notable example is "C++ Tutorial for Complete Beginners," which is designed for newcomers and covers core concepts thoroughly.
Specializations within C++ Learning
C++ isn't just for general programming; it can be specialized for different fields. Many courses delve into game development using C++ with engines like Unreal Engine. Additionally, you can find tailored courses focusing on systems programming or using C++ for data structures and algorithms, providing you with a well-rounded skill set.
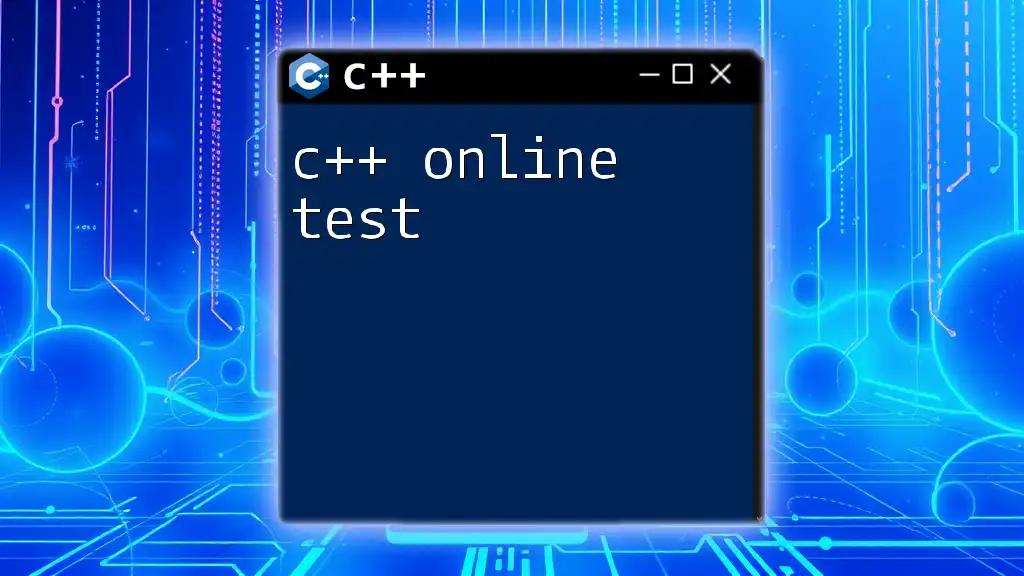
How to Choose the Right Free C++ Course
Assessing Course Quality
Choosing a free C++ course requires careful consideration of course quality. Look for courses with reputable instructors who have industry experience. Course ratings and reviews provide insight into others' opinions, which can guide your decision on the class's efficacy. Furthermore, ensuring the course covers relevant content deeply is crucial for a comprehensive learning experience.
Structure and Format of the Course
Consider the structure of each course. Does it feature video lessons, written material, or both? Identifying your preferred learning style is essential to maintaining engagement. Furthermore, emphasis on practical exercises allows you to apply what you learn, which solidifies your knowledge in a meaningful way.
Personal Learning Style
Personal preference plays a significant role. Do you prefer self-paced courses, allowing you freedom with your schedule, or structured classes with set deadlines? Consider your own routine and choose accordingly to maximize your learning experience.
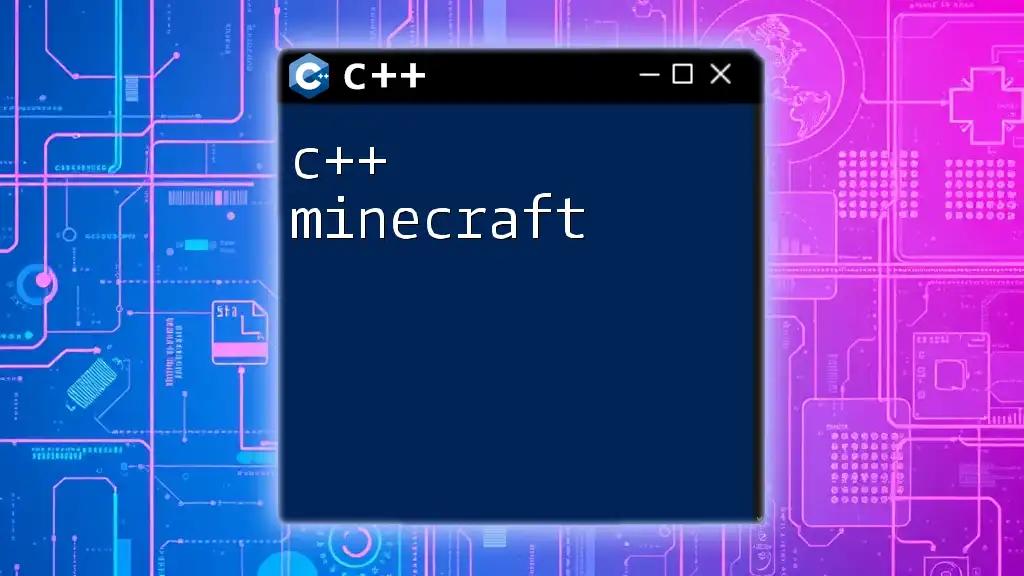
Essential Topics Covered in C++ Courses
Getting Started with C++
The first step to learning C++ is setting up your development environment. Common compilers like GCC or MinGW can be installed on various operating systems. Once installed, writing your first C++ program is both exciting and essential to grasping the language’s syntax.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program introduces you to the basic structure of C++ code, including the use of libraries and functions.
Basic C++ Syntax and Constructs
As you progress, you will explore the core constructs of C++. Understanding variables and data types is crucial, as is mastering control structures like conditionals (if statements, switch cases) and loops (for, while). Creating and using functions allows for modular code, while also highlighting the concept of scope.
Object-Oriented Programming in C++
C++ is well-known for its support of Object-Oriented Programming (OOP). Learning the four main principles of OOP—encapsulation, inheritance, polymorphism, and abstraction—will help you design cleaner and more efficient programs. Code examples, such as defining classes and creating objects, will anchor your understanding in practical applications.
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Advanced C++ Concepts
After grasping the basics, advanced topics are essential for a proficient understanding of C++. These include templates, which allow for generic programming, and exception handling, which is critical for building robust applications. Additionally, pointers and memory management are fundamental C++ features that offer extensive control over memory usage.
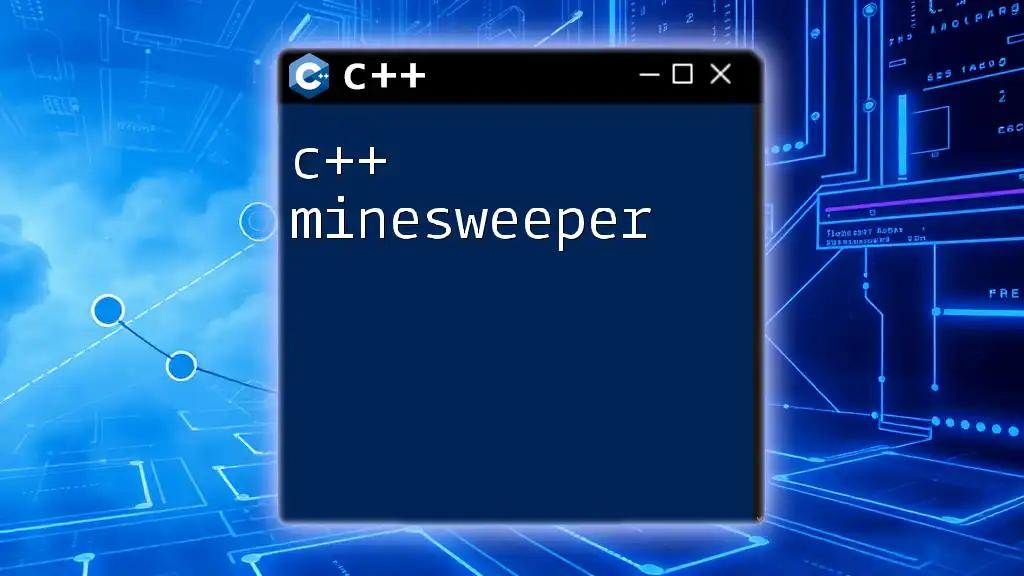
Tips for Succeeding in Your C++ Learning Journey
Set Clear Learning Goals
Establishing clear and achievable learning objectives will keep you motivated. Set both short-term and long-term goals, and create milestones to track your progress along the way.
Practice Regularly
Practical application is key to mastering C++. Engage in regular coding practice, tackle coding challenges, and participate in exercises. Websites like LeetCode and HackerRank offer numerous problems that cater to various skill levels and will help reinforce your knowledge.
Engage with the Community
Becoming active in developer communities provides additional resources and support. Joining forums, discussion groups, or online meetups can help you connect with fellow learners and experienced developers, broadening your understanding of C++ and offering insights into real-world applications.
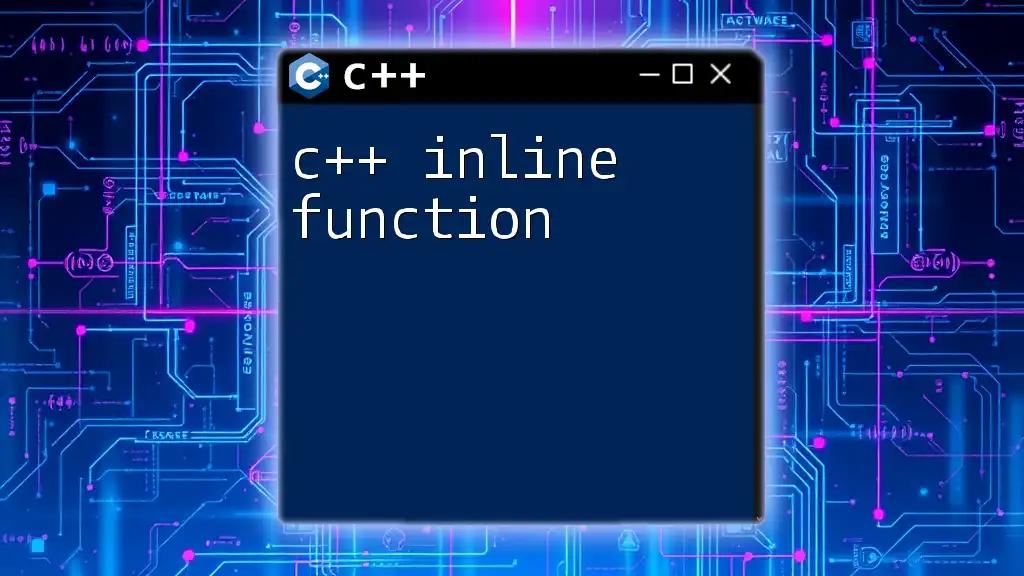
Conclusion
Investing time in learning C++ through free online courses can significantly benefit your programming career. The knowledge you gain will provide a strong foundation in software development and open doors across various tech domains. Don't hesitate to explore the plethora of resources available and start your C++ learning journey today!
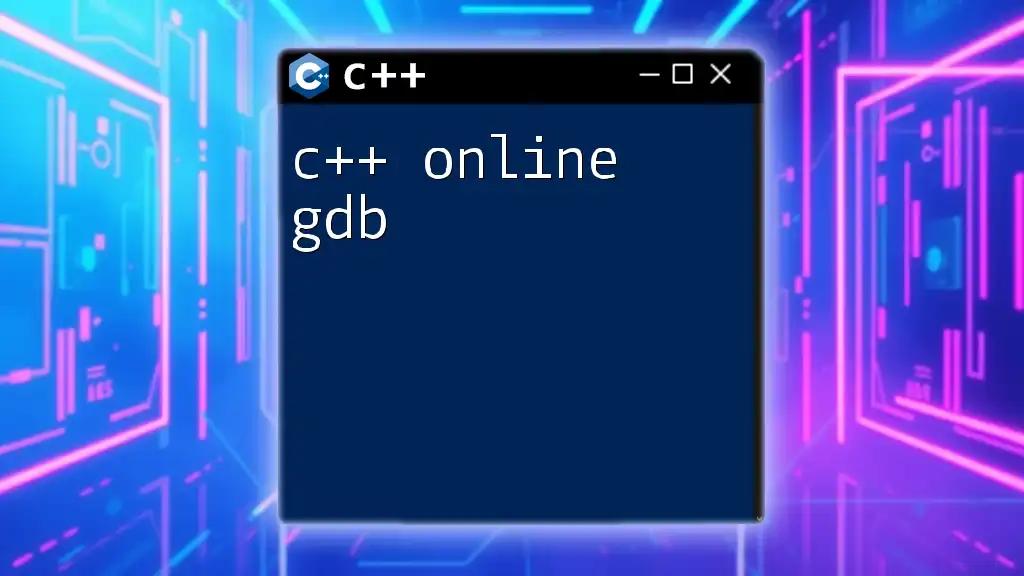
Additional Resources
For those seeking to deepen their understanding further, consider exploring recommended books and links to forums where you can ask questions, share insights, or even find mentors. While many certifications require payment, numerous free options can help validate your skills as you progress in your learning.

FAQ Section
Is it possible to learn C++ for free?
Absolutely! With the plethora of free online courses, tutorials, and resources available, you can begin your C++ journey without any financial investment.
How long does it take to learn C++ effectively?
The learning duration varies based on your background and dedication. Typically, individuals may take several weeks to months to become proficient, depending on the frequency and structure of their study.
What are the prerequisites for learning C++?
A basic understanding of programming concepts and logic can be helpful, but complete beginners are also welcome. C++ is designed to accommodate diverse levels of learner experience, making it accessible for everyone.