A C++ online test is a web-based assessment tool designed to evaluate an individual's proficiency with C++ programming commands and concepts through practical coding challenges.
Here's a simple code snippet to demonstrate a basic C++ command:
#include <iostream>
int main() {
std::cout << "Hello, C++ Online Test!" << std::endl;
return 0;
}
Understanding C++ Online Tests
What is a C++ Online Test?
A C++ online test is a digital assessment designed to evaluate an individual's proficiency in the C++ programming language. Unlike traditional paper-based exams, these tests are conducted online, allowing for greater accessibility and flexibility.
The key differences between traditional exams and online tests include instant feedback, varied question formats, and the ability to practice at one's own pace. Online tests often incorporate different modes of assessment, such as multiple-choice questions, coding challenges, and debugging exercises, all aimed at gauging comprehensive knowledge of C++.
Types of C++ Online Tests
Different levels of proficiency require different types of assessments:
Basic Level Tests focus on fundamental concepts. Topics often include:
- Syntax
- Operators
- Data Types
For example, understanding syntax is crucial as it forms the foundation of writing any C++ program. Here is a simple C++ program that displays "Hello, World!" to illustrate basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Intermediate Level Tests delve deeper into complex topics such as:
- Object-Oriented Programming (OOP)
- Pointers
- Memory Management
A good grasp of OOP is essential for writing reusable code. The following code snippet illustrates a simple class implementation demonstrating the principles of OOP:
class Animal {
public:
virtual void sound() {
std::cout << "Animal makes a sound\n";
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark\n";
}
};
void testSound(Animal &a) {
a.sound();
}
Advanced Level Tests require an in-depth understanding of topics including:
- Templates
- Standard Template Library (STL)
- Multithreading
These tests are designed for those who already have a strong foundation and are looking to specialize further in C++.
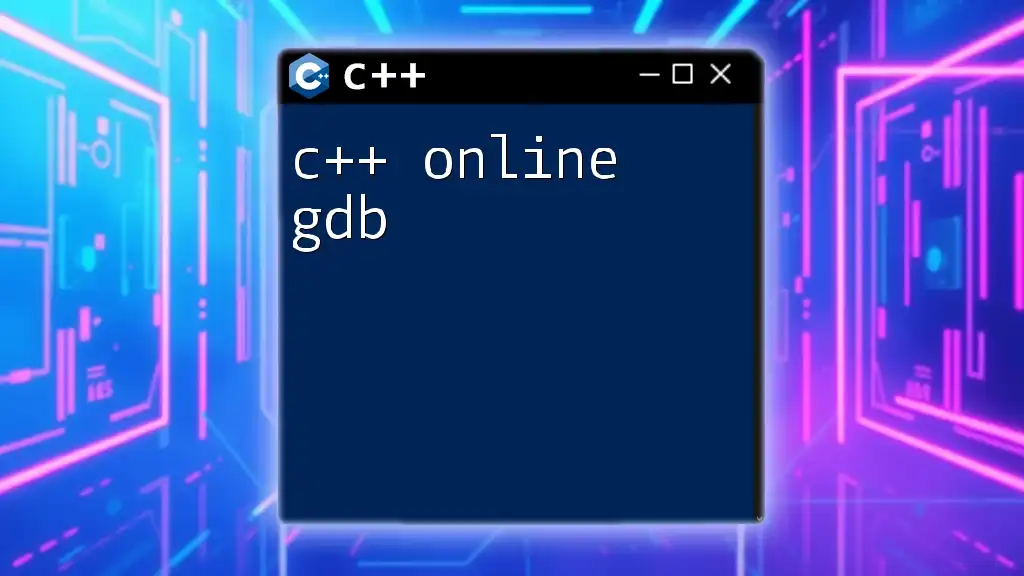
Preparing for a C++ Online Test
Essential Concepts to Review
Before taking a C++ online test, it’s vital to review critical concepts. This includes understanding the structure of the programming language, which allows you to solve problems efficiently.
Syntax and Structure: Reinforcing your knowledge of basic syntax is essential. Recognize the differences between various data types and know how to declare variables and methods.
Object-Oriented Programming: Mastering OOP principles is necessary for tackling design-oriented questions. Be prepared to recognize inheritance, polymorphism, and encapsulation in problem statements.
Recommended Study Resources
To enhance your C++ skills, consider the following resources:
-
Online Courses and Tutorials: Platforms like Coursera, Udacity, or edX offer comprehensive courses on C++ that cater to different skill levels.
-
Books and Documentation: Books such as "C++ Primer" and "Effective C++" provide in-depth insights and best practices.
-
Practice Platforms: Websites like LeetCode, HackerRank, and Codecademy offer various challenges and sample tests that can prepare you for online exams.
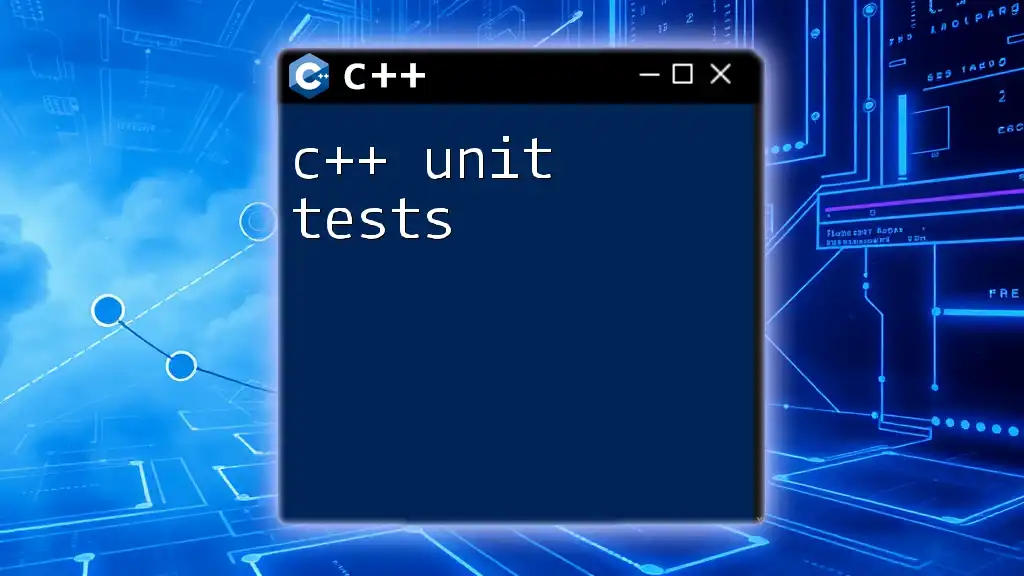
Taking a C++ Online Test
What to Expect During the Test
During a C++ online test, you'll typically encounter various question formats including multiple-choice, coding challenges, and debugging tasks. Familiarize yourself with the overall structure and approximate duration of these exams.
Tips for Success
To enhance your chances of performing well on the test, consider the following strategies:
Time Management Strategies: Allocate time wisely for each question; prioritize solving simpler questions first.
Reading Instructions Carefully: Misunderstanding a question can lead to avoidable mistakes. Always take a moment to confirm that you understand the requirements.
Debugging Practices: Develop a systematic approach to identify and fix errors in your code quickly. Familiarize yourself with common error messages within C++ as they often provide hints about the underlying issue.
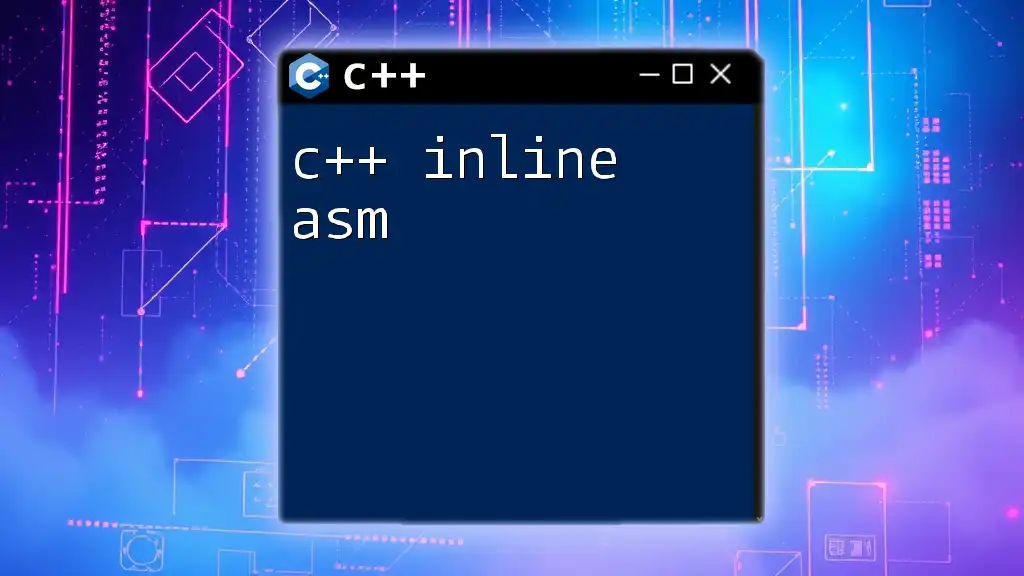
After the C++ Online Test
Analyzing Your Results
Once you receive your score, take the time to interpret it critically. Utilize feedback to identify strengths and areas that necessitate improvement. This reflection is crucial for continuous growth in C++ programming.
Continuous Learning and Improvement
Based on your test results, set definitive goals to build on areas where your performance may have flagged. Engaging with advanced resources or taking on new projects can effectively bolster your skills.
Retaking the Test
If you didn’t achieve the desired outcome, don’t be discouraged about retaking the test. Assess which areas you struggled with and focus your subsequent study efforts there. Refining your skills can often lead to significantly better results on later attempts.
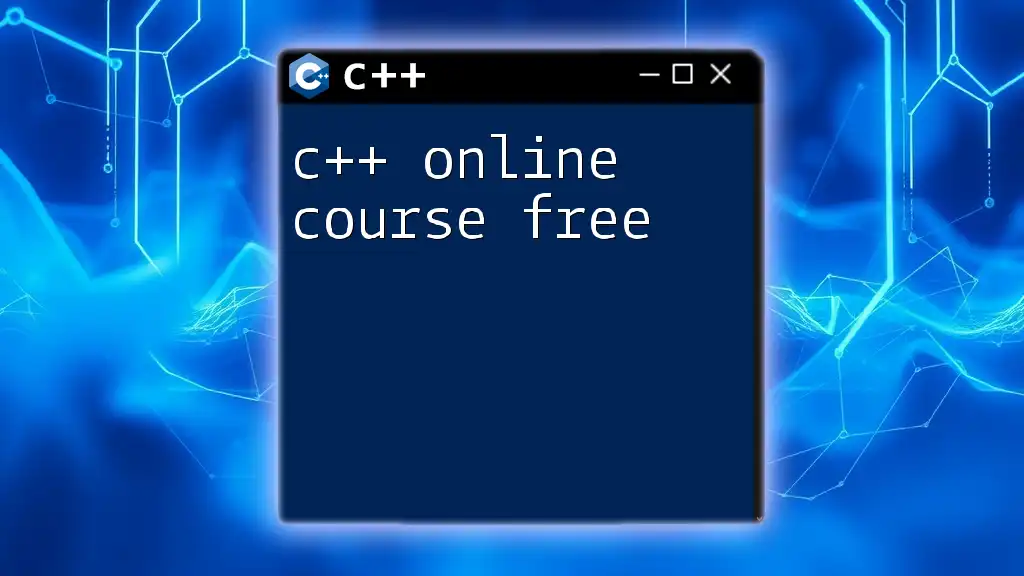
Conclusion
Participating in a C++ online test is an effective way to gauge and enhance your programming skills. The ability to learn and test your knowledge continuously will enable you to stay current in the evolving tech landscape. Embrace the journey of mastering C++, and don’t hesitate to leverage tests as a tool for improvement.
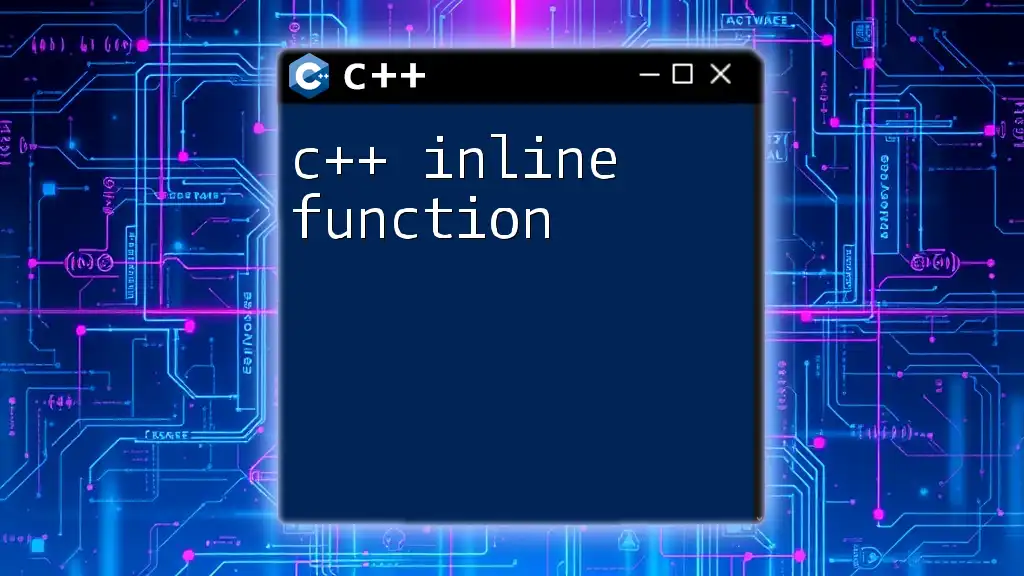
Call to Action
Are you ready to enhance your C++ skills? Explore our online test preparation courses designed to help you succeed in your C++ journey! Share your experiences or any questions regarding C++ tests below; we’d love to hear from you!