C++ Online GDB is a web-based compiler that allows users to write, compile, and run C++ code directly in their browser, making it a convenient tool for quick experimentation and learning.
Here's a simple code snippet to demonstrate its usage:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is C++ Online GDB?
C++ Online GDB is a web-based integrated development environment (IDE) that allows programmers to write, compile, and execute C++ code directly from their browsers without the need for local installations. This powerful tool empowers students, educators, and professionals to quickly prototype and test C++ code in a user-friendly environment.
Importance of Online IDEs
Online IDEs like C++ Online GDB have revolutionized the way coding is taught and learned. They make programming accessible to anyone with an internet connection. Here are some key benefits of using online IDEs:
- No Installation Required: Users can get started immediately without going through the tedious process of installing software.
- Cross-Platform Compatibility: As long as you have a web browser, you can code on any device, regardless of the operating system.
- Instant Feedback: Code can be compiled and run with a single click, providing immediate results and streamlining the learning process.
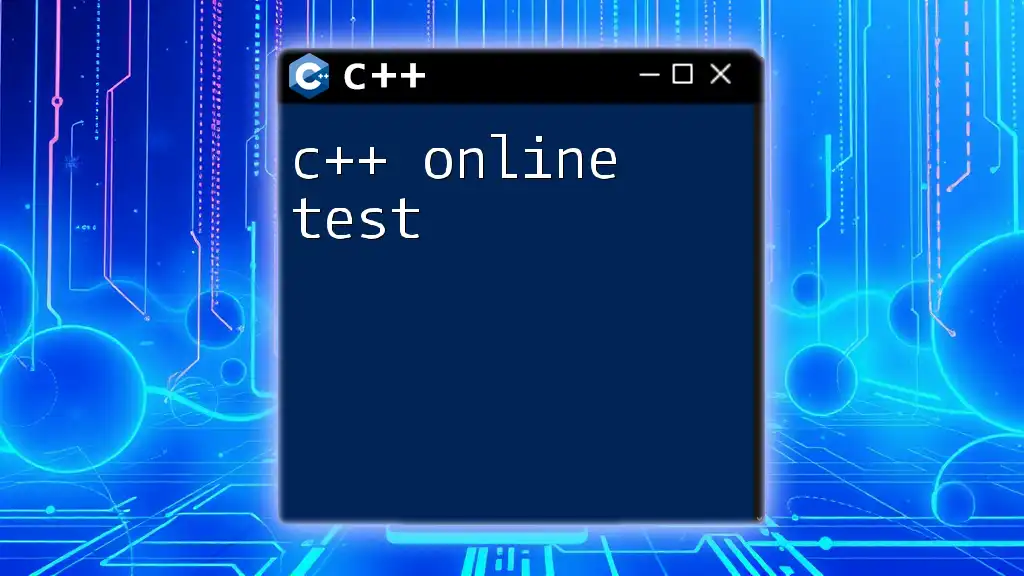
Getting Started with C++ Online GDB
How to Access Online GDB
To start using C++ Online GDB, simply navigate to the website. You will find a clean interface that is intuitive for both beginners and experienced programmers. Creating an account is optional, but it allows you to save your work and access it later.
Overview of the Interface
The layout of Online GDB is straightforward:
- Code Editor: Where you write your C++ code. It includes syntax highlighting and basic code completion features.
- Console Output Section: Displays the results of your program and any errors encountered during execution.
- Input Handling: If your program requires user input, Online GDB provides a user-friendly interface for entering data.
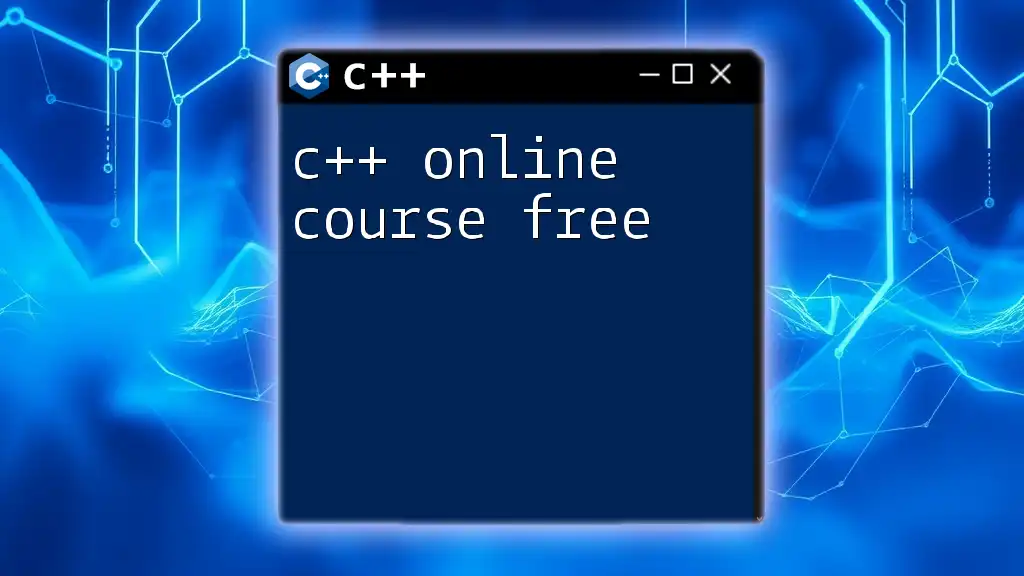
Writing Your First C++ Program
Setting Up Your First Program
To familiarize yourself with the environment, let’s write a basic "Hello, World!" program. Understanding the basic syntax of C++ is crucial. Here’s the code you’ll be using:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- Breakdown of the Code:
- `#include <iostream>`: Includes the Input/Output stream library necessary for console output.
- `using namespace std;`: Allows us to use standard names without prefixing them with `std::`.
- `int main()`: The main function where execution starts.
- `cout << "Hello, World!" << endl;`: Outputs "Hello, World!" to the console.
- `return 0;`: Indicates successful completion of the program.
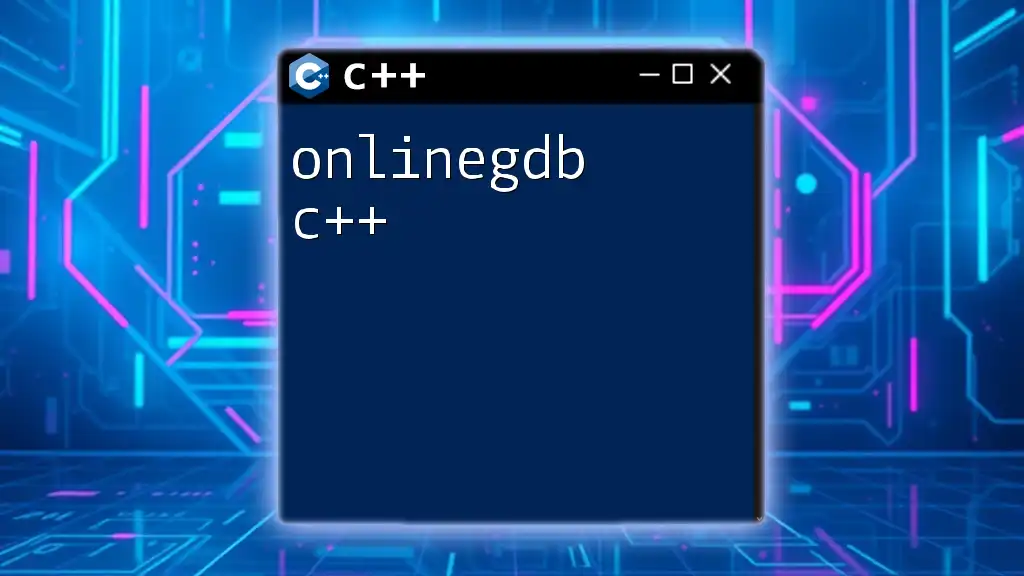
Compiling and Running Code
How to Compile Your Code in Online GDB
Once you write your C++ code, the next step is to compile it. Compiling converts your C++ source code into machine code that the computer can execute. If there are errors, Online GDB will highlight them for you, allowing you to debug easily.
Running Your Program
After compilation, you can run your program with a single click. The Console Output Section will display the results of your program. For example, let’s create a simple calculation program:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
cout << "Sum: " << a + b << endl;
return 0;
}
- Explanation of Input and Output:
- The program prompts the user to enter two integers.
- It captures the input using `cin` and computes the sum, then prints it to the console.
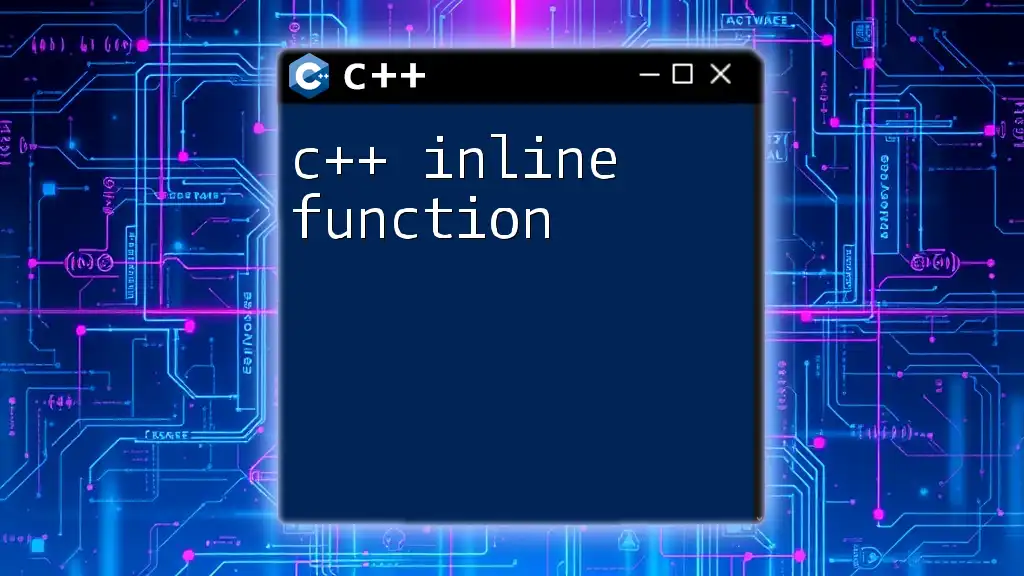
Debugging Your Code
Common Errors in C++
Debugging is a vital skill every programmer should master. Here are some common types of errors you may encounter:
- Syntax Errors: Mistakes in the code structure that prevent compilation.
- Runtime Errors: Occur when code is syntactically correct but causes problems during execution (e.g., division by zero).
Using Online GDB's Debugger Tools
Online GDB offers built-in debugging tools. You can step through your code line by line to track variable values and identify where things are going wrong.
Example: Debugging a Factorial Function
Here’s a recursive function to calculate the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
if (n < 0) return -1; // Handle negative input error
return n == 0 ? 1 : n * factorial(n - 1);
}
int main() {
int num = 5;
cout << "Factorial of " << num << " is " << factorial(num) << endl;
return 0;
}
- This program computes the factorial of 5, showcasing recursion. Understanding how the function calls itself helps solidify your grasp of both recursion and debugging techniques.
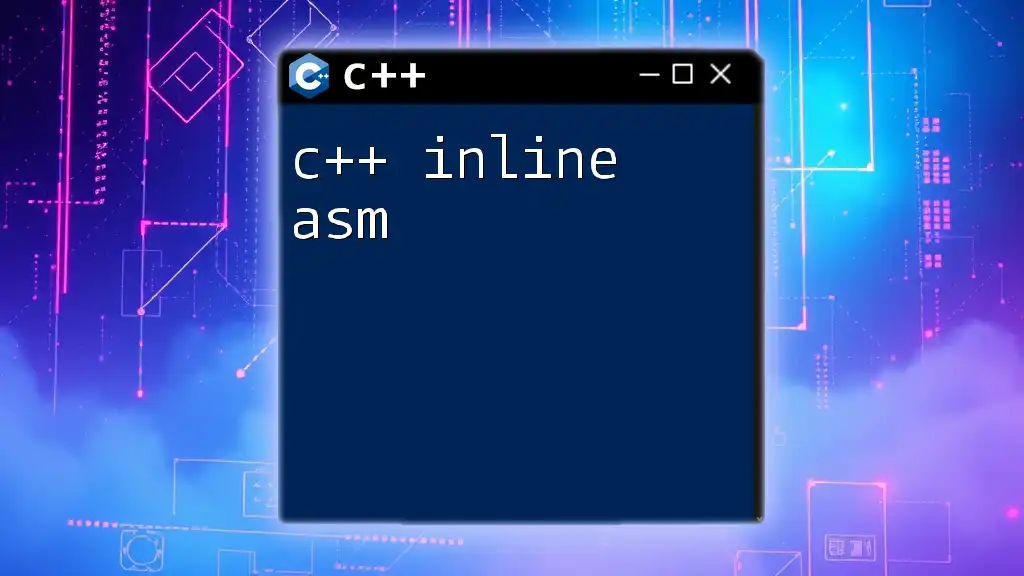
Advanced Features of Online GDB
Using Multiple Files
For larger projects, you can manage multiple source files. This functionality is crucial when working on extensive applications. You can create a project structure that separates logic into different files, making maintenance easier.
Collaborative Coding
Another exciting feature of Online GDB is its ability to facilitate collaborative coding. You can share your projects with peers and even work in real-time on the same codebase. This feature is invaluable for teamwork and peer reviews.
Utilizing Libraries and External Code
You can enhance your C++ programs by including external libraries, such as the Standard Template Library (STL). Here’s a sample code snippet that demonstrates the use of STL:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
cout << number << " ";
}
return 0;
}
- This example demonstrates how to use vectors, a powerful tool in C++ that simplifies handling dynamic arrays.
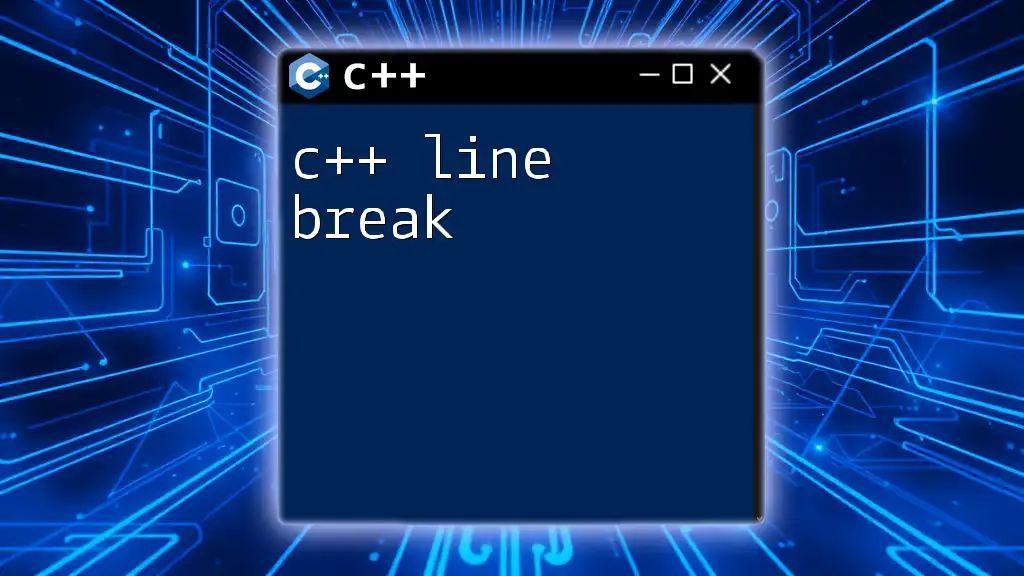
Best Practices for Using C++ Online GDB
Efficient Coding Practices
To make the most out of Online GDB, follow these best practices:
- Write Modular and Organized Code: This makes your project manageable and easier to debug.
- Keep Codes Clean: Clear code improves readability, making it easier to spot errors during debugging.
Learning Resources and Documentation
To enhance your C++ skills, consider exploring recommended books, online tutorials, and active coding communities. Engaging with communities can provide support and facilitate learning.
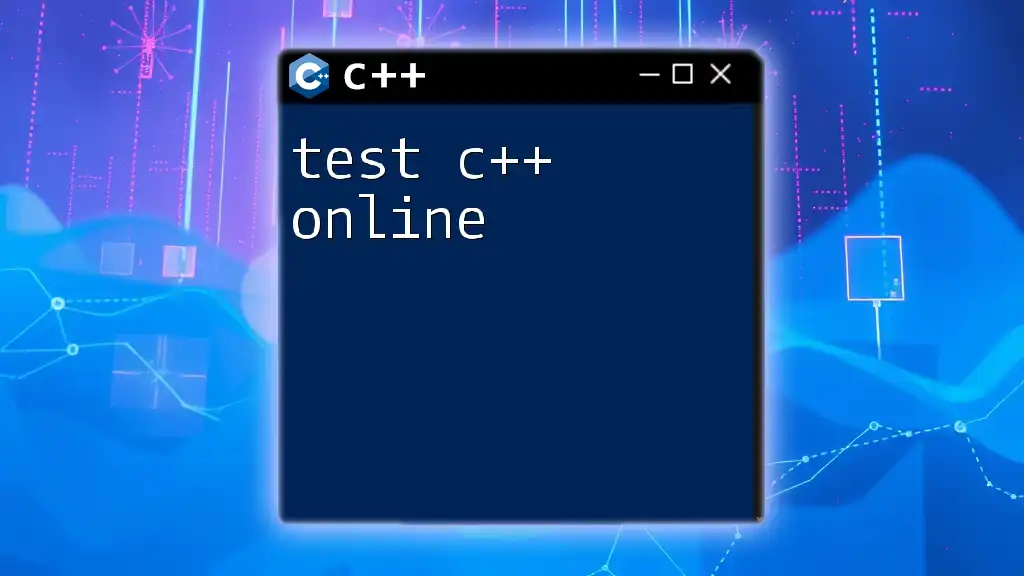
Conclusion
C++ Online GDB is an essential tool for anyone interested in learning C++ programming. By offering a user-friendly environment for writing, compiling, and debugging code, it empowers individuals to practice and improve their coding skills anywhere, anytime. Now, it's time to dive in and start writing your own C++ programs!
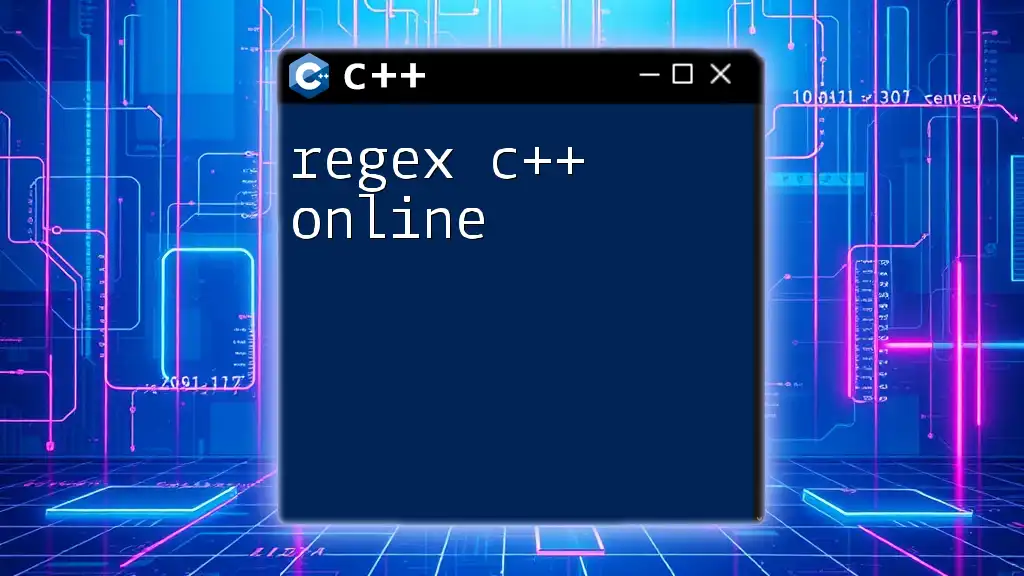
FAQs about C++ Online GDB
-
What are the system requirements for using Online GDB?
- You'll need a modern web browser and an internet connection to access Online GDB.
-
Can Online GDB be used for professional development?
- Yes, it’s suited for both educational purposes and professional development.
-
How safe is it to use Online GDB for coding practice?
- Online GDB takes measures to protect your data, but it's always wise to avoid sharing sensitive information.