GDB (GNU Debugger) is a powerful debugging tool for C++ that allows developers to inspect the execution of their programs, identify issues, and manipulate runtime conditions.
Here’s a basic example of how to use GDB to run a C++ program:
g++ -g myprogram.cpp -o myprogram // Compile the program with debugging information
gdb ./myprogram // Start GDB with the compiled program
In GDB, you can then use commands like `break main` to set a breakpoint at the `main()` function and `run` to start the program execution.
What is GDB?
Introduction to GDB
GDB, or GNU Debugger, is a powerful tool integral to the C++ development process. Debugging is an essential part of software development, enabling programmers to identify and fix errors in their code effectively. GDB allows developers to monitor program execution, inspect variables, manipulate program flow, and analyze crashes, making it a vital asset for understanding code behavior.
Key Features of GDB
The versatility of GDB comes from its robust features:
- Command-line interface: GDB operates primarily through a command-line interface, empowering developers to manage their debugging tasks efficiently without the overhead of a graphical user interface.
- Support for multiple programming languages: While it primarily focuses on C and C++, GDB also accommodates several other programming languages, broadening its applicability across various programming projects.
- Breakpoints and watchpoints: Breakpoints enable developers to pause program execution at designated lines, allowing for deeper inspection of the code at critical moments. Watchpoints provide a mechanism to stop execution whenever a specified variable changes, aiding in tracking down errant behavior.
- Backtrace functionality: With backtrace, users can view the call stack at any point during execution. This feature helps identify the sequence of function calls that led to a specific point, especially useful during error diagnosis.

Setting Up GDB for C++
Installation Steps
Setting up GDB is straightforward, and it can be done on various platforms:
-
Linux: Use your package manager to install GDB. For example, on Debian-based systems, run:
sudo apt-get install gdb
-
Windows: Utilize MinGW or Cygwin to install GDB. This setup allows running GDB within a UNIX-like environment.
-
macOS: Install GDB using Homebrew by executing:
brew install gdb
Compiling Your C++ Program for GDB
For effective debugging, you need to compile your C++ program with debugging symbols. This allows GDB to provide more meaningful information. To do this, use the following command:
g++ -g your_program.cpp -o your_program
The `-g` flag is critical, as it tells the compiler to include the necessary symbols for debugging, ensuring that you can trace through your code effectively.
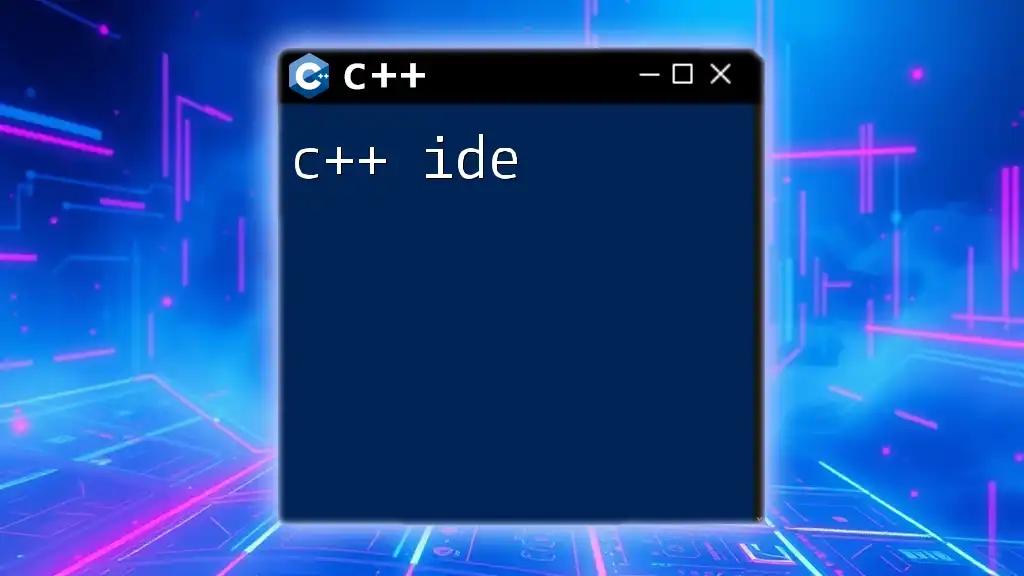
Basic GDB Commands
Starting GDB
To start working with GDB, you must launch it alongside your compiled program. The following command demonstrates how to initiate GDB:
gdb ./your_program
This command opens GDB with your specified program, ready for debugging.
Navigating Within GDB
Once in GDB, you can execute several essential commands.
-
Running the Program: Use the `run` command to start executing your program within the debugger.
-
Setting Breakpoints: Breakpoints are set to halt program execution at specific points. To set a breakpoint at line 10, enter:
break 10
-
Listing Source Code: To view the current line of code alongside surrounding lines, use the `list` command.
Controlling Program Execution
GDB offers several commands to manage program execution:
-
Step Through Code: The `step` command executes the next line of code, entering functions if necessary, while `next` executes the next line but skips over function calls, making it suitable for stepping through loops or large functions without delving into each one.
-
Continue Execution: If your program hits a breakpoint, you can resume execution by using the `continue` command. This allows for seamless progress through your code after pausing.
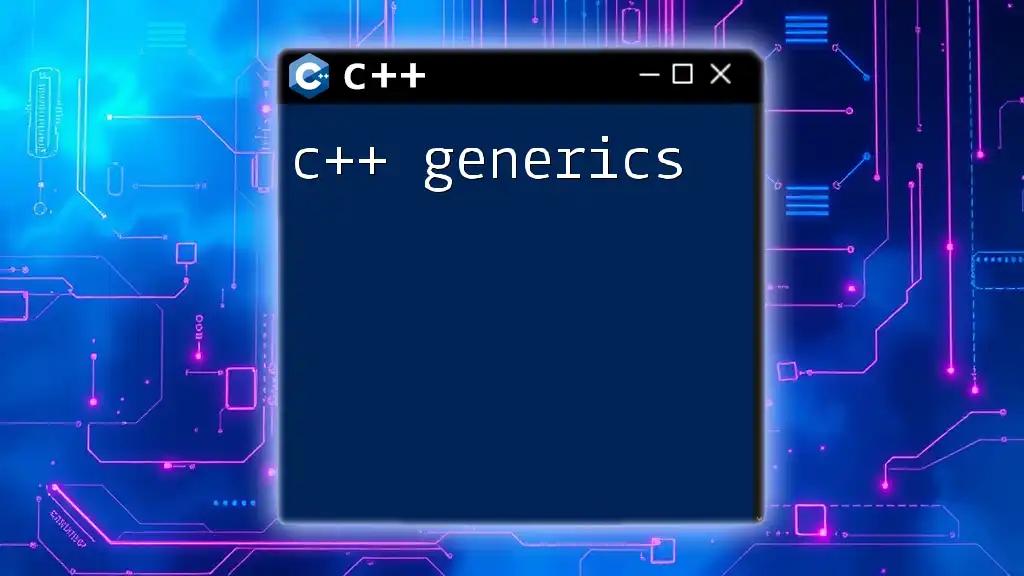
Advanced GDB Features
Inspecting Variables
One of the most powerful aspects of GDB is its ability to inspect program variables:
-
Printing Variable Values: To check the value of a variable, employ the `print` command. For instance, to view the value of a variable named `myVariable`, use:
print myVariable
-
Watching Variables for Changes: You can track variable changes during execution by using the `watch` command:
watch myVariable
This command pauses program execution whenever `myVariable` is modified, alerting you to changes you might need to investigate.
Navigating the Call Stack
Understanding the call stack is crucial for tracing function calls, particularly during a crash. GDB provides a simple way to navigate this:
-
Backtrace: By entering the `backtrace` command, you can view the call stack, which lists all active function calls at the current moment. This can help pinpoint the origin of an error.
-
Frame Navigation: Use the `frame` command to switch to a different frame in the stack, allowing for inspection of variables and execution context specific to that function call.
Debugging Multi-threaded Programs
Debugging multi-threaded applications can be challenging, but GDB simplifies the process:
-
Understanding Threads in GDB: GDB handles multi-threaded programs by allowing you to inspect and control individual threads, essential for diagnosing threading issues.
-
Commands for Managing Threads: Use `info threads` to list all threads. To switch to a specific thread, apply the `thread <thread-number>` command to focus your debugging efforts on the appropriate context.
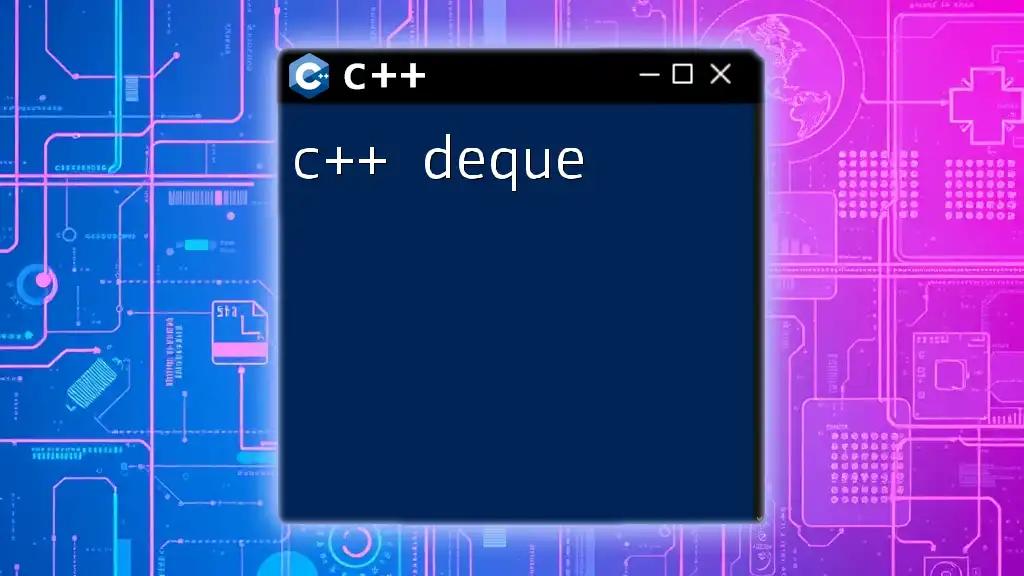
Tips and Best Practices for Using GDB
Efficient Debugging Techniques
To enhance your GDB experience, consider the following techniques:
-
Use Prefixes for Commands: Many GDB commands can be abbreviated, enabling rapid input and minimizing typing. For instance, `b` instead of `break`.
-
Scripting in GDB: GDB allows you to automate debugging tasks using scripts. This can significantly expedite repetitive debugging processes.
Common Pitfalls and Mistakes
As with any tool, there are common pitfalls to avoid:
-
Failing to Compile with Debugging Symbols: Always ensure your program is compiled with the `-g` flag, or GDB will be less effective.
-
Overusing Breakpoints: While breakpoints are useful, setting too many can complicate debugging. Use them judiciously to maximize their effectiveness.
Additional Resources
For those looking to deepen their understanding of GDB, consider consulting the official [GDB documentation](https://www.gnu.org/software/gdb/documentation/) as well as various online tutorials and books that provide further insights into debugging practices.
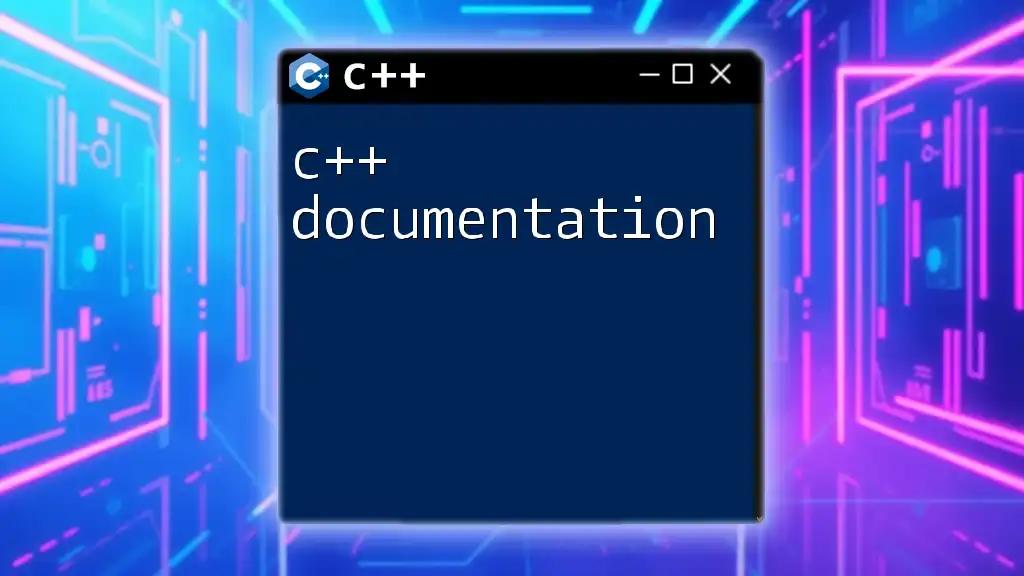
Conclusion
Mastering GDB is a profound step toward becoming an adept C++ programmer. The ability to debug efficiently can significantly enhance your coding skills and help produce robust software. By understanding GDB's features and commands, you position yourself to tackle even the most complex programming challenges with confidence.
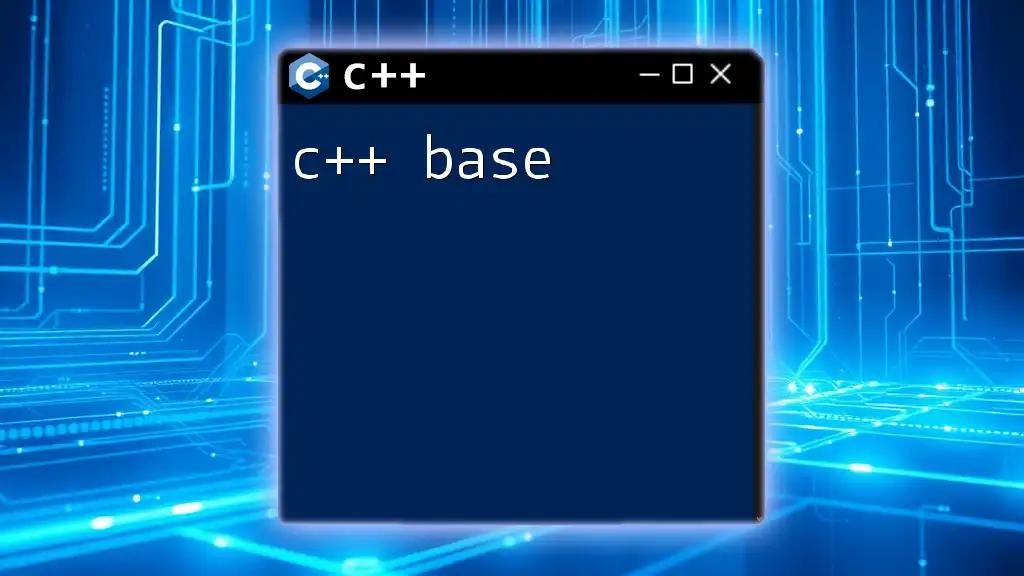
Call to Action
If you found this guide helpful, sign up for more insights into C++ and GDB usage. Take a deeper dive into debugging techniques and gain access to additional resources designed to elevate your programming skills.