The `rdbuf` function in C++ is used to access the underlying stream buffer of a stream object, allowing direct manipulation of the stream's input or output buffer.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <sstream>
int main() {
std::stringstream ss;
ss << "Hello, World!";
std::cout.rdbuf(ss.rdbuf()); // Redirects cout to the stringstream's buffer
std::cout << "This will be stored in the stringstream." << std::endl;
return 0;
}
Understanding Stream Buffers
What is a Stream Buffer?
Stream buffers in C++ are an integral part of the I/O library, acting as intermediaries that temporarily hold data during input and output operations. They enhance performance by reducing the number of I/O calls made directly to the operating system, allowing multiple bytes of data to be processed in bulk instead. This system helps streamline the flow of data, ensuring efficient communication between your program and external data sources.
Types of Stream Buffers
C++ provides various types of stream buffers, each tailored for different needs. The most fundamental among them is `std::streambuf`, which serves as the base class for implementing custom input and output stream buffers. It offers functionalities for handling character-based I/O and managing data efficiently between the program and the data sources.
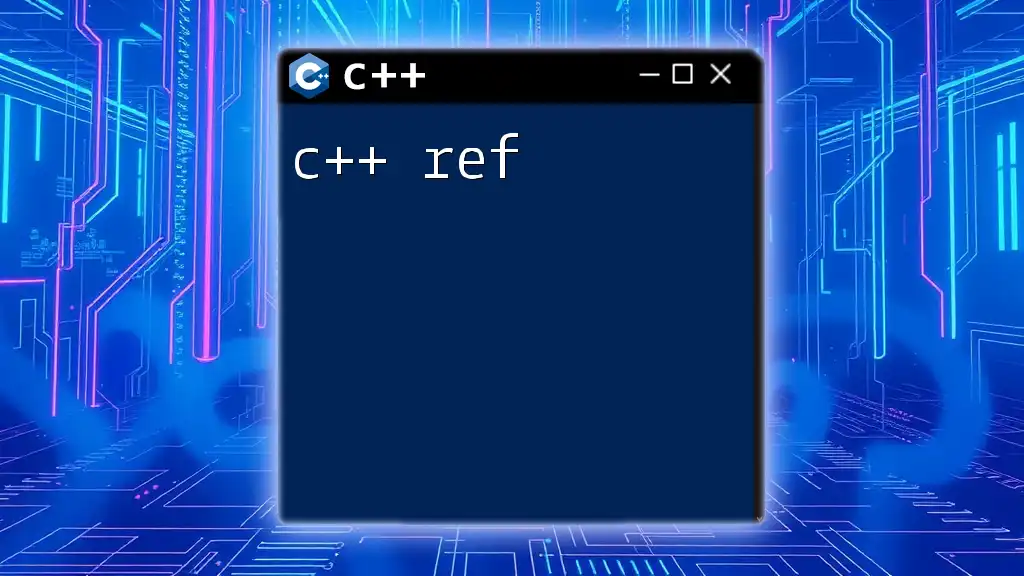
The rdbuf Member Function
Definition and Purpose
The `rdbuf` member function is a critical component of stream classes in C++. Its primary purpose is to provide access to the underlying buffer of a stream. Essentially, it allows you to retrieve or set the stream buffer associated with an input or output stream. This enables developers to perform advanced operations like redirecting I/O or swapping buffers while maintaining usability and abstraction.
Syntax of rdbuf
Using `rdbuf` is straightforward. The general syntax for using the `rdbuf` function is as follows:
streambuf* rdbuf() const;
This syntax indicates that calling `rdbuf()` will return a pointer to the stream buffer currently associated with the stream.
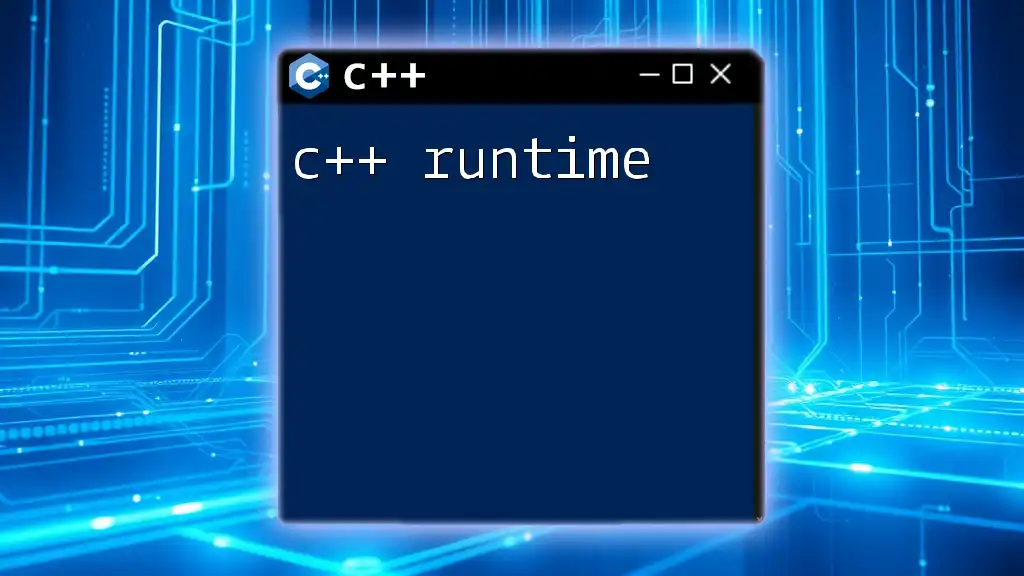
Common Use Cases for rdbuf
Redirecting Input and Output
One of the most common uses of `rdbuf` is to redirect output from the console (i.e., `std::cout`) to a file. This allows developers to log program output or save results without cluttering the console.
Example: Redirecting std::cout to a File
Here’s a brief example demonstrating how to accomplish this:
#include <iostream>
#include <fstream>
int main() {
std::ofstream file("output.txt");
std::streambuf* oldCoutBuf = std::cout.rdbuf(file.rdbuf());
std::cout << "This will be written to the file!" << std::endl;
// Restore original buffer
std::cout.rdbuf(oldCoutBuf);
return 0;
}
In this code:
- We create an `ofstream` object named `file`, which opens "output.txt".
- The original buffer of `std::cout` is saved in `oldCoutBuf`.
- We use `rdbuf` to redirect the output stream to the file's buffer.
- Key Point: After outputting to the file, it’s crucial to restore the original `std::cout` buffer. This ensures that subsequent outputs go back to the console.
Using rdbuf for Custom Stream Buffers
Developers can also implement their own custom stream buffers by subclassing `std::streambuf`. The `rdbuf` function is instrumental when working with these custom streams.
Example: Creating a Custom Stream Buffer
The following code snippet illustrates a simple custom stream buffer implementation:
#include <iostream>
#include <streambuf>
class MyBuffer : public std::streambuf {
protected:
int overflow(int c) override {
std::cout << static_cast<char>(c); // Output character to console
return c;
}
};
int main() {
MyBuffer myBuffer;
std::ostream myStream(&myBuffer);
myStream << "This goes through my custom buffer!" << std::endl;
return 0;
}
In this example:
- We derived a class `MyBuffer` from `std::streambuf`.
- The `overflow` function is overridden to handle character overflow by printing characters directly to the console.
- This custom buffer can be used in any output context, showcasing the flexibility of `rdbuf`.
Synchronizing Multiple Streams
Sometimes, it's essential to synchronize multiple output streams, especially in complex applications where output needs to be consistent across different destinations.
Example: Synchronizing with rdbuf
The following example demonstrates how to synchronize output between `std::cout` and a file stream:
#include <iostream>
#include <fstream>
int main() {
std::ofstream file("sync_output.txt");
std::ostream myStream(&file);
// Syncing buffers
myStream.rdbuf(std::cout.rdbuf());
myStream << "This will also appear in sync_output.txt" << std::endl;
return 0;
}
Here, the example shows:
- The desired output stream (`myStream`) is set to use the same buffer as `std::cout`.
- Any output written through `myStream` is also reflected in "sync_output.txt", demonstrating effective synchronization.
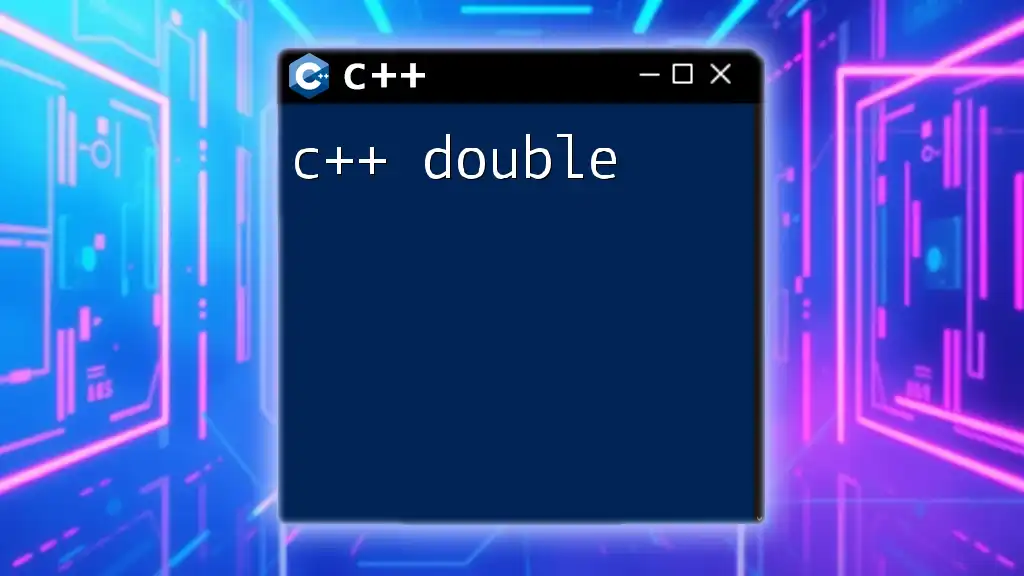
Practical Considerations
Performance Considerations
While using `rdbuf`, it's important to consider performance implications. Directly manipulating buffers can be more efficient than repeated calls to I/O functions. However, users should be cautious and perform appropriate testing, as improper handling may lead to exceptions or undefined behavior.
Best Practices
To maximize the benefits when working with `rdbuf`, follow these best practices:
- Always restore original buffers after redirecting.
- When creating custom buffers, ensure threadsafety if used in concurrent contexts.
- Regularly check for errors with I/O operations to maintain data integrity.
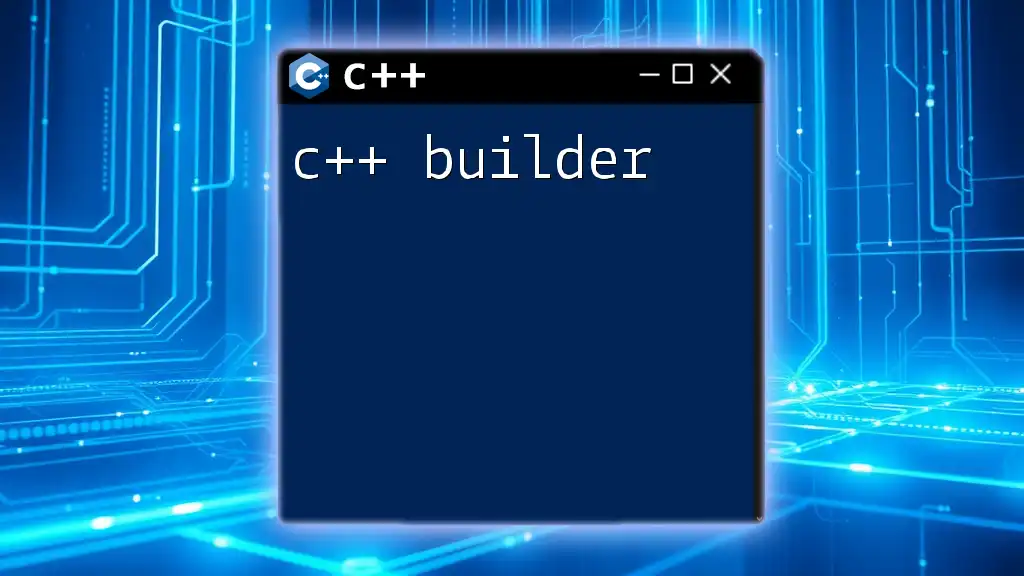
Conclusion
The C++ rdbuf function is a powerful tool in the realm of C++ I/O operations. It enables developers to redirect outputs, create custom stream buffers, and synchronize multiple streams seamlessly. Understanding and mastering `rdbuf` can enhance the efficiency and flexibility of your C++ applications.
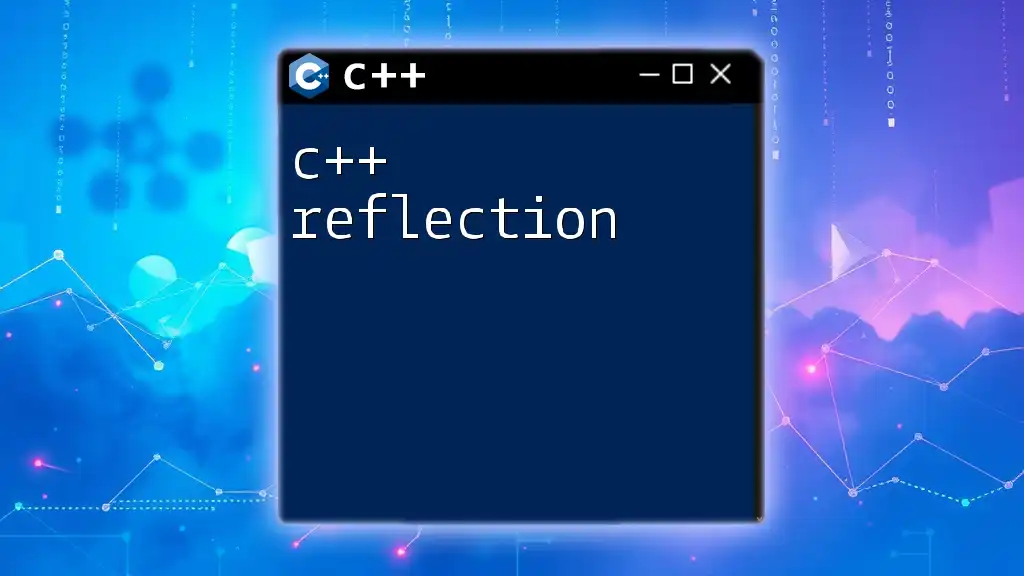
Further Reading
For additional resources on C++ streams and intensive studies on `rdbuf`, consider referring to official C++ documentation, books on C++ programming, and articles focused on advanced stream operations.
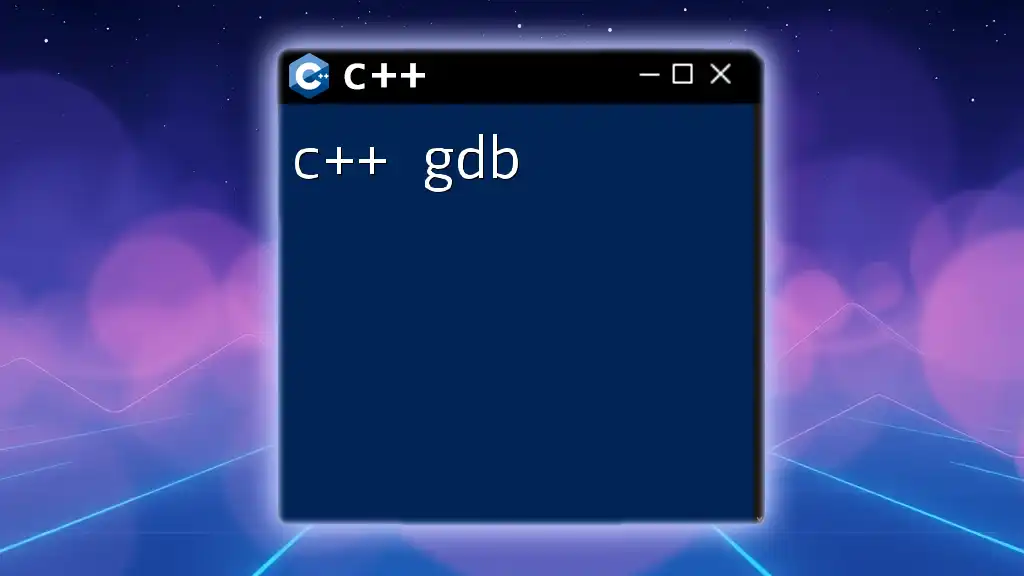
Call to Action
We encourage you to experiment with these examples and discover the full potential of C++ streams and `rdbuf`. Join us for more informative articles packed with tips and tricks designed for C++ enthusiasts!