The `rfind` function in C++ searches for the last occurrence of a specified substring within a string and returns its position or `string::npos` if not found.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world! Welcome to the world of C++.";
std::string word = "world";
size_t position = str.rfind(word);
if (position != std::string::npos) {
std::cout << "Last occurrence of '" << word << "' found at: " << position << std::endl;
} else {
std::cout << "'" << word << "' not found." << std::endl;
}
return 0;
}
What is rfind?
The `rfind` function is a powerful string method in C++ that allows developers to search for the last occurrence of a specified substring within a string. This functionality is particularly useful when you need to locate the most recent instance of a substring or character in a larger string.
When using `rfind`, you can simply retrieve the position of the substring from the end of the main string, making it different from `find`, which starts searching from the beginning.
Syntax of rfind function
The `rfind` function can be called in two ways. Here’s the syntax:
size_t rfind(const string& str, size_t pos = npos) const noexcept;
size_t rfind(char c, size_t pos = npos) const noexcept;
This syntax indicates that `rfind` can either search for a character or a substring (string). The `pos` parameter allows you to start the search at a specific index, or by default, it searches the entire string if you don't specify a position.
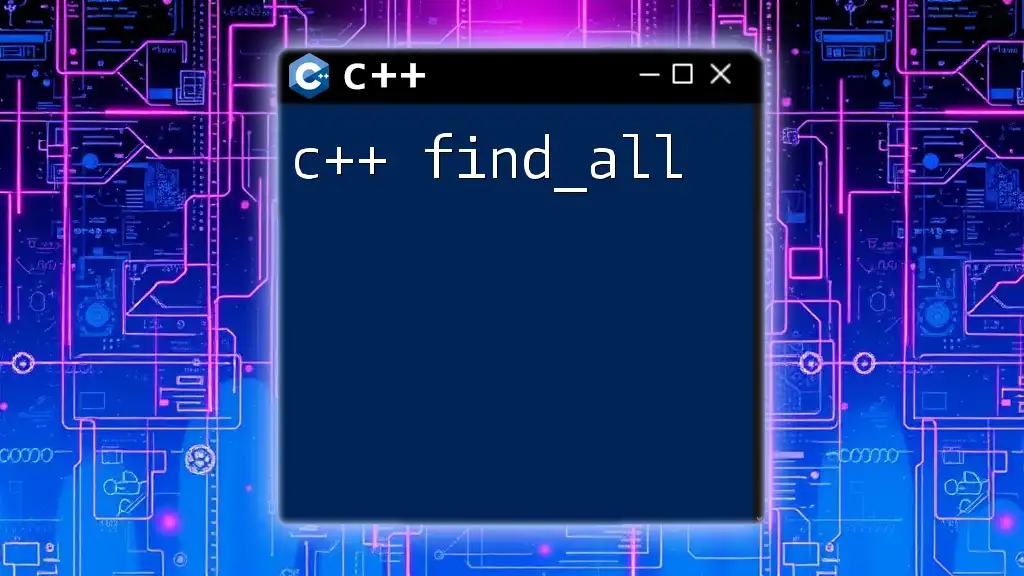
Understanding Parameters of rfind
Search String Parameter
The first parameter, `const string& str`, is the substring you want to search for within the main string. You can pass any sequence of characters, including empty strings. The function will return the position of the last occurrence of this substring.
Position Parameter
The second parameter, `size_t pos`, determines the ending index for your search. If you don’t provide this parameter, the search will include the entire string. On the other hand, if you want to restrict your search to a specific portion, passing an index value that limits the search range can be beneficial.
Return Value
The `rfind` function returns the position of the found substring as a `size_t` value. If the substring is not found, it returns `std::string::npos`, a constant that signifies "not found". This is important for error handling, allowing you to control the flow of your program effectively.
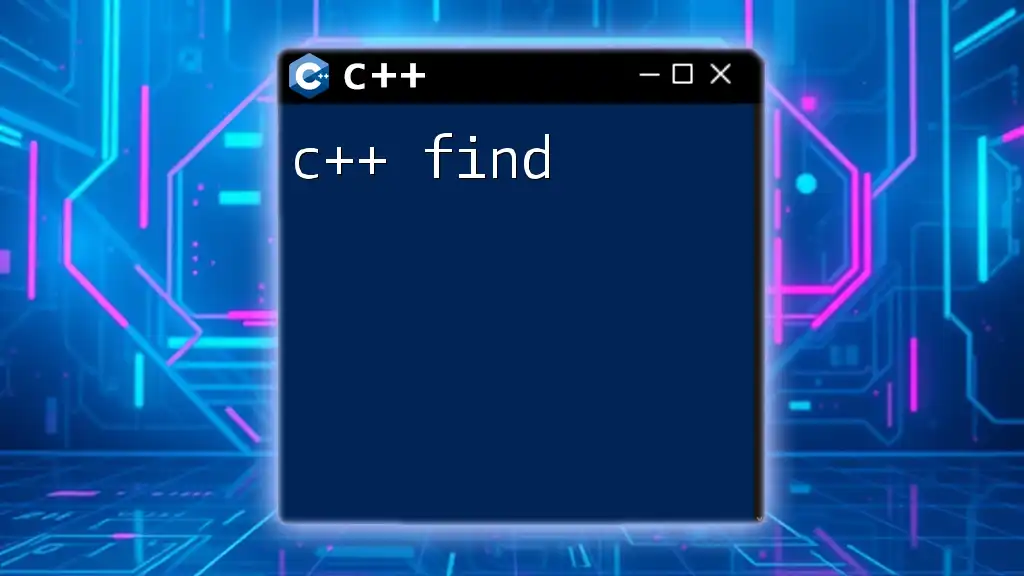
How to Use rfind in Your Code
Basic Example of rfind
One of the simplest ways to use `rfind` is by searching for a substring in a given string. For example:
std::string sentence = "Learning C++ is enjoyable. C++ is powerful.";
size_t pos = sentence.rfind("C++");
if (pos != std::string::npos) {
std::cout << "'C++' found at position: " << pos << std::endl;
} else {
std::cout << "'C++' not found." << std::endl;
}
In this example, the program searches for the last occurrence of "C++" in the `sentence`. If found, it outputs the position; otherwise, it indicates that the substring was not found.
Using rfind with Character Parameter
You can also use `rfind` to locate the last occurrence of a character. Here is an example illustrating this concept:
std::string text = "hello world";
size_t pos = text.rfind('o');
if (pos != std::string::npos) {
std::cout << "'o' found at position: " << pos << std::endl;
} else {
std::cout << "'o' not found." << std::endl;
}
In this case, the search is for the character `o`. The program prints the location of this character in the string, demonstrating the versatility of `rfind`.
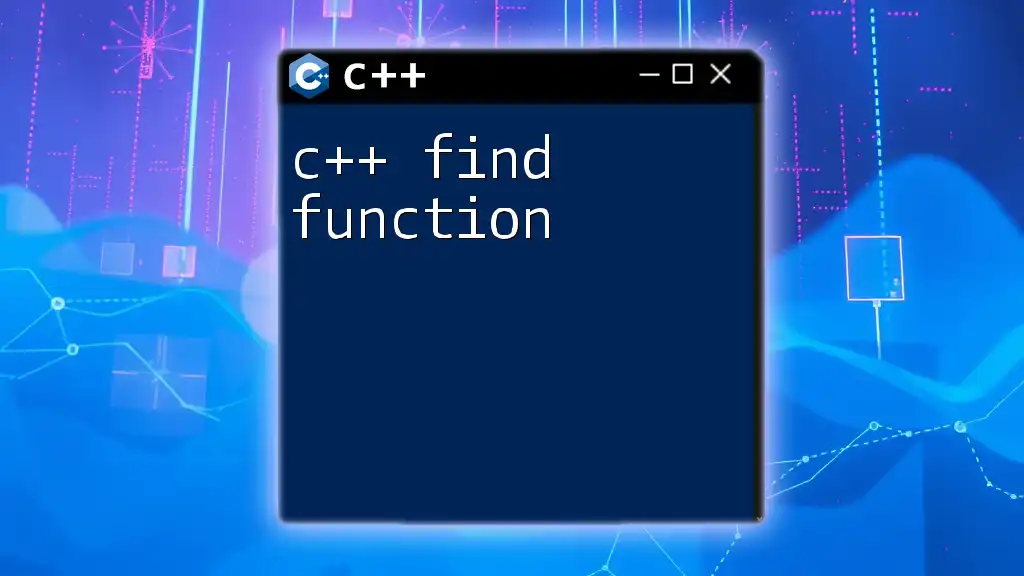
Advanced Usage of rfind
Searching from a Specific Position
One of the advantages of `rfind` is the ability to input a position from which to start the search. This feature can be particularly useful in larger strings where you may want to limit your search scope. Here's an example:
std::string str = "abcdefgabcdefg";
size_t pos = str.rfind('a', 10); // Search limited to the first 10 characters
if (pos != std::string::npos) {
std::cout << "'a' found at position: " << pos << std::endl;
} else {
std::cout << "'a' not found in the specified range." << std::endl;
}
In this code, the search for the character `a` is constrained to the first 10 characters of the string. This could yield a different result than searching the entire string.
Handling Cases where rfind Fails
Programming requires not just finding values but also managing cases where searches fail. When `rfind` does not find the requested substring, it returns `npos`. This requires checking the return value to handle errors appropriately. Here’s an example:
size_t pos = str.rfind('z'); // Searching for a character not present
if (pos == std::string::npos) {
std::cout << "'z' not found in the string." << std::endl;
}
This code checks if the result of `rfind` is `npos` and handles it cleanly by notifying the user.
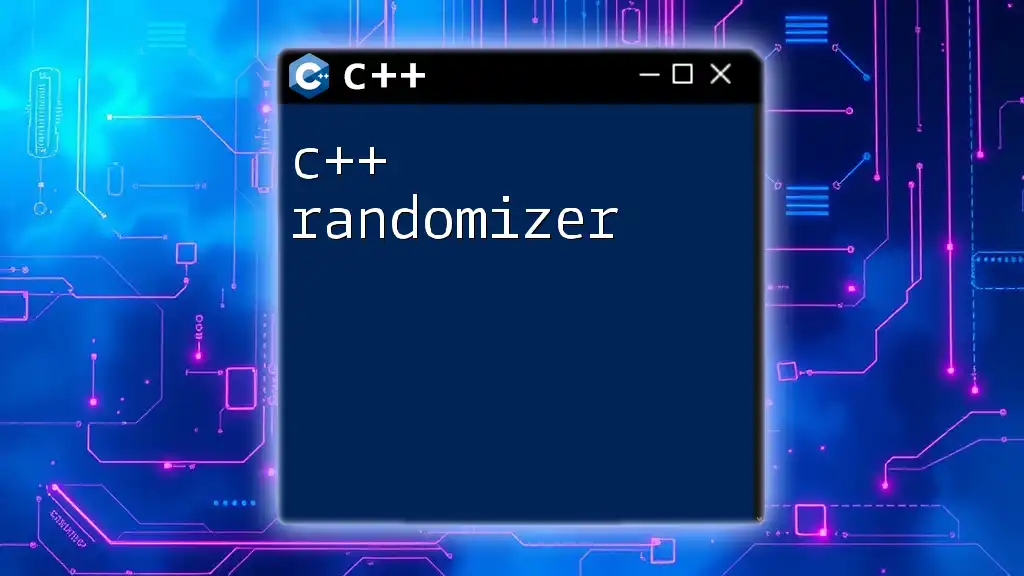
Common Use Cases for rfind
Text Processing Applications
The `rfind` function is especially useful in text processing applications. For instance, in word processors, users can implement this function to find the last occurrence of a word or character for editing purposes. The most recent search position may guide the user back to their last edit or selection.
Data Parsing
Another common application of `rfind` is in data parsing. Developers frequently encounter situations where they need to locate significant markers (like special characters or substrings) in logs or data streams to extract meaningful information. Using `rfind`, they can effectively find the last important entry, assisting in accurate data evaluation.
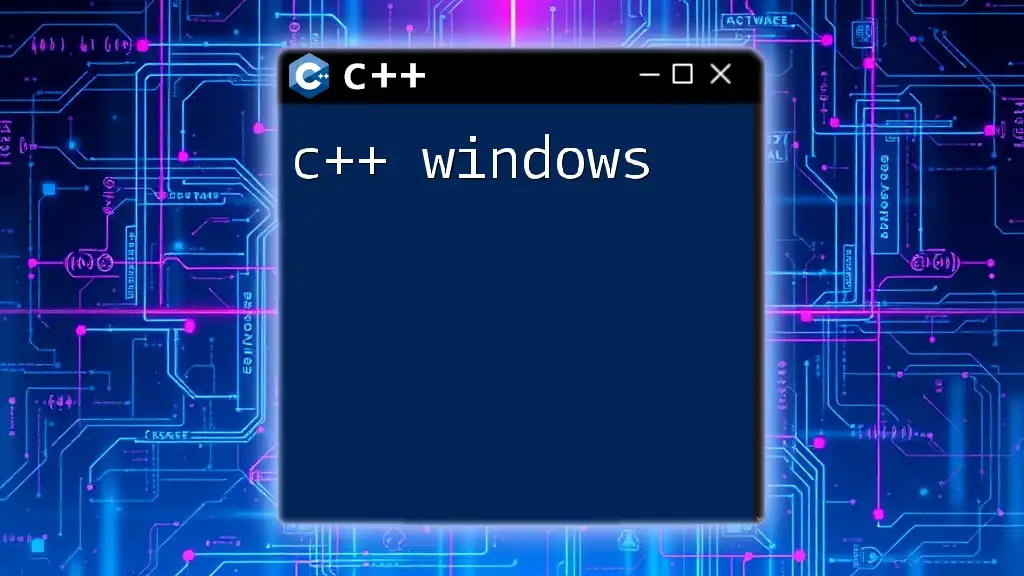
Performance and Best Practices
Time Complexity of rfind
The time complexity of `rfind` is linear, O(n), because it may have to traverse the entire searched portion of the string to find the last occurrence. Keep this in mind, especially when working with very long strings, as it may lead to performance bottlenecks.
Best Practices
When deciding between using `rfind` and `find`, consider the specific context of your search. If you need a recent instance of a substring, `rfind` is the clear choice. However, if your search does not require reverse searching, `find` may be more efficient. Additionally, always handle the `npos` return to avoid segmentation faults or unpredictable behaviors in your program.
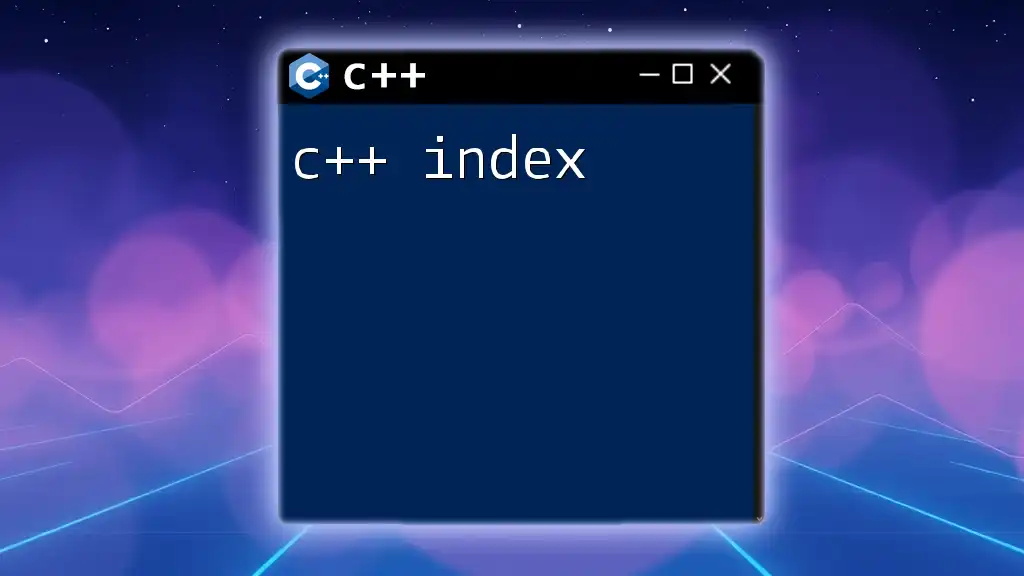
Conclusion
In summary, `c++ rfind` is an essential tool for any C++ developer working with string manipulation. Its simplicity and versatility make it invaluable when searching for substrings and characters efficiently. By understanding how to implement this function effectively, you will enhance your ability to handle strings in C++, leading to better and more robust applications.
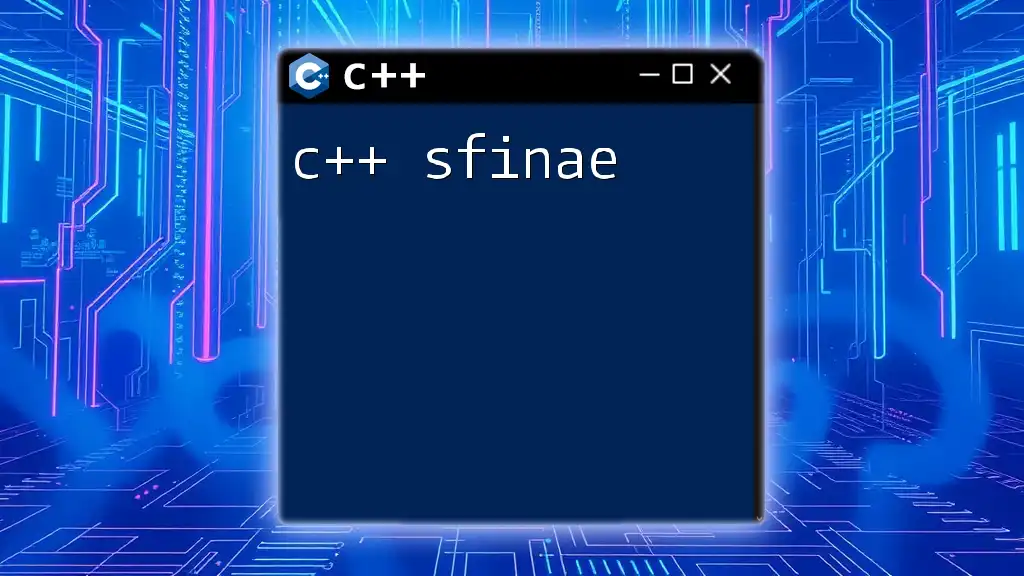
Additional Resources
For further learning about string manipulation in C++, consider exploring the official C++ documentation and tutorials that delve deeper into advanced string functions. Participating in coding forums or GitHub discussions can also provide valuable insights and community support as you continue to refine your C++ skills.
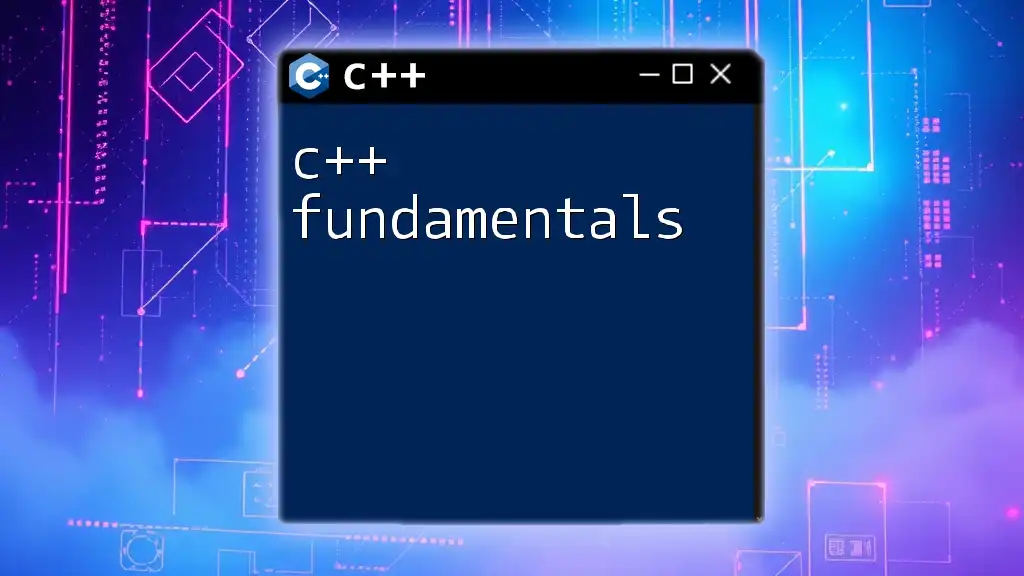
Call to Action
We encourage you to share your own code examples using `rfind` in the comments below. Join our mailing list for more concise C++ tips and tricks that will enhance your programming journey!