In C++, you can round a `double` value to the nearest integer using the `std::round` function from the `<cmath>` library.
#include <iostream>
#include <cmath>
int main() {
double value = 3.7;
double roundedValue = std::round(value);
std::cout << "Rounded Value: " << roundedValue << std::endl; // Outputs: Rounded Value: 4
return 0;
}
Understanding Double Data Type in C++
In C++, a double is a fundamental data type that represents a double-precision floating-point number. It is capable of holding a larger range of values than a float, making it more suitable for calculations that require increased precision. While a float typically uses 32 bits, a double allocates 64 bits, which helps mitigate errors in calculations that may arise from limited precision.
When deciding between `float` and `double`, it's essential to consider the significance of the numbers you are working with and the requirements of your application. For instance, when dealing with scientific calculations or any operations where precision is crucial, using a double is often preferred.
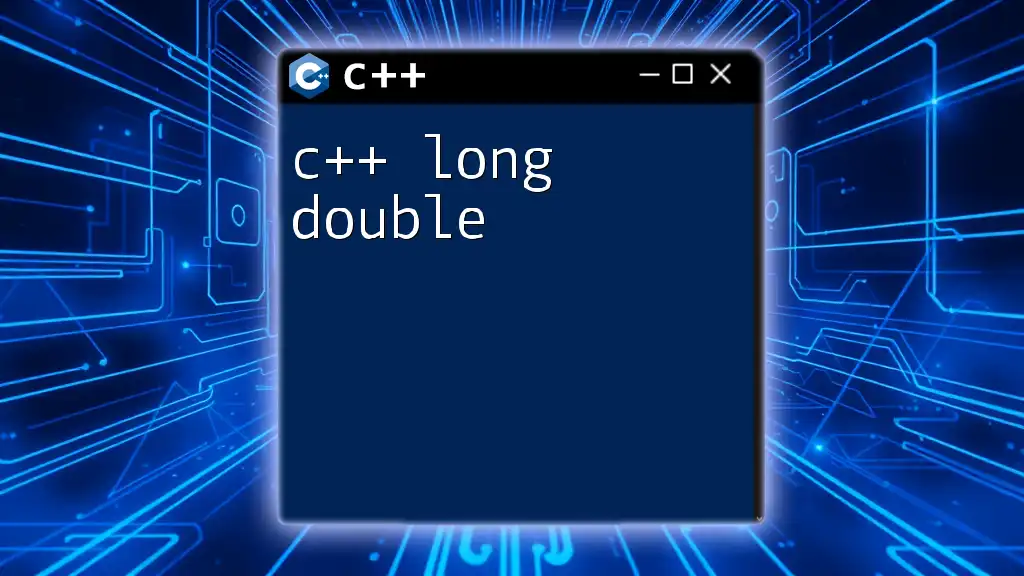
The Need for Rounding Doubles
Rounding becomes indispensable when performing numerical computations. Unrounded doubles can lead to unexpected behaviors, especially in cases like financial calculations, where precise values are crucial. For example, calculating taxes or interest rates with unrounded doubles could generate slight inaccuracies that compound over time.
A classic illustration of issues arising from floating-point arithmetic is the representation of decimal numbers. For instance, the double value `0.1` cannot be precisely represented in binary, leading to potential discrepancies in calculations.
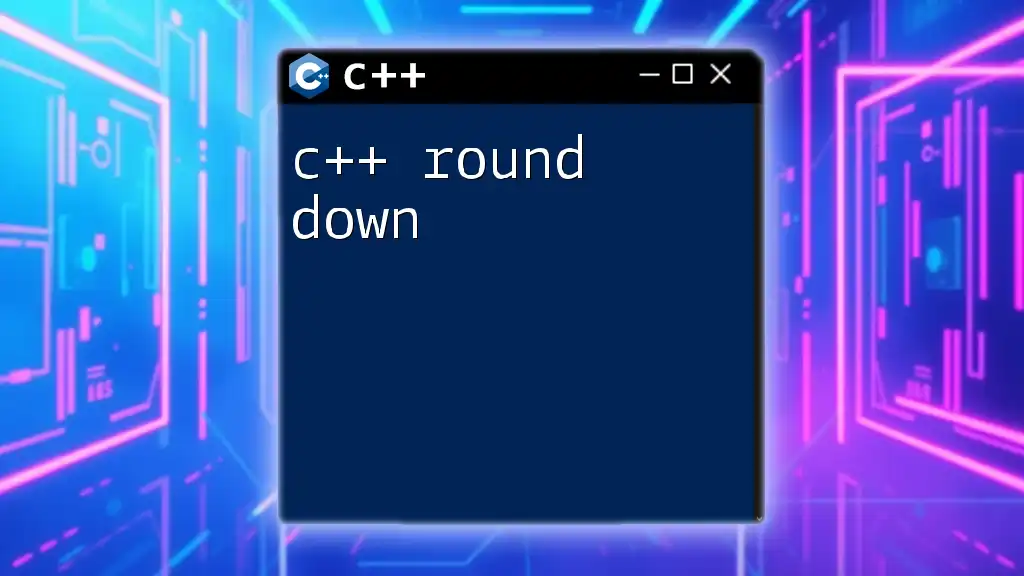
The C++ Standard Library and Rounding Functions
C++ provides various functions for rounding floating-point numbers through the `<cmath>` library. Understanding these functions is key to handling `c++ round double` effectively.
The round() Function
The `round()` function in C++ is used to round a floating-point number to the nearest integer. Its significance lies in its ability to handle both positive and negative values, rounding towards the nearest value based on standard rounding rules.
Syntax and Usage:
#include <iostream>
#include <cmath>
int main() {
double value = 3.6;
double roundedValue = round(value);
std::cout << "Rounded Value: " << roundedValue << std::endl; // Output: 4
return 0;
}
Moreover, it is essential to understand how `round()` treats negative numbers. For example, when using `round()` on -3.6, the output is -4 because it rounds away from zero.
double negativeValue = -3.6;
double roundedNegative = round(negativeValue);
// Output: -4
The floor() and ceil() Functions
The `floor()` and `ceil()` functions serve different purposes but are equally important when working with `c++ round double`.
Overview of floor(): This function rounds down to the nearest integer. It is particularly useful when you want to discard the decimal part completely.
double floorValue = floor(3.6); // Outputs 3
Overview of ceil(): In contrast, the `ceil()` function rounds up to the nearest integer, which is useful in various scenarios where rounding up is preferable.
double ceilValue = ceil(3.6); // Outputs 4
The trunc() Function
The `trunc()` function is another option that rounds a floating-point number towards zero by removing the decimal part without applying any rounding.
double truncValue = trunc(3.6); // Outputs 3
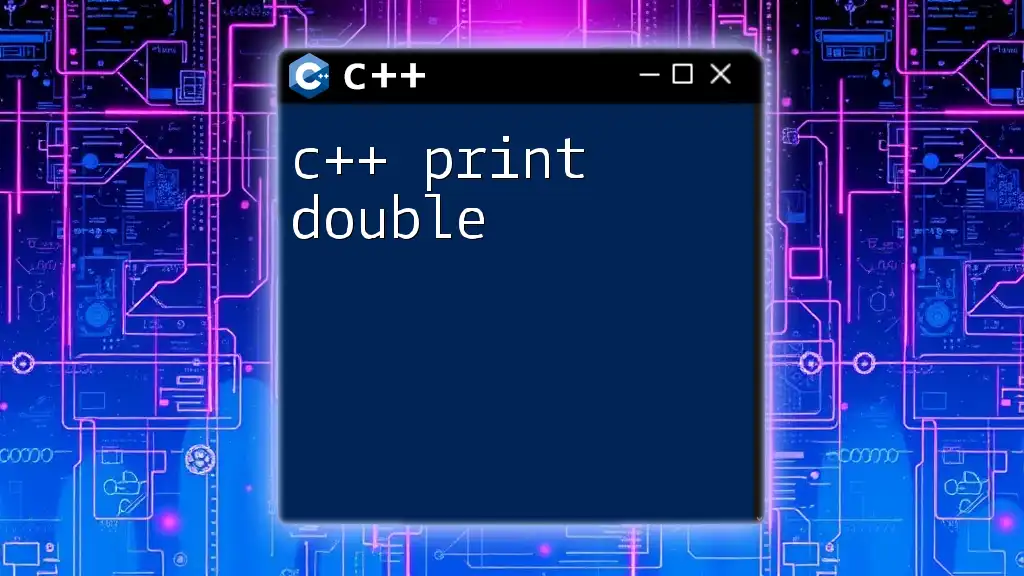
Custom Rounding Functions
In some cases, the default rounding methods may not suffice. Creating a custom rounding function allows for greater control over the rounding behavior, especially when rounding to a specific number of decimal places is necessary.
Implementing a Custom Round Function
Here’s an example of a simple custom function that rounds a double to a specified number of decimal places:
double customRound(double value, int decimalPlaces) {
double scale = pow(10, decimalPlaces);
return round(value * scale) / scale;
}
This function works by scaling the number up by the required decimal places, applying rounding, and then scaling it back down. This approach provides a flexible solution when working with the `c++ round double` needs.
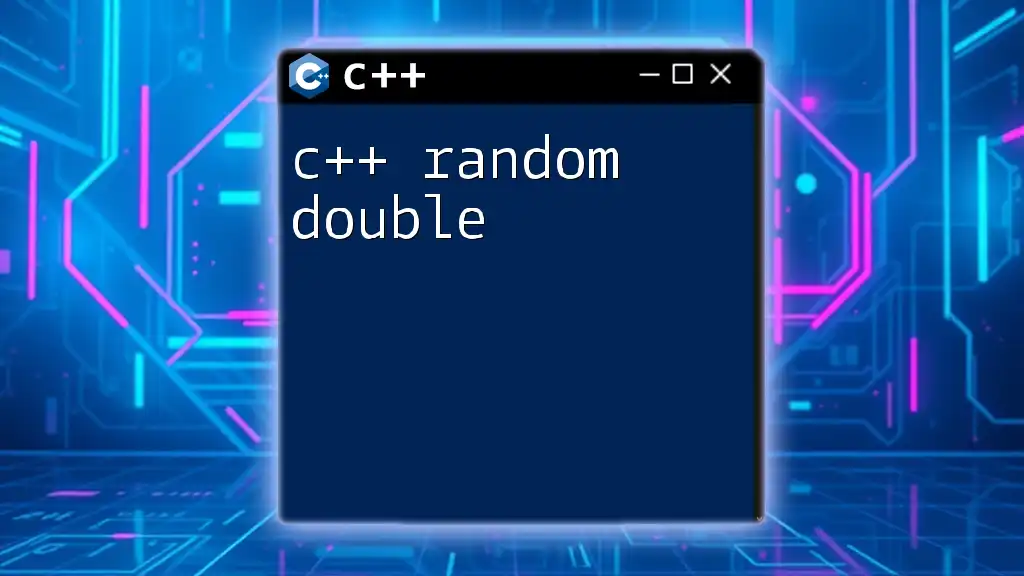
Using Rounding in Real-World Applications
Rounding is not merely an academic exercise; it has practical implications in various fields, including:
- Numerical analysis and scientific computations: When simulations require high precision, proper rounding can prevent significant errors.
- Financial applications: In business and finance, values like currency must often be rounded, especially when displaying prices or calculating totals to avoid fractional cents.
For example, in financial software, rounding to two decimal places is often necessary:
double price = 19.995;
double roundedPrice = customRound(price, 2); // Outputs 20.00
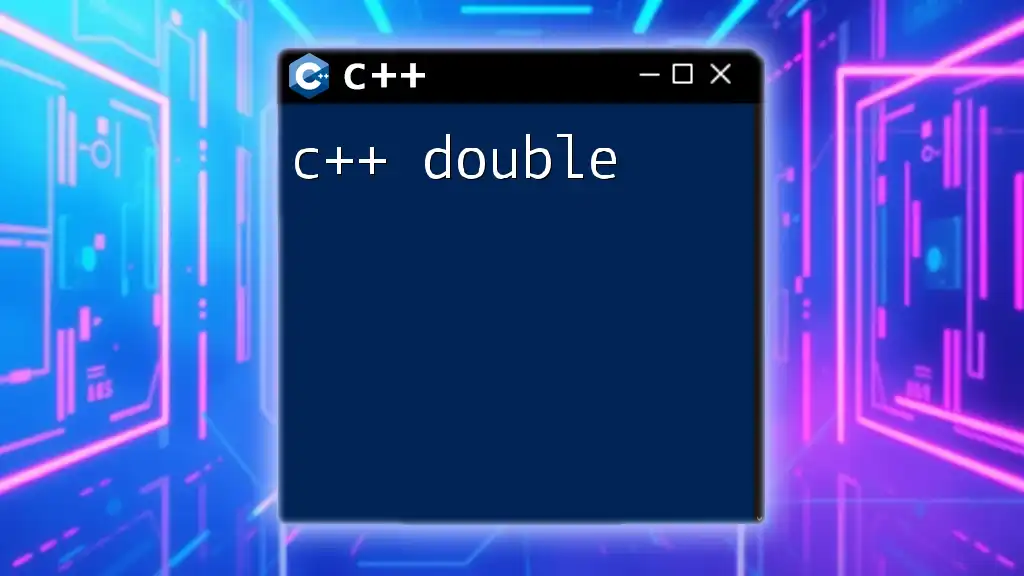
Common Errors and Best Practices
While working with `c++ round double`, several common errors may arise. One significant issue is the inherent inaccuracies of floating-point arithmetic. These can lead to unexpected results, especially when comparing double values.
To avoid pitfalls, always prefer double over float when precision matters. Additionally, always check rounding behavior, particularly around boundary values, to ensure your application maintains integrity.
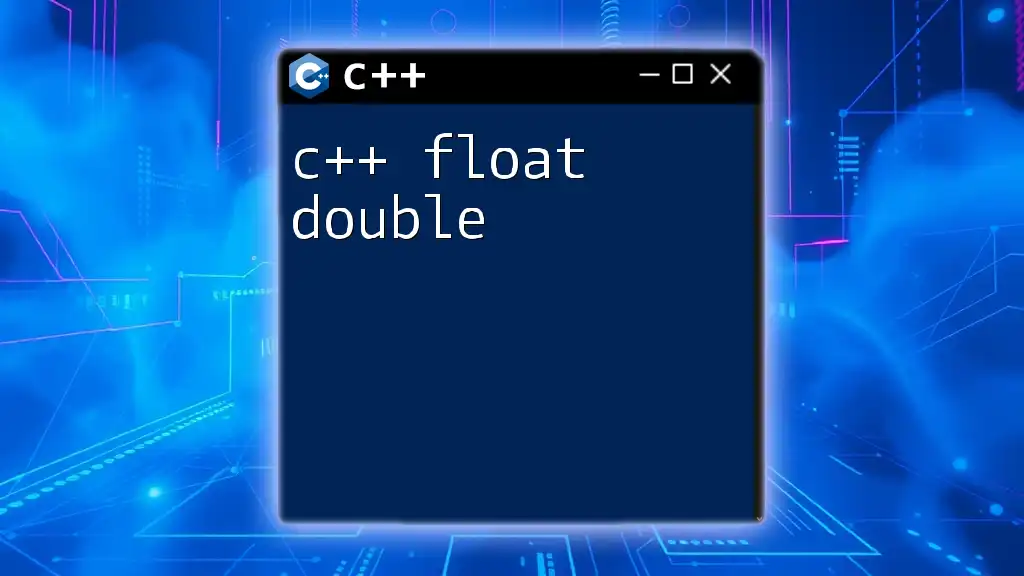
Conclusion
Rounding doubles in C++ is crucial for ensuring accuracy in numerical computations. Whether employing built-in functions like `round()`, `floor()`, and `ceil()`, or creating your custom functions, mastering these techniques enhances both the reliability and usability of your applications. By understanding and applying proper rounding techniques, you can mitigate precision issues and ensure that your calculations stand up to scrutiny.
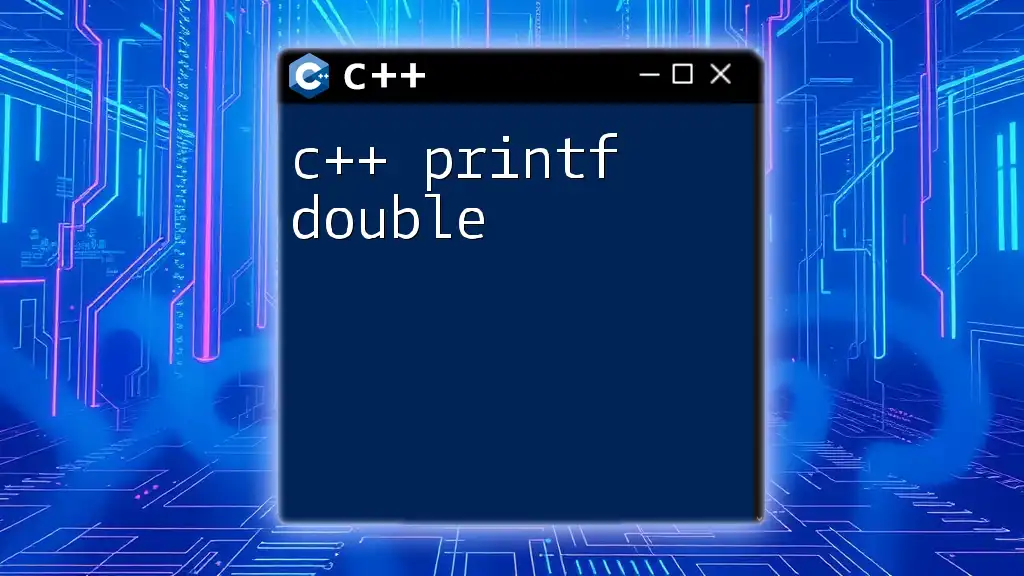
Further Reading
For additional information on rounding techniques and advanced C++ topics, consult the official C++ documentation, and consider exploring textbooks that delve deeper into numerical methods and data types in C++.
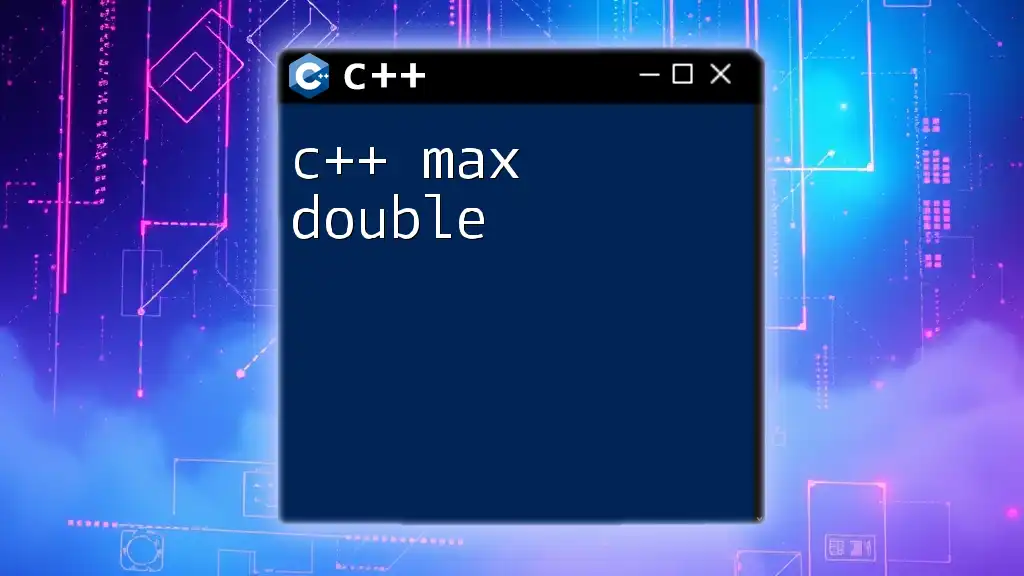
Call to Action
Have you encountered challenges with rounding in your C++ projects? We encourage you to share your experiences and questions as we continue to refine our rounding skills!