In C++, the `double` data type is used to represent floating-point numbers with precision, allowing for more accurate calculations than the `float` type.
Here’s a simple code snippet demonstrating the use of `double` with precision in C++:
#include <iostream>
#include <iomanip> // for std::setprecision
int main() {
double pi = 3.14159265358979323846;
std::cout << "Value of pi with 5 decimal places: " << std::setprecision(5) << pi << std::endl;
return 0;
}
Understanding Floating-Point Representation
Floating-point numbers are fundamental in programming, representing real numbers that allow for a fractional component. In C++, the floating-point representation is crucial for many applications, from scientific calculations to financial modeling. Precision and accuracy are essential when performing calculations with floating-point numbers to ensure correct results.

What is Double Precision?
Double precision refers to a floating-point format that uses more bits than single precision, allowing for greater accuracy. Specifically, a double-precision number typically allocates 64 bits to store its value, with 1 bit for the sign, 11 bits for the exponent, and 52 bits for the fraction (or mantissa).
This increased bit count translates to approximately 15 to 17 decimal digits of precision, making it suitable for calculations needing higher accuracy than what single-precision floats can provide, which store data in 32 bits.

Declaring and Initializing Double Variables
To utilize double precision in C++, you begin by declaring and initializing double variables. The syntax is straightforward:
double myNumber = 3.14159;
This line of code initializes a double variable called `myNumber` with the value of Pi. Double precision is especially beneficial in cases where the range and accuracy of the data are critical.

Characteristics of Double Precision in C++
When using double precision in C++, you benefit from a wider range and increased accuracy over the float type. A double can represent numbers as large as approximately ±1.7 × 10^308, and as small as ±5.0 × 10^-324. The additional bits for the fraction result in fewer rounding errors, making doubles ideal for more sensitive calculations.

How Precision is Defined in C++
In C++, precision is defined by the number of significant digits that a floating-point type can represent accurately. For double precision, this means that operations performed on double variables are typically accurate to 15 to 17 significant digits.
The IEEE 754 standard describes how floating-point arithmetic should be handled, ensuring consistent behavior across systems. Understanding this standard helps programmers predict floating-point behavior in computations.
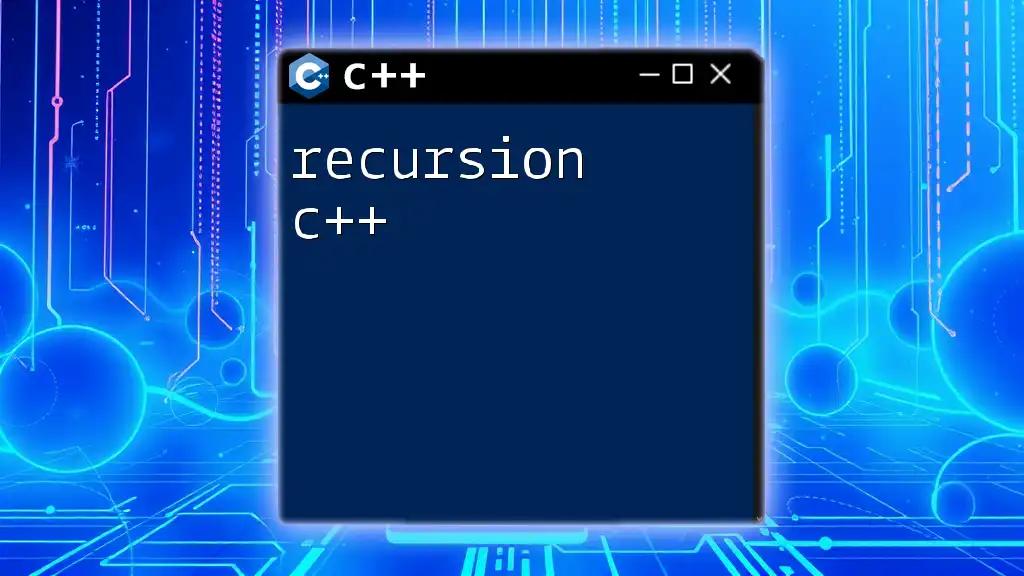
Precision Limitations
Despite the benefits of double precision, it's important to recognize its limitations. Floating-point arithmetic can lead to unexpected results due to rounding errors. For instance, consider the code:
double a = 0.1;
double b = 0.2;
double c = a + b; // might not equal 0.3 due to precision issues.
In this example, the variable `c` may not exactly equal `0.3` due to how 0.1 and 0.2 are represented in binary. Thus, it is essential to account for potential inaccuracies.
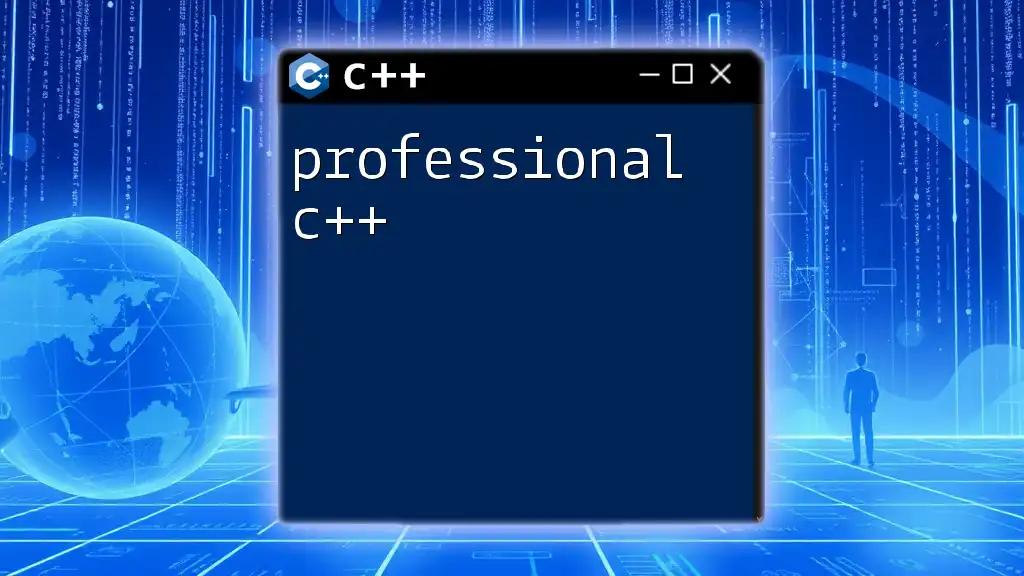
Calculating with Double Precision
When performing mathematical operations using double precision, you can expect accurate results, provided you are aware of its limitations. Here’s an example showing a calculation with double:
double a = 1.0 / 3.0;
std::cout << "Double precision result: " << a << std::endl;
This code prints the result of `1/3`, demonstrating the precision of double values in C++.
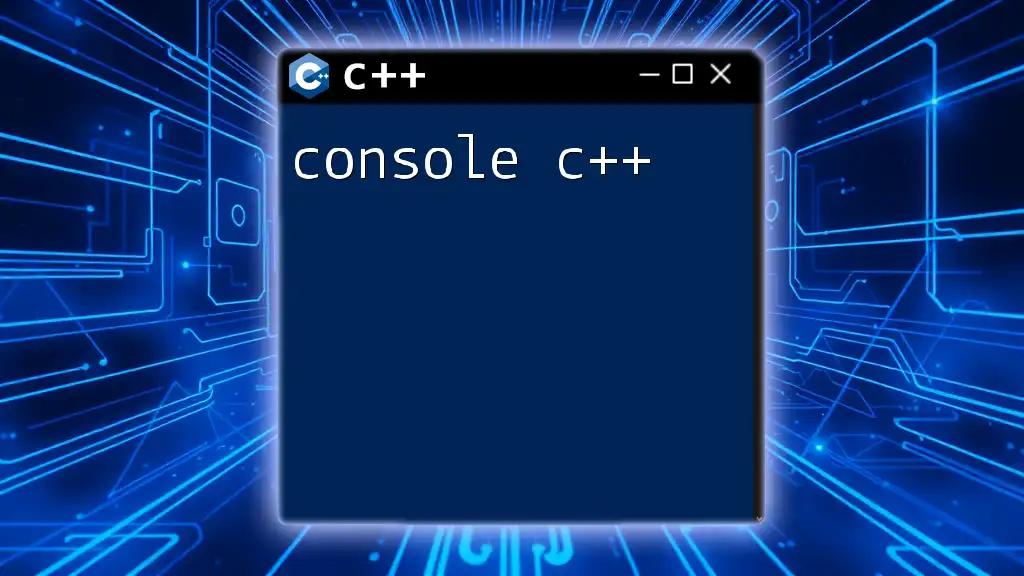
Utilizing Libraries for Enhanced Precision
Sometimes, the inherent precision of doubles might not suffice for very sensitive applications, such as cryptography or high-precision financial calculations. In such cases, utilizing libraries that offer greater precision, like Boost Multiprecision, is advantageous.
Below is an example of how to implement Boost to handle high-precision calculations:
#include <boost/multiprecision/cpp_dec_float.hpp>
using namespace boost::multiprecision;
cpp_dec_float_50 pi("3.141592653589793238462643383279502884");
std::cout << "High precision Pi: " << pi << std::endl;
This code demonstrates using the Boost library to store and manipulate Pi with remarkable precision, far exceeding the capabilities of standard doubles.

Common Precision Problems
When working with double precision, you may encounter various floating-point errors and rounding issues. These limitations can lead to unexpected results, especially in equality comparisons. For example:
double x = 0.1 + 0.2;
if (x == 0.3) {
std::cout << "They are equal!" << std::endl;
}
You may be surprised to find that this comparison does not always yield true due to floating-point representation errors.

Strategies to Mitigate Precision Errors
To address floating-point inaccuracies, several strategies can be employed:
- Rounding Techniques: You can use rounding functions to ensure results conform to expected values. For instance, using `std::round()`:
double value = 2.675;
double roundedValue = std::round(value * 100.0) / 100.0; // Rounds to 2.68
- Tolerance Levels: When comparing floating-point values, instead of using direct equality, define a small epsilon value within which two numbers are considered equal:
double epsilon = 1e-10;
if (fabs(x - 0.3) < epsilon) {
std::cout << "They are close enough!" << std::endl;
}
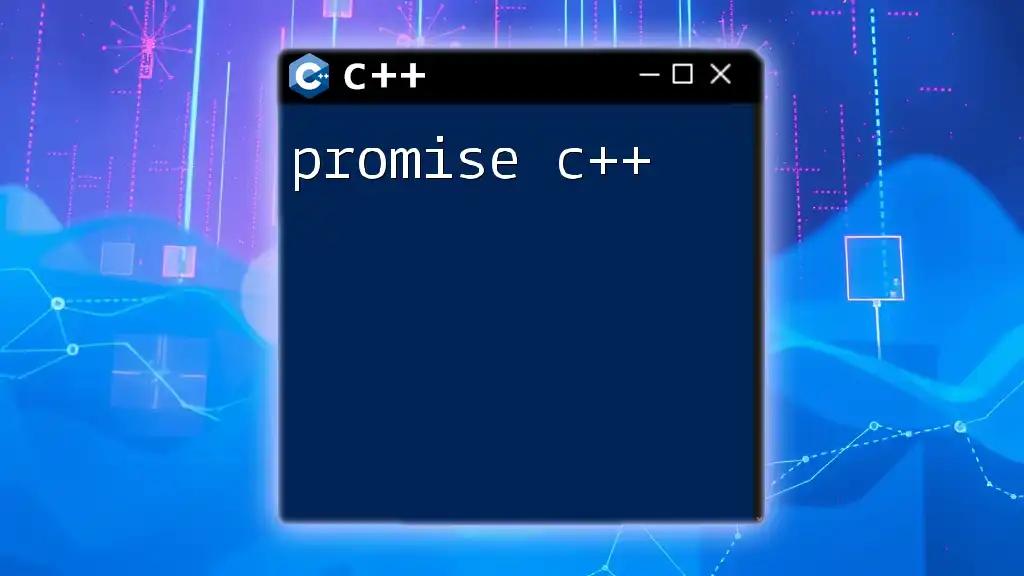
Double vs Float
When deciding between double and float, consider the required precision and performance. While double provides greater accuracy due to its higher number of significant digits, float may be sufficient for less exact calculations and can offer performance benefits in terms of speed and memory consumption. In performance-critical applications, especially those with large datasets (like graphics), using float may be more appropriate.
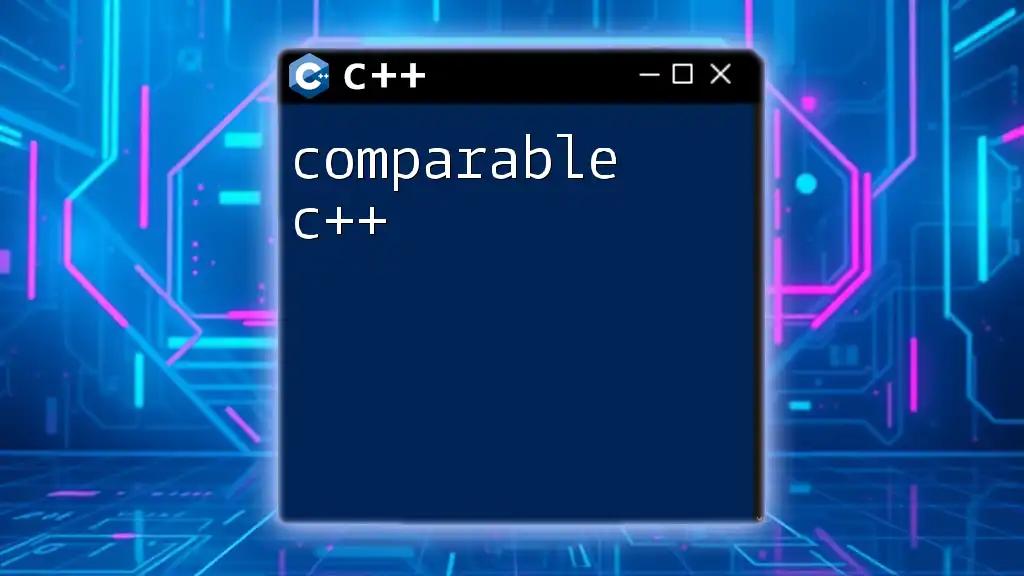
When to Use Double Precision
Double precision shines in scenarios where accuracy is crucial. Here are some scenarios where it’s preferred:
- Scientific Calculations: In research, slight differences in computed values can significantly affect results.
- Financial Applications: Precise monetary calculations are essential to avoid costly errors.
- Complex Simulations: In fields like physics and engineering, high-precision calculations ensure realistic models.
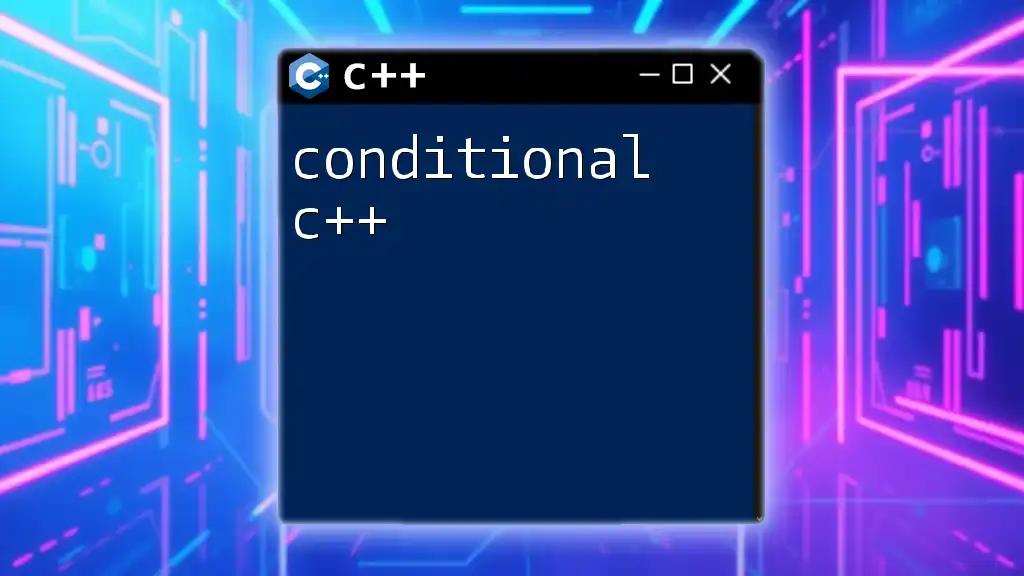
Summary of Key Points
Understanding precision double c++ is fundamental for programming tasks involving numerical computations requiring high accuracy. By recognizing how floating-point representation works, knowing the range, and mastering the precise usage of double variables, one can mitigate common pitfalls and employ best practices for numerical calculations.

Further Resources
For those interested in delving deeper into double precision and C++ programming, consider checking official C++ documentation, advanced tutorials on floating-point arithmetic, and specialized books on numerical algorithms.
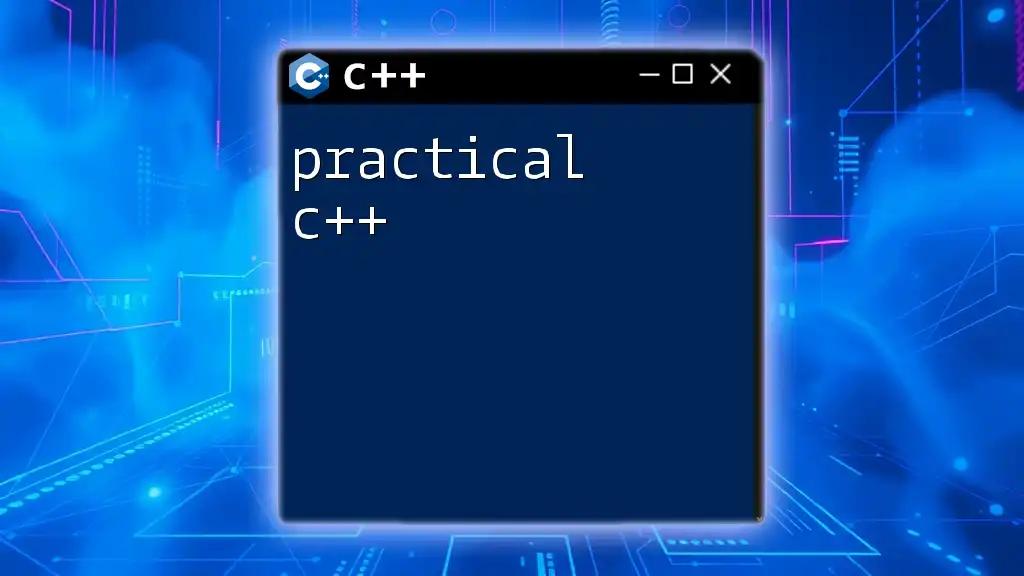
Encouraging Readers to Learn More
As you explore the intricacies of precision double in C++, remember that gaining a thorough understanding of data types will enhance your programming skills. Join us in our courses and tutorials to master C++ and improve your coding proficiency today!