ASCII code in C++ refers to the standard character encoding used to represent text, where each character is assigned a unique integer value, allowing programmers to manipulate and display text using its corresponding ASCII values.
Here’s a simple C++ code snippet to print the ASCII value of a character:
#include <iostream>
int main() {
char ch = 'A'; // character
std::cout << "The ASCII value of " << ch << " is " << int(ch) << std::endl;
return 0;
}
Introduction to ASCII Code
What is ASCII Code?
ASCII (American Standard Code for Information Interchange) is a character encoding standard used for representing text in computers and other devices that use text. It was developed in the early 1960s and provides a common encoding scheme that allows computers to communicate with one another effectively. Each letter, digit, and symbol is assigned a unique numerical value ranging from 0 to 127.
Why Use ASCII Code in C++?
ASCII code is particularly essential in C++ programming as it underlies the representation of characters in the language. Understanding ASCII allows programmers to manipulate and store characters more efficiently, perform string operations, and handle low-level data processing tasks effectively. With a solid foundation in ASCII code, developers can also troubleshoot character encoding issues that may arise when working with different data formats.

Understanding ASCII Values
ASCII Characters and Their Corresponding Values
ASCII encompasses a range of characters classified into two main categories: printable and non-printable. Printable characters include letters (A-Z, a-z), digits (0-9), punctuation marks, and a few special symbols. Non-printable characters include control codes, such as carriage return and newline.
Here is a brief overview of some common ASCII characters and their corresponding values:
- A-Z: 65-90
- a-z: 97-122
- 0-9: 48-57
- Space: 32
- newline: 10 (LF)
- tab: 9 (HT)
Common ASCII Codes for C++ Programmers
Several ASCII codes frequently pop up in programming tasks. Recognizing these codes can enhance code readability and improve debugging efficiency. For example, the newline and tab characters are often used for formatting output, while backspace (ASCII 8) is useful for text editing applications.
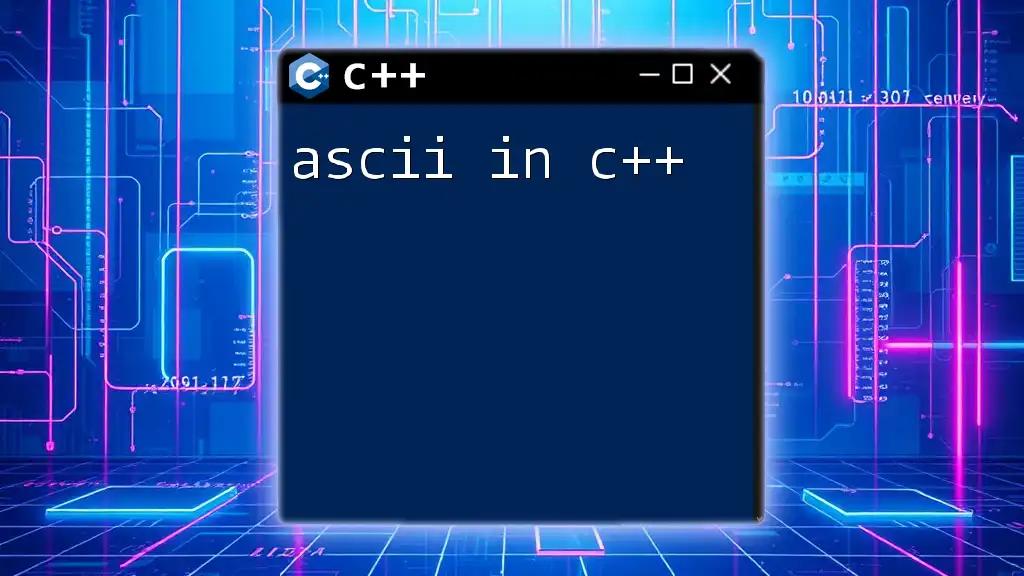
Working with ASCII Code in C++
How to Input ASCII Codes in C++
To work with ASCII codes in C++, you can utilize character literals that directly represent their ASCII value. For instance, the character 'A' can be represented by its ASCII value of 65. With C++, you can also convert between character literals and their ASCII integer representations.
#include <iostream>
using namespace std;
int main() {
int asciiValue = 65; // Code for 'A'
char character = static_cast<char>(asciiValue);
cout << "The character for ASCII value " << asciiValue << " is " << character << endl;
return 0;
}
In this example, the program outputs the character representation for the ASCII value 65, which is 'A'.
Outputting ASCII Codes in C++
You might want to display the ASCII values of various characters in your programs. The following code snippet demonstrates how to print the ASCII value of each character in a string:
#include <iostream>
#include <string>
using namespace std;
int main() {
string text = "Hello";
for (char c: text) {
cout << "ASCII value of " << c << " is " << static_cast<int>(c) << endl;
}
return 0;
}
This program will iterate through each character in the string "Hello" and print its ASCII value, showcasing the relationship between characters and their ASCII representations.

ASCII Code and String Manipulation
Using ASCII for Character Comparisons
ASCII values provide a mechanism for comparing characters in C++. When you compare characters, you're effectively comparing their underlying ASCII values. Here’s a quick example:
#include <iostream>
using namespace std;
int main() {
char a = 'a';
char b = 'A';
if (a > b) {
cout << a << " is greater than " << b << " based on ASCII values." << endl;
} else {
cout << b << " is greater than " << a << " based on ASCII values." << endl;
}
return 0;
}
In this example, since the ASCII value of 'a' (97) is greater than that of 'A' (65), the output will correctly indicate that 'a' is greater than 'A'.
Transforming Characters Using ASCII Values
You can also transform characters using their ASCII codes. For instance, converting an uppercase letter to a lowercase letter involves changing its ASCII value. This can be done by adjusting the ASCII value by 32. Below is an example of how to toggle the case of a character based on its ASCII value:
#include <iostream>
using namespace std;
char toggleCase(char c) {
if (c >= 'a' && c <= 'z') {
return c - 32; // Convert to uppercase
} else if (c >= 'A' && c <= 'Z') {
return c + 32; // Convert to lowercase
}
return c; // Return the character unchanged if not an alphabet
}
int main() {
char inputChar = 'a';
cout << "Toggled case: " << toggleCase(inputChar) << endl;
return 0;
}
This function takes a character as input and toggles its case based on ASCII values.
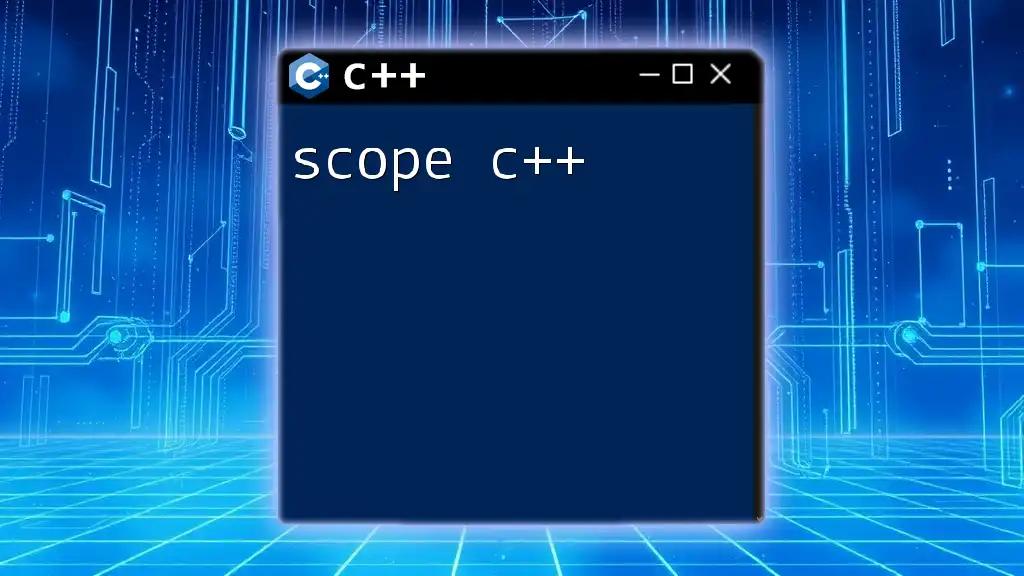
Advanced Usage of ASCII in C++
Encoding and Decoding Messages with ASCII
One of the fascinating applications of ASCII is in encoding and decoding messages. Simple encoding techniques, such as the Caesar cipher, utilize ASCII values to shift characters by a specified number. Here's how you can implement a basic Caesar cipher in C++:
#include <iostream>
using namespace std;
string encodeMessage(const string& input, int shift) {
string result = "";
for (char c : input) {
if (isalpha(c)) {
char base = islower(c) ? 'a' : 'A';
result += char(int(base + (c - base + shift) % 26));
} else {
result += c; // Non-alphabetic characters remain unchanged
}
}
return result;
}
int main() {
string message = "Hello, World!";
cout << "Encoded message: " << encodeMessage(message, 3) << endl;
return 0;
}
In this example, the `encodeMessage` function shifts characters based on the specified shift value, preserving non-alphabetic characters.
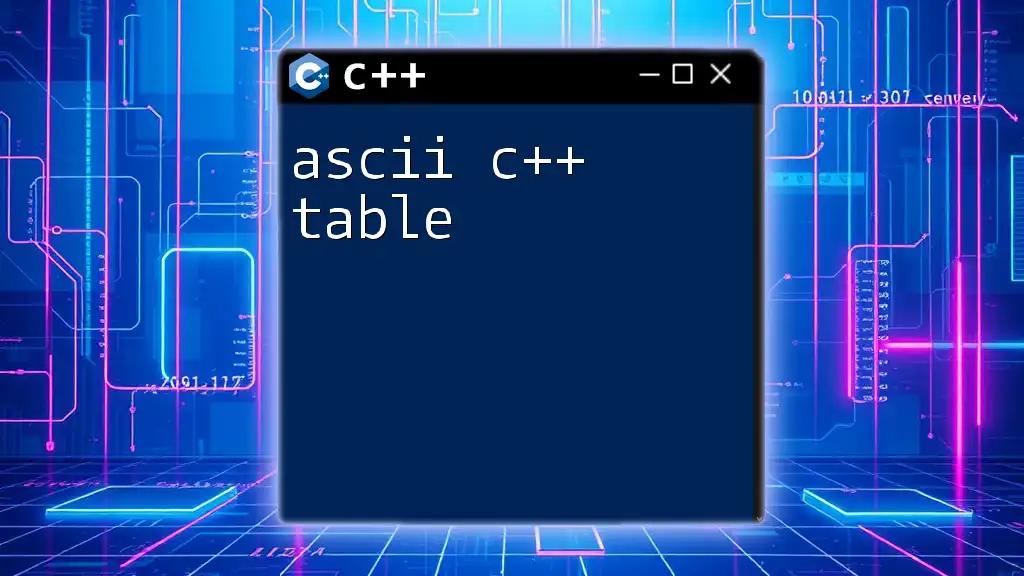
Conclusion
Recap of Key Points
In summary, understanding ASCII code in C++ is fundamental for manipulating characters, performing string operations, and solving coding challenges effectively. The examples demonstrated the importance and utility of ASCII values in various programming contexts, from character comparison to encoding messages.
Future Topics Related to ASCII in Programming
In future articles, we can explore more complex character encoding systems such as Unicode, which expands upon ASCII by accommodating a wider variety of characters, including those from different languages. We can also discuss the significance of character encoding when dealing with files and data transmission.
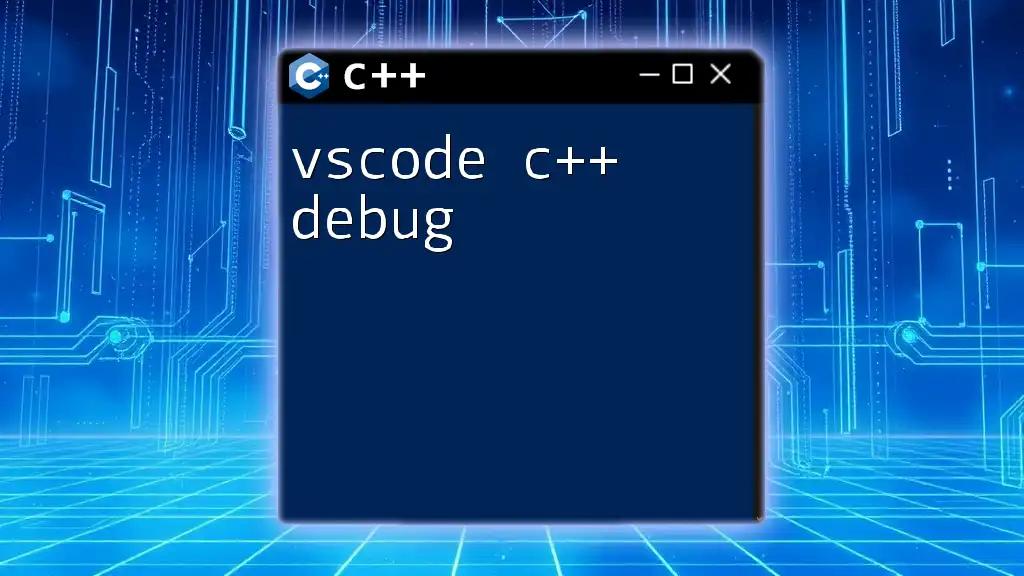
Additional Resources
References for Further Learning
For further learning, you can explore online resources such as C++ documentation, ASCII character tables, and online programming communities. Engaging in forums and discussions will enhance your learning experience as you progress in understanding ASCII and its applications in C++.
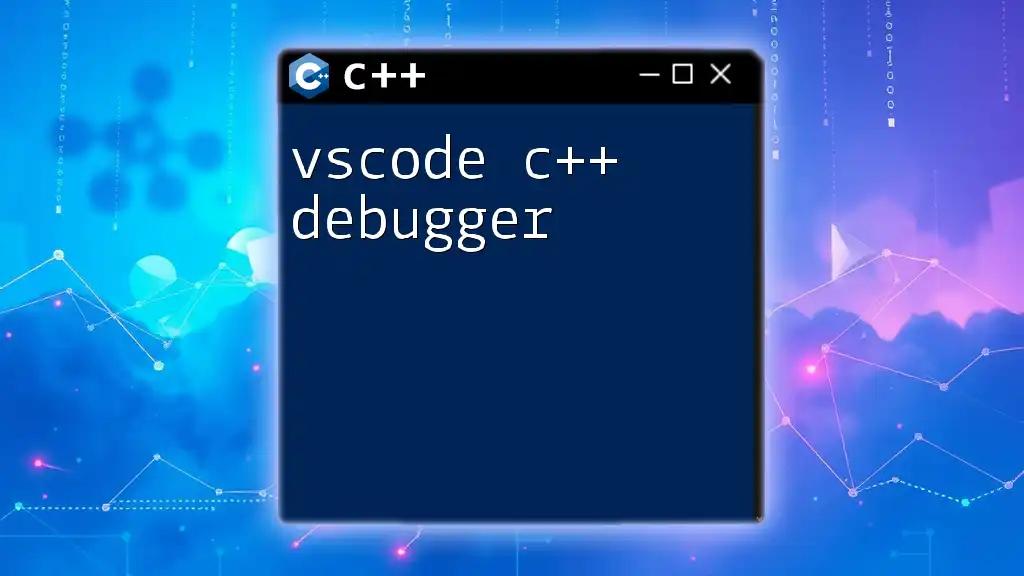
Call to Action
Join Us for More Learning
Be sure to subscribe to receive updates on new articles focused on enhancing your C++ skills. Start applying the concepts of ASCII in your coding practice, and watch how your proficiency grows!