In C++, ASCII (American Standard Code for Information Interchange) characters can be easily manipulated using their integer values for various purposes, such as displaying characters or converting between characters and their ASCII values.
Here's a simple code snippet to demonstrate how to print the ASCII value of a character:
#include <iostream>
int main() {
char ch = 'A';
std::cout << "The ASCII value of " << ch << " is " << static_cast<int>(ch) << std::endl;
return 0;
}
What is ASCII?
ASCII, or the American Standard Code for Information Interchange, is a character encoding standard that represents text in computers. Originally developed in the 1960s, ASCII assigns a numerical value to each character, allowing diverse computing systems to share information. This is particularly important for standardizing communication between devices.
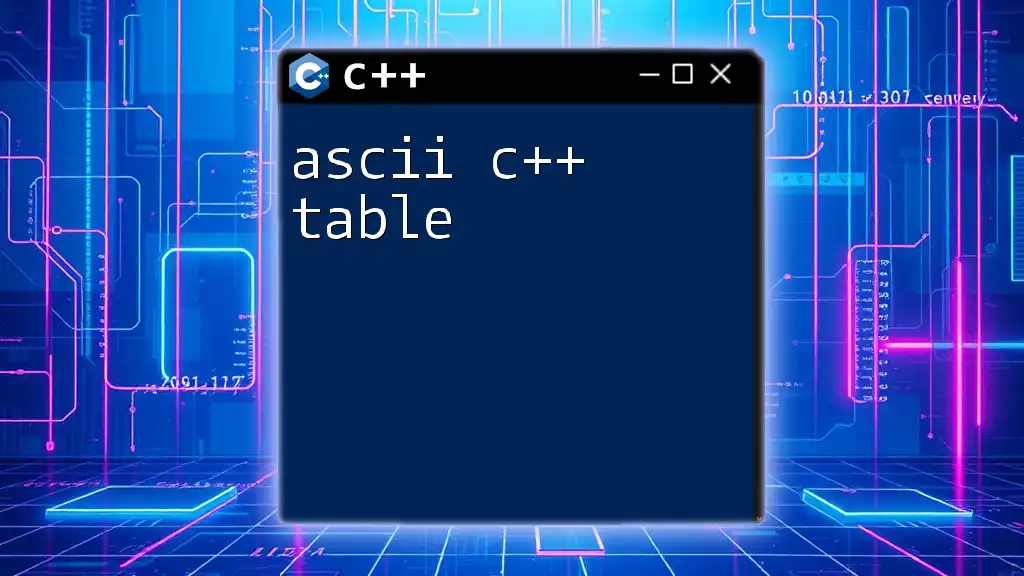
Why Use ASCII in C++?
In the realm of C++ programming, ASCII serves as a foundational tool for simplifying character representation. Understanding ASCII can significantly enhance string manipulation, data storage, and even data transmission, empowering programmers to efficiently handle textual information.
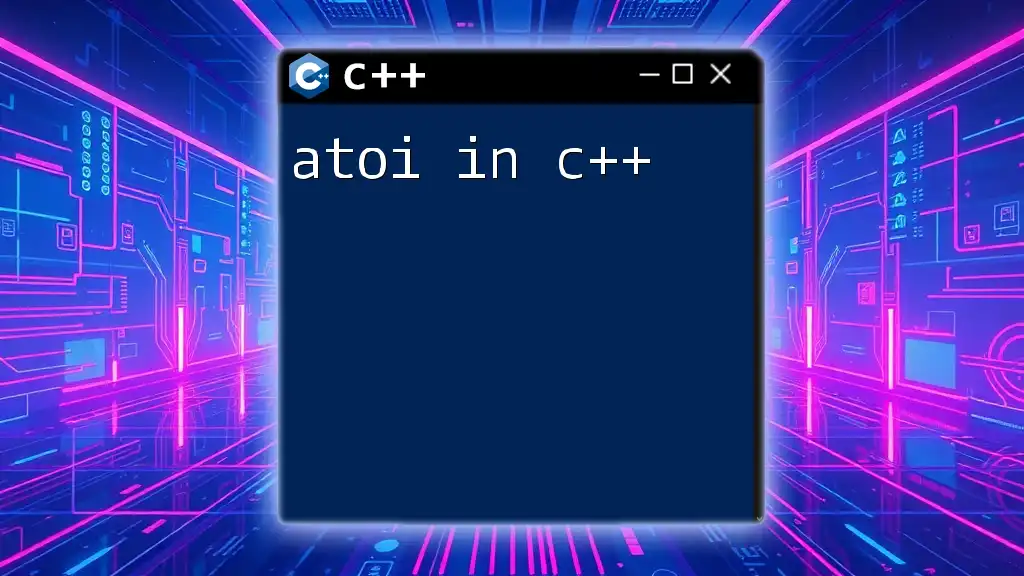
Understanding ASCII Values
ASCII Character Set Overview
ASCII encompasses a range of characters, primarily covering the first 128 characters, which include:
- Control Characters (0-31): Non-printable characters used for formatting and control purposes.
- Printable Characters (32-126): Included are numbers (0-9), uppercase letters (A-Z), lowercase letters (a-z), and various symbols.
- Extended ASCII (128-255): This includes additional characters specific to certain languages and graphical symbols, though not all systems interpret these uniformly.
How ASCII Maps to C++ Data Types
In C++, the `char` data type is often employed to store ASCII characters. When you assign a character to a `char` variable, it is represented internally by its corresponding ASCII integer value. For example, the character 'A' is represented by the integer 65.
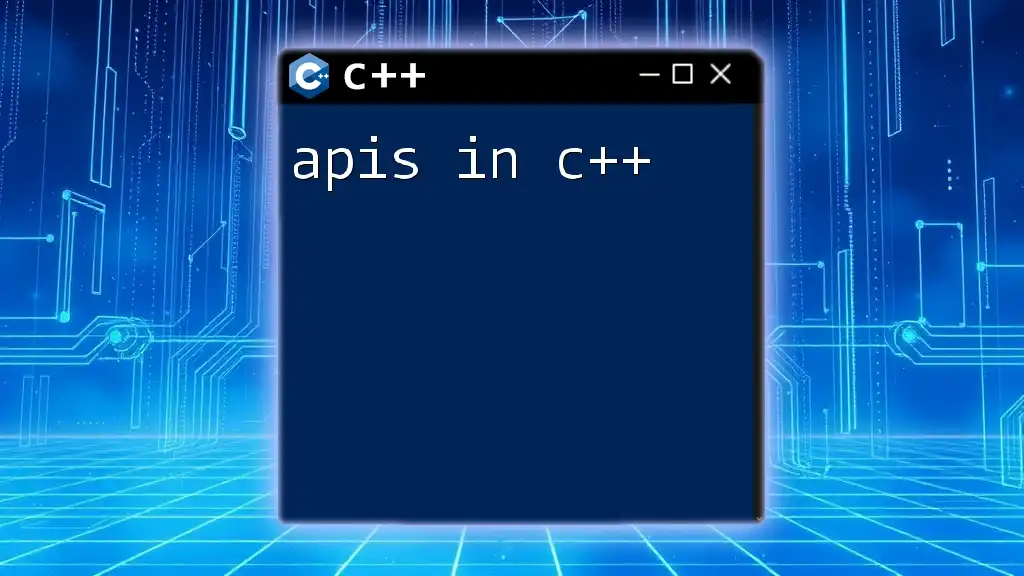
Working with ASCII in C++
Outputting ASCII Characters
One of the simplest ways to explore ASCII in C++ is by outputting the characters along with their corresponding ASCII values. Here's an example:
#include <iostream>
int main() {
for (int i = 0; i < 128; i++) {
std::cout << "ASCII " << i << ": " << static_cast<char>(i) << std::endl;
}
return 0;
}
This snippet loops through all the ASCII values from 0 to 127 and prints them out. Using `static_cast<char>` is crucial here as it converts the integer into its corresponding character representation, allowing us to display both the numerical and character forms seamlessly.
Getting ASCII Values from Characters
To understand how characters are encoded in ASCII, you can convert a character to its ASCII value. Consider the following example:
#include <iostream>
int main() {
char c = 'A';
int asciiValue = static_cast<int>(c);
std::cout << "ASCII value of " << c << " is " << asciiValue << std::endl;
return 0;
}
In this code, the character 'A' is cast to an integer, exposing its ASCII value, which is 65. This conversion is vital for scenarios where comparisons or calculations based on ASCII values are necessary.
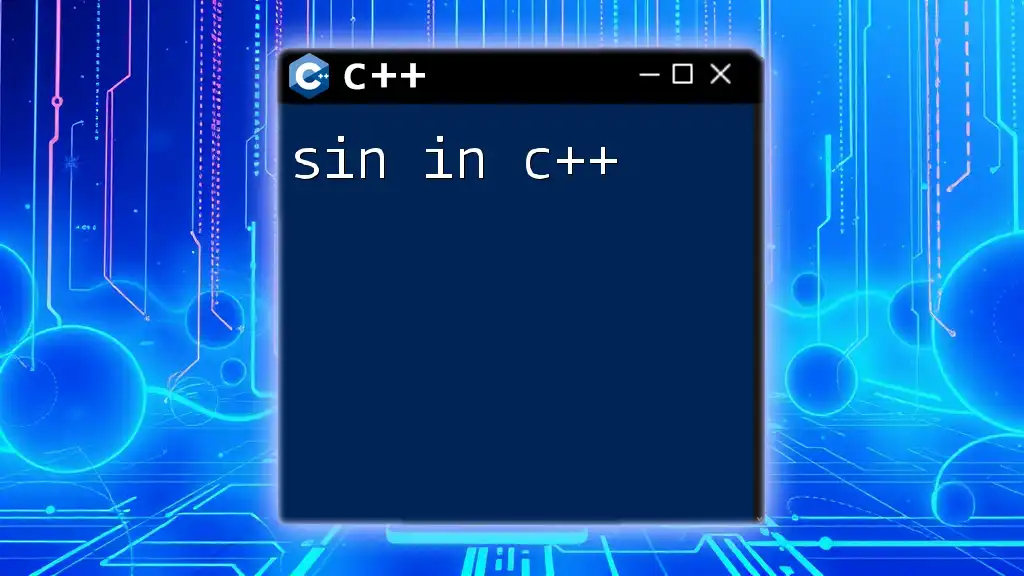
ASCII and String Manipulation
String Initialization with ASCII
Using ASCII values can also facilitate string initialization. Here's how:
#include <iostream>
#include <string>
int main() {
std::string asciiString = "Hello, ASCII!";
std::cout << "The string is: " << asciiString << std::endl;
return 0;
}
This example demonstrates a straightforward initialization of a string containing printable ASCII characters. Understanding how strings correlate with ASCII enables effective text handling, ensuring data integrity throughout various programming tasks.
Modifying Strings with ASCII
You can also manipulate strings based on their ASCII values for customized transformations. Here's an example:
#include <iostream>
#include <string>
void incrementString(std::string& str) {
for (char& c : str) {
c++; // Increment character ASCII values
}
}
int main() {
std::string original = "ABC";
incrementString(original);
std::cout << "Incremented String: " << original << std::endl;
return 0;
}
In this code, the function `incrementString` takes a string and increments each character's ASCII value. The result is that every character is raised by one in the ASCII chart, transforming "ABC" to "BCD". This technique is prevalent in encryption and encoding practices.
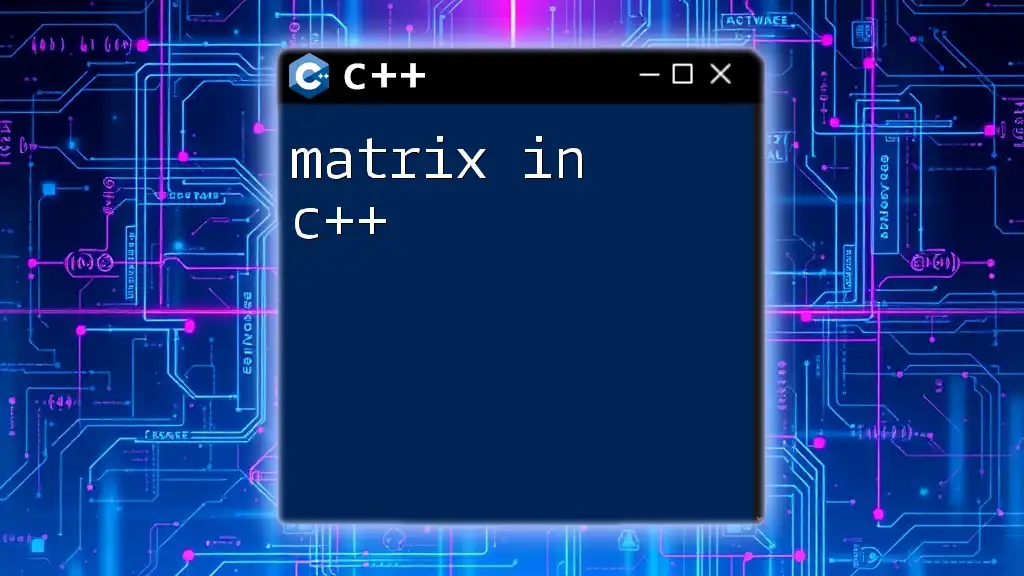
ASCII Art in C++
Creating Simple ASCII Art
ASCII isn't limited to just character representation; it can also be used creatively to generate art. Here’s how to create basic ASCII art:
#include <iostream>
int main() {
std::cout << " * " << std::endl;
std::cout << " *** " << std::endl;
std::cout << "*****";
return 0;
}
This snippet demonstrates the construction of a simple pyramid shape using asterisks. While ASCII art might find more relevance in projects like text-based games, it can also serve as a fun way to beautify console outputs.
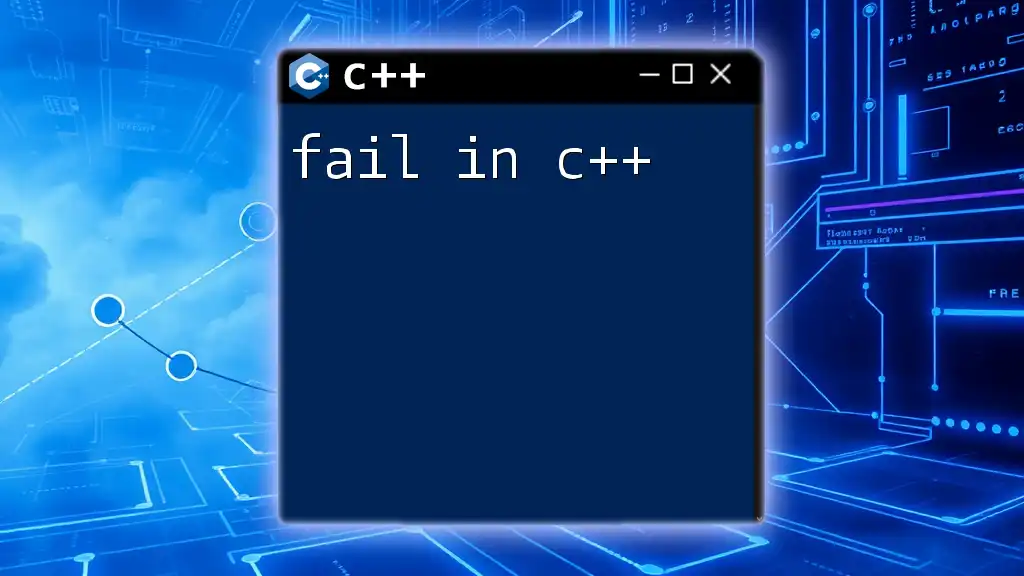
Practical Applications of ASCII in C++
Using ASCII for Data Encoding
The ASCII standard plays a pivotal role in data encoding. It ensures that different systems can read and write text data consistently, making it crucial for network communication and various file formats. When transferring data, ASCII helps maintain clarity and consistency, which is vital for correctness.
ASCII in Text-Based Games
ASCII art finds its footing in text-based games, where graphics are represented using characters. For example, creating game elements like characters or obstacles can be achieved using print statements to depict various ASCII shapes, enriching the user experience despite the constraints of a console application.
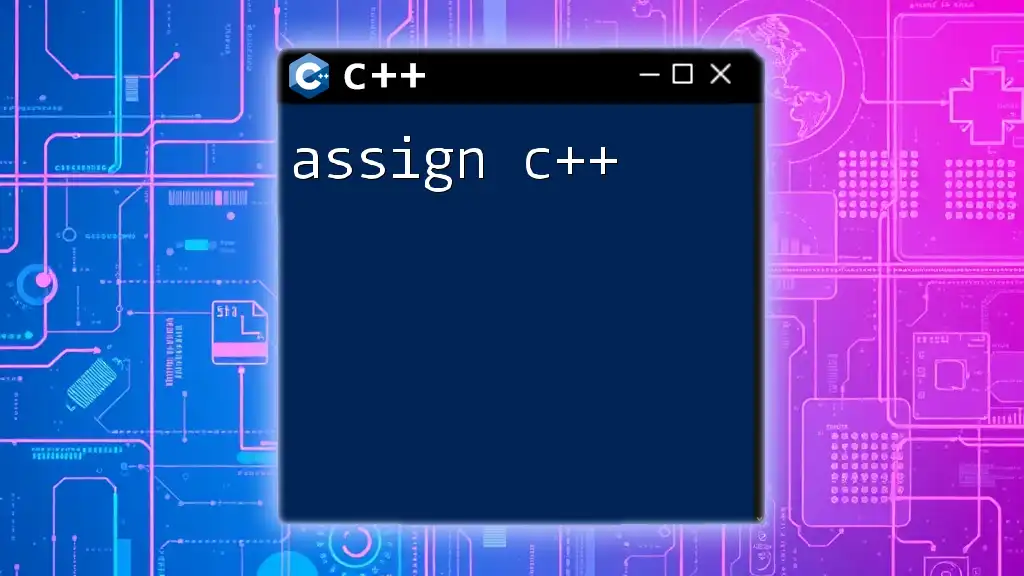
Debugging and Common Issues
Common Problems with ASCII in C++
Working with ASCII characters can lead to issues, especially when dealing with non-printable ASCII characters (0-31). These characters can affect program flow and formatting unexpectedly. Understanding and anticipating these pitfalls ensures stability in your application’s performance.
Tips for Effective Debugging
When encountering ASCII-related bugs, here are some tips for debugging effectively:
- Check for Encoding Issues: Ensure your files and data streams are properly encoded.
- Utilize Print Statements: Leveraging print statements to log ASCII values can shed light on unintended manipulations.
Employing such practices helps identify problem areas efficiently, thereby improving overall code quality.
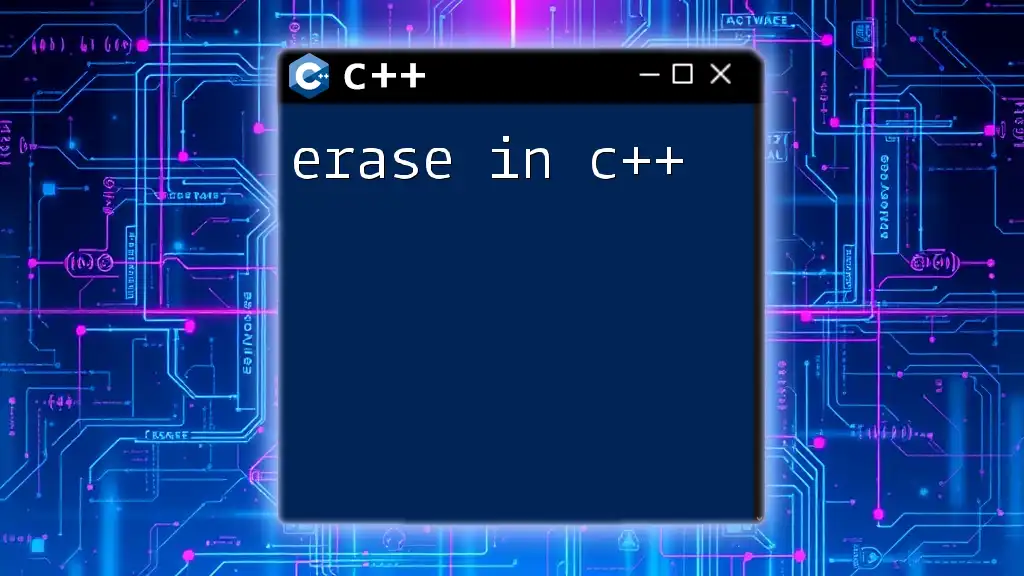
Conclusion
ASCII in C++ serves as an essential framework for handling character data effectively. By mastering ASCII concepts, programmers can enhance their coding skills in string manipulation, data transmission, and much more. Understanding this standard opens up myriad possibilities for creative and practical applications, making it crucial in the toolkit of a proficient C++ developer.
Further Reading and Resources
To deepen your understanding of ASCII in C++, consider exploring comprehensive books, online tutorials, and the official C++ documentation. These resources will provide valuable insights into advanced usage and techniques, solidifying your command over ASCII and its implications in programming.