The `pop_back` function in C++ is used to remove the last element from a `std::vector`, reducing its size by one.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.pop_back(); // Removes the last element (5)
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4
}
return 0;
}
What is a Vector in C++?
A vector in C++ is a dynamic array provided by the Standard Template Library (STL). It offers a way to store a sequence of elements, allowing for automatic memory management and dynamic resizing as elements are added or removed. Unlike traditional arrays that have a fixed size, a vector can grow or shrink based on your needs.
Key features of vectors
-
Dynamic sizing: Vectors can adjust their size automatically when elements are added or removed. You don't need to specify the size ahead of time, which provides flexibility when dealing with data.
-
Memory management: Vectors handle memory allocation and deallocation for you. When elements are added, vectors may reallocate their underlying storage to accommodate the new size, which enhances performance over manual memory management.

The pop_back Function in C++
The `pop_back` function is a crucial member function of the vector class in C++. This function is designed to remove the last element from a vector, effectively decreasing its size by one.
Syntax of pop_back
The syntax for using `pop_back` is straightforward:
vector_name.pop_back();
Here, `vector_name` refers to the specific vector instance you want to operate on. It’s important to remember that `pop_back` does not return any value; instead, it simply modifies the vector by removing the last element.
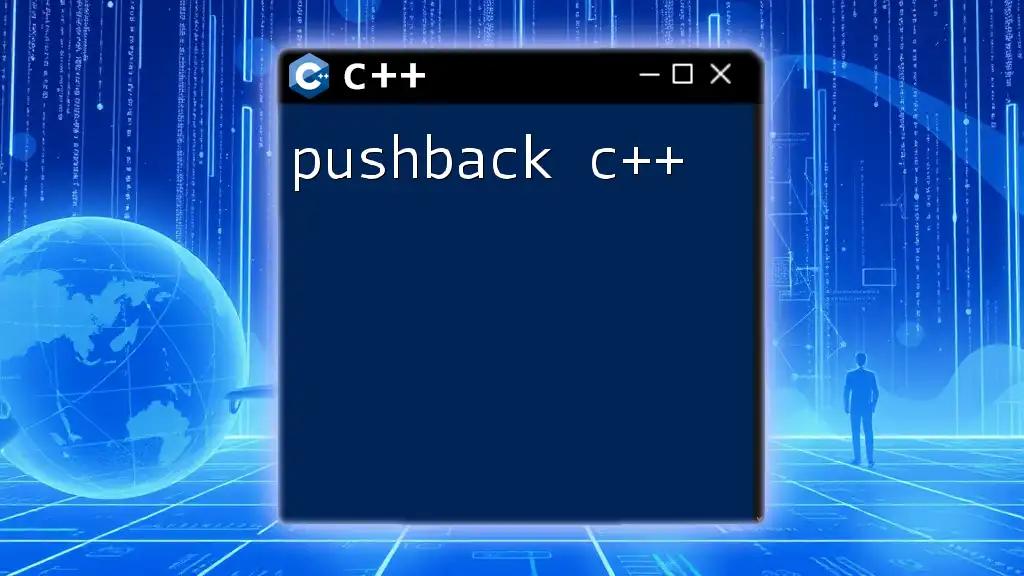
How to Use pop_back with Vectors
To understand how `pop_back` works in practice, let’s look at a basic implementation.
Here is an example that illustrates its usage:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.pop_back(); // Removes the last element
// Display remaining elements
for (const auto& num : numbers) {
std::cout << num << " "; // Outputs: 1 2 3 4
}
return 0;
}
In this example, the vector `numbers` initially contains five integers. The `pop_back` function is called, which removes the last element (5). The remaining elements are then printed, demonstrating that the vector now holds four integers: 1, 2, 3, and 4.
Understanding Memory Management
When using `pop_back`, it’s important to recognize that this function does not necessarily free up all the memory allocated for the vector. Instead, it simply removes the last element and reduces the logical size of the vector. The capacity may remain unchanged unless the vector is explicitly resized. This behavior enhances performance by minimizing memory reallocations.

Error Handling with pop_back
What happens if you use pop_back on an empty vector?
Utilizing `pop_back` on an empty vector leads to undefined behavior. This means that the program could crash or produce unexpected results, making it essential to guard against this scenario.
Best practices for using pop_back safely
To ensure the safe use of `pop_back`, always check if the vector is empty before calling the function. Here’s a sample code snippet that demonstrates this precaution:
if (!numbers.empty()) {
numbers.pop_back(); // Safe to call
}
This code ensures that `pop_back` is only executed if there is at least one element in the vector, safeguarding against potential runtime errors.
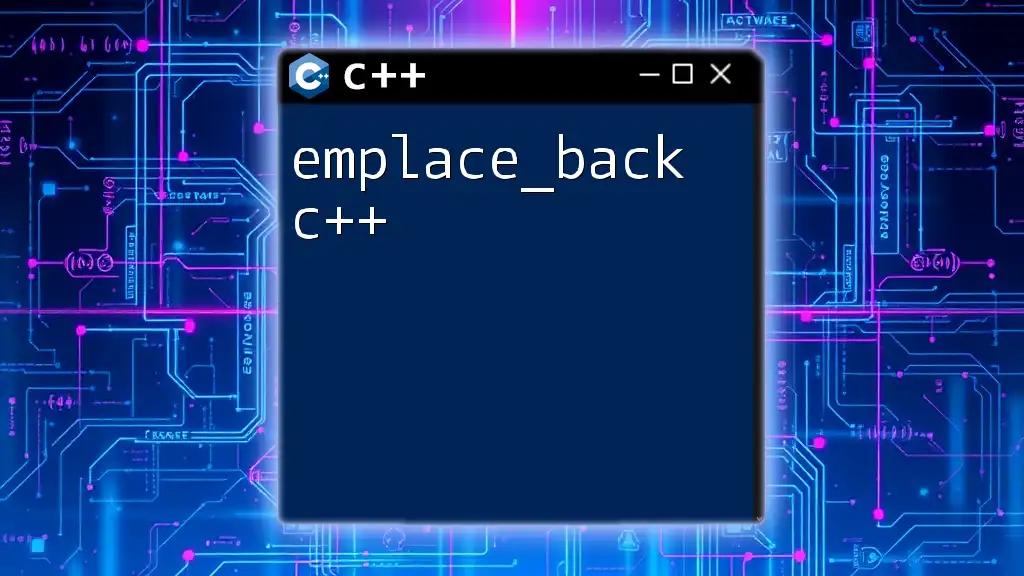
Common Use Cases of pop_back in C++
The versatility of `pop_back` allows it to be used across various programming scenarios:
Practical examples
-
Stack implementation: The pop_back function can be used to simulate stack behavior. By treating a vector as a stack, pop_back can remove the last pushed element, reflecting the last-in-first-out (LIFO) principle.
-
Managing a to-do list: If you are maintaining a list of tasks, using `pop_back` allows you to easily mark the most recent task as completed and remove it from the list.
Alternative methods for element removal
While `pop_back` is a straightforward way to remove the last element, there are other methods available for element removal in C++:
-
erase: This method can be used to remove specific elements from any position in the vector. It provides more versatility but is less efficient than pop_back when removing the last element.
-
clear: The clear function removes all elements from the vector, effectively resetting its size to zero. It is useful when you want to start fresh with your vector.
Comparing `pop_back` against these alternatives can help you choose the best method for your specific use case. In general, if you only need to remove the last item, `pop_back` is quick and efficient.
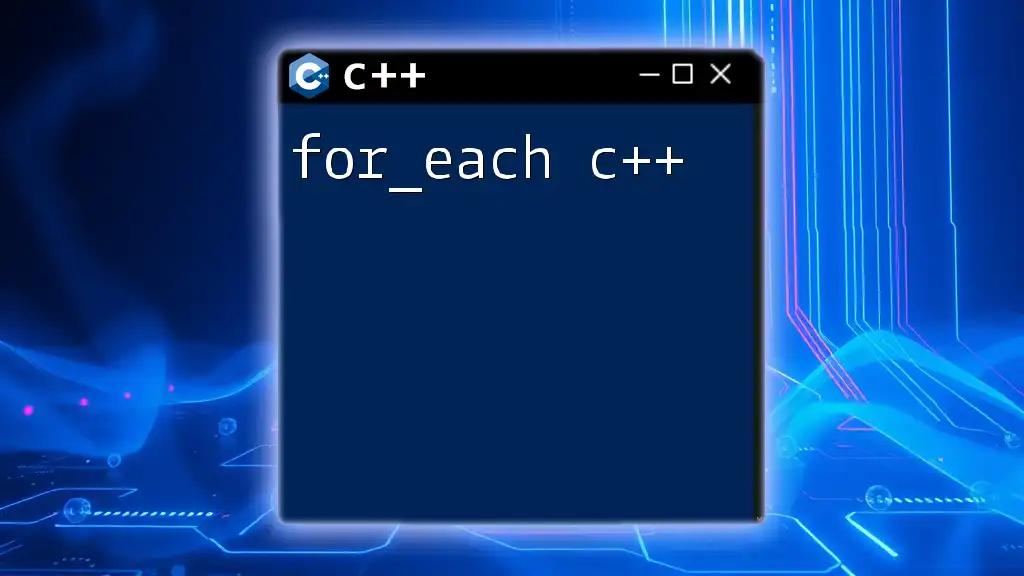
Conclusion
In summary, understanding how to use `pop_back` in C++ is essential for effective vector manipulation. This function provides a simple yet powerful means of managing collections of data dynamically. By leveraging the functionalities of vectors through `pop_back`, you can handle various application requirements efficiently.
Encouragement for practice
To solidify your understanding, it's recommended to practice using `pop_back` in various contexts, such as creating stacks, managing lists, or experimenting with other STL algorithms. The more you engage with these concepts, the more proficient you'll become in leveraging C++ capabilities for real-world applications.

Additional Resources
For those eager to learn more, consider exploring additional materials on C++ vectors and the STL. Books, online courses, and tutorials are excellent resources for further deepening your knowledge in this area.
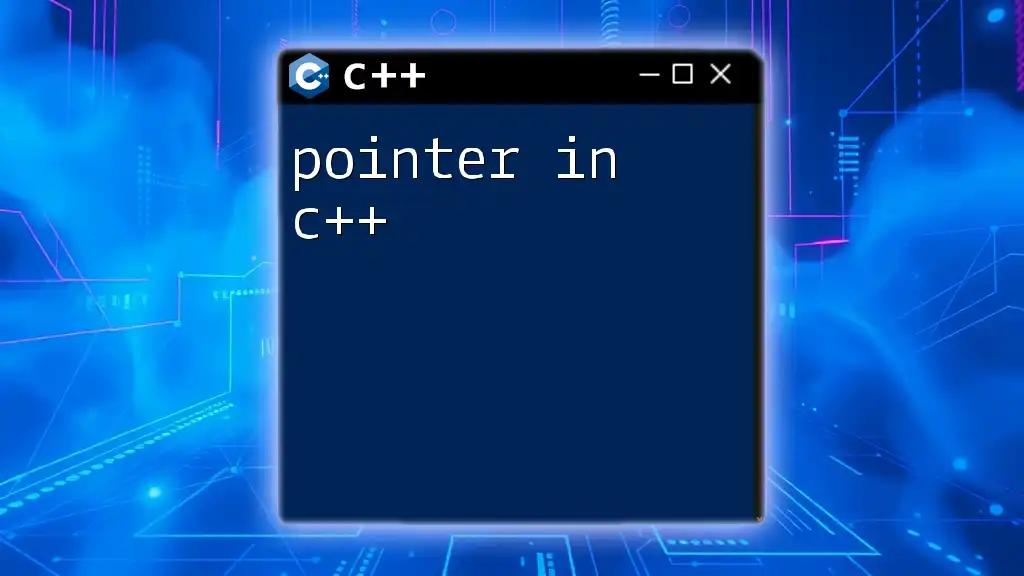
Call to Action
Join our learning community today to enhance your understanding of C++ functions like `pop_back` and many more. Stay updated and master the art of efficient programming in C++.