In this post, we will explore a simple C++ implementation of the game Blackjack, where players aim to get as close to 21 as possible without exceeding it.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(time(0)); // Seed for random number generation
int playerTotal = rand() % 11 + 10; // Randomly set player's total to be between 10 and 21
int dealerTotal = rand() % 11 + 10; // Randomly set dealer's total to be between 10 and 21
std::cout << "Player's Total: " << playerTotal << "\nDealer's Total: " << dealerTotal << std::endl;
if (playerTotal > 21) std::cout << "Player busts! Dealer wins." << std::endl;
else if (dealerTotal > 21 || playerTotal > dealerTotal) std::cout << "Player wins!" << std::endl;
else if (playerTotal < dealerTotal) std::cout << "Dealer wins!" << std::endl;
else std::cout << "It's a push!" << std::endl;
return 0;
}
Overview of the Game
Blackjack, also known as 21, is one of the most popular card games in casinos around the world. Its objective is straightforward: players aim to beat the dealer by having a hand value greater than the dealer's hand, without exceeding 21. The game provides an engaging mix of strategy, chance, and psychology, making it both exciting and challenging.
The Basic Rules and Gameplay Mechanics
In Blackjack, each player is dealt two cards, while the dealer has one card face up (the "upcard") and one card face down (the "hole card"). Players can "hit" (take another card) or "stand" (keep their current hand). If a player's total exceeds 21, they "bust" and automatically lose. The game continues as the dealer reveals their hole card, and must follow strict rules (usually hitting until they reach at least 17).
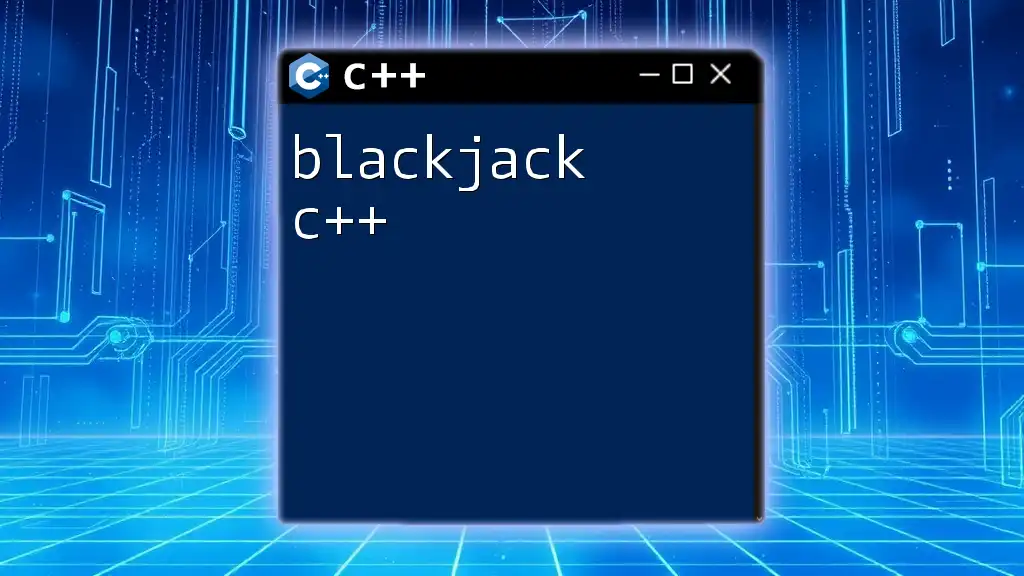
Importance of Learning via C++
Learning to program is akin to mastering a new language; it opens doors to creativity and logical thinking. By using C++ to create a simple yet robust game like Blackjack, you gain not just programming skills but also an understanding of object-oriented programming, which is fundamental in the development of complex software and games.
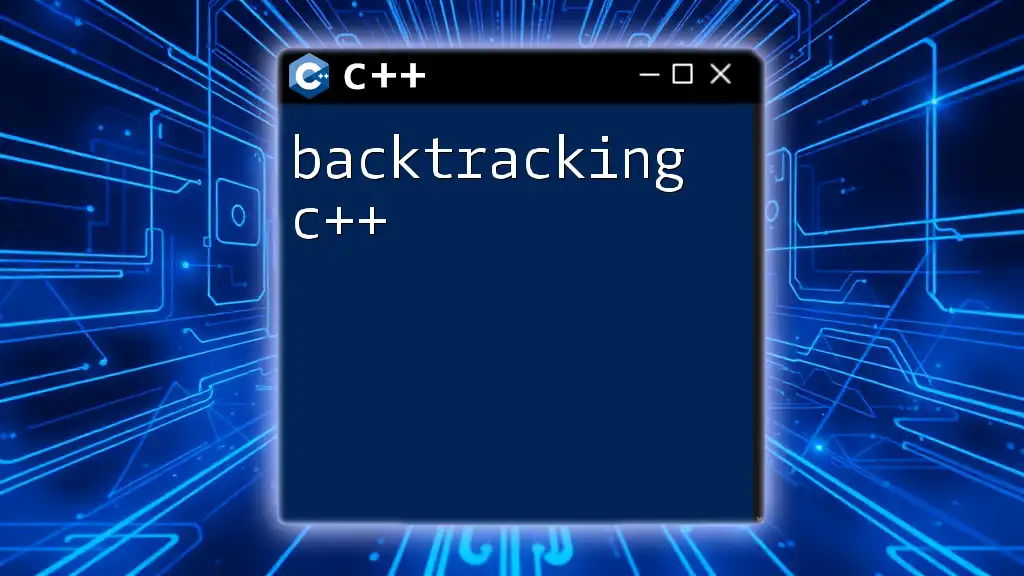
Setting Up Your C++ Environment
Required Tools and Software
Before diving into programming, you need the right tools:
- An Integrated Development Environment (IDE): Options like Code::Blocks, Visual Studio, or CLion can help you write, debug, and run your C++ code more efficiently.
- C++ Compiler: Ensure you have a compatible C++ compiler installed, such as GCC or MSVC.
Creating Your First C++ Program
Starting with a simple program can help you get familiar with the language. Create a new project in your chosen IDE and write the classic “Hello World” program. Here’s how the code looks:
#include <iostream>
int main() {
std::cout << "Hello World!" << std::endl;
return 0;
}
Running this snippet will familiarize you with the basic structure of C++ programs and how to compile and execute them.
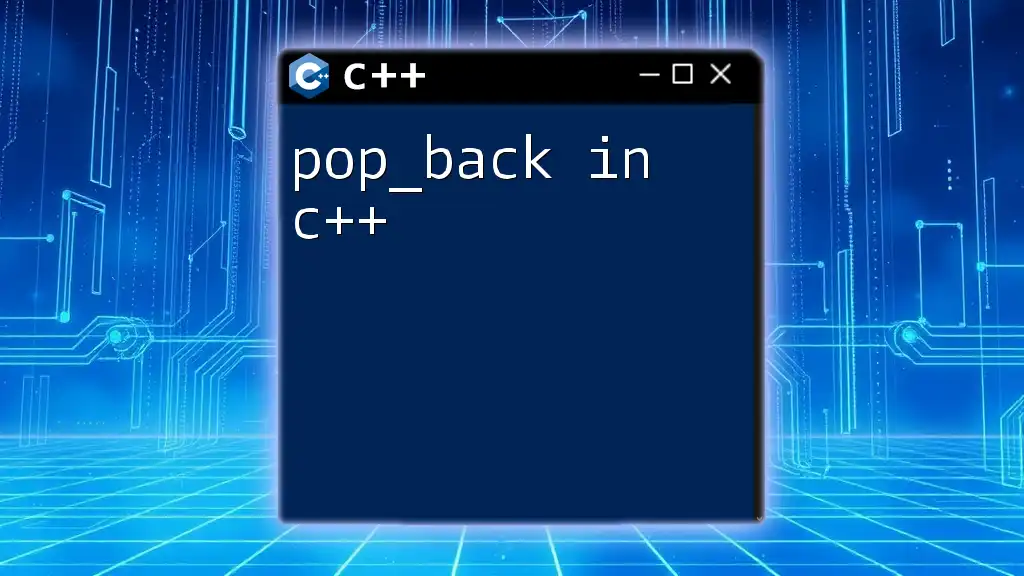
Implementing Blackjack in C++
Basic Structure of the Program
Before coding, it’s essential to plan the structure of your Blackjack game. The key components will include a player, the dealer, and a deck of cards. Each entity will be represented by a class in C++.
Here’s a simple scaffolding for your implementation:
#include <iostream>
// Classes for Card, Deck, Player, and Game will go here
int main() {
// Placeholder for the Blackjack game implementation
return 0;
}
Designing the Card Class
A vital part of the game is the Card class which models the cards in a deck. Each card has a suit (Hearts, Diamonds, Clubs, Spades) and a value (ranging from 2 to Ace).
Code Snippet: Card Class Example
class Card {
public:
enum Suit { Hearts, Diamonds, Clubs, Spades };
enum Value { Two = 2, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack = 10, Queen = 10, King = 10, Ace = 11 };
Card(Suit s, Value v) : suit(s), value(v) {}
private:
Suit suit;
Value value;
};
This class consists of an enumerated type for suits and values and a constructor for card initialization.
Creating a Deck Class
The Deck class manages all the cards, allowing you to shuffle and deal them. This class is critical for gameplay, as it ensures that cards are drawn from a standard 52-card deck.
Code Snippet: Deck Class Example
#include <vector>
#include <algorithm>
#include <random>
class Deck {
public:
Deck() {
// Initialize the deck with cards
for (int s = Card::Hearts; s <= Card::Spades; ++s) {
for (int v = Card::Two; v <= Card::Ace; ++v) {
cards.push_back(Card(static_cast<Card::Suit>(s), static_cast<Card::Value>(v)));
}
}
}
void shuffle() {
std::shuffle(cards.begin(), cards.end(), std::default_random_engine());
}
Card deal() {
Card dealtCard = cards.back();
cards.pop_back();
return dealtCard;
}
private:
std::vector<Card> cards;
};
This implementation initializes the deck with all card combinations, includes a shuffle function using the C++ standard library, and implements a method to deal cards.
Managing Player and Dealer
Class Design for Player
The Player class is responsible for handling the current player's state, including their hand and balance.
class Player {
public:
void hit(Card card) {
hand.push_back(card);
}
int calculateHandValue() {
int totalValue = 0;
int acesCount = 0;
for (const Card& card : hand) {
totalValue += static_cast<int>(card.getValue());
if (card.getValue() == Card::Ace) {
acesCount++;
}
}
while (totalValue > 21 && acesCount > 0) {
totalValue -= 10; // Treat Ace as 1 instead of 11
acesCount--;
}
return totalValue;
}
private:
std::vector<Card> hand;
int balance;
};
This class allows players to hit a card and calculate their hand's value, adjusting for Aces as needed.
Implementing Game Logic
Now that we have our classes, we need to implement the rules of Blackjack.
Starting the Game
The game kicks off by initializing the players and the deck. Both the player and dealer will be dealt two cards each, and the user is prompted for their betting amount.
Player Actions
The player can choose to hit or stand. If the player hits and exceeds 21, they bust and lose immediately.
Dealer Gameplay
The dealer must adhere to the rules of drawing cards, often requiring them to hit until they reach at least 17.
Determining the Winner
At the conclusion of the game, the hands are compared to determine the winner. If the player has a greater hand value than the dealer without busting, they win. Payouts can be calculated accordingly.
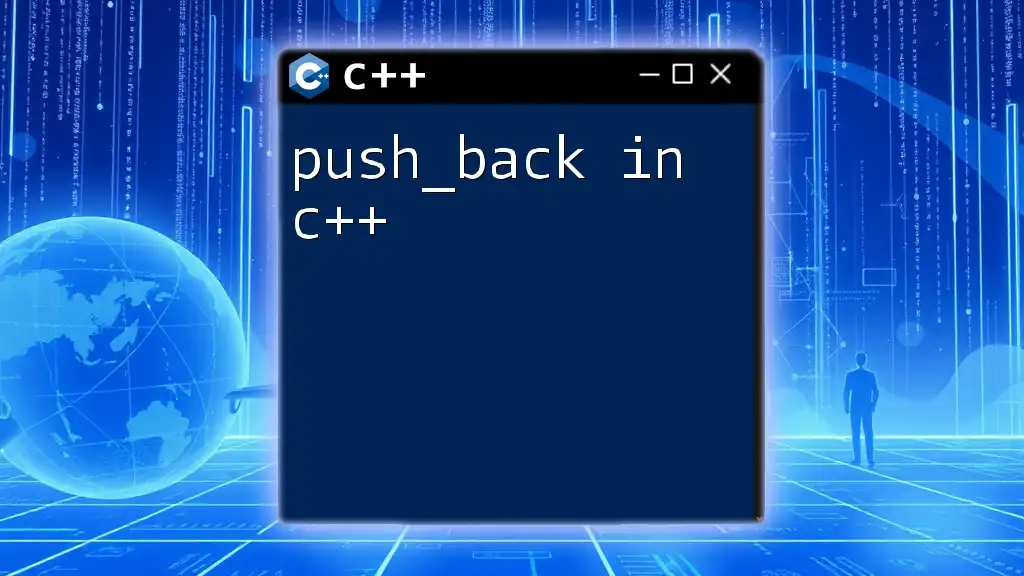
Example C++ Blackjack Game
Putting It All Together
As you bring all components together, the main function would look something like this:
int main() {
Deck deck;
Player player;
Player dealer;
// Initialize game logic and flow
deck.shuffle();
// Deal initial cards
player.hit(deck.deal());
player.hit(deck.deal());
dealer.hit(deck.deal());
dealer.hit(deck.deal());
// Implement player's decision-making
// Implement dealer's gameplay logic
// Determine the winner
}
In this central part of your game, you would implement the full logic of player actions and dealer compliance, leading to a complete gameplay experience.
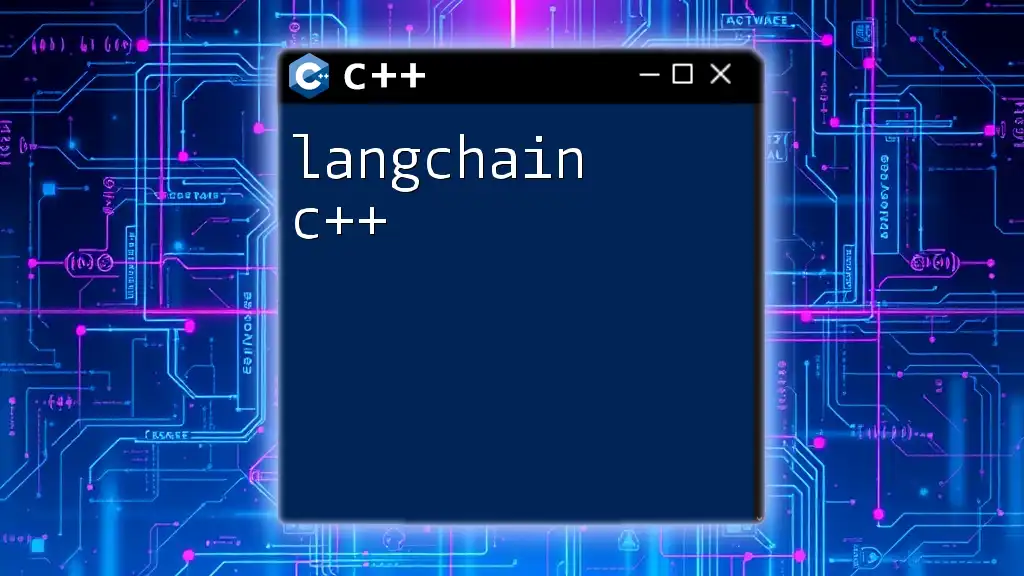
Running and Testing Your Program
How to Compile and Run Your C++ Code
You can compile and run your code via your IDE's interface, or by using command-line tools. Here’s a command you might use:
g++ -o blackjack blackjack.cpp
./blackjack
Testing for Bugs and Errors
Testing is crucial. Start by playing through various scenarios to identify possible bugs. Debugging techniques, such as printing out hand values at each step, can help locate problems in your logic.
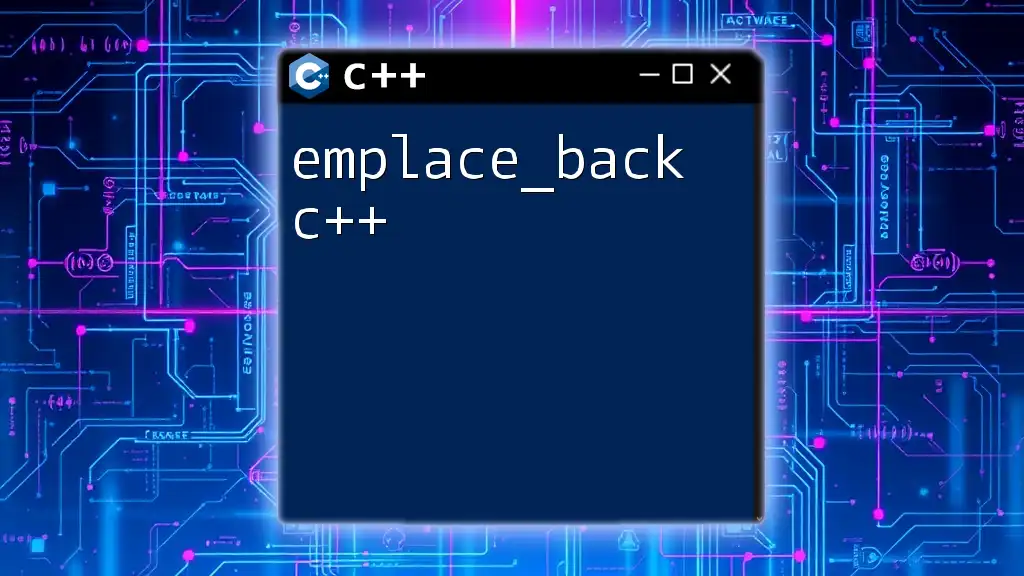
Conclusion
In summation, developing a simple Blackjack game in C++ not only showcases your growing programming skills but also illustrates the power of object-oriented programming principles. This exercise solidifies your understanding of key C++ concepts such as classes, methods, and dynamic memory management.
Encouragement to Explore Further
Once you’re comfortable with Blackjack, consider expanding your skills by exploring other card games or even enhancing your Blackjack game with more features such as betting systems or multiplayer functionality. Continuous learning is key in mastering C++.
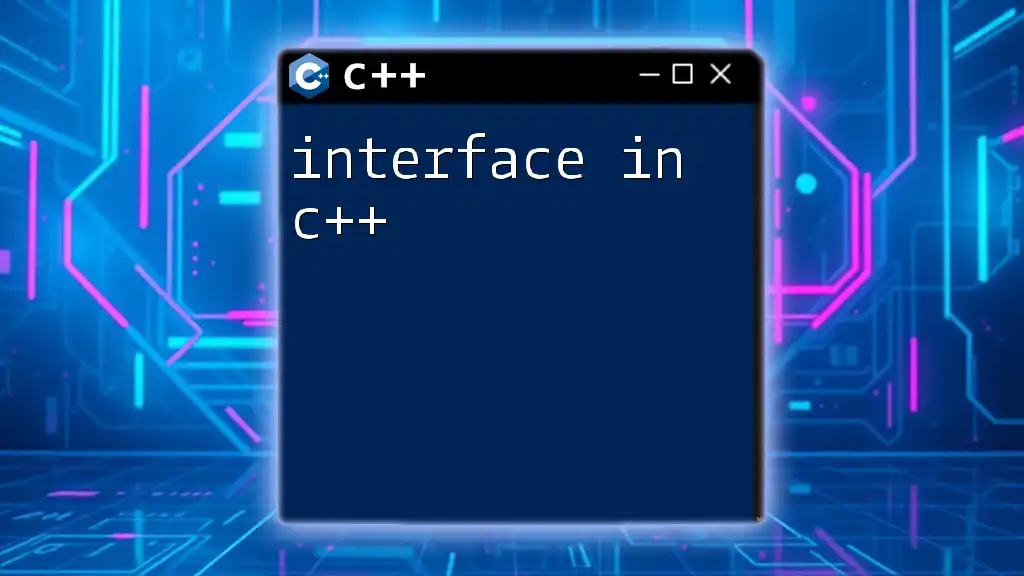
Additional Resources
Recommended Books and Online Tutorials
Seek out comprehensive books on C++ programming, as well as online resources that can deepen your understanding of both the language and the concepts of game design and development.
FAQs about Blackjack in C++
Feel free to reach out to coding communities for assistance with questions or issues you encounter as you develop your game in C++. You are not alone, and many programmers are eager to share their knowledge and experiences!