The `getchar` function in C++ reads a single character from the standard input, which is useful for capturing user input directly from the keyboard.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
int main() {
char ch;
std::cout << "Enter a character: ";
ch = getchar(); // Reads a single character
std::cout << "You entered: " << ch << std::endl;
return 0;
}
Understanding getchar in C++
What is getchar?
The function `getchar` is a standard function in C and C++ that reads the next character from the standard input (stdin). Its primary purpose is to provide a method for capturing single-character input from users efficiently.
Importance of getchar in C++
Developers often choose `getchar` for its simplicity when handling single-character input. It's particularly useful in scenarios where you want to directly interact with user input without much overhead. Common use cases include:
- Basic console applications that require character input.
- Creating simple text-based games that require instant feedback on single keystrokes.
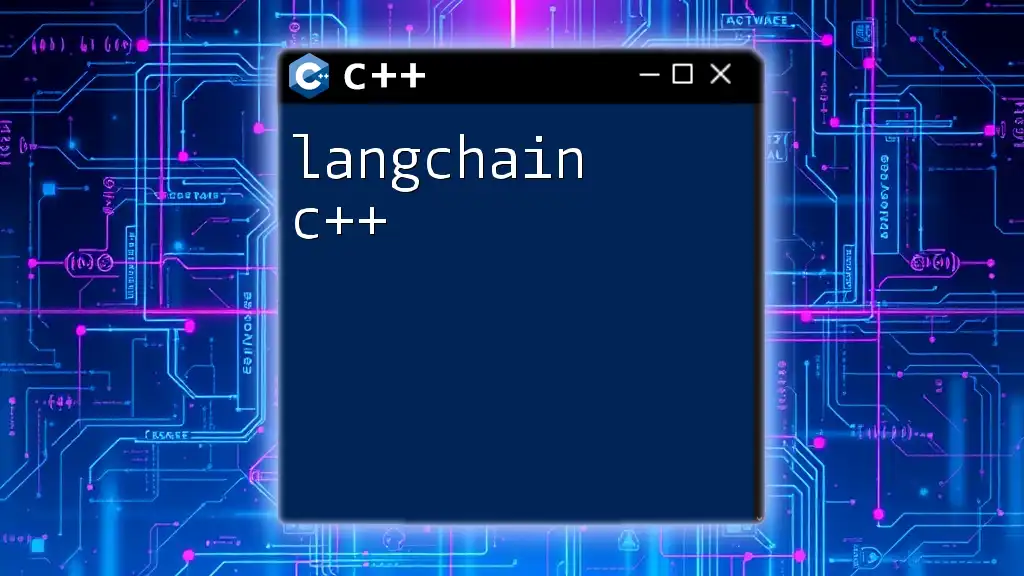
Syntax and Basic Usage
Syntax of getchar
The syntax of `getchar` is straightforward:
int getchar(void);
It does not take any parameters and returns the next character as an integer value. This allows it to also handle the EOF (End Of File) condition, which is represented by `-1`.
Simple Example
Here’s a simple example demonstrating the basic usage of `getchar`:
#include <iostream>
int main() {
char c;
std::cout << "Enter a character: ";
c = getchar();
std::cout << "You entered: " << c << std::endl;
return 0;
}
In this example, the program prompts the user for input and reads a single character. The `getchar` captures this character and immediately displays it back to the user. This flow illustrates the fundamental way `getchar` interacts with user input.
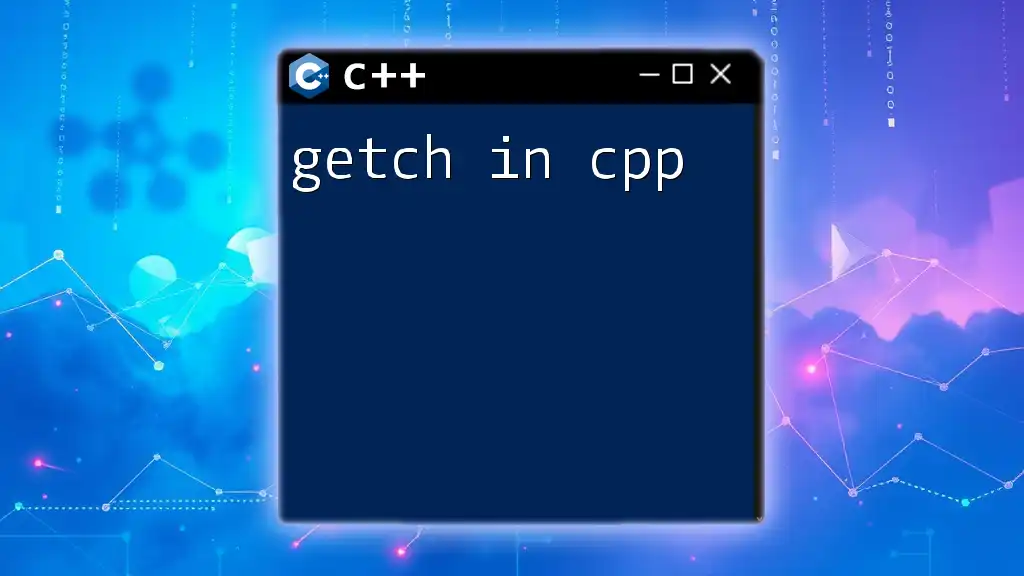
Working of getchar in C++
How getchar Works
The operation of `getchar` revolves around the concept of buffering. When a user types a character and presses Enter, the input is stored in a buffer. `getchar` retrieves this character directly from the buffer. This behavior means that `getchar` will only read characters until the newline character is encountered.
Handling Input
`getchar` reads characters one by one, including whitespaces. This property can be both useful and troublesome depending on your needs. For instance, if you are expecting only non-whitespace characters, you will need to implement additional logic to handle unwanted inputs.
Example: Continuous Input
The following code demonstrates how to read multiple characters until an EOF signal is sent:
#include <iostream>
int main() {
char c;
std::cout << "Enter characters (press Ctrl+D to stop): " << std::endl;
while ((c = getchar()) != EOF) {
std::cout << "You entered: " << c << std::endl;
}
return 0;
}
In this example, the program continuously reads characters, providing a prompt for the user. The loop terminates on EOF, allowing for a fluid user experience when inputting multiple characters.
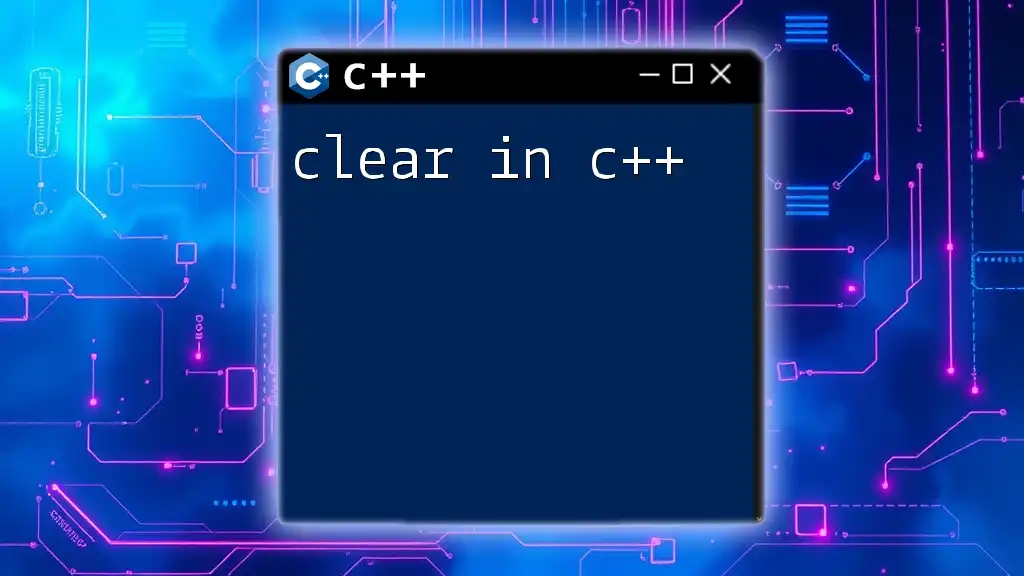
Comparing getchar with Other Functions
getchar vs. cin
While both `getchar` and `cin` are used for input, they differ significantly:
- Data Types: `cin` can handle various data types (like string, int, float), whereas `getchar` strictly deals with characters.
- Buffering: `cin` employs formatted input, meaning it waits for the newline before processing, while `getchar` reads characters one at a time and processes them immediately.
getchar vs. getch
The `getch` function, primarily found in `<conio.h>` on DOS systems, reads characters without waiting for the Enter key. This difference makes `getch` suitable for interactive applications that require immediate feedback from the keyboard. However, `getch` is not part of the standard C++ library, making it less portable than `getchar`.
Pros and Cons of getchar
Pros:
- Easy to use for single-character input.
- Outputs characters immediately upon input.
Cons:
- Lack of error handling, making it less robust for advanced scenarios.
- Possible confusion when mixing with other input functions requiring different input paradigms.
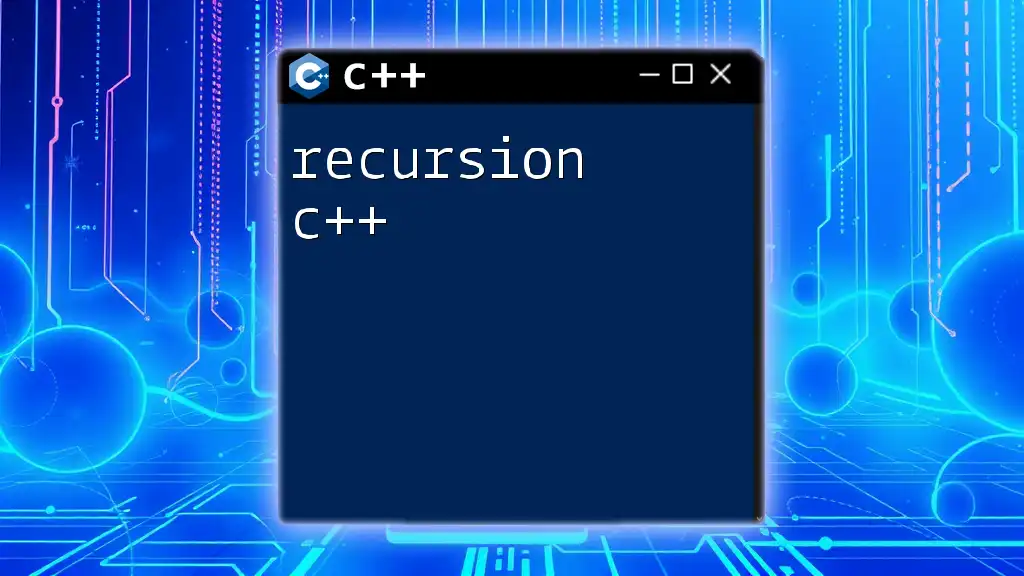
Best Practices for Using getchar in C++
Avoiding Common Pitfalls
When using `getchar`, it’s essential to be aware of common pitfalls like buffer issues. If you mix `getchar` with other input functions like `cin`, it may lead to unexpected behavior due to lingering newline characters in the input buffer. Always consider clearing the input buffer before or after using `getchar`.
Tips for Efficient Usage
Choosing `getchar` should be guided by the need for simple character reading. For instances where performance is crucial and only single character input is required, `getchar` offers an efficient option.
Example: Combining getchar with cin
You might sometimes want to combine `getchar` with `cin`, which is a common scenario. Here’s how this could look:
#include <iostream>
int main() {
std::string str;
char c;
std::cout << "Enter a string: ";
std::getline(std::cin, str);
std::cout << "Now press any key: ";
c = getchar();
std::cout << "You typed: " << str << " and pressed: " << c << std::endl;
return 0;
}
In this case, we read a full line of text into a string and then use `getchar` to capture the subsequent character input. However, be cautious of the lingering newline character that can affect how `getchar` behaves.
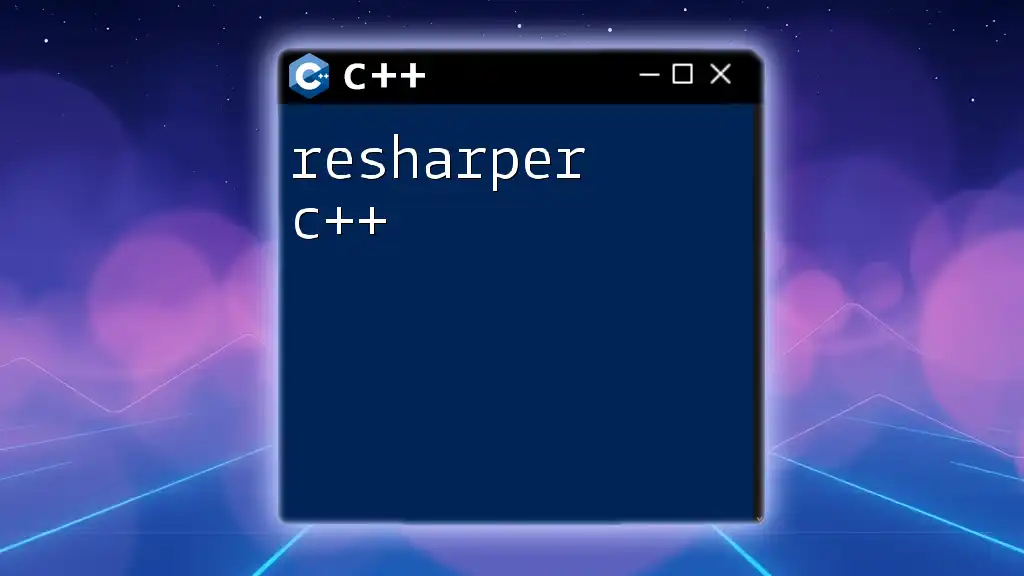
Conclusion
Final Thoughts on getchar in C++
Understanding `getchar in C++` is a stepping stone for anyone looking to manage user input effectively in console applications. By grasping the nuances of its usage, strengths, and weaknesses, you can utilize it to great effect when building your applications.
Further Reading and Resources
To enhance your knowledge further, consider diving into additional resources on C++ I/O management. Online tutorials, documentation, and community forums can provide invaluable insights and examples.
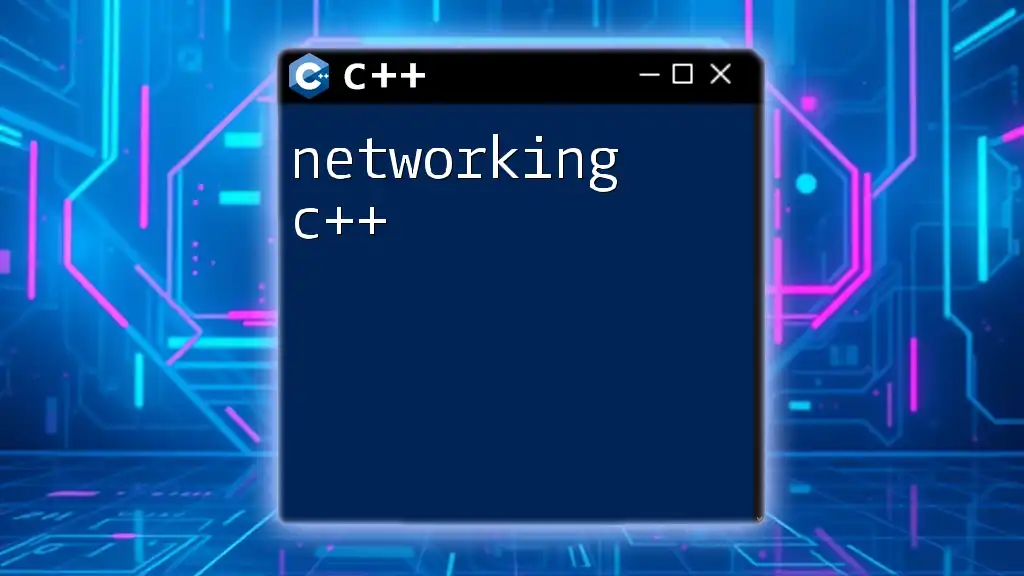
Call to Action
Join the conversation! If you've had experiences using `getchar` in your C++ projects or unique use cases, share them in the comments below. If you found this guide helpful, don't hesitate to share it with fellow programmers looking to deepen their understanding of C++ input handling.