Methods in C++ are functions that are associated with a class and can operate on the class's member variables or perform specific actions related to that class.
Here's a simple example of a method in a C++ class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
Understanding C++ Methods
What is a Method in C++?
A method in C++ can be defined as a function that is associated with a class. Unlike regular functions, methods operate primarily within the context of an object. This means that methods can manipulate the data of the objects they belong to, making them essential for achieving the principles of encapsulation and abstraction in Object-Oriented Programming (OOP).
It's also important to distinguish methods from regular functions. While functions can exist independently, methods are invoked on objects of a class, leveraging the state and behavior encapsulated within these objects.
Example:
#include <iostream>
using namespace std;
void showMessage() {
cout << "This is a regular function." << endl;
}
class Greeting {
public:
void greet() {
cout << "Hello from a method!" << endl;
}
};
In this example, `showMessage()` is a standard function, while `greet()` is a method belonging to the `Greeting` class.
Syntax of a C++ Method
The syntax for defining a method consists of several key components. Understanding this structure is crucial for writing effective C++ methods:
- Return Type: Specifies the type of value the method will return. If no value is returned, use `void`.
- Method Name: A descriptive name that indicates what the method does.
- Parameters: Variables that are passed to the method. They are optional.
- Method Body: Contains the code that defines what the method does.
Code Snippet: Typical Method Syntax
class MyClass {
public:
int add(int a, int b) { // Return type: int
return a + b; // Method body
}
};
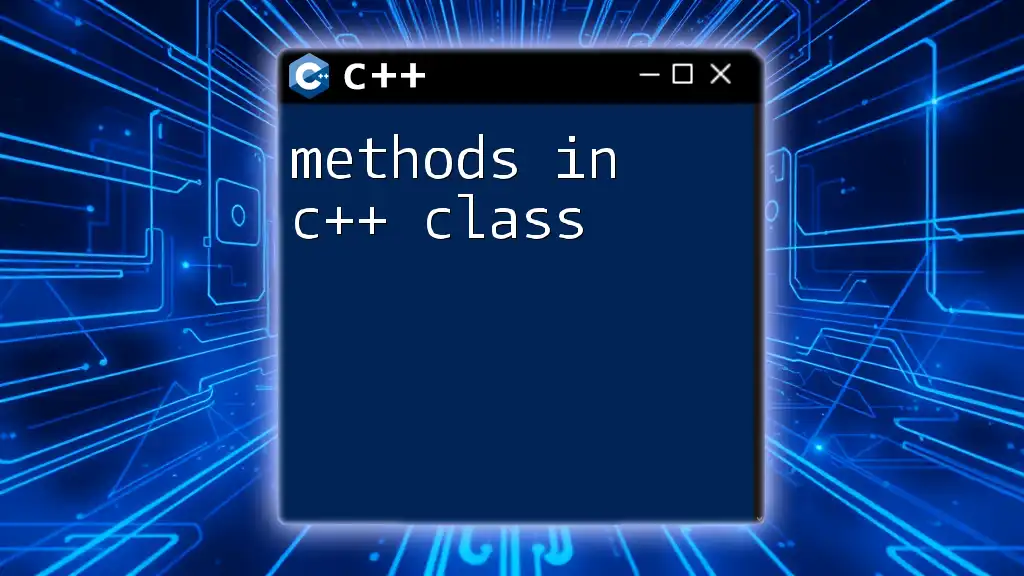
Types of Methods in C++
Instance Methods
Instance methods are perhaps the most common type of methods. They relate to a specific instance of a class and can access and modify instance variables. This allows each object created from a class to maintain its own state.
Code Snippet: Example of an Instance Method
class Counter {
public:
int count;
Counter() : count(0) {} // Constructor
void increment() {
count++; // Modifying instance variable
}
int getCount() {
return count; // Accessing instance variable
}
};
In this snippet, `increment()` and `getCount()` are instance methods that modify and read the state of `count`, respectively.
Static Methods
Static methods belong to the class rather than a particular object instance. This means you do not need to create an object to call a static method. Static methods are typically used for utility operations that don’t depend on instance variables.
Code Snippet: Example of a Static Method
class MathUtils {
public:
static int square(int x) { // Static method
return x * x;
}
};
// Usage
int result = MathUtils::square(5);
In this case, `square(int x)` is a static method, allowing it to be invoked using the class name itself.
Const Methods
Const methods are those which do not modify the state of the object. They are declared with the `const` keyword at the end of the method declaration. Using `const` is useful for ensuring that a method does not inadvertently modify class attributes.
Code Snippet: Creating a Const Method
class Sample {
public:
int value;
Sample(int v) : value(v) {}
int getValue() const { // Const method
return value;
}
};
In this example, `getValue()` is a const method that guarantees it will not alter the state of the `Sample` class instance.
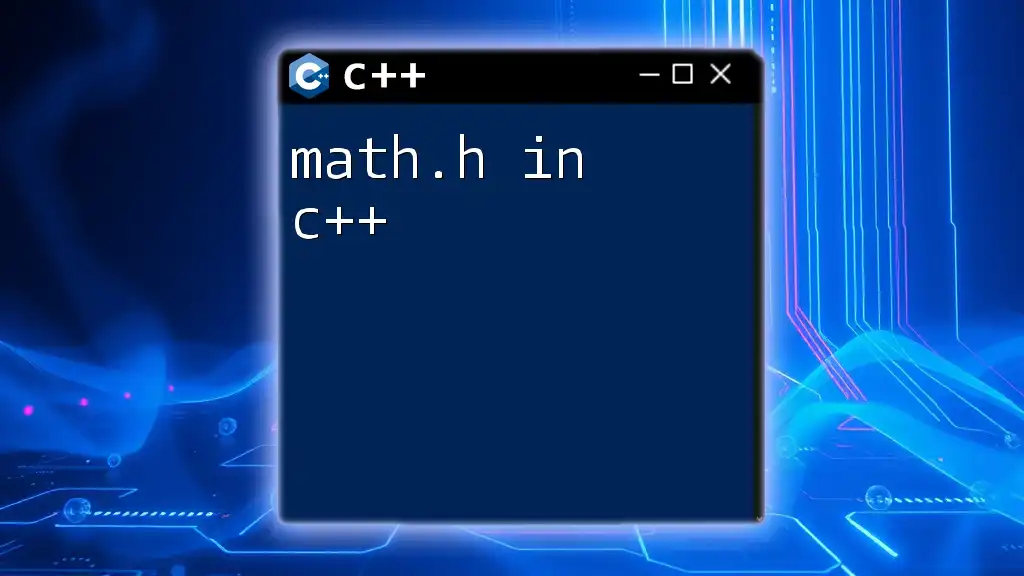
Method Overloading in C++
What is Method Overloading?
Method overloading allows multiple methods with the same name but different parameter types or counts within the same class. This feature enhances readability by enabling consistency in method names while allowing different ways to call the same functionality.
Example: Overloaded Methods with Different Parameters
class Display {
public:
void show(int i) {
cout << "Integer: " << i << endl;
}
void show(double d) {
cout << "Double: " << d << endl;
}
void show(string s) {
cout << "String: " << s << endl;
}
};
In this code, the `show` method is overloaded to handle different types of input.
Rules for Method Overloading
Method overloading must adhere to certain rules. These include:
- Methods must have the same name.
- They must differ in the type or number of parameters.
- Return type alone is not sufficient to differentiate overloaded methods.
Code Snippet: Valid vs. Invalid Overload Examples
class Example {
public:
void func(int x);
void func(double x); // valid
void func(int x, double y);
// void func(double x, int y); // invalid due to identical signatures with differing return types
};
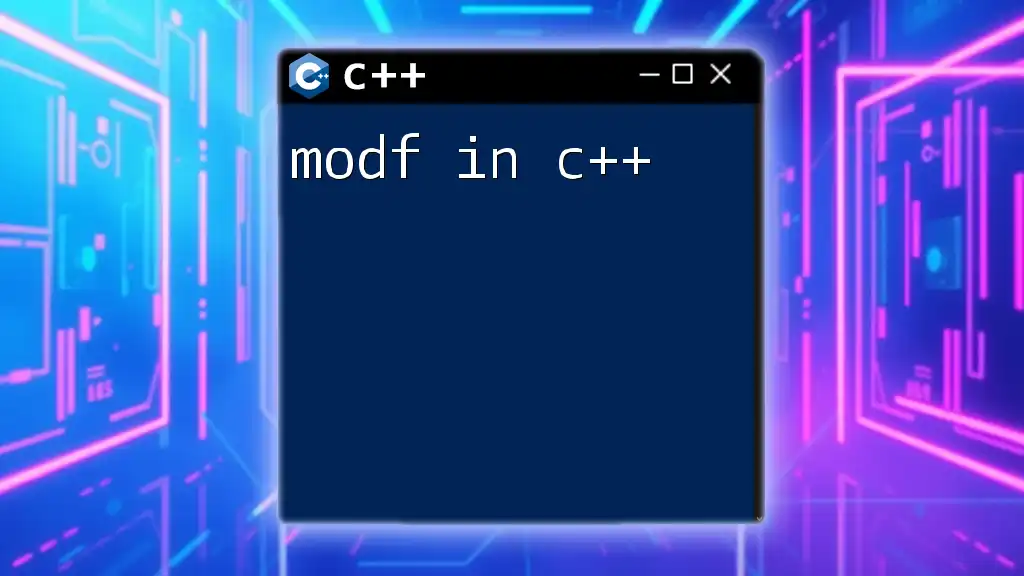
Method Overriding in C++
What is Method Overriding?
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. Overriding supports runtime polymorphism and allows for dynamic method dispatch.
Code Snippet: Basic Example of Method Overriding
class Base {
public:
virtual void show() { // Virtual method
cout << "Base class show method." << endl;
}
};
class Derived : public Base {
public:
void show() override { // Overriding the base method
cout << "Derived class show method." << endl;
}
};
In this example, the `show()` method in `Derived` overrides the `show()` method in `Base`.
Virtual Methods
Using virtual methods ensures that the correct method is called for an object, regardless of the type of reference (or pointer) used for the function call. This is crucial for polymorphic behavior enabling dynamic binding, allowing programs to select the right method implementation at runtime.
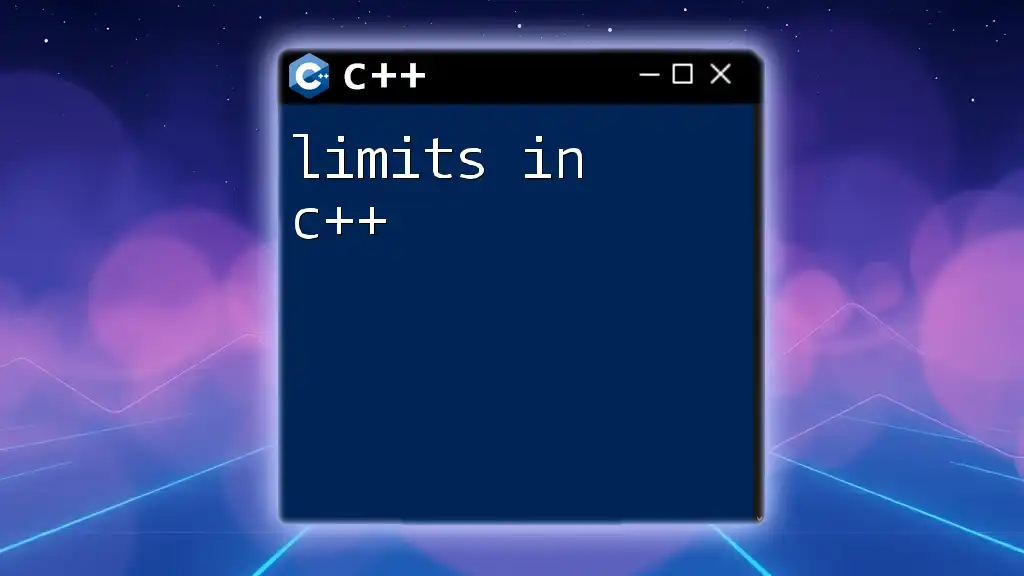
Access Modifiers for C++ Methods
Public, Private, and Protected Methods
Access modifiers dictate how methods can be accessed.
- Public methods can be called from anywhere in the codebase.
- Private methods can only be accessed by other methods within the same class.
- Protected methods can be accessed by derived classes.
For example:
class AccessDemo {
public:
void publicMethod() {
cout << "Public method." << endl;
}
private:
void privateMethod() {
cout << "Private method." << endl;
}
protected:
void protectedMethod() {
cout << "Protected method." << endl;
}
};
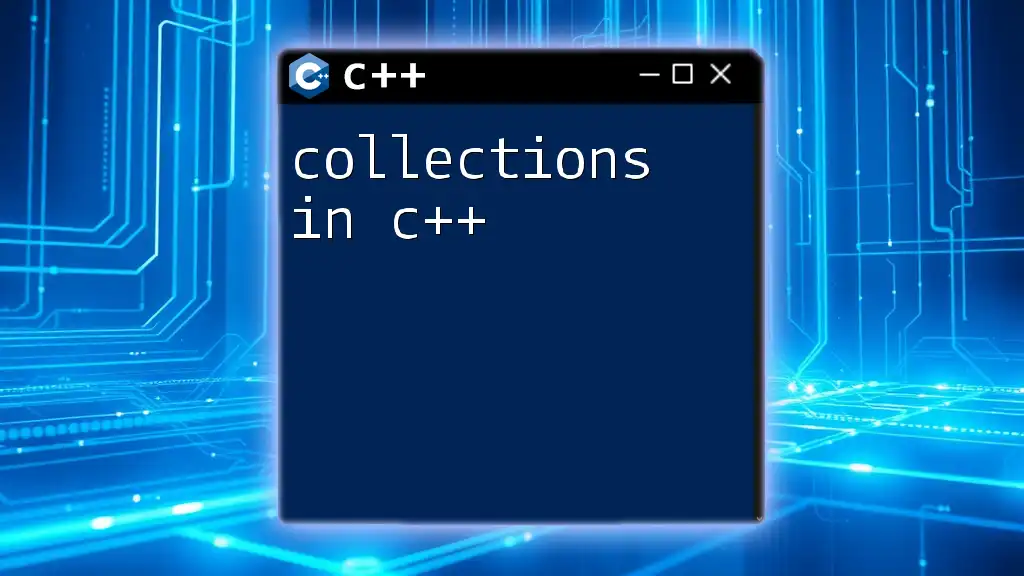
Using Methods Effectively in C++
Best Practices for Writing C++ Methods
To maximize the efficiency and readability of your methods, consider the following best practices:
- Keep methods short and focused: A method should accomplish one task. Longer methods can lead to confusion and difficulty in maintenance.
- Use descriptive names: This enhances readability and provides clarity regarding the method's functionality.
- Document your code: Always include comments explaining what the method does and any significant parameters.
Performance Considerations
The design of methods can significantly impact performance.
- Inline Methods: For small methods that are called frequently, consider using `inline` to suggest the compiler to replace the method call with the method code. This can reduce overhead from function calls.
Example: Inline Method Usage
class InlineExample {
public:
inline int multiply(int a, int b) {
return a * b; // Inline method
}
};
However, be mindful not to overuse inlines, as this can potentially increase the size of the binary.
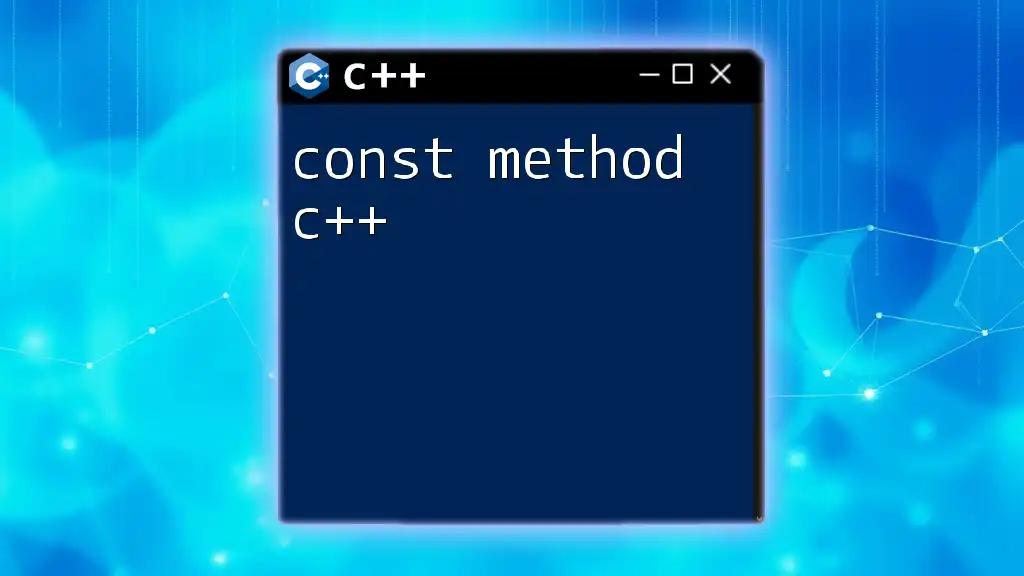
Conclusion
Methods in C++ are pivotal for encapsulating behavior and data management in object-oriented programming. By mastering the various types and usage of methods—such as instance methods, static methods, and const methods—you will gain a deeper understanding of how to create better-structured and maintainable applications.
Practice creating C++ classes with diverse methods for better comprehension of this essential feature!