A `const` method in C++ is a member function that guarantees not to modify the object it belongs to, ensuring safer code by preventing unintended changes to class members.
class Example {
public:
int getValue() const { // const method
return value;
}
private:
int value = 42;
};
What is a Const Method in C++?
A const method in C++ is a member function that guarantees not to modify any member variables of the class. This means that when a member function is declared as `const`, it can be called on both const and non-const instances of the class. However, non-const member functions can only be called on non-const instances.
The significance of const methods is profound, especially in large codebases where unintended modifications can lead to bugs that are difficult to track down. Utilizing const methods enhances code safety and makes your intentions clearer to anyone who may read or maintain your code.
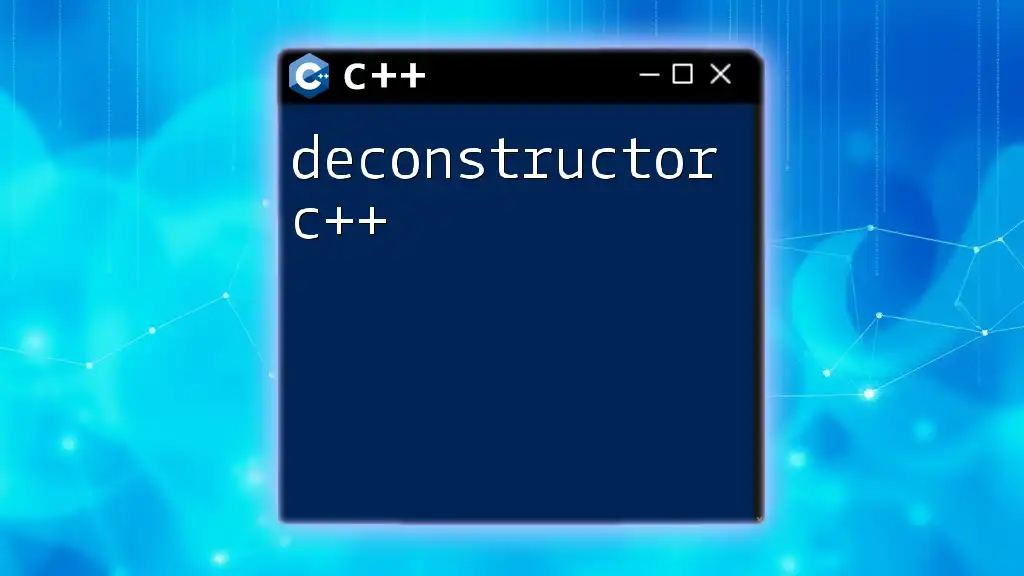
Syntax of Const Member Functions
General Syntax Structure
Declaring a const method in C++ is straightforward. The syntax requires you to append the `const` keyword to the method's declaration. Here's a basic example:
class ClassName {
public:
void methodName() const; // Const member function
};
In this structure:
- `ClassName` refers to the class in which the method exists.
- `methodName()` is the function that will perform some action without modifying the object’s state.
- The `const` keyword at the end indicates that this function will not modify any data members.
Declaring a Const Method
To declare a const member function, simply add the `const` keyword at the end of the function declaration in the class. For instance:
class Example {
public:
void display() const;
};
This declaration specifies that the `display` function does not modify the instance of `Example`.
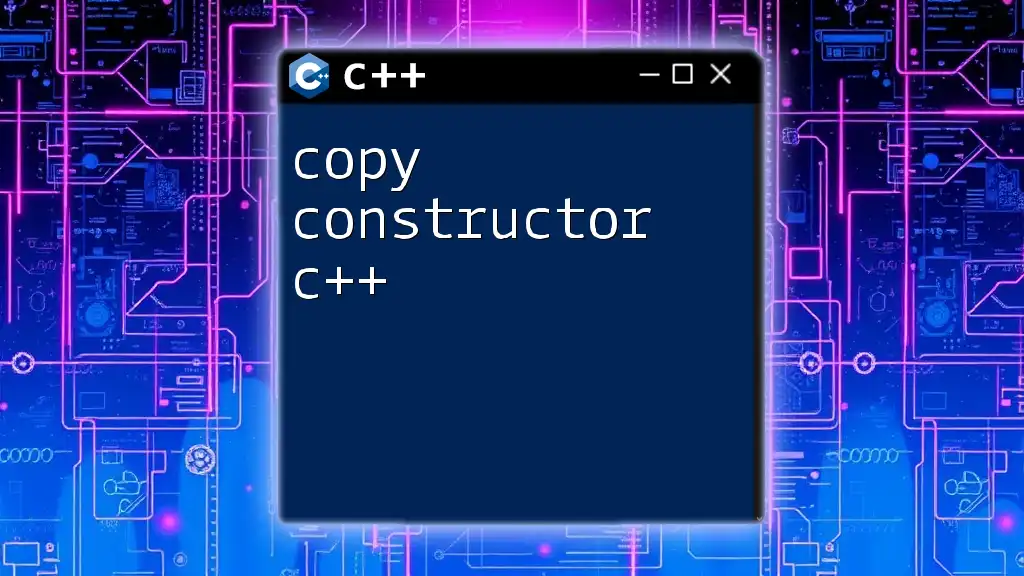
How Const Member Functions Work
Understanding the Behavior
When you call a const method on an object, it ensures that no member variables or data are altered. This behavior is crucial when working with complex data structures and multi-threaded applications, where concurrent modifications may lead to unpredictable behavior.
Accessing Data Members
In a const method, you can read member variables but cannot modify them. This is beneficial as it allows for getting values without risking unintended side effects. Consider the following example:
class Data {
private:
int value;
public:
Data(int v) : value(v) {}
int getValue() const {
return value; // Allowed: returns member variable
}
};
In this example, `getValue` is a const method that simply returns the member variable `value`. The const qualifier reinforces that `getValue` does not change any state within the `Data` class.
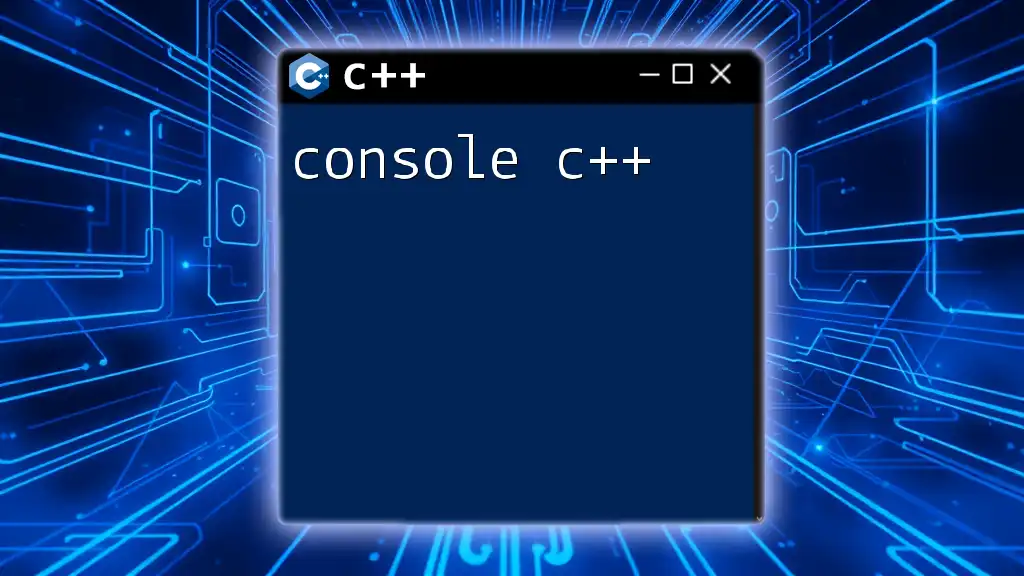
Practical Applications of Const Methods
Use Cases in C++ Programming
Const methods promote safe programming practices by preventing unintentional data modifications. They are especially useful in functions that are supposed to provide read-only access. For example, when retrieving configuration data for a system, you want to ensure that the retrieval methods do not accidentally alter your configuration.
Examples of Const Methods in Action
Example 1: Using Const Method in Class
Here is a more concrete example of a const method in action:
class Point {
private:
int x;
int y;
public:
Point(int xVal, int yVal): x(xVal), y(yVal) {}
void display() const {
std::cout << "Point(" << x << ", " << y << ")" << std::endl;
}
};
In this code, the `display` method will output the coordinates without modifying `x` or `y`. The use of `const` ensures that anyone using this method understands it will not affect the state of the `Point` object.
Example 2: Const Methods with Return Types
Another key aspect of const methods is how they interact with return types. For example:
class String {
private:
std::string text;
public:
String(const std::string& txt) : text(txt) {}
const std::string& getText() const {
return text; // Returns const reference
}
};
In this example, `getText` returns a const reference to `text`. This provides read-only access to `text`, allowing the caller to retrieve the string without the risk of modifying the `text` attribute.
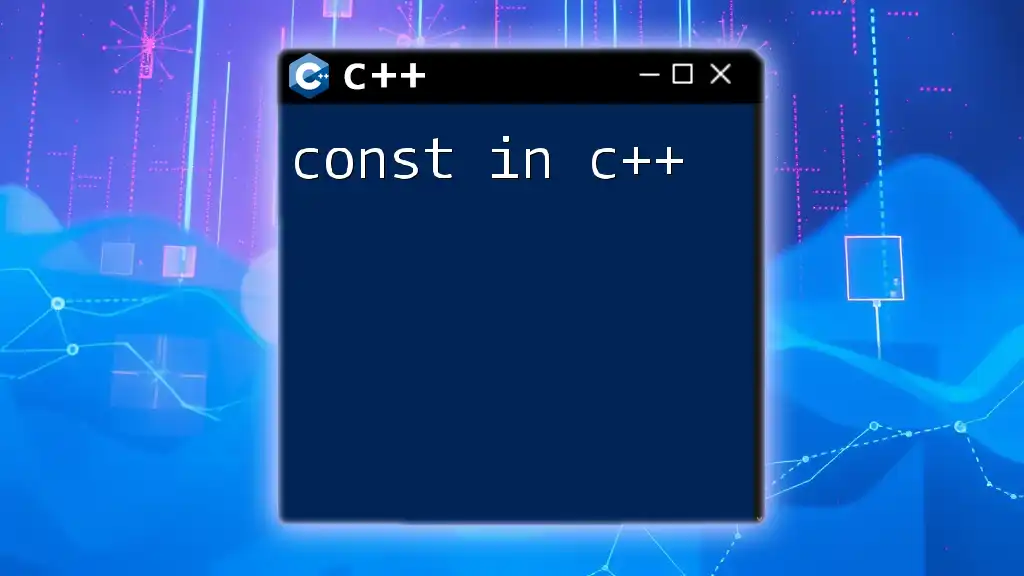
Common Mistakes to Avoid with Const Methods
Misunderstanding Const Behavior
One of the most common mistakes when working with const methods is misunderstanding the implications of the const qualifier. Developers may mistakenly believe that declaring a method as const allows modifications to member variables when, in reality, it does not.
Modifying Member Variables
Another frequent error occurs when attempting to modify member variables within a const method. For instance:
class Test {
private:
int value;
public:
Test(int val) : value(val) {}
void changeValue(int newValue) const {
value = newValue; // Error: Cannot modify value
}
};
This code will trigger a compile-time error because the `changeValue` method is marked as const, which prohibits any changes to `value`.
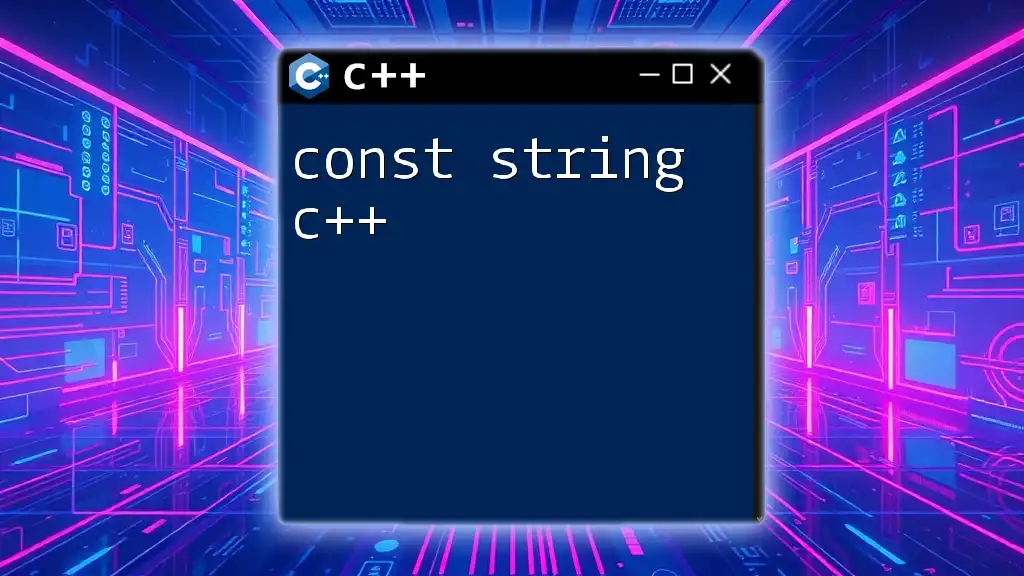
Best Practices for Using Const Methods
When to Use Const Methods
It's advisable to use const methods whenever possible, especially for getters or any methods that do not require changes to object state. By doing so, you express your intent and help other developers understand the operations that are safe to call on different instances of your class.
Organizing Your Code
There’s an undeniable benefit to structuring your classes with const methods. Adopting const methods leads to cleaner, more maintainable code. When reading or reviewing code, seeing const qualifying methods, developers can quickly ascertain which methods won’t alter the object, fostering better understanding and fewer bugs.
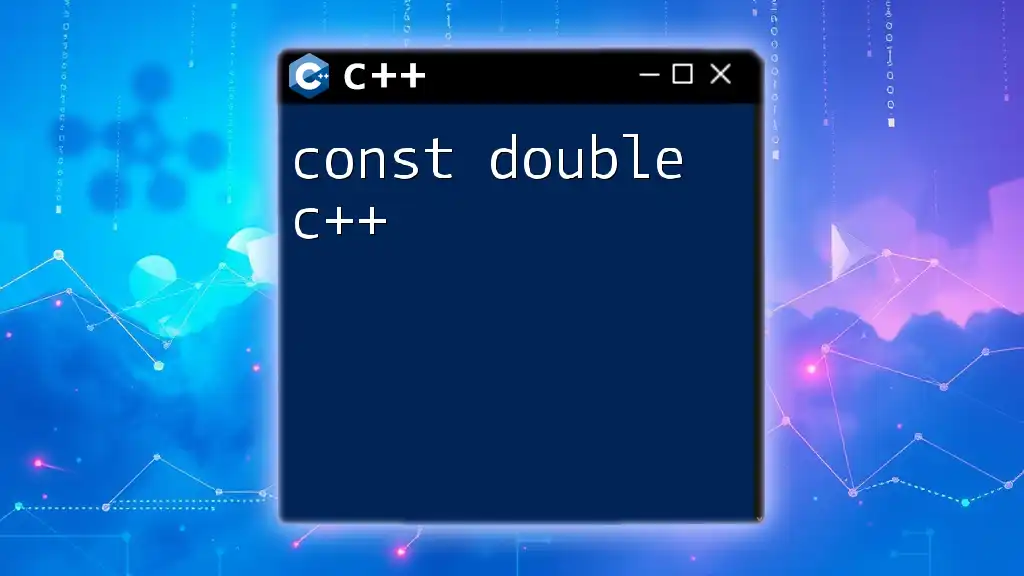
Conclusion
In summary, understanding const methods is crucial for writing reliable and maintainable C++ code. They not only provide a mechanism to safeguard against unintended side effects but also enable a clearer communication of intent within your code.
Leveraging const methods will allow you to design robust classes that are easier to use and understand, ensuring high quality in your programming endeavors.