In C++, concepts are a feature that allows developers to specify constraints on template parameters, ensuring that they meet certain requirements for more robust and readable code.
Here's a simple example of a concept in C++:
#include <iostream>
#include <concepts>
// Define a concept that requires a type to be integral
template<typename T>
concept Integral = std::is_integral_v<T>;
// A function that uses the concept to ensure it only accepts integral types
template<Integral T>
void displayValue(T value) {
std::cout << "The integral value is: " << value << std::endl;
}
int main() {
displayValue(42); // Valid
// displayValue(3.14); // This line would cause a compilation error
return 0;
}
Understanding C++ Concepts
What are C++ Concepts?
C++ concepts are a powerful feature introduced in C++20 that enable programmers to specify constraints on template parameters. A concept defines a set of requirements that types must satisfy to be used in a given template. This approach complements the traditional use of templates, enhancing type checking at compile time and allowing for clearer and more expressive code.
By using concepts, you gain the ability to enforce certain type characteristics, leading to more robust code as you can avoid many of the pitfalls associated with less restrictive template programming.
Why Use Concepts in C++?
Using concepts in C++ provides numerous advantages:
-
Improved Code Readability: Concepts allow you to express your intent clearly in function declarations, making it easier for readers to understand how a function is intended to be used and what types are valid.
-
Enhanced Error Messages: When a type doesn’t satisfy the requirements of a concept, the compiler provides clearer error messages. This is in stark contrast to traditional templates, where errors can often be cryptic and difficult to trace.
-
Better Optimization Opportunities: By specifying precise constraints, the compiler has more information to make optimizations, which can lead to improved performance in certain scenarios.

C++20 Concepts: A Paradigm Shift
Key Features of C++20 Concepts
The introduction of the `concept` keyword in C++20 marks a significant change in how templates are used. Concepts are defined using the following syntax:
template<typename T>
concept Addable = requires(T a, T b) {
{ a + b } -> std::same_as<T>;
};
In this example, we define a concept called `Addable` which checks if a type `T` can be added to itself, resulting in another type of `T`. The use of `requires` and `->` in this definition provides a clean way to specify the conditions without having to rely on more complex SFINAE (Substitution Failure Is Not An Error) techniques.
Applying C++20 Concepts in Code
Implementing concepts in templated functions streamlines the development process significantly. Here’s how you might apply the `Addable` concept in a function:
template<Addable T>
T add(T a, T b) {
return a + b;
}
In this scenario, the function `add()` will only accept types that fulfill the criteria of the `Addable` concept. If you attempt to call `add()` with types that don't meet these criteria, the compiler will issue a clear error message.
Constraints and the Importance of Semantics
The semantics of concepts are crucial for creating well-defined interfaces in your code. When you define your own concepts, you are effectively creating rules that types must adhere to. This is especially helpful in contexts where you have multiple types that share similar operations but require specific implementations.
For instance, consider the following definition of a custom type and its usage:
struct MyInt {
int value;
MyInt operator+(const MyInt& other) {
return MyInt{this->value + other.value};
}
};
You can now use `MyInt` with the `Addable` concept seamlessly, enhancing the type safety of your program.
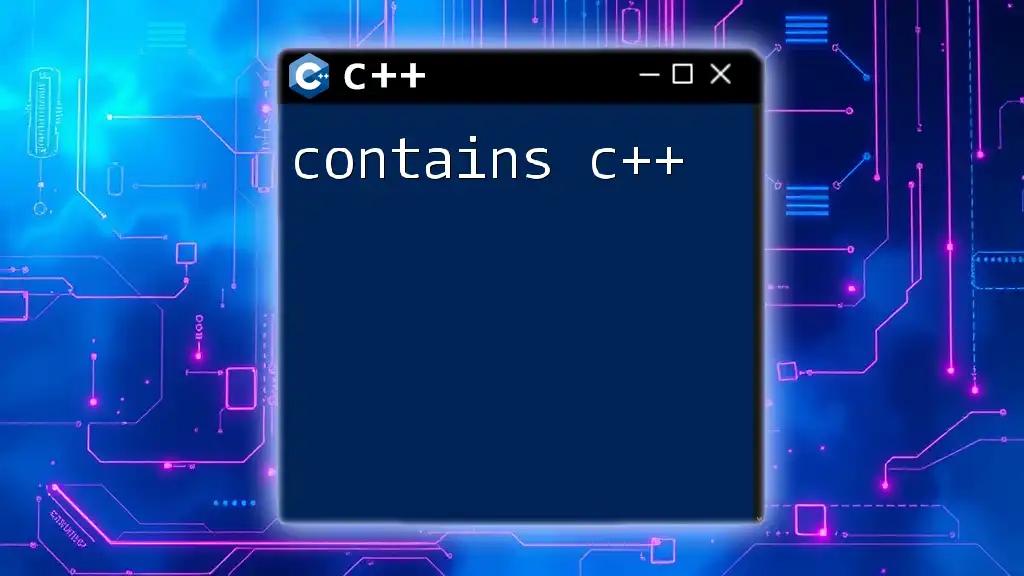
Common C++20 Concepts
Standard Library Concepts
C++20 introduced various standard library concepts that are immediately useful for developers. These include `std::integral`, `std::floating_point`, and many others. Here’s a brief overview of how to use one of these concepts:
template<std::integral T>
T multiply(T a, T b) {
return a * b;
}
In this example, the `multiply` function restricts its input to only integral types, thereby preventing potential runtime errors associated with invalid type inputs.
Custom Concepts for Specific Use Cases
Creating custom concepts tailored to your application can significantly improve code clarity and functionality. Here’s how you can implement a `Sortable` concept:
template<typename T>
concept Sortable = requires(T a) {
{ a.sort() };
};
This `Sortable` concept checks if an instance of type `T` has a `sort` method. By leveraging it in your sorting algorithm, you ensure that only valid types are passed, catching potential errors at compile time.

Practical Applications of C++ Concepts
Enhancing Libraries with Concepts
The integration of concepts in C++ libraries allows for a more robust API that clearly communicates its expectations to users. By using concepts, library authors can help ensure that users provide the correct types, thereby promoting better software practices.
Use Cases in Real-World Applications
Numerous companies and developers have adopted concepts in their codebases to enhance type safety and maintainability. For example, library designs that leverage concepts can yield clear documentation and improve usability. Constraining template parameters with concepts can significantly reduce bugs, which translates into lower development costs and faster time-to-market.

Best Practices for Implementing Concepts in C++
Designing Concepts Thoughtfully
When creating your own concepts, thoughtful naming and clear constraints are essential. Choose names that convey meaning and avoid making concepts overly complicated. Simple and expressive concepts are easier to understand and use.
Integration with Existing Code
If you're working with legacy code, integrating concepts can be approached gradually. You might start by wrapping existing templates in concepts or creating new interfaces that use concepts while keeping the old code intact. The goal is to ensure backward compatibility while progressively enhancing the type safety of your code.
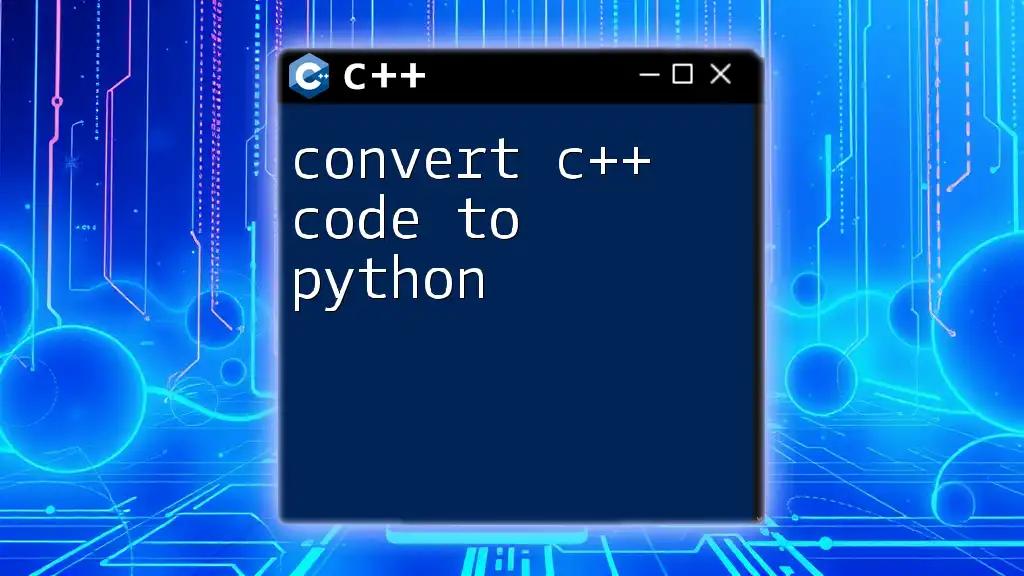
Conclusion
In summary, concepts in C++ represent a major leap forward in how developers can enforce and communicate rules about types in their code. By leveraging the power of concepts introduced in C++20, programmers can create clearer, more maintainable, and safer code. Concepts not only facilitate better understanding but also enhance the compilation experience by providing clearer error messages. As we look to the future of C++ development, the adoption of concepts will undoubtedly play a crucial role in pushing the boundaries of what is possible with type-safe programming. Exploring and implementing concepts in your projects can yield significant rewards, both in code quality and in team collaboration.