The "count" command in C++ typically refers to the `std::count` function from the `<algorithm>` header, which is used to count the occurrences of a specific value in a range of elements.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 2, 5, 2};
int countOfTwos = std::count(vec.begin(), vec.end(), 2);
std::cout << "Number of 2s: " << countOfTwos << std::endl;
return 0;
}
Understanding Counting in C++
In programming, counting typically refers to keeping track of the number of occurrences of a specific element or value within data structures. Understanding how to effectively use counting techniques is fundamental to efficient programming in C++. The need for counting arises in various applications, such as data analysis, sorting algorithms, and iterative processes, making it a core concept every programmer should master.
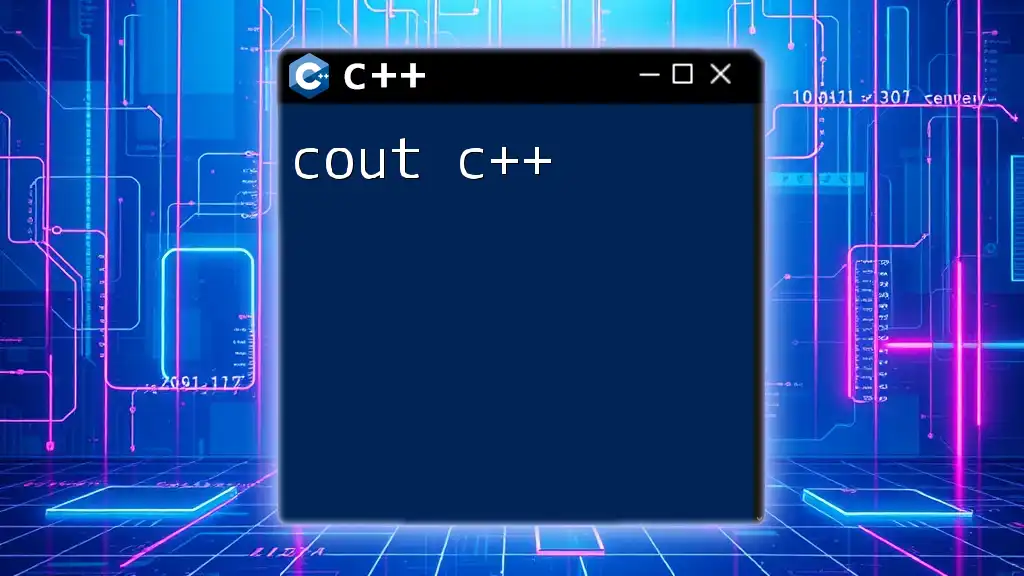
Basic Counting Techniques in C++
Counting with Variables
One of the simplest forms of counting in C++ is through the use of variables. A variable acts as a container for storing data, including counts. Here’s how you can declare and initialize a count variable:
int count = 0; // Initializing a count variable
This initializes `count` to zero, setting you up to start counting as you process data.
Utilizing Loops for Counting
Loops are powerful tools in C++ for automating repetitive tasks, and they can serve as an effective mechanism for counting.
For Loops
For loops are particularly useful when the number of iterations is known beforehand. The basic structure of a for loop allows for concise counting. Consider the following example where we count from 0 to 9:
for (int i = 0; i < 10; i++) {
count++;
}
Here, `count` increments each time the loop runs, resulting in a final value of 10 after the loop completes.
While Loops
In scenarios where the loop continues until a certain condition is met, while loops are more appropriate. Below is an example that demonstrates counting using a while loop:
int count = 0;
while (count < 10) {
// Increment count until it reaches 10
count++;
}
This loop continues running until `count` equals 10, effectively achieving the same end goal as the for loop but with a different structure.
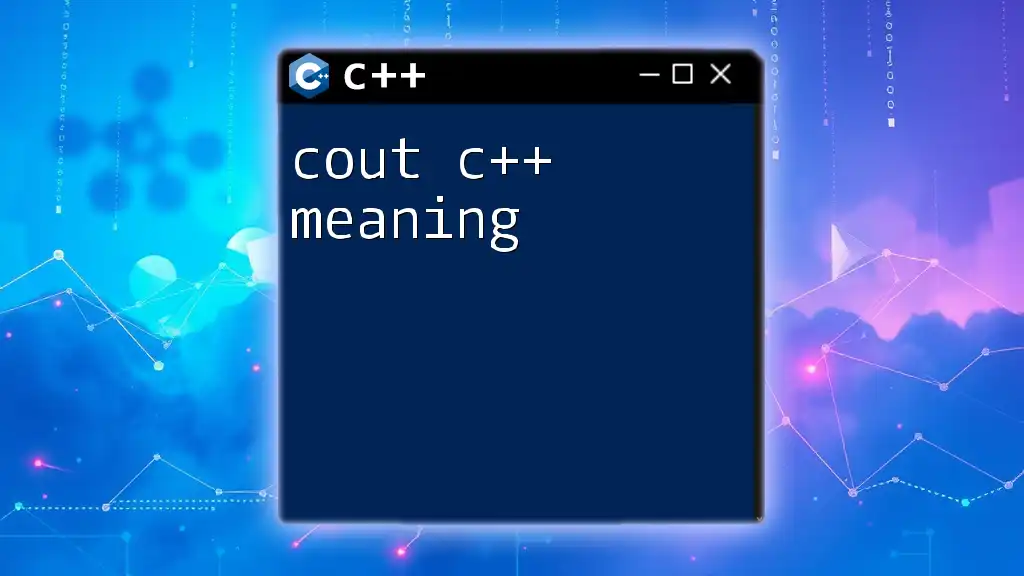
Advanced Counting Ideas
Counting Elements in an Array
C++ arrays provide a fixed-size structure to store multiple values. To count occurrences of a specific value within an array, a basic loop can be employed. Below is a sample code snippet that counts how many times the number `1` appears in the array:
int arr[] = {1, 2, 3, 1, 4, 1};
int count = 0;
for (int i = 0; i < 6; i++) {
if (arr[i] == 1) {
count++;
}
}
In this example, the loop iterates through each element of the array `arr` and increments `count` each time `1` is found.
Counting with STL Containers
The Standard Template Library (STL) in C++ offers dynamic container types like `std::vector` and `std::map`, which simplify counting tasks.
Using `std::vector`
Vectors can dynamically grow in size, making them handy for counting different occurrences effectively. Here’s how to count occurrences of `1` in a vector:
#include <vector>
#include <algorithm> // For std::count
std::vector<int> numbers = {1, 2, 3, 1, 4, 1};
int count = std::count(numbers.begin(), numbers.end(), 1); // Counting 1's
This code utilizes the STL's `std::count` function, which efficiently counts the number of times `1` appears in the vector.
Using `std::map`
Maps offer the ability to associate keys with values, making them suitable for counting unique occurrences of items. Here’s an example of using a map to count each number’s frequency:
#include <map>
#include <vector>
std::vector<int> numbers = {1, 2, 3, 1, 4, 1};
std::map<int, int> countMap;
for (int number : numbers) {
countMap[number]++; // Increment the count for this number
}
In this example, each element's count is stored in `countMap`, effectively tracking how many times each unique number appears.
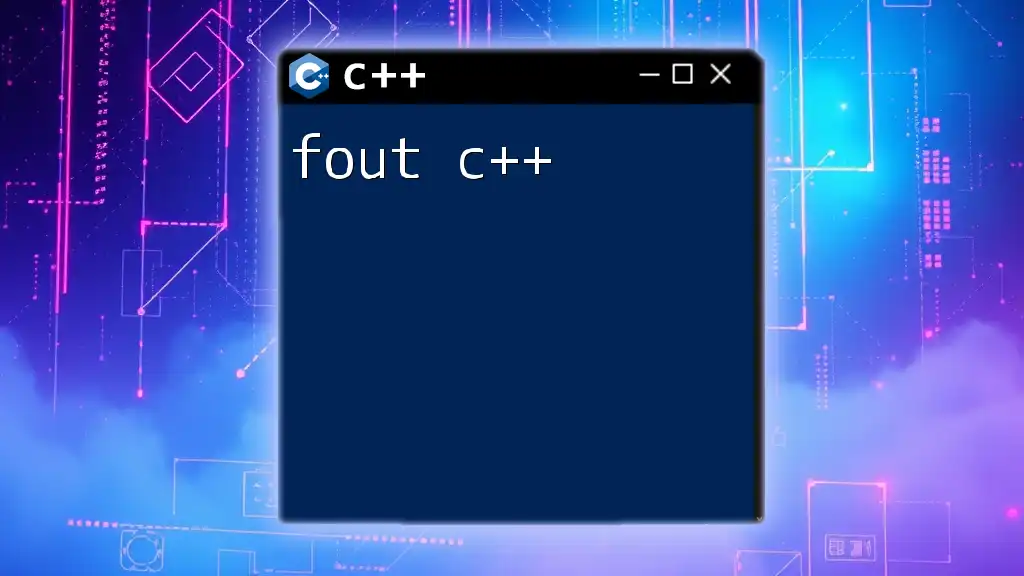
Special Counting Techniques
Factorials and Recursive Counting
In addition to simple counting, C++ supports recursive functions, which can be utilized for more complex counting scenarios, such as calculating factorials. The factorial of a number \( n \) is the product of all positive integers up to \( n \). Here’s how you might code this:
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
This recursive function calls itself until it reaches the base case of \( n \leq 1 \), demonstrating the power of recursion in counting-related tasks.
Counting with Conditionals
Sometimes, you need to apply conditions to your counting logic. For instance, counting how many numbers in a list are even and how many are odd can be useful:
int evenCount = 0, oddCount = 0;
for (int number : numbers) {
if (number % 2 == 0) {
evenCount++;
} else {
oddCount++;
}
}
In this snippet, the loop evaluates each number. Based on whether it's even or odd, it increments the respective counter accordingly.
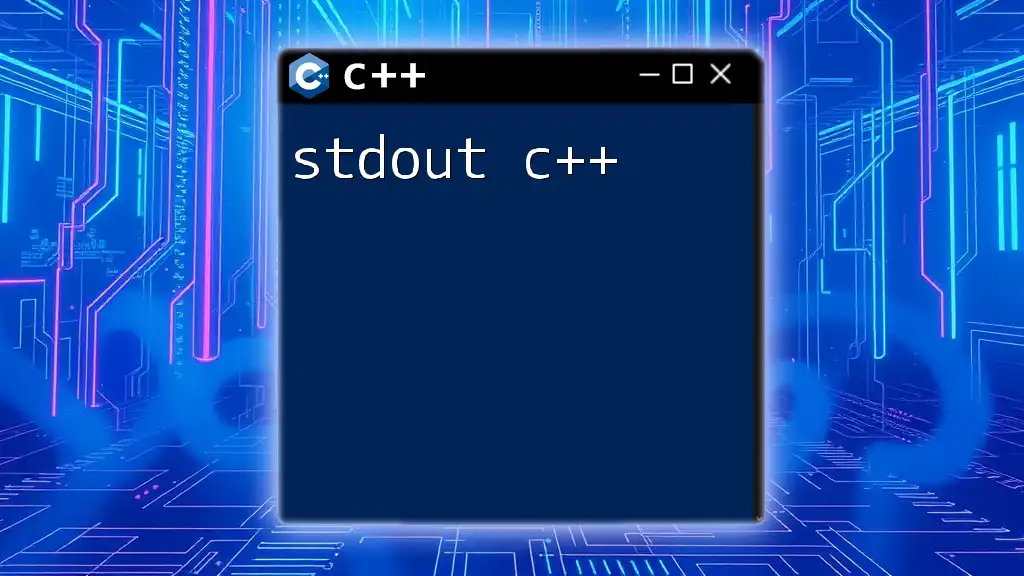
Best Practices for Counting in C++
When working with counting in C++, it’s essential to follow best practices for clarity and efficiency:
-
Use clear and descriptive variable names for your count variables so that the purpose is immediately clear to anyone reading the code.
-
Keep counting logic separate from other calculation parts whenever possible. This enhances readability and maintainability of the code.
-
Consider performance implications of your counting strategies; precomputing certain counts can improve efficiency in scenarios involving large data sets.
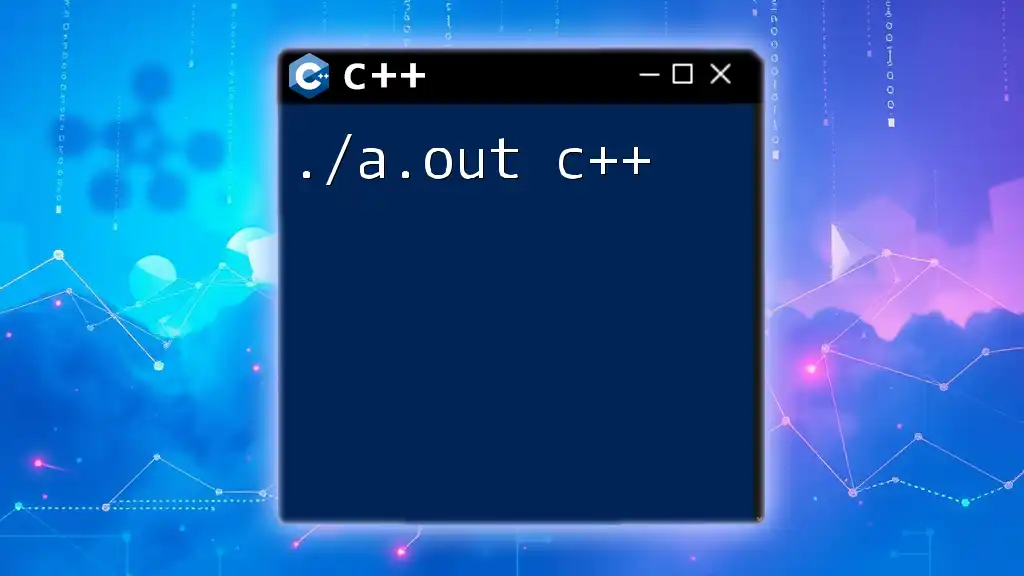
Conclusion
In summary, counting in C++ encompasses a variety of techniques and strategies for effectively tracking occurrences. By mastering the counting methods discussed, programmers can improve their efficiency and problem-solving capabilities in code. Readers are encouraged to practice these techniques to solidify their understanding of how counting works in C++.
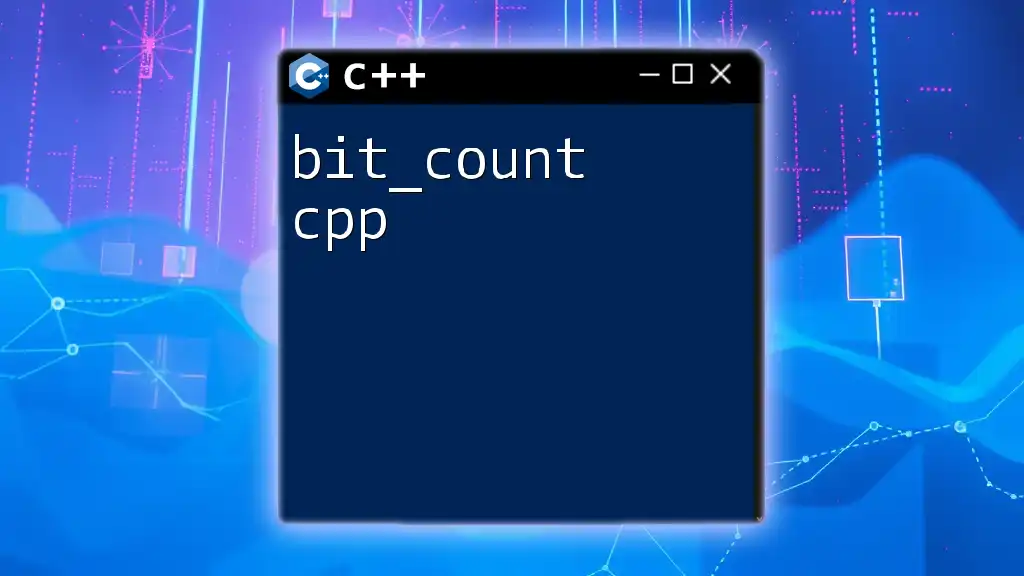
Code Snippets Repository
For those interested in compiling the code snippets discussed in this article, additional resources and downloadable files are recommended for hands-on practice.
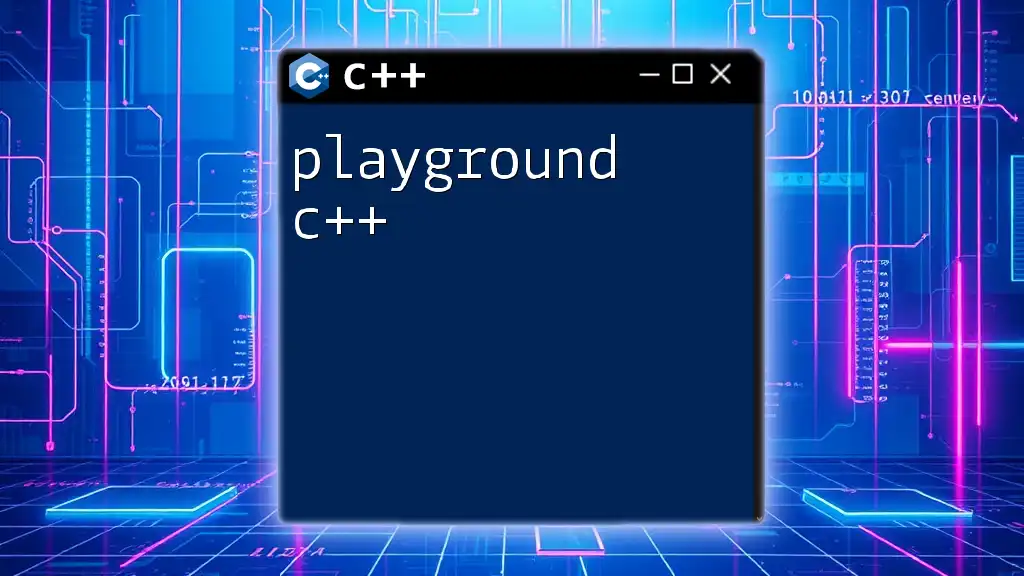
FAQs
What are the common mistakes in counting in C++?
Many common mistakes can arise during counting tasks, such as off-by-one errors, using incorrect data types for counts, or neglecting to reset counters between iterations. Being mindful of these pitfalls can save valuable debugging time.
How does counting help in algorithm efficiency?
Effective counting is often critical in optimizing algorithms, particularly in algorithms that rely on frequency distributions, sorting, and searching tasks. Properly managing counts can lead to significant time savings and improved performance.
Where can I practice my counting skills in C++?
Numerous online platforms such as LeetCode, HackerRank, and Codewars provide coding challenges focused on counting, allowing users to practice and enhance their skills in real-world scenarios.