The `bit_count` function in C++ is used to count the number of set bits (1s) in an integer's binary representation.
Here's a simple example using C++20's `std::bitset`:
#include <iostream>
#include <bitset>
int main() {
int number = 29; // Binary: 11101
std::cout << "Number of bits set in " << number << " is " << std::bitset<32>(number).count() << std::endl;
return 0;
}
Understanding Bitwise Operations
What are Bitwise Operations?
Bitwise operations allow programmers to manipulate individual bits within binary representations directly. Common operators in C++ include:
- AND `&`: Compares each bit of two numbers; if both bits are 1, the result is 1.
- OR `|`: If at least one bit in either number is 1, the result is 1.
- XOR `^`: If bits are different, the result is 1; if they're the same, the result is 0.
- NOT `~`: Flips all the bits; converts 1s to 0s and vice versa.
- Shift Left `<<`: Moves bits to the left, adding zeros on the right.
- Shift Right `>>`: Moves bits to the right, potentially dropping bits on the left.
Understanding these operations is fundamental to mastering binary manipulation, which can result in significant performance improvements in various applications.
Why Count Bits?
Counting bits becomes relevant in numerous scenarios, such as:
- Data Compression: Efficiently representing data often hinges on knowing the number of bits required.
- Error Detection: Algorithms like parity checks rely on counting bits to detect transmission errors.
- Cryptography: In cryptographic applications, understanding bit patterns can help strengthen data security.
- Algorithm Optimization: Certain algorithms can perform better when you have a quick way to count bits.
By leveraging bit counting, you can enhance efficiency and tailor algorithms to create faster, more robust applications.
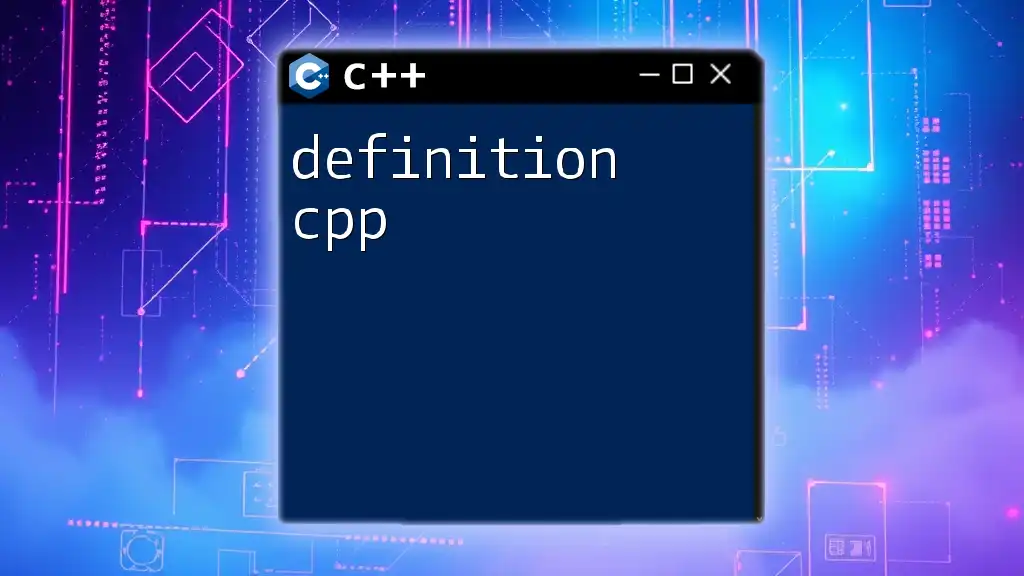
Overview of `bit_count`
Definition and Purpose
The `bit_count` function is a powerful utility in modern C++ that counts the number of set bits (1s) in an integer's binary representation. This simple yet effective feature enables developers to quickly assess the bit composition of numbers without manual calculations.
Syntax and Parameters
The syntax for the `bit_count` function is straightforward:
std::bit_count(number);
Here, `number` is the unsigned integer for which you want to count the set bits. The return type is an `unsigned int` representing the count of set bits. There are no additional parameters needed, keeping the function simple and easy to use.
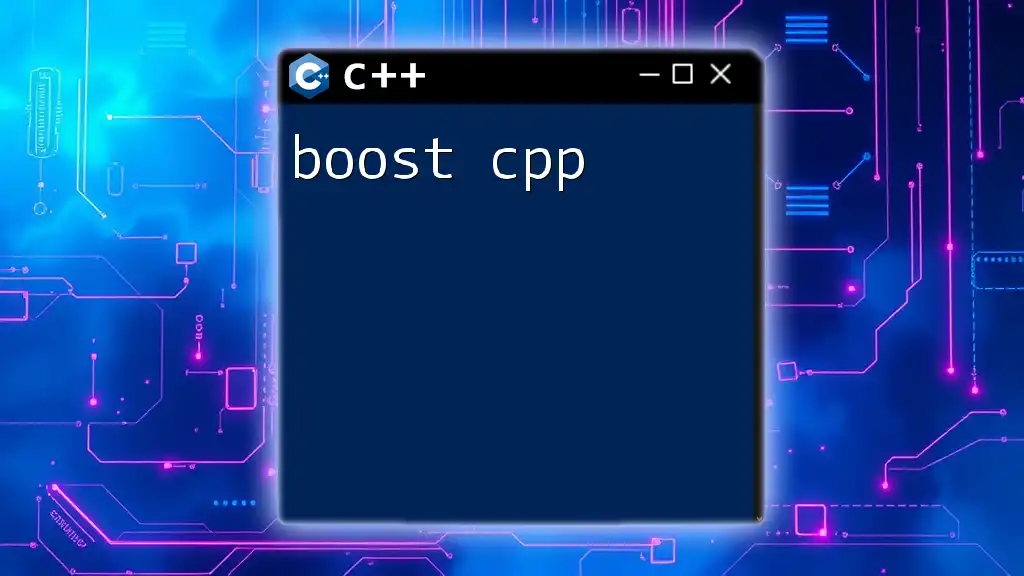
Using `bit_count` in C++
Including Necessary Headers
To use `bit_count`, you must include the appropriate header file:
#include <bit>
This header supplies the required definitions and declarations for bit manipulation functions, making your code cleaner and more efficient.
Basic Example of `bit_count`
Here’s a simple example demonstrating the use of `bit_count`:
#include <iostream>
#include <bit>
int main() {
unsigned int number = 29; // Binary: 11101
std::cout << "The number of set bits is: " << std::bit_count(number) << std::endl;
return 0;
}
In this example, the binary representation of 29 is `11101`, which contains four set bits (1s). The `bit_count` function returns this value, allowing you to display the count effortlessly.
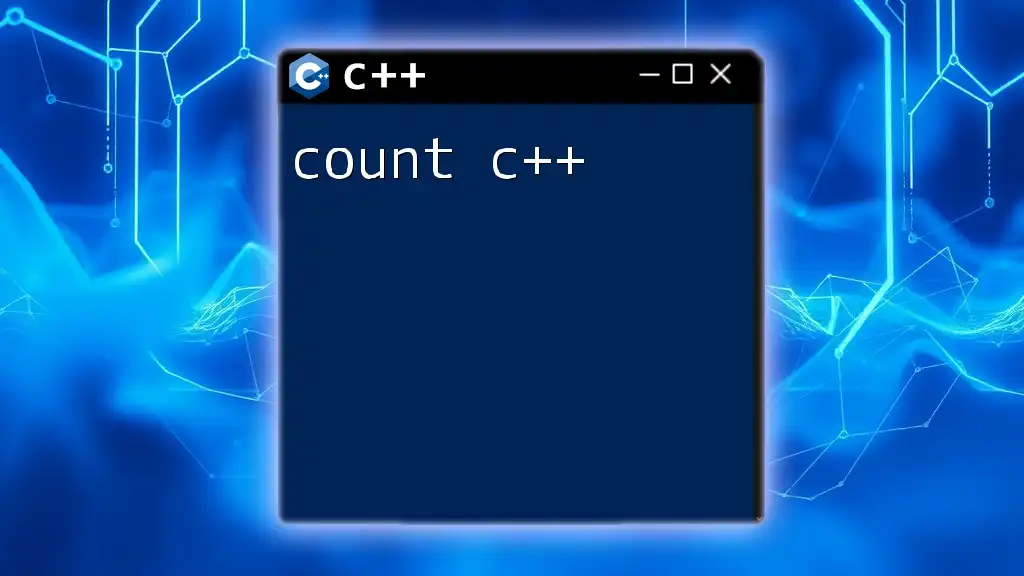
Advanced Use Cases of `bit_count`
Performance Considerations
Using `bit_count` is highly optimized in modern C++. Under the hood, it often utilizes hardware-supported operations that count bits more efficiently than manual methods. When performance is critical, especially in large data structures or real-time systems, employing `bit_count` can yield significant speed improvements.
Handling Large Numbers
`bit_count` is not limited to smaller integers. It can also efficiently count bits in larger data types, such as `long long`. Here’s how you can use it with a large number:
#include <iostream>
#include <bit>
int main() {
long long bigNumber = 123456789012345LL; // Example with a large number
std::cout << "The number of set bits in bigNumber: " << std::bit_count(bigNumber) << std::endl;
return 0;
}
This snippet reveals how effortlessly `bit_count` adapts to larger types, maintaining its efficiency and simplicity.
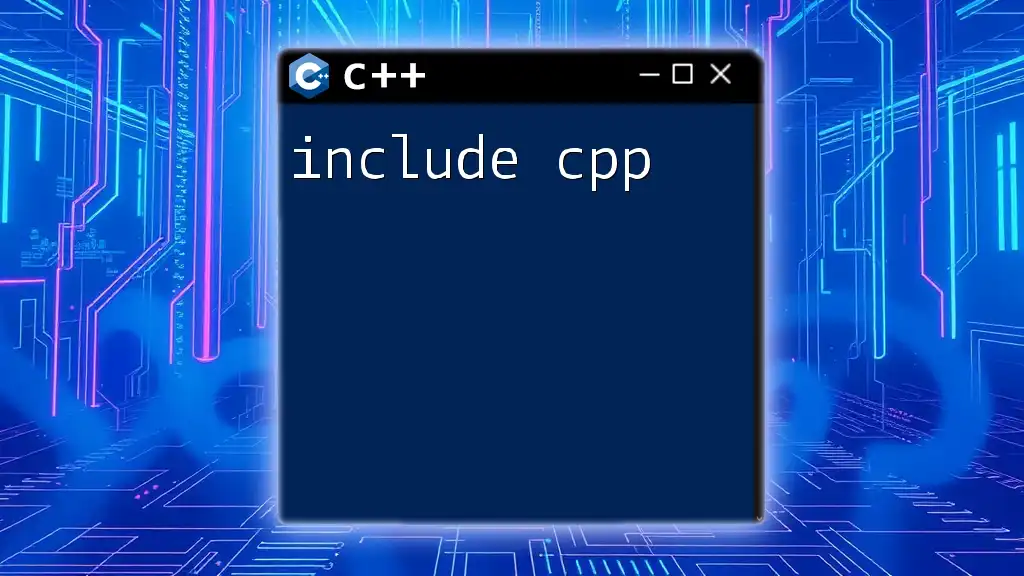
Comparison with Other Methods
Manual Counting of Set Bits
Not all developers are privy to `bit_count`. Traditional methods for counting bits usually involve looping through each bit. For instance, consider the following example:
int countSetBits(unsigned int n) {
int count = 0;
while (n) {
count += n & 1; // Check if least significant bit is set
n >>= 1; // Shift right
}
return count;
}
Though this method works, it can be slower due to the overhead of looping through bits, especially for larger numbers. In contrast, `bit_count` delivers a faster, more direct solution.
Use of Bit Manipulation Techniques
While manual methods and loops are functional, exploring alternative bit manipulation strategies can often yield even more efficiency. Techniques involving bitwise operations combined with clever mathematical insights can lead to optimized algorithms that reduce runtime complexity.
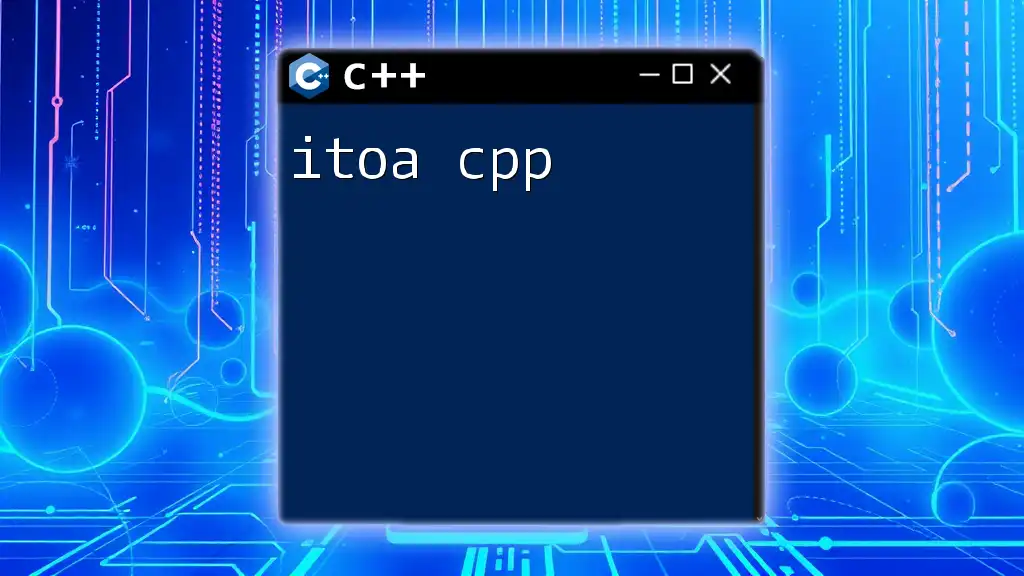
Miscellaneous Tips and Tricks
Common Pitfalls
When using `bit_count`, be aware of some common mistakes:
- Unsigned vs. Signed Integers: Always use unsigned integers with `bit_count` to prevent miscalculations when negative values are involved.
- Inclusion of Headers: Failing to include `<bit>` will lead to compilation errors (undefined functions).
Further Resources for Learning
To deepen your understanding of `bit_count` and bit manipulation in general, consider exploring:
- Online courses focusing on C++ programming concepts.
- Books covering advanced data structures and algorithms.
- Community forums where developers share code snippets and insights.
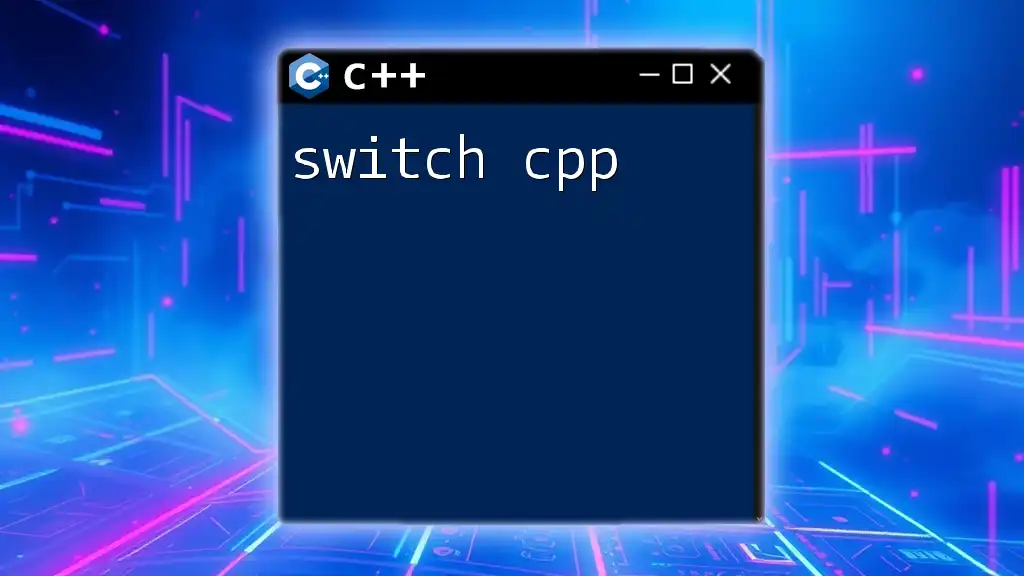
Conclusion
In conclusion, mastering the `bit_count` function in C++ can significantly enhance your programming toolkit. Understanding its syntax, performance benefits, and real-world applications will empower you to solve problems efficiently and effectively in your projects. Experiment with it in various scenarios to unveil its full potential!
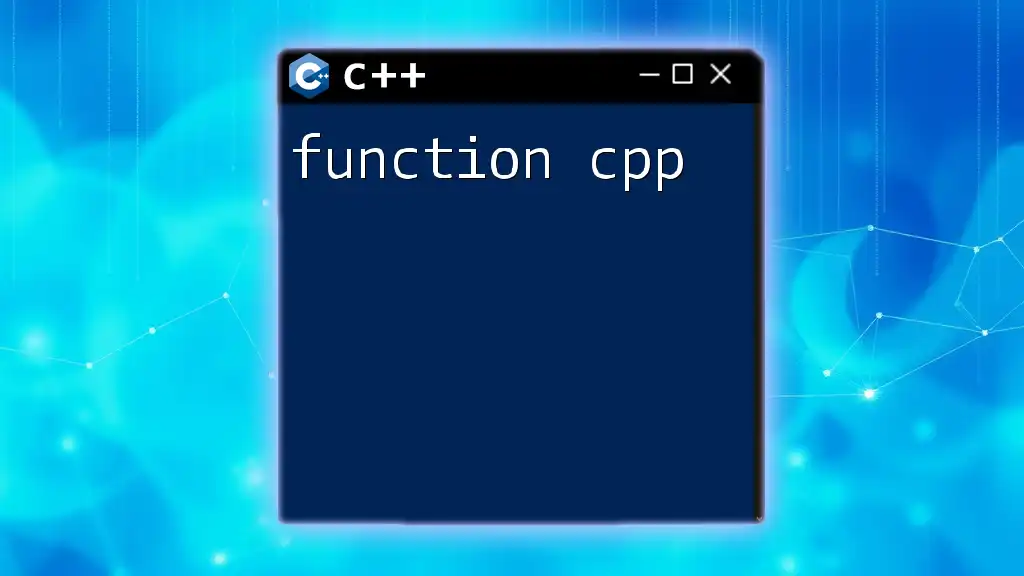
Call to Action
If you found this guide helpful, consider subscribing to our blog for more insightful articles and tutorials on C++ programming and bit manipulation topics. Your journey towards becoming a C++ expert starts here!