The `.cpp` extension signifies a C++ source code file, which contains the written code that can be compiled to create executable programs.
Here is a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that combines the efficiency of low-level programming with the features of high-level languages. Known for its performance and flexibility, C++ supports procedural, object-oriented, and generic programming, making it a versatile choice for a wide range of applications, from system software to game development. The language has a rich set of features, including classes, inheritance, polymorphism, and templates.
Historically, C++ was developed by Bjarne Stroustrup as an extension of the C language in the late 1970s and has since evolved through various versions, with the most recent being C++20. This evolution reflects the ongoing effort to enhance the capabilities of the language, paving the way for the understanding of "extension cpp."
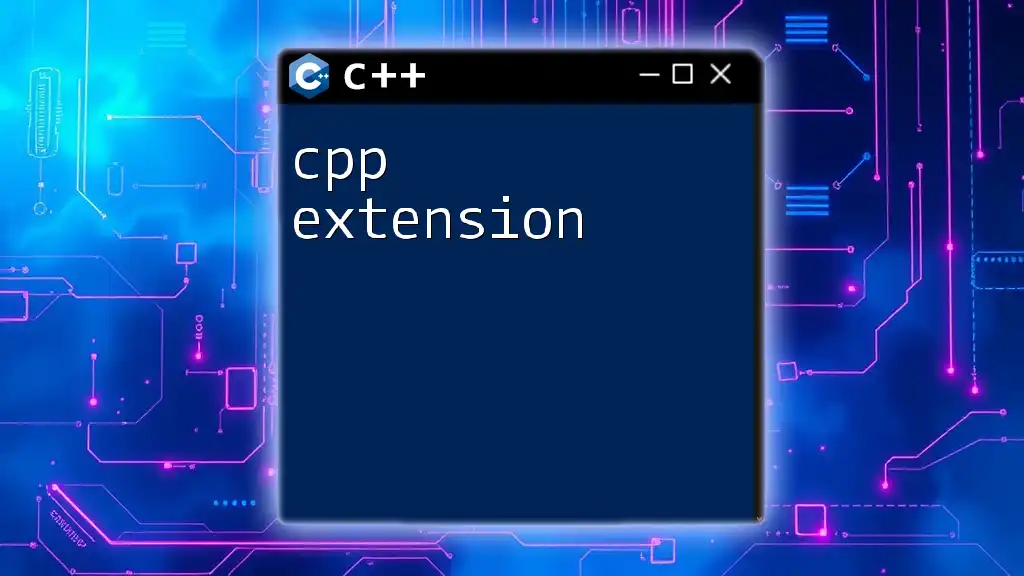
Understanding Extensions in C++
In the context of programming languages, extensions refer to additional features, functionalities, or libraries that enhance the core language capabilities. C++ extensions are crucial for developers as they offer tools to tackle complex problems more efficiently.
The common types of C++ extensions include:
Libraries and Frameworks
Libraries and frameworks are essential for developers to add pre-built functionalities to their applications. They save time and effort by providing ready-to-use code.
Boost is one of the most widely used libraries in the C++ ecosystem. It provides a collection of peer-reviewed portable C++ source libraries that extend C++ functionalities significantly. For example, to use the Boost library for smart pointers:
#include <boost/shared_ptr.hpp>
int main() {
boost::shared_ptr<int> ptr(new int(42));
std::cout << *ptr << std::endl;
return 0;
}
Qt is a comprehensive framework for developing cross-platform applications, particularly for graphical user interface (GUI) applications. It allows developers to create visually appealing and high-performance applications with ease.
OpenCV, short for Open Source Computer Vision Library, is another widely-used library in C++ for real-time computer vision tasks. Using OpenCV, you can easily perform advanced image processing, like so:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
cv::imshow("Display window", image);
cv::waitKey(0);
return 0;
}
Language Extensions
C++ language extensions refer to new features or adjustments to the language syntax that allow developers more expressive and efficient coding. One significant addition is C++20, which introduced several exciting features.
Concepts are one of the most notable features introduced in C++20. They allow for more precise template programming by enabling developers to specify constraints on template parameters. For instance:
#include <concepts>
#include <iostream>
template<typename T>
concept Integral = std::is_integral<T>::value;
template <Integral T>
void printValue(T value) {
std::cout << "Value: " << value << std::endl;
}
int main() {
printValue(10); // works
// printValue(10.5); // would cause a compile error
return 0;
}
Compiler Extensions
Compiler extensions enhance the capabilities of the standard C++ language through specific implementations offered by various compilers, like GCC or Microsoft Visual C++. These extensions often include optimizations or additional features that can increase code performance.
For example, the GCC compiler offers attributes for optimizing the performance of your functions. Consider the following snippet where we use the `always_inline` attribute:
__attribute__((always_inline)) inline void fastFunction() {
// Function code that should always be inlined
}
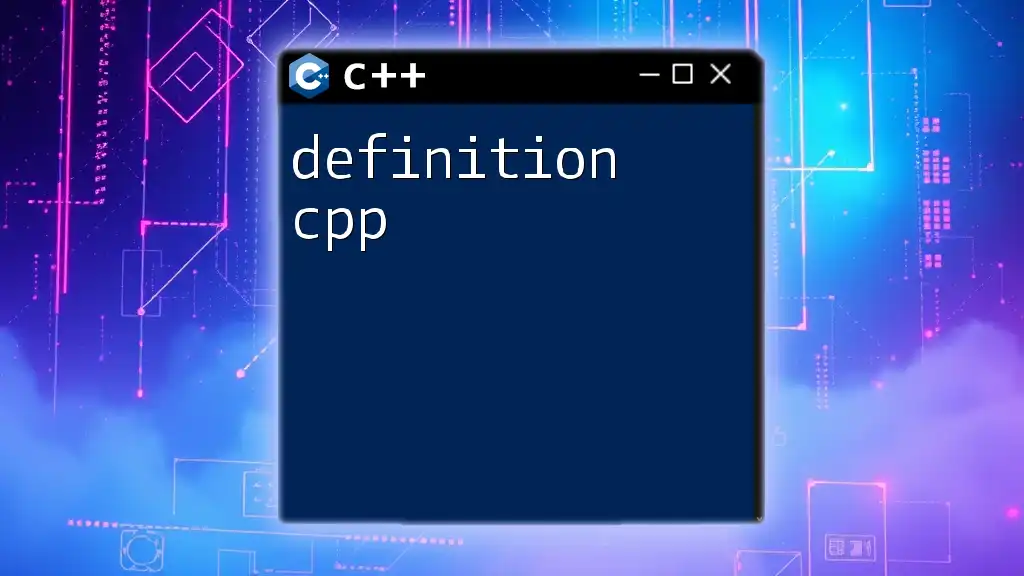
Benefits of C++ Extensions
Using C++ extensions provides countless advantages, improving both productivity and performance in programming projects:
Enhanced Productivity and Functionality
Extensions allow developers to implement complex functionalities without reinventing the wheel. Libraries enable developers to focus on application logic rather than writing boilerplate code.
Improved Standard Library
C++ extensions contribute to improvements in the standard library, providing developers with efficient, pre-tested code implementations that can be utilized directly in projects.
Modular Programming
Modular programming, made possible by C++ extensions, facilitates better organization and easier maintenance of code. Developers can split large code bases into smaller, manageable modules. Using a modular approach encourages better testing and debugging practices.
Here's an example showcasing the use of an extension in a modular design:
// custom_math_extension.h
#pragma once
int square(int number);
// custom_math_extension.cpp
#include "custom_math_extension.h"
int square(int number) {
return number * number;
}
// main.cpp
#include <iostream>
#include "custom_math_extension.h"
int main() {
std::cout << "Square of 5 is: " << square(5) << std::endl;
return 0;
}
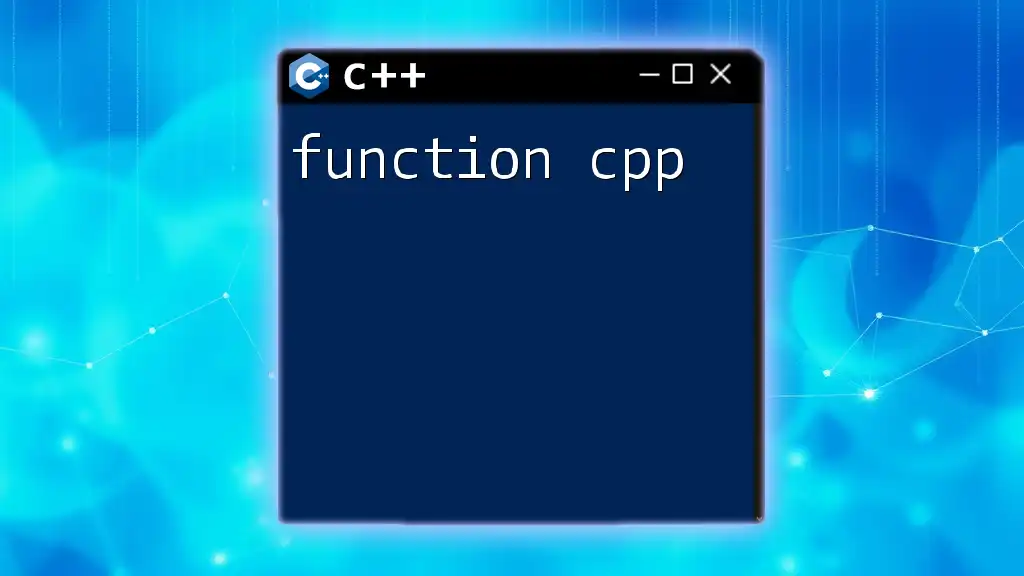
Best Practices for Using C++ Extensions
When incorporating extensions in C++ projects, following best practices can lead to a more organized and efficient workflow:
Choose Wisely and Prioritize Documentation
Select extensions that suit your project needs rather than overloading your application with unnecessary features. Always read through documentation and examples provided by extension maintainers to ensure you fully grasp how to implement them.
Utilize Community Support
Active community support can be invaluable. Engage in forums or support groups to discuss challenges you face and learn from others’ experiences.
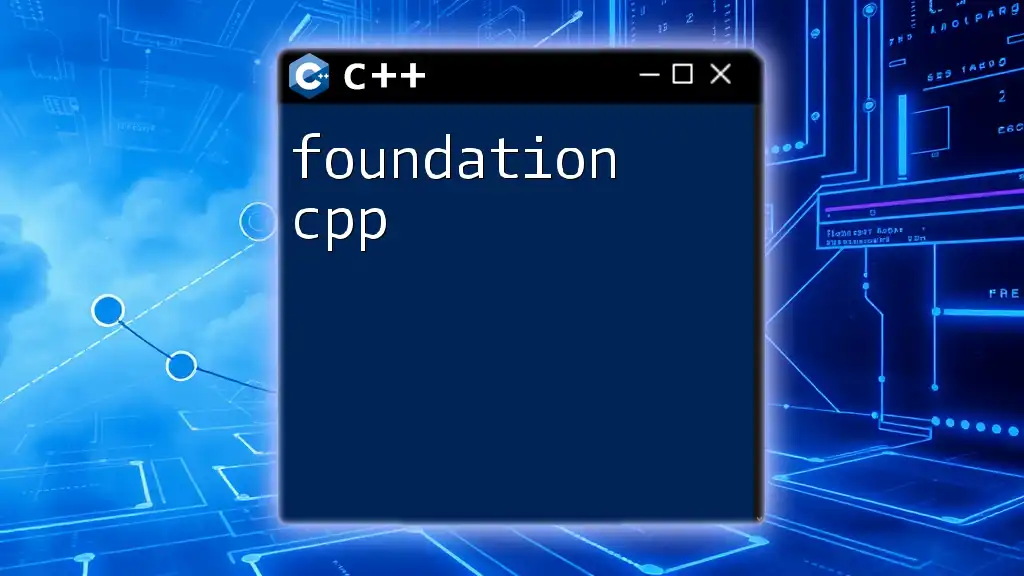
Common Pitfalls and Challenges
While C++ extensions provide many benefits, there are also challenges developers may encounter:
Compatibility Issues
Different compilers implement extensions in various ways. Attributes or functionalities that work in one compiler may not be available in another, causing potential cross-platform issues. Always ensure that the extensions you use are compatible with your target compiler.
Overhead and Complexity
Extensions might introduce additional complexity into your codebase, especially if not documented properly. Be cautious about the reliance on third-party extensions; they may have their own bugs or less community support.
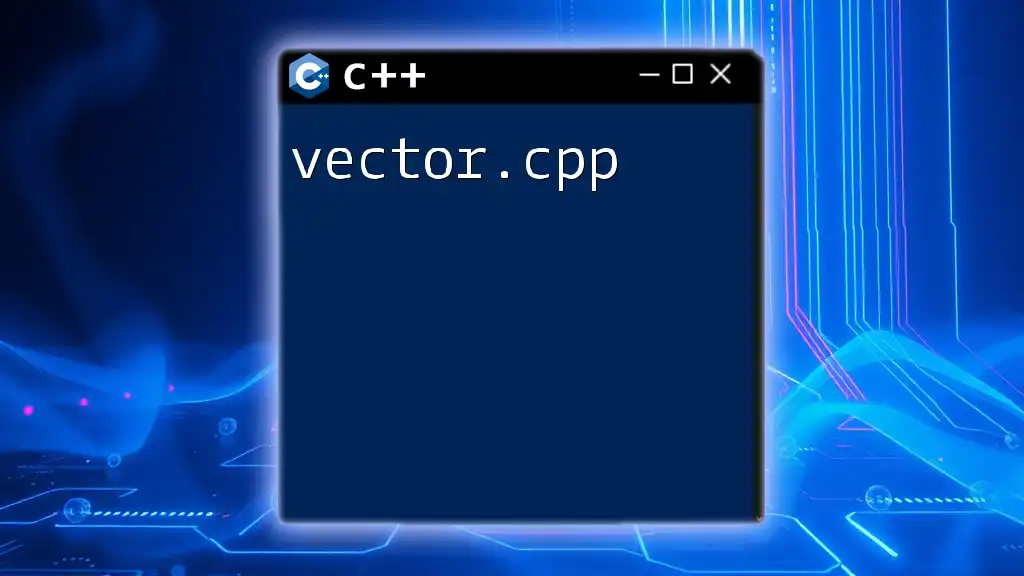
Conclusion
C++ extensions are vital for any programmer seeking to maximize efficiency and capability within the language. They provide tools that can address a wide variety of programming challenges, saving time and improving performance. As you explore the diverse options available with extension cpp, you'll discover countless ways to leverage these features in your projects and ultimately enhance your programming experience.
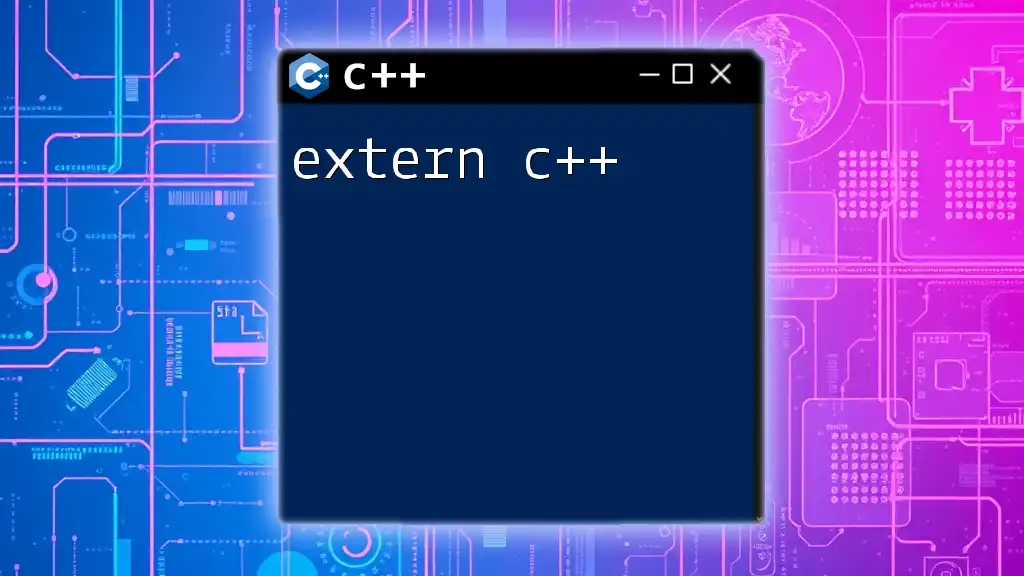
Call to Action
We encourage you to explore various C++ extensions and integrate them into your coding practices. Share your experiences with C++ extensions in the comments section below, and let’s build a community of proficient C++ developers together!