`memset` in C++ is a standard library function used to set a block of memory to a specific value, commonly used for initializing arrays or structures.
#include <cstring>
int main() {
int arr[5];
memset(arr, 0, sizeof(arr)); // Sets all elements of arr to 0
return 0;
}
Introduction to `memset`
`memset` is a powerful and widely used function in C++ that allows developers to quickly set a block of memory to a specific value. This can be particularly useful when initializing arrays or structures, where performance and clarity are crucial. Understanding how to utilize `memset in cpp` efficiently can significantly enhance your coding process and ensure that your data structures are cleanly initialized and reset.
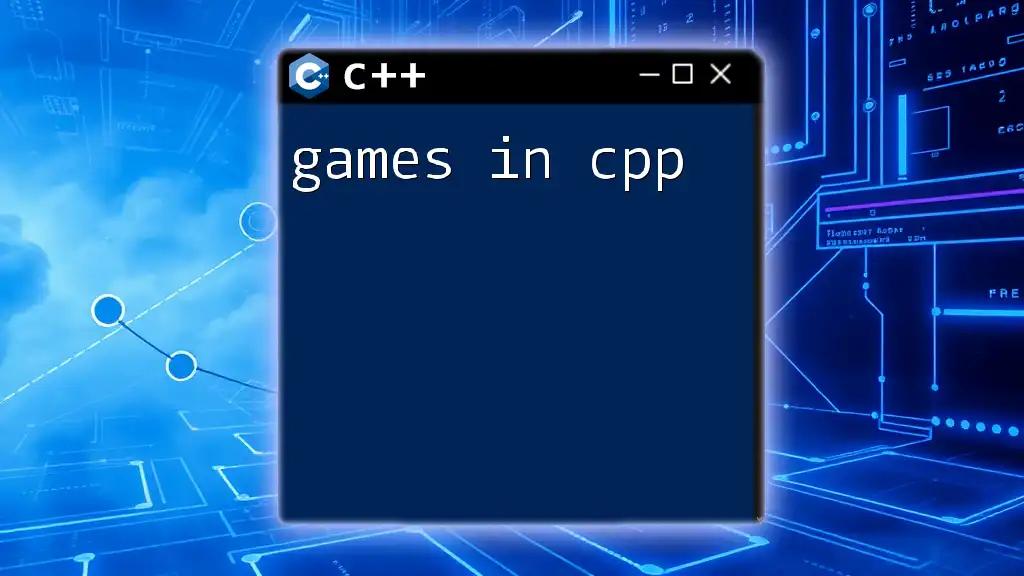
Understanding the Basics of `memset`
What is `memset`?
At its core, `memset` is part of the C standard library and is declared in the `<cstring>` header. It is designed to fill a specified area in memory with a particular byte value. This low-level approach to memory management can make clear and concise initializations much easier.
Function Signature of `memset`
The syntax for `memset` is quite straightforward:
void* memset(void* ptr, int value, size_t num);
Here’s a breakdown of the parameters:
- Pointer to the memory block (`ptr`): This is the starting address of the memory you want to modify.
- Value to be set (`value`): This is the byte value that you want to use to fill the memory. It is treated as an unsigned character.
- Number of bytes to set (`num`): This specifies how many bytes from the start of the address should be filled with the given value.
Return Value of `memset`
The function returns a pointer to the memory block (`ptr`). This allows for function chaining where you can continue to work with the pointer.

When to Use `memset`
Initializing Arrays with `memset`
One of the most common applications of `memset` is initializing arrays. This is crucial because uninitialized arrays can lead to unpredictable behavior in programs. For example, to set all elements of an integer array to zero:
int arr[5];
memset(arr, 0, sizeof(arr));
In this example, all the elements in `arr` are set to zero efficiently in one line.
Clearing or Resetting Memory
Another typical use for `memset` is clearing a data structure or object to ensure it has a known state. For instance, consider resetting a structure to zero:
struct Sample {
int a;
float b;
};
Sample sampleInstance;
memset(&sampleInstance, 0, sizeof(sampleInstance));
This effectively resets all fields of `sampleInstance` to zero, ensuring that no garbage values loom around.
Performance Considerations
Using `memset` can be more efficient than manually looping through each element to assign a value. When set against a background of performance-critical applications, `memset` can save both time and CPU cycles, making it a preferred choice among experienced developers.

Important Points to Consider with `memset`
Limitations of `memset`
While `memset` is a versatile tool, it also comes with limitations. For instance, it should not be used on non-trivial data types, such as classes that contain dynamic memory allocation or complex constructors and destructors.
For example:
class NonTrivial {
public:
NonTrivial() { /* constructor code */ }
~NonTrivial() { /* destructor code */ }
int data;
};
NonTrivial nt;
memset(&nt, 0, sizeof(nt)); // Dangerous: calls non-trivial destructor on deallocation
Using `memset` on such types can lead to undefined behaviors, often resulting in memory leaks or crashes.
Type-Safety Concerns
`memset` does not check for types, which can lead to unintended consequences if used incorrectly. For example, if you were to use `memset` on a class type with pointers:
class PointerClass {
public:
int* ptr;
};
PointerClass pc;
memset(&pc, 0, sizeof(pc)); // Dangerous: this may leave dangling pointers
In this case, `ptr` does not point to a valid memory after the operation, leading to possible crashes if dereferenced.
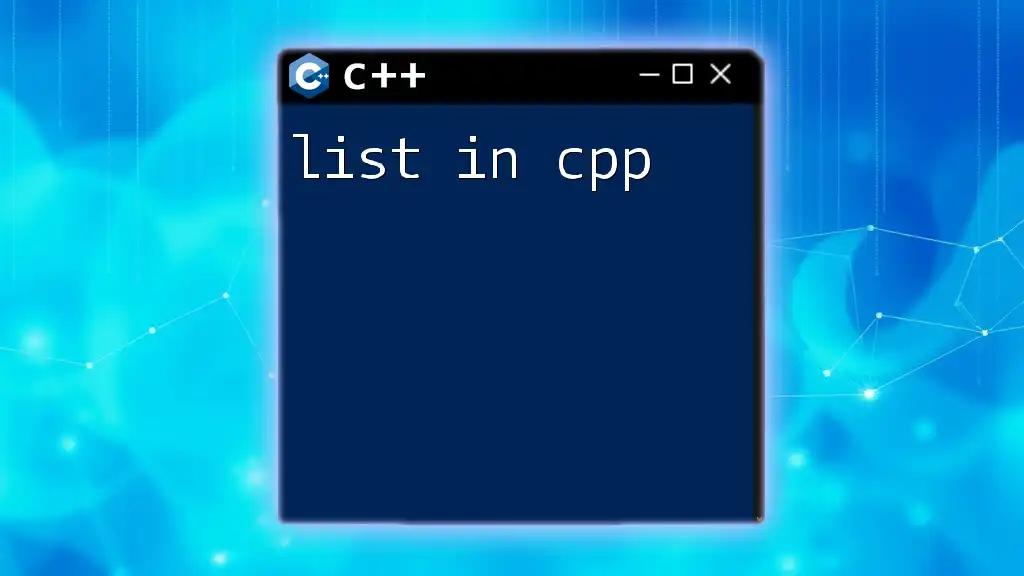
Practical Examples of `memset`
Example 1: Initializing an Array
Here's another example of using `memset` with a character array to populate it with a specific character:
char buffer[10];
memset(buffer, 'A', sizeof(buffer));
In this way, all ten elements in the `buffer` will be set to the character 'A'.
Example 2: Resetting a Class Instance
Consider the following example where we reset a simple class instance:
class Point {
public:
int x;
int y;
};
Point p;
memset(&p, 0, sizeof(p)); // All members are set to zero
This code will reset both `x` and `y` to zero while utilizing the efficiency of `memset`.
Example 3: Using `memset` with 2D Arrays
For multi-dimensional arrays, `memset` can still be used effectively. Here’s an example of resetting a 3x3 integer matrix:
int matrix[3][3];
memset(matrix, 0, sizeof(matrix)); // All elements set to zero
This whole matrix is quickly reset, ensuring that it is initialized correctly.
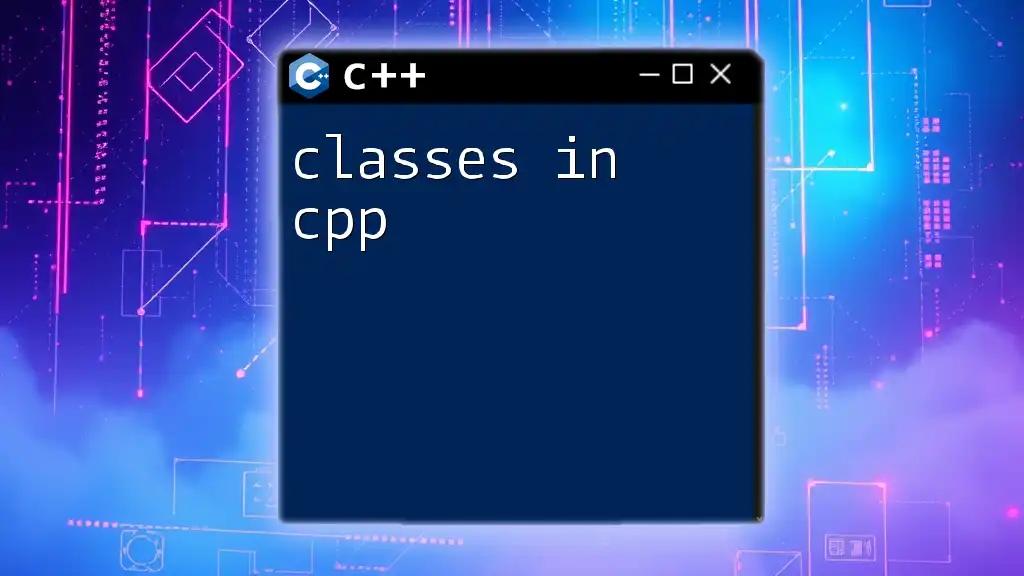
Best Practices for Using `memset`
Always Use with Caution
While `memset` is powerful, it should be employed with caution. Always ensure that the types being passed to it are trivial, meaning they have no complex constructors or destructors.
Alternatives to `memset`
In instances where you are dealing with complex data types, consider using alternative approaches such as constructor initialization or the `std::fill` function from the `<algorithm>` library, which provides a safer approach to initializing or resetting elements.
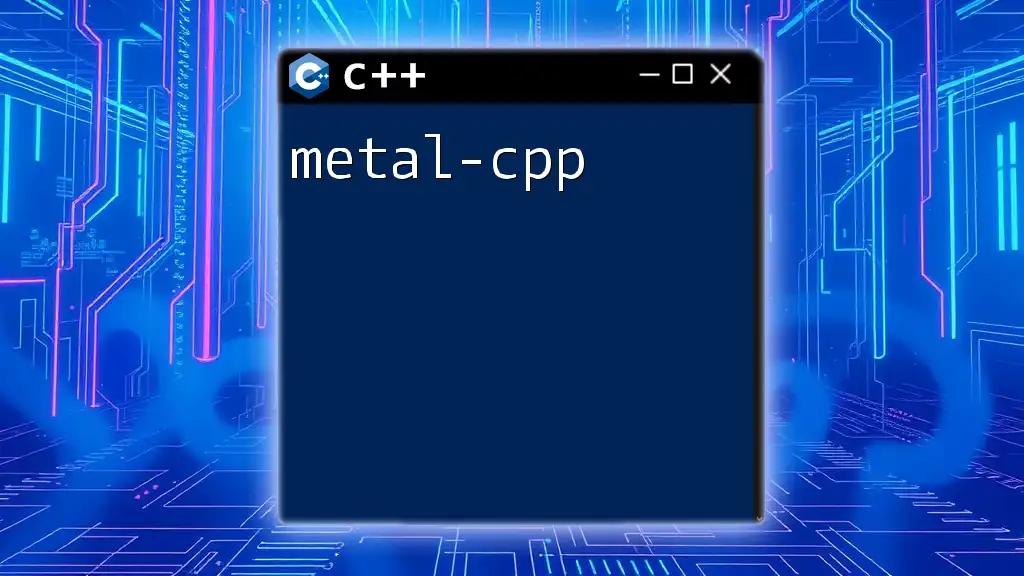
Conclusion
In conclusion, understanding how to use `memset in cpp` is essential for efficient memory management and clear coding practices. Its ability to quickly set large blocks of memory is invaluable in a variety of programming scenarios. However, remember to use it judiciously and be aware of its limitations, particularly with complex data structures. Practicing and experimenting with `memset` will make you more proficient in C++. For deeper knowledge, explore additional learning resources, and consider enrolling in workshops focused on advanced memory management techniques.