`memcpy` is a standard C library function used in C++ to copy a specified number of bytes from one memory location to another, commonly utilized for efficient data transfer between buffers.
#include <cstring> // For memcpy
#include <iostream>
int main() {
char source[] = "Hello, World!";
char destination[50];
memcpy(destination, source, sizeof(source)); // Copying bytes
std::cout << destination << std::endl; // Output: Hello, World!
return 0;
}
Understanding Memory Management in C++
What is Memory Management?
Memory management is a critical aspect of programming that involves allocating and deallocating memory when it is needed and freeing it when it is no longer. In C++, understanding how memory is managed can help avoid leaks, fragmentation, and other issues that arise from inefficient memory handling.
There are two primary types of memory in C++: Stack and Heap. The stack is used for static memory allocation, while the heap is used for dynamic memory allocation. The stack is managed automatically, while the heap requires manual control through pointers and dynamic allocations.
Role of `memcpy` in C++ Memory Management
The function `memcpy` plays a crucial role in directly manipulating raw memory. It allows for efficient copying of blocks of memory from one location to another. This capability is especially useful when dealing with large data structures or when you need to duplicate data quickly without looping through every element individually.
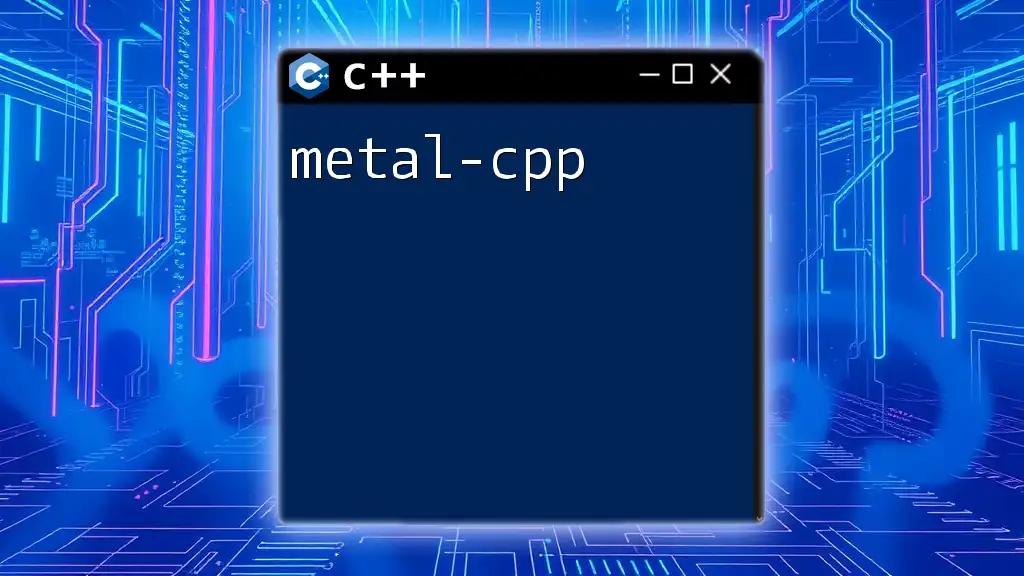
Overview of `memcpy`
Syntax of `memcpy`
The syntax for `memcpy` is straightforward and follows this format:
void* memcpy(void* dest, const void* src, size_t n);
In this function, `dest` is a pointer to the destination memory where the content will be copied. The `src` parameter is a pointer to the source, and `n` is the number of bytes to copy from the source.
Parameters of `memcpy`
- `dest`: Memory address where data will be copied.
- `src`: Memory address from where data will be copied.
- `n`: Specifies the total number of bytes to be copied.
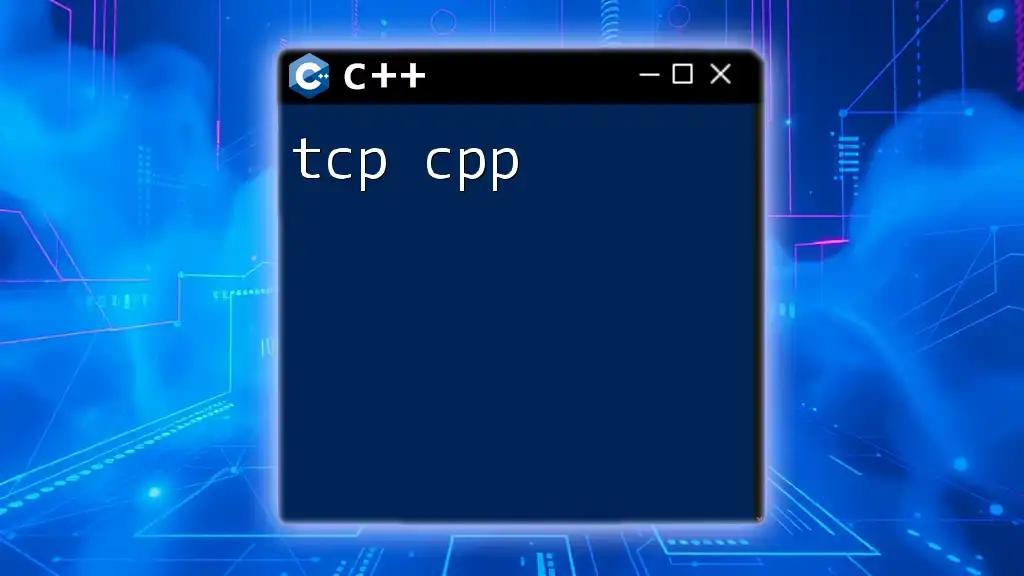
How to Use `memcpy` in C++
Basic Example of `memcpy`
Using `memcpy` is quite straightforward. Here’s a simple example that demonstrates how to copy a string from one character array to another:
#include <iostream>
#include <cstring>
int main() {
char src[] = "Hello, World!";
char dest[20];
memcpy(dest, src, strlen(src) + 1); // Including null character
std::cout << "Copied string: " << dest << std::endl;
return 0;
}
In this code, we declare a source string and a destination character array. We use `memcpy` to copy the contents from `src` to `dest`, ensuring to include the null terminator by adding `1` to the string length.
Advanced Usage
`memcpy` is not limited to character arrays. It can be used to copy complex data types as well. Below is an example demonstrating the usage of `memcpy` with a structure:
struct Data {
int id;
double value;
};
Data srcData = {1, 3.14};
Data destData;
memcpy(&destData, &srcData, sizeof(Data));
Here, we create a structure with two members, `id` and `value`. We then use `memcpy` to copy the contents of `srcData` into `destData`, ensuring that the entire size of the structure is copied.
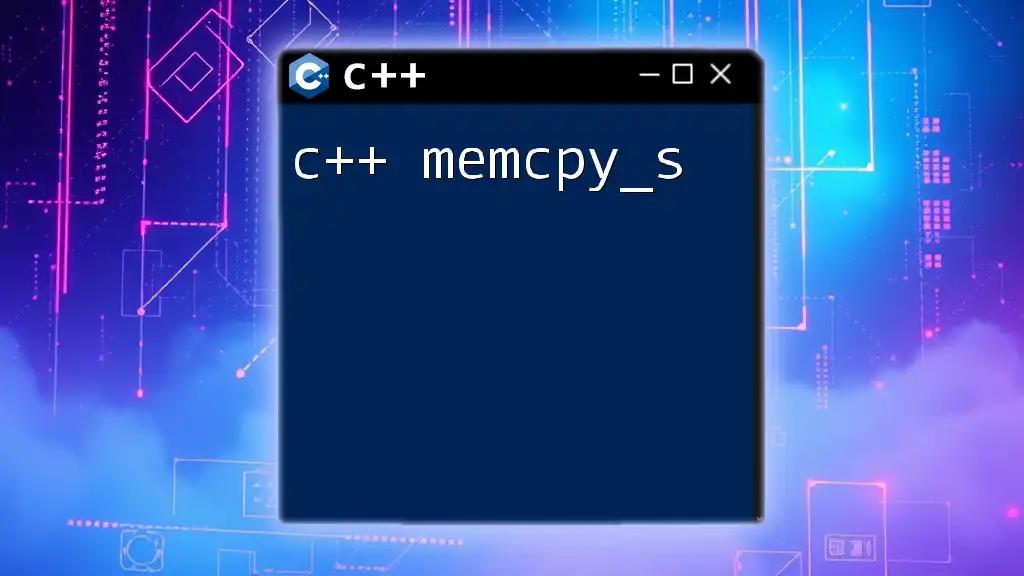
Common Mistakes with `memcpy`
Memory Overlap Issues
One of the critical errors programmers encounter while using `memcpy` is overlapping memory regions. If the source and destination overlap, the results can be unpredictable. To handle such scenarios, you should opt for `memmove`, which safely manages overlapping regions.
Incorrect Size Calculation
Another common mistake is improperly calculating the size of bytes to copy. It's essential to ensure that the `n` parameter correctly represents the number of bytes. In scenarios where this is miscalculated, you could be copying more bytes than the destination can handle, leading to buffer overflow scenarios.
Consider the example below, where the usage is incorrect:
// Incorrect usage
memcpy(dest, src, sizeof(src)); // May exceed buffer size
This will copy a potentially invalid number of bytes, risking corruption of memory.
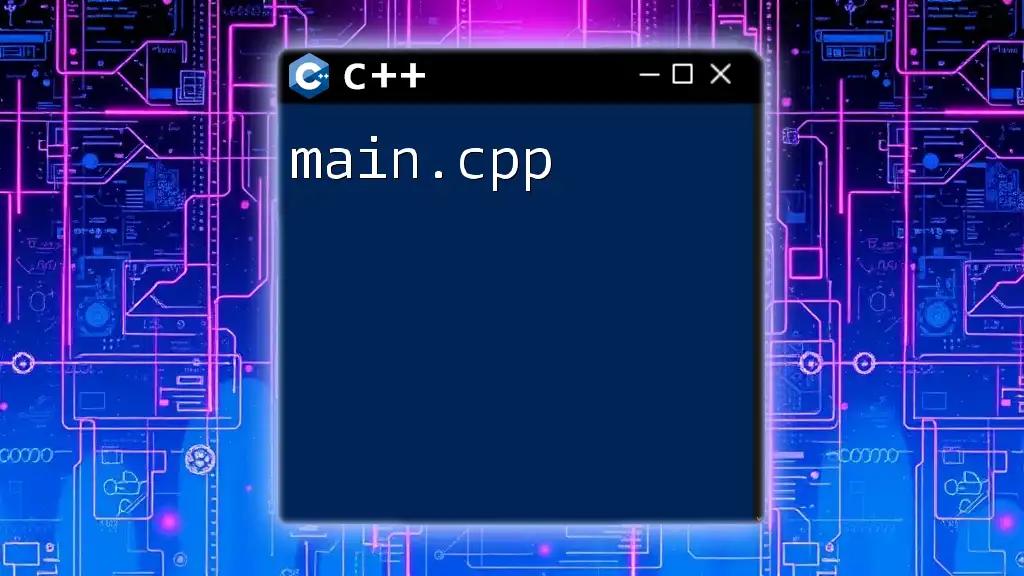
Performance Considerations
Efficiency of `memcpy`
When considering performance, `memcpy` is often faster than manually copying data element by element within a loop. This function is optimized by compilers and can take advantage of hardware-level features for bulk data manipulation.
Compiler Optimizations
Modern compilers can optimize `memcpy` in various ways, including using assembly code under the hood to speed up the operation. Understanding these optimizations can help you appreciate when to use `memcpy` versus relying on manual loops that could introduce unnecessary delays.
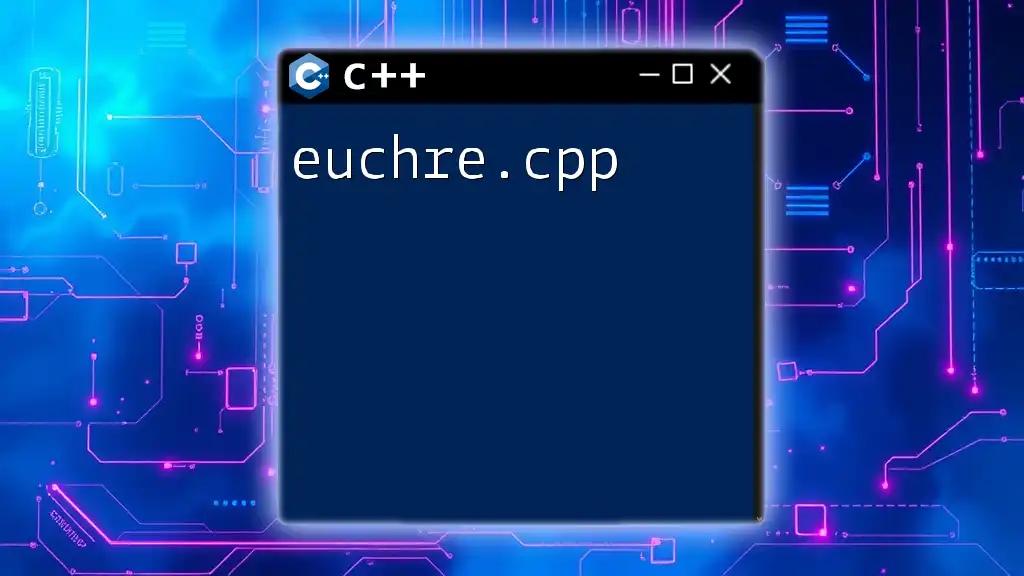
Alternatives to `memcpy`
Using `std::copy` from <algorithm>
In certain situations, using the C++ Standard Library's `std::copy` is preferred, especially when working with STL containers. This function provides type safety and can be more readable than `memcpy`.
#include <algorithm>
std::copy(src, src + size, dest);
This approach also handles overlapping regions safely, which is crucial in software development.
Utilizing `std::memmove`
When you expect that the source and destination regions may overlap, it’s safer to use `std::memmove`. Unlike `memcpy`, which does not guarantee safety in such cases, `memmove` ensures that data is copied correctly without corruption:
std::memmove(dest, src, n);
This is essential when you’re uncertain whether your memory blocks will intersect.

Conclusion
Key Takeaways
The `memcpy` function is an invaluable tool in C++ for efficiently copying memory. However, understanding its intricacies, including the potential pitfalls and proper usage, is essential for effective memory management. Always be mindful of memory overlaps and buffer sizes to avoid unstable behavior and crashes.
Encouragement to Practice
To truly master `memcpy cpp`, practice with various data types, sizes, and scenarios. Consider experimenting with both `memcpy` and its alternatives to see which fits your needs best. This hands-on approach will improve your coding skills and increase your understanding of memory management in C++.
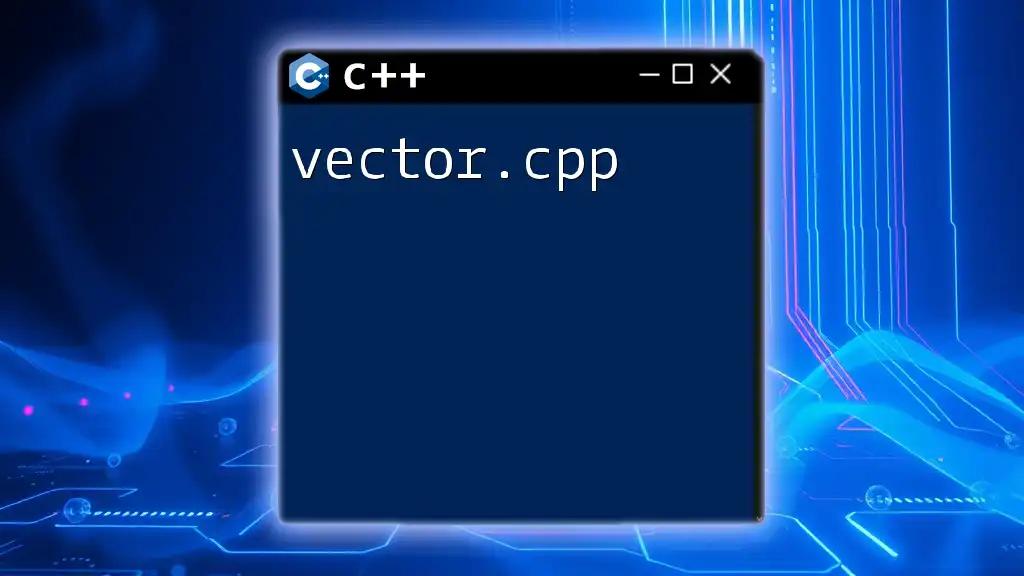
Additional Resources
Further Reading and References
Investigate further into books like "The C++ Programming Language" by Bjarne Stroustrup, as well as online resources and tutorials focused on memory management in C++.
Links to Tutorials and Practice Problems
Take the initiative to tackle practice problems and coding challenges that utilize `memcpy` and similar functions, which will sharpen your understanding and application of these concepts in real-world scenarios.