OpenTelemetry is an observability framework designed to collect and export telemetry data such as metrics and traces from C++ applications, and you can find its GitHub repository at [OpenTelemetry C++ GitHub](https://github.com/open-telemetry/opentelemetry-cpp).
Here's a code snippet demonstrating how to initialize OpenTelemetry's tracing:
#include <opentelemetry/sdk/trace/tracer_provider.h>
#include <opentelemetry/exporters/otlp/otlp_http.h>
namespace trace = opentelemetry::trace;
namespace sdktrace = opentelemetry::sdk::trace;
int main() {
// Create an OTLP exporter
auto exporter = std::make_shared<opentelemetry::exporter::otlp::OtlpHttpExporter>();
// Create a trace provider
auto tracer_provider = std::unique_ptr<trace::TracerProvider>(
new sdktrace::TracerProvider(std::move(exporter)));
// Set the global tracer provider
trace::Provider::SetTracerProvider(std::move(tracer_provider));
// Get a Tracer
auto tracer = trace::Provider::GetTracerProvider()->Get("example_tracer");
}
Overview of OpenTelemetry
What is OpenTelemetry?
OpenTelemetry is a unified framework designed for observability across distributed systems. By providing standardized tools for tracing, metrics, and logging, it helps developers monitor their applications effectively. The goal is to simplify observability in cloud-native environments, allowing for better performance tracking and debugging.
The Importance of OpenTelemetry in C++
In the realm of software development, particularly for C++ applications, observability is crucial. Instrumentation allows developers to gain insights into application performance, resource usage, and user behavior. By implementing OpenTelemetry, developers can track performance in real-time, thus identifying bottlenecks and optimizing system efficiency.
C++ is often used in systems programming, game development, and high-performance applications. In these scenarios, the need for precise monitoring and tracing becomes even more significant. OpenTelemetry serves as a versatile solution, capable of adapting to various C++ use cases to enhance performance monitoring.
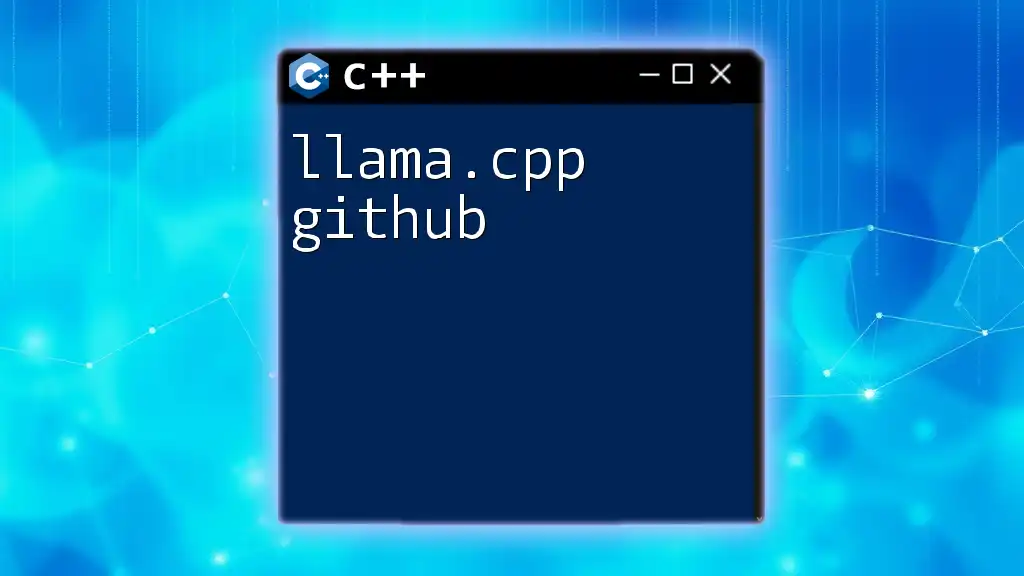
Getting Started with OpenTelemetry C++
Setting Up Your Environment
Before diving into OpenTelemetry, it's essential to ensure your development environment is ready. You will need:
- A compatible C++ compiler (such as g++, Clang, or Visual Studio).
- CMake, which is crucial for managing project builds.
- Git, to clone the OpenTelemetry repository.
Once you have the necessary tools installed, you're ready to move on to the installation process.
Cloning the OpenTelemetry C++ Repository
To start using OpenTelemetry in your C++ applications, you can easily clone the repository from GitHub. Use the following command:
git clone https://github.com/open-telemetry/opentelemetry-cpp.git
After cloning, explore the directory structure. The key directories include:
- include/: Contains header files for OpenTelemetry.
- src/: This folder comprises the source files for the libraries.
- examples/: Offers practical examples of instrumentation.
Understanding this structure will assist you as you integrate OpenTelemetry into your projects.
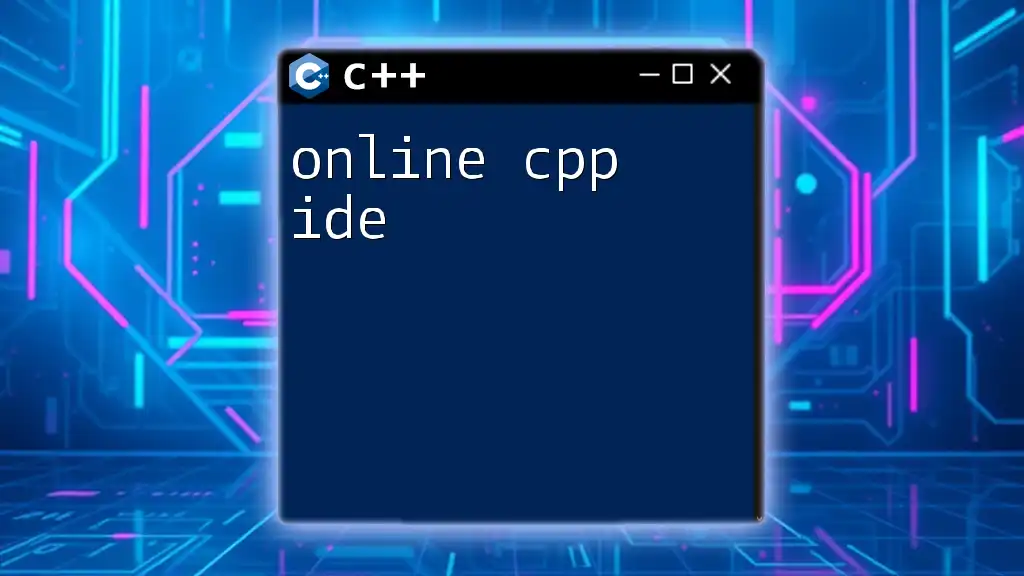
Essential Components of OpenTelemetry C++
Overview of Key Libraries
OpenTelemetry for C++ is made up of several essential libraries:
-
Tracing Library: This library facilitates the creation and management of spans, which represent work units. Using this library, you can trace how requests propagate through your application.
-
Metrics Library: This library allows you to collect quantitative data regarding application performance and resource usage, enabling you to analyze trends and make informed decisions.
-
Logging: Integrating logging with OpenTelemetry provides a comprehensive view of your application's behavior, helping diagnose issues effectively.
Example: Basic Tracing in C++
Setting up basic tracing is a straightforward process. You can create a simple application that demonstrates the use of spans in OpenTelemetry.
Here's an illustrative example of a simple C++ application:
#include <opentelemetry/trace/tracer.h>
using namespace opentelemetry::trace;
void exampleFunction() {
auto tracer = global_tracer->GetTracer("example_tracer");
auto span = tracer->StartSpan("exampleFunction");
// Your code logic here...
span->End(); // End the span after completing the function's logic
}
In this code snippet, we create a tracer instance and start a span to represent the execution of `exampleFunction`. This allows tracking the function’s execution within your application.
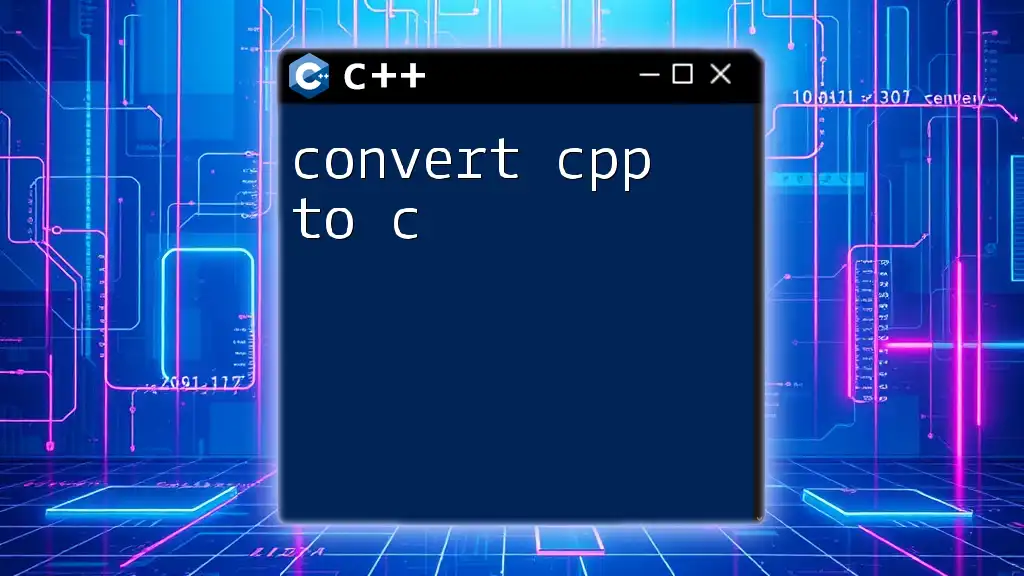
Integrating OpenTelemetry with Your Application
Instrumentation Approaches
Integrating OpenTelemetry can be done in two primary ways: manual instrumentation and automatic instrumentation.
-
Manual Instrumentation: This approach gives you explicit control over what you want to track. It involves adding telemetry code directly into your application where necessary, providing tailored insights. However, it can be labor-intensive.
-
Automatic Instrumentation: OpenTelemetry offers various tools and libraries that automatically instrument your existing code, capturing telemetry without requiring significant code changes. This method is generally more convenient, especially in larger applications.
Real-world Example: Adding OpenTelemetry to a C++ Service
Imagine you have a basic HTTP service written in C++. By instrumenting this service, you can gain visibility into its performance metrics and traces.
Here’s how you might approach this:
- Create a basic service: Lay out the fundamental structure of your C++ HTTP service.
- Integrate OpenTelemetry: Add relevant headers and configure the OpenTelemetry components.
Code Snippet: Adding automatic instrumentation to an HTTP client:
#include <opentelemetry/instrumentation/http_client.h>
// Configure automatic instrumentation for an HTTP client
auto http_client = opentelemetry::instrumentation::HttpClient();
In this example, we set up automatic instrumentation for an HTTP client, allowing you to track requests and responses without further modifications to your client code.
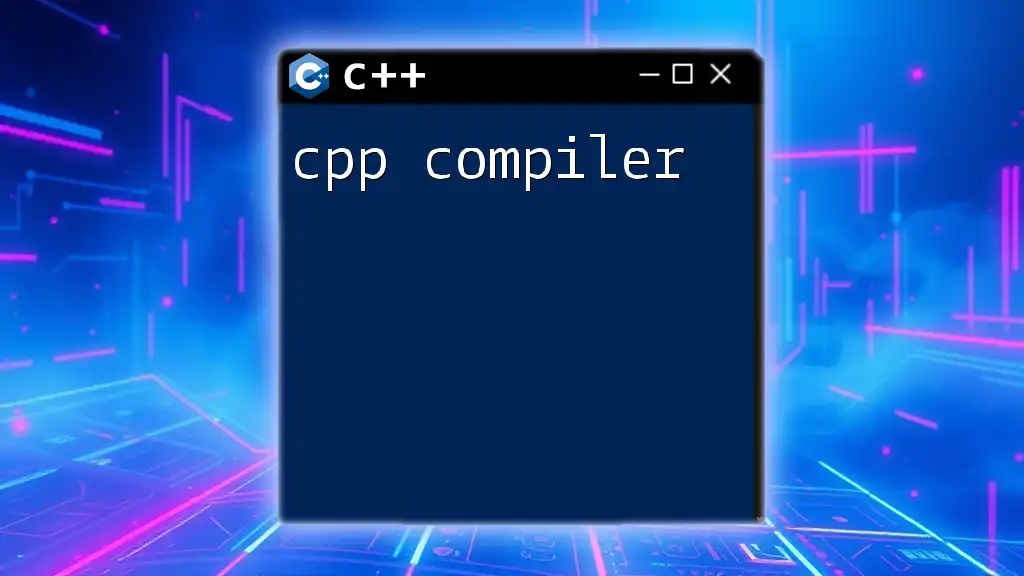
Exporting Telemetry Data
Available Exporters
For the telemetry data captured through OpenTelemetry, you need to have an effective way of exporting and visualizing this data. OpenTelemetry supports various exporters, such as:
- Logging Exporter: Outputs telemetry data to standard logs for debugging.
- OTLP Exporter: Provides a protocol (OpenTelemetry Protocol) for exporting telemetry data to backend systems.
- Prometheus Exporter: Enables metrics to be collected by Prometheus for visualization.
Choosing an appropriate exporter depends on your system architecture and monitoring requirements.
Example: Setting Up a Jaeger Exporter
Jaeger is a prominent tool for distributed tracing. Let's walk through configuring the Jaeger exporter in your application.
Code Snippet: Configuring the exporter:
#include <opentelemetry/exporters/jaeger/jaeger_exporter.h>
// Create a Jaeger exporter
auto exporter = opentelemetry::exporters::jaeger::JaegerExporter::Create();
This snippet showcases how to instantiate a Jaeger exporter. Once configured, it will allow your application to send trace data to Jaeger, where you can analyze application performance.
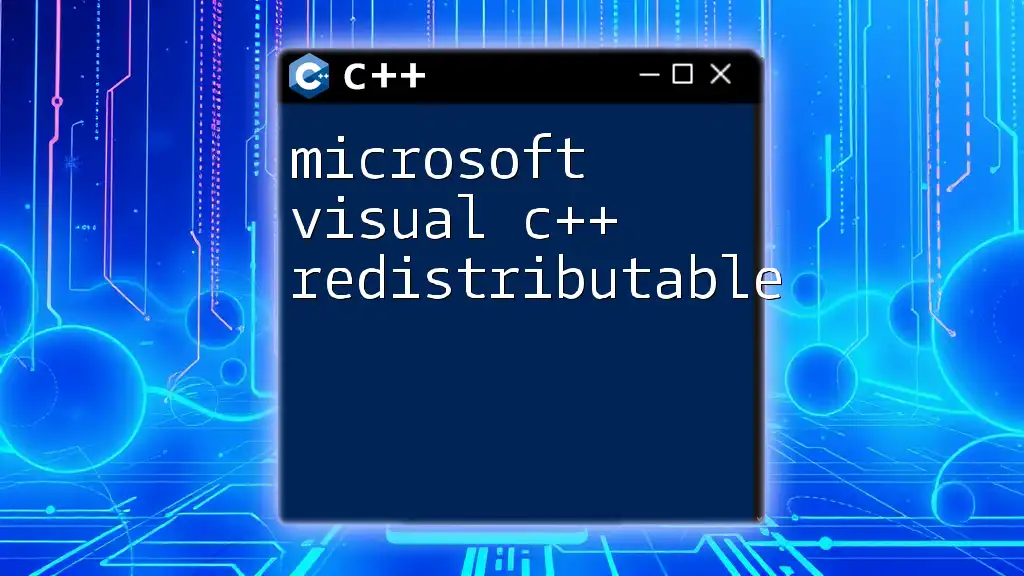
Monitoring and Visualizing Data
Integrating with Visualization Tools
For effective monitoring of your telemetry data, integrating with visualization tools is essential. Popular tools include:
- Grafana: Provides a sleek interface for visualizing metrics and logs.
- Prometheus: Collects and stores metrics data, enabling sophisticated queries and time-series analysis.
- Jaeger UI: Allows tracing analysis through interactive visualizations.
These tools can significantly enhance your ability to monitor and analyze application behaviors.
Example Visualization Setup
To visualize your metrics using Grafana, follow these steps:
- Install Grafana: Ensure Grafana is running and accessible.
- Connect Prometheus as a data source: Configure Prometheus as the data source in Grafana, enabling seamless access to metrics.
> By configuring these tools, you can visualize data and gain insights into your application's performance and behavior over time.
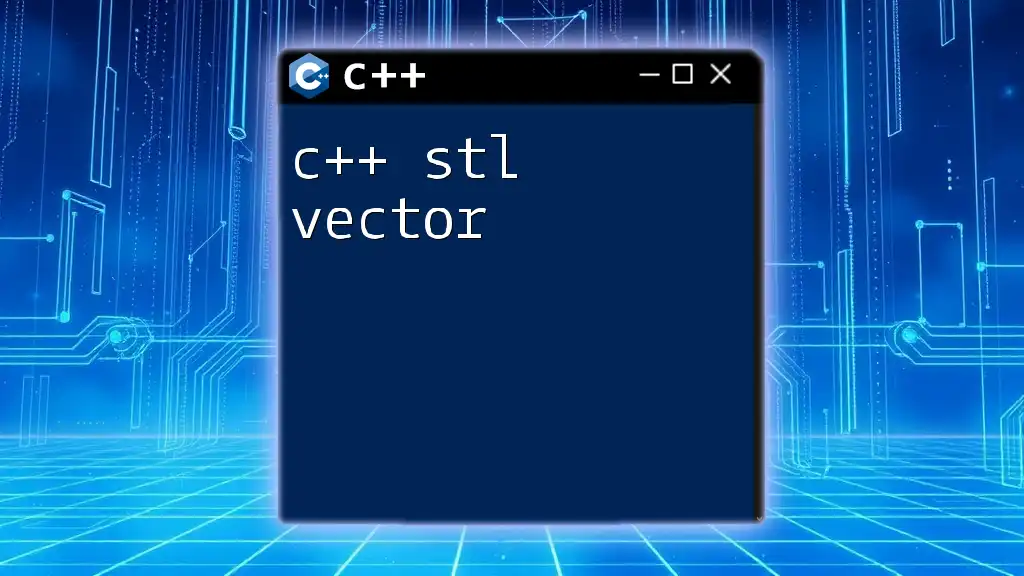
Troubleshooting Common Issues
Debugging Help
In your journey of implementing OpenTelemetry, you may run into some common issues, such as:
- Missing dependencies: Ensure all required libraries are installed.
- Failing builds: Investigate error messages to identify missing components.
Adopting best practices in logging and debugging your instrumentation code can streamline the troubleshooting process.
Community and Support Resources
The OpenTelemetry community is active and supportive. Utilize resources such as GitHub issues, community forums, and chat channels to ask questions or seek assistance. Engaging with the community can also foster collaboration and enhance your learning.
Consider contributing back to the open-source community by reporting bugs or improving documentation, as this benefits everyone involved.
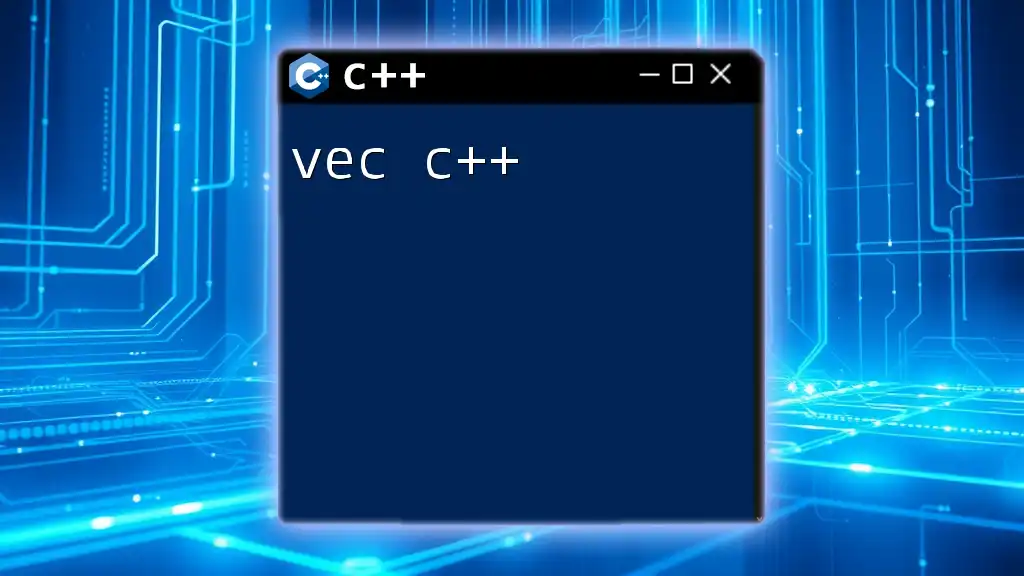
Conclusion
Summary of Benefits
Implementing OpenTelemetry in your C++ applications dramatically improves observability. By tracking telemetry data, you can optimize application performance, ensure reliability, and facilitate seamless debugging.
Call to Action
Now is the time to take action! Start implementing OpenTelemetry in your projects to unlock the full potential of observability. Leverage the provided resources and documentation to embark on this journey toward enhanced performance tracking and monitoring.
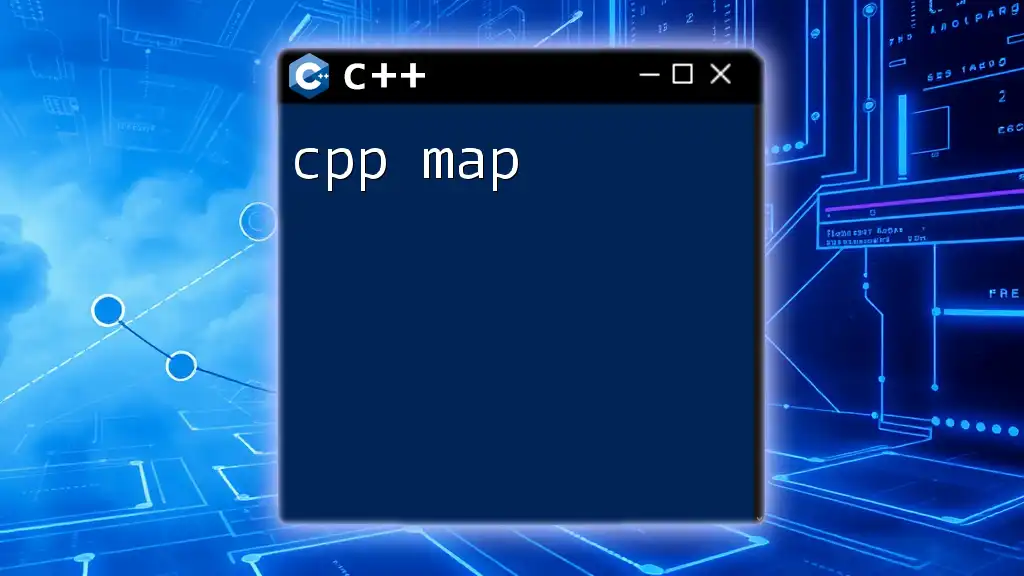
Additional Resources
References
- Official Documentation: [OpenTelemetry C++ GitHub](https://github.com/open-telemetry/opentelemetry-cpp) for further reading and examples.
- Explore other relevant libraries and tools that can complement your use of OpenTelemetry.
Community Links
Keep in touch with the OpenTelemetry community through GitHub discussions, forums, and contributing back to the project. Engaging with fellow developers is a great way to expand your knowledge and stay updated with the latest developments.
By leveraging OpenTelemetry with C++, you position yourself at the forefront of observability in modern application development.