Converting C++ (cpp) code to C involves simplifying C++ features such as classes, objects, and templates, as C does not support these constructs; instead, you can use structures and functions to achieve similar functionality.
Here’s an example of a simple C++ class converted to C:
// C++ Code
class Rectangle {
public:
int width, height;
Rectangle(int w, int h) : width(w), height(h) {}
int area() { return width * height; }
};
// Converted C Code
struct Rectangle {
int width, height;
};
void initRectangle(struct Rectangle* rect, int w, int h) {
rect->width = w;
rect->height = h;
}
int area(struct Rectangle* rect) {
return rect->width * rect->height;
}
Understanding C++ and C
What is C++?
C++ is an extension of the C programming language that introduces object-oriented programming (OOP) features. This allows developers to create complex systems by encapsulating data and operations within classes and objects.
Some notable features of C++ include:
- Classes and objects: Allow for data encapsulation and inheritance, which promotes code reuse and modularity.
- Polymorphism and inheritance: Enable developers to create dynamic and hierarchical relationships, improving code organization.
- Standard Template Library (STL): Offers a rich set of functions, algorithms, and data structures.
Common applications of C++ include game development, systems programming, and performance-critical applications, making it a popular choice for developers.
What is C?
C is a powerful, low-level programming language known for its efficiency and direct manipulation of system resources. It is primarily a procedural programming language, which focuses on writing procedures or functions to operate on data.
Key features include:
- Efficiency: C is designed to produce minimal overhead, making it suitable for system-level programming.
- Pointer arithmetic: Provides fine-grained control over memory, enhancing performance.
- Standard libraries: Offer basic functionalities, though not as extensive as those in C++.
Common applications of C include operating systems, embedded systems, and performance-intensive applications.

Key Differences Between C++ and C
Language Features
Object-Oriented vs. Procedural Programming: The core difference between C++ and C lies in their programming paradigms. While C++ focuses on objects and classes, C emphasizes sequences of function calls and structured programming. This affects how data is organized and manipulated in each language.
Memory Management: C++ incorporates advanced memory management through constructors, destructors, and operator overloading, while C relies on manual memory management with functions like `malloc()` and `free()`.
Standard Libraries: C++ benefits from the Standard Template Library, providing developers with pre-built data structures and algorithms. In contrast, C’s standard library offers only basic functionalities.
Syntax Differences
Classes vs. Structs
In C++, classes provide data protection through private and public access modifiers, whereas structs in C do not restrict access by default. This leads to the fundamental need to adapt C++ class constructs when converting to C.
For example, in C++:
class Example {
private:
int value;
public:
Example(int v) : value(v) {}
};
This can be converted to a struct in C as follows:
struct Example {
int value; // Access is public by default.
};
Function Overloading
C++ supports function overloading, allowing multiple functions with the same name but different parameter types. C does not support overloading, so this feature must be addressed during conversion.
For example, in C++:
void display(int value);
void display(double value);
In C, you would have to differentiate the functions by name:
void display_int(int value);
void display_double(double value);
Variable Declarations
C++ supports default initialization and complex declaration syntax that needs simplification when converting to C. For example:
int a = 10;
float b(3.14);
In C, the declaration remains simple:
int a = 10;
float b = 3.14f;

Converting C++ Code to C
Step-by-Step Approach to Conversion
Identify C++ Specific Features
Begin by identifying C++ features that do not exist in C—these often include classes, inheritance, function overloading, and templates. It is essential to note these before you start conversion to avoid complications later on.
Simplifying Object-Oriented Code
To convert C++ classes to C, change class definitions to struct and replace member functions with external functions that take the struct as an argument.
For example, in C++, a class with member functions may look like this:
class Circle {
private:
float radius;
public:
Circle(float r) : radius(r) {}
float area() { return 3.14 * radius * radius; }
};
In C, you will convert it as follows:
struct Circle {
float radius;
};
float area(struct Circle *circle) {
return 3.14 * circle->radius * circle->radius;
}
Handling Function Overloading
As explained, C does not allow function overloading. Therefore, you must rename functions to reflect their types or purposes, ensuring you create distinctly named functions.
Tools and Converters Available
C++ to C Converter Tools
Several online tools can assist in converting C++ code to C, such as `Cxx2C` or `C++ to C Converter`. These tools analyze C++ code and produce C equivalents, significantly speeding up the conversion process. However, the results may not always be perfect and usually require manual adjustments.
Manual vs. Automated Conversion
Manual Conversion: Ideal for small projects or when you need precise control over the code quality. It allows for optimizations and a better understanding of the underlying logic.
Automated Conversion: Suitable for large codebases where many files need to be converted quickly. Be aware that automated tools may not handle complex features well, necessitating a thorough review post-conversion.

Challenges and Considerations
Limitations of Converting C++ to C
Certain aspects of C++ cannot be directly translated into C:
- Inheritance: C does not support inheritance. You may need to create a flat structure or use composition.
- Templates: Templates in C++ cannot be converted; consider using macros in C instead.
Workarounds may include creating an interface in C through function pointers or manual typecasting.
Maintaining Code Quality
Best Practices: Focus on writing clear, maintainable C code. This includes using descriptive variable names, keeping functions short, and avoiding deep nesting of logic.
Testing: Thoroughly test the converted code to ensure it behaves as expected in both functionality and performance. Use unit tests whenever possible to validate the integrity of the conversion.
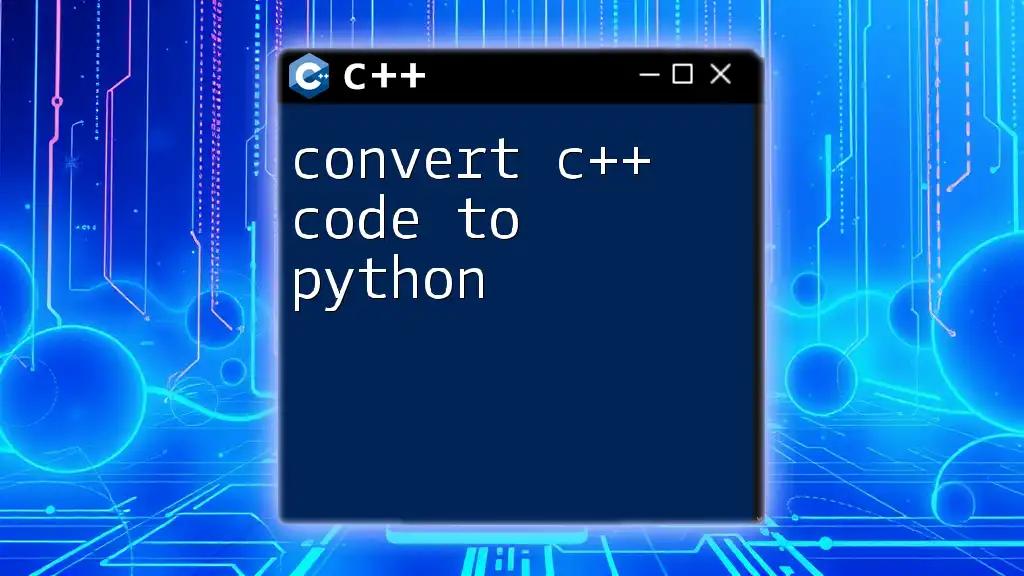
Conclusion
Understanding how to convert C++ to C is crucial for developers working with both languages. Familiarity with their differences and application of conversion strategies can vastly improve your coding efficiency. Practice converting small projects to refine your skills and apply the techniques discussed.
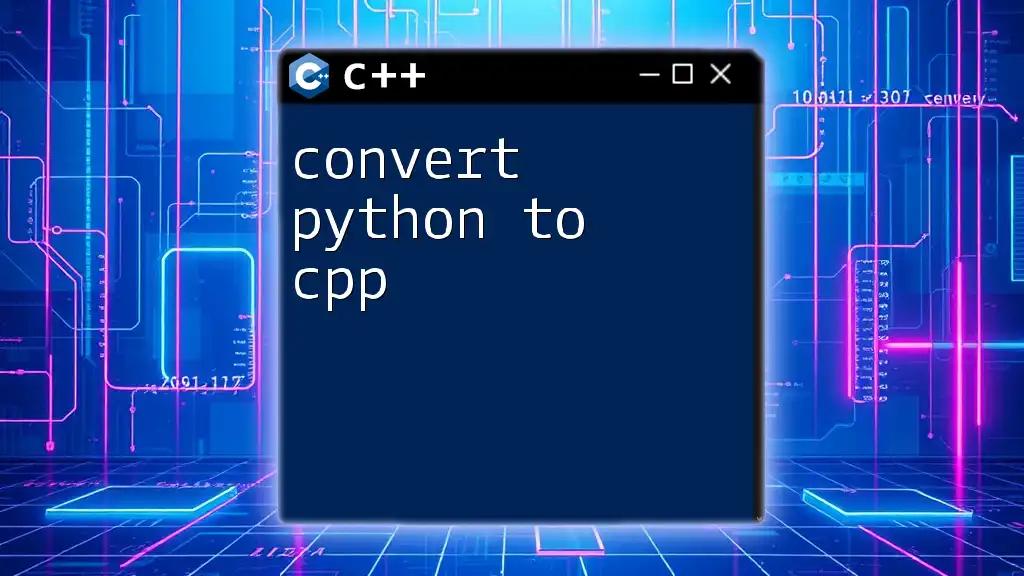
Additional Resources
Explore further through recommended books and online courses dedicated to C and C++. Online forums and communities can also be invaluable for seeking help during your conversion projects.

Final Thoughts
Converting code between languages can be challenging but rewarding. Share your experiences and learn from others in the community as you tackle projects involving C and C++. Stay curious and continue to enhance your programming skills!