Converting Python to C++ involves translating Python's dynamic syntax and built-in functionalities into C++'s statically typed language constructs, as illustrated in the following code snippet that demonstrates a simple addition function.
#include <iostream>
int main() {
int a = 5;
int b = 3;
int sum = a + b;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Why Convert Python to C++?
Performance Improvements
One of the most compelling reasons to convert Python to C++ is the significant performance boost that C++ can provide. C++ is a statically typed, compiled language, which allows it to execute code much faster than Python, an interpreted and dynamically typed language. In scenarios where execution speed is crucial—such as game development or real-time systems—the advantages of using C++ can make a notable difference.
Memory Management
C++ offers manual memory management, giving developers granular control over resource allocation and deallocation. This can lead to more efficient memory use, particularly in applications that handle large amounts of data or require complex data structures. However, it also necessitates a more careful approach to avoid memory leaks and mishandling of resources. In contrast, Python abstracts memory management through its garbage collector, which can introduce performance overhead in memory-intensive applications.
Integration with Existing C++ Codebases
Converting Python code to C++ can also provide a seamless way to integrate with existing C++ libraries or frameworks. This is particularly beneficial in contexts where standardized C++ libraries bring performance advantages and robust functionality. When you convert from Python to C++, you can leverage this power while maintaining important logic from your Python implementation.
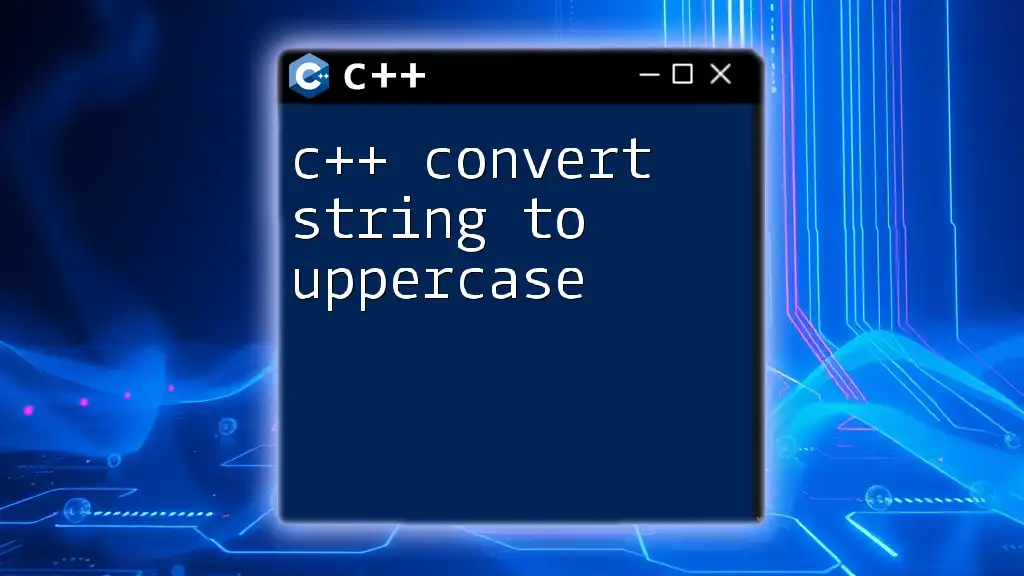
Understanding the Differences Between Python and C++
Syntax Differences
The syntax between Python and C++ differs significantly. Python utilizes indentation to define code blocks, creating a visually straightforward structure, while C++ uses braces to designate these blocks.
Example: A simple “Hello, World!” program in both languages:
Python:
print("Hello, World!")
C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Data Types and Structures
When converting data types, understanding the differences between Python's dynamic types (like lists, dictionaries, and strings) and C++'s static types (like arrays and vectors) is essential.
Code Snippet: Demonstrating list vs. array initialization:
Python:
my_list = [1, 2, 3, 4, 5]
C++:
#include <vector>
std::vector<int> my_array = {1, 2, 3, 4, 5};
Error Handling
Error handling is implemented quite differently in both languages. Python employs exceptions that can be caught with try and except blocks, while C++ uses try and catch statements.
Example: Error handling patterns:
Python:
try:
x = 1 / 0
except ZeroDivisionError:
print("Division by zero!")
C++:
#include <iostream>
int main() {
try {
throw std::runtime_error("Division by zero!");
} catch (std::exception &e) {
std::cout << e.what() << std::endl;
}
return 0;
}
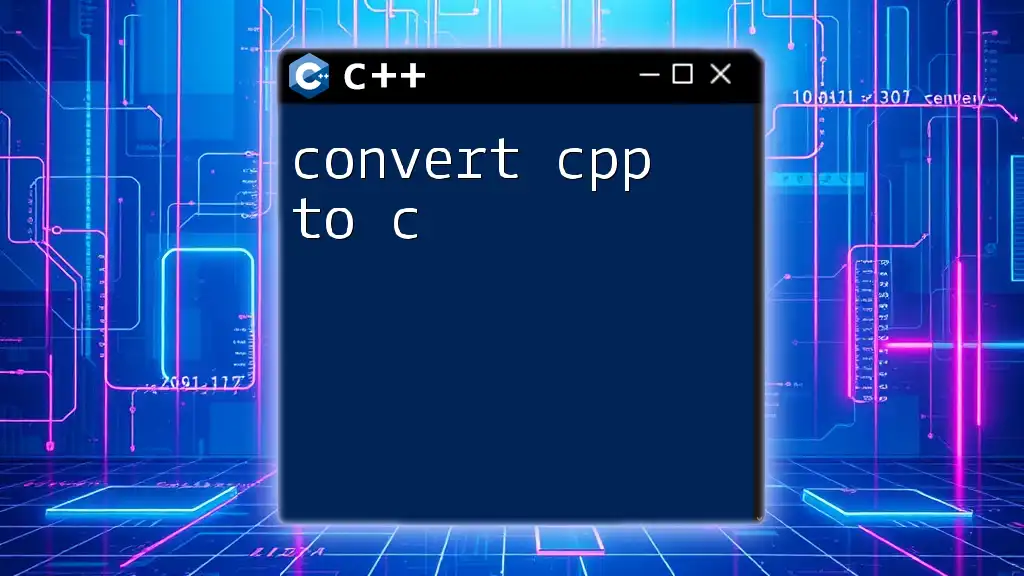
Tools for Converting Python to C++
Python C++ Converter
Several tools can assist in converting Python scripts into C++. A dedicated Python C++ converter can automate some aspects of this process, making it easier for developers to shift languages without redoing significant chunks of code from scratch. However, it is crucial to review the output since automatic conversions may not always yield optimal or error-free C++ code.
Manual Conversion Techniques
While tools can help, manual conversion is often necessary to achieve the best results. When converting code, take a systematic approach by breaking down Python scripts into simpler components, focusing on common code constructs like loops and conditional statements.
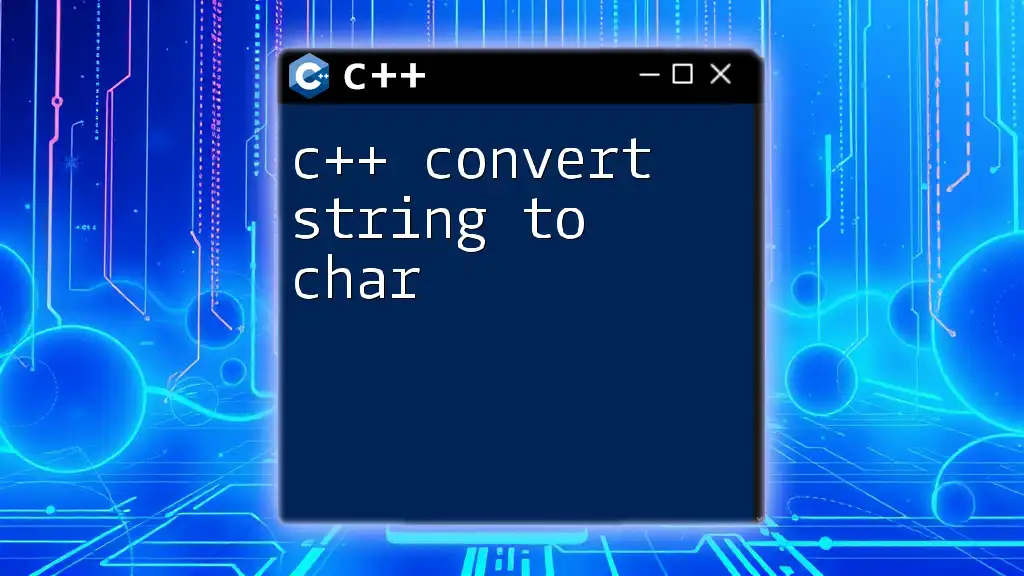
Step-by-Step Guide to Converting Python Code to C++
Analyzing Your Python Script
Begin by analyzing your Python code. Look for key areas of complexity like loops, conditionals, and function calls. This will give you insight into how you can approach the conversion to C++.
Example Python Script to Analyze:
def sum_of_squares(n):
return sum(i * i for i in range(n))
result = sum_of_squares(5)
print(result)
Translating Code Constructs
When translating common constructs, be sure to adjust for differences in indentation and syntax.
Code Snippet: Conversion of a simple conditional statement:
Python:
if age < 18:
print("Minor")
else:
print("Adult")
C++:
if (age < 18) {
std::cout << "Minor" << std::endl;
} else {
std::cout << "Adult" << std::endl;
}
Handling Functions and Methods
Python's functions can easily be translated into C++ methods. Pay close attention to return types in C++.
Code Snippet: A function example in both languages:
Python:
def multiply(a, b):
return a * b
C++:
int multiply(int a, int b) {
return a * b;
}
Classes and Object-Oriented Programming
Both Python and C++ support object-oriented programming, but the class structures differ.
Example: Class structure comparison:
Python:
class Dog:
def bark(self):
print("Woof!")
C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Using Libraries and Frameworks
Consider how to handle dependencies. Popular Python libraries like NumPy may not have direct C++ equivalents, but other libraries can provide similar functionality.
Comparison Chart: Useful Libraries in Both Languages:
- NumPy (Python) ↔ Eigen (C++)
- Pandas (Python) ↔ Poco C++ Libraries (C++)
Memory Management in C++
Pay special attention to memory management. Use pointers and references to handle dynamic memory effectively. While C++ provides more control, it also requires more care.
Code Example: Basic pointer usage in C++:
int* ptr = new int(5);
// Use the pointer...
delete ptr; // Don't forget to free the memory
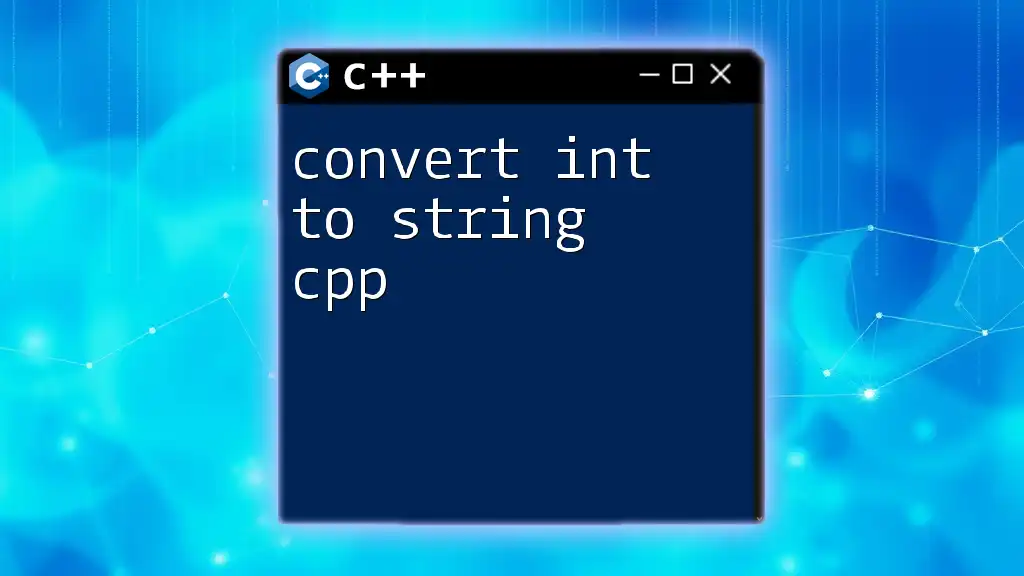
Common Pitfalls and How to Avoid Them
Type Mismatches
One common issue when converting Python to C++ is type mismatches, particularly with collections and numeric values. C++ is strict with data types, so be careful to define your types explicitly to avoid runtime errors.
Examples: How to handle type conversions correctly: In Python, you might use:
my_number = "7"
converted_number = int(my_number)
In C++, you'll want to ensure you are working with the correct types from the start.
Managing External Libraries
Identifying and managing dependencies becomes crucial during conversion. Ensure that you take the time to match Python libraries with their C++ counterparts when necessary.
Debugging Converted Code
Debugging is an essential aspect of the conversion process. When converting code, consider using tools like gdb (GNU Debugger) or the integrated debuggers available in many C++ IDEs.
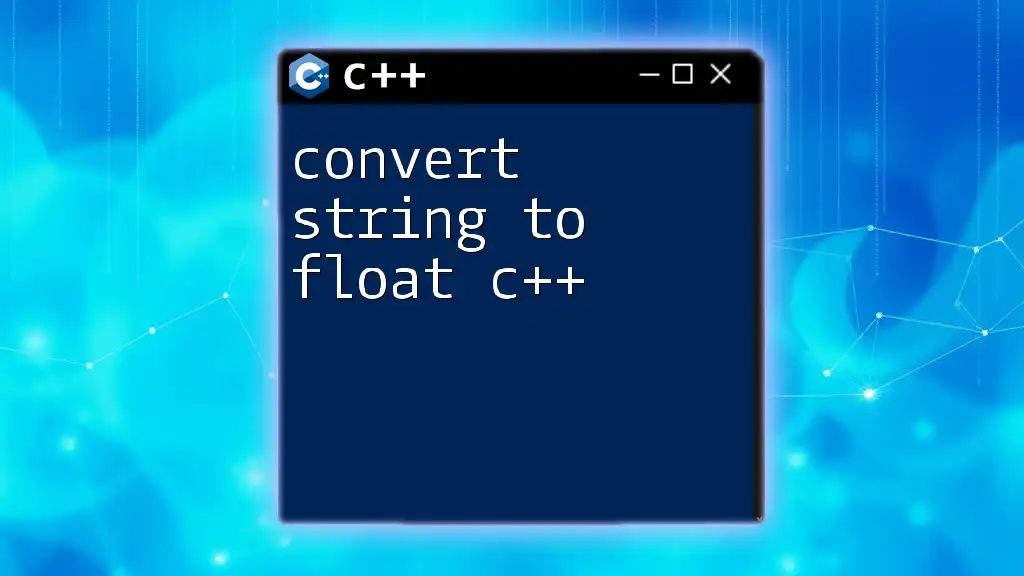
Best Practices for Efficient Conversion
Write Clean, Maintainable Code
One hallmark of good programming is writing clean code. Before conversion, ensure that your Python code is refactored and understandable. This will make the transition to C++ smoother.
Examples: Refactoring Python code before conversion could include breaking down complex functions and removing redundancies.
Testing Your C++ Code
After converting Python to C++, thoroughly test your C++ code. Employ unit testing frameworks such as Google Test or Catch2 to ensure functionality matches your expectations and to catch any errors early in the process.
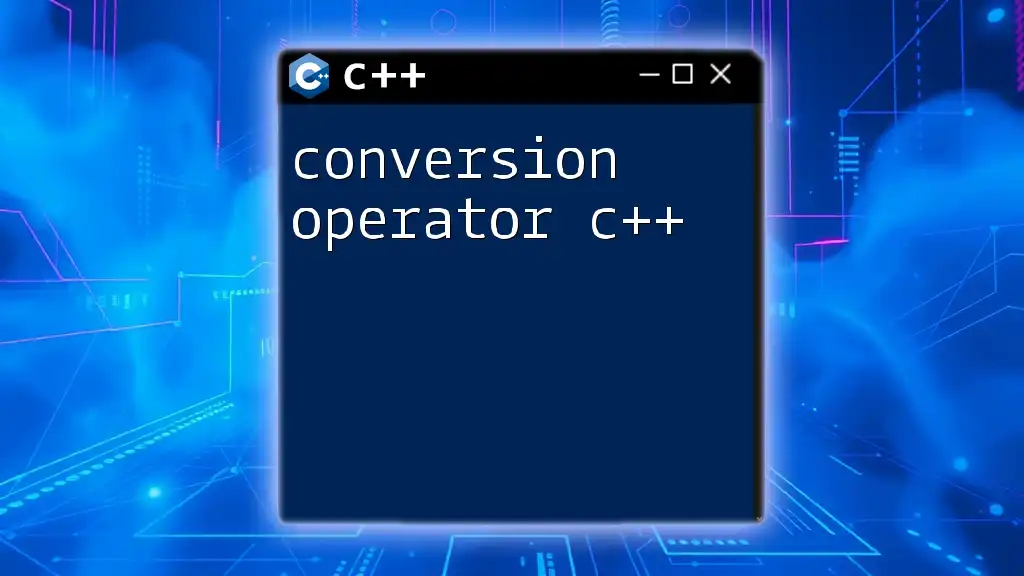
Conclusion
Converting Python to C++ is not merely a technical exercise; it’s an opportunity to leverage the strengths of both languages. With careful analysis, attention to detail, and an understanding of syntax differences, you can effectively transition your code. Whether for performance, memory management, or integration, mastering this conversion enhances your skill set as a developer. Embrace the challenge, practice with simple scripts, and feel free to explore the extensive resources available in the programming community!