Converting C++ code to Python involves translating the syntax and constructs of C++ into Python's simpler, more readable format, often resulting in fewer lines of code.
Here's an example of converting a simple C++ program that calculates the sum of two numbers into Python:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
cout << "Sum: " << (a + b) << endl;
return 0;
}
Converted to Python:
a, b = map(int, input("Enter two numbers: ").split())
print("Sum:", a + b)
Understanding C++ and Python Differences
Syntax Differences
When you convert C++ code to Python, the first aspect that becomes evident is the difference in syntax. C++ is a statically typed language, meaning you must declare the type of variables explicitly. In contrast, Python uses dynamic typing, allowing you to assign values to variables without declaring their types.
Example of Variable Declaration:
C++ code:
int number = 10;
Python code:
number = 10
In the example above, the C++ code explicitly states that `number` is of type `int`, while in Python, the type is inferred automatically.
Memory Management
Another significant difference involves memory management. C++ gives developers control over memory allocations through pointers, whereas Python employs references and garbage collection for memory management.
In C++, you might see pointers like this:
int* ptr = new int(5);
In Python, referencing is more straightforward:
number = 5
With pointers in C++, managing memory can lead to complexities such as memory leaks and dangling pointers. Python mitigates these issues through automatic garbage collection, making programming easier and less error-prone.
Object-Oriented Features
Both C++ and Python support object-oriented programming (OOP), but they do so in slightly different ways. C++ utilizes encapsulation, inheritance, and polymorphism through class declarations, which involve multiple syntax rules. Python also supports these OOP principles but has a simpler and more readable syntax.
Example of Class Definition:
C++ class:
class Animal {
public:
void sound() {
cout << "Generic animal sound";
}
};
Python class:
class Animal:
def sound(self):
print("Generic animal sound")
In this example, you can see that the Python class definition is less verbose and easier to read.
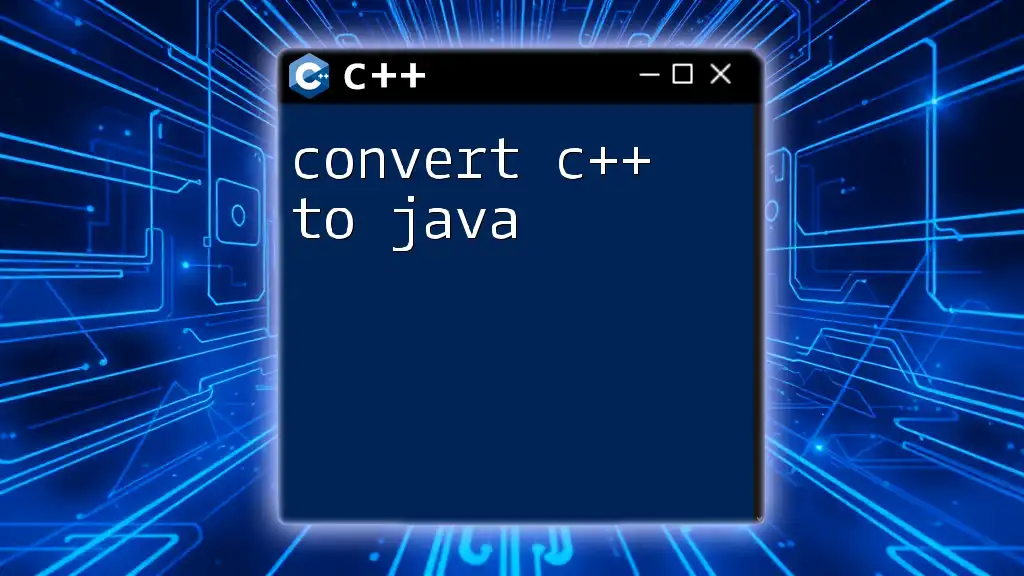
Tools for Converting C++ to Python
Using C++ to Python Converters
Before diving into manual coding techniques, consider utilizing automated tools designed to convert C++ to Python. Some of the popular converters include Cython, Pybind11, and SWIG. These tools can significantly speed up the conversion process while maintaining a good level of accuracy.
Pros and Cons of Using Automated Converters
Benefits:
- Speed: Automated converters can turn your code into Python almost instantly.
- Ease of Use: Most converters require minimal setup and can handle simple translations without complicated configurations.
Drawbacks:
- Possible Inaccuracies: Automated converters might miss nuances or complexities in your C++ code, necessitating further manual adjustments.
- Limited Customization: Many converters do not allow for extensive adjustments, which can be a downside for more complex applications.
Step-by-Step Guide to Using a C++ to Python Converter
To effectively use a C++ to Python converter, follow these steps:
- Choose a converter based on your needs.
- Input your C++ code into the converter.
- Run the converter and review the generated Python code.
- Manually tweak generated code, paying particular attention to logical correctness and performance.
Example Conversion:
Here’s a simple example using a hypothetical converter:
C++ code:
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, World!" << endl;
}
Generated Python code:
def greet():
print("Hello, World!")
Though the automated converter produces valid Python code, you should ensure that the logic aligns with your expectations.
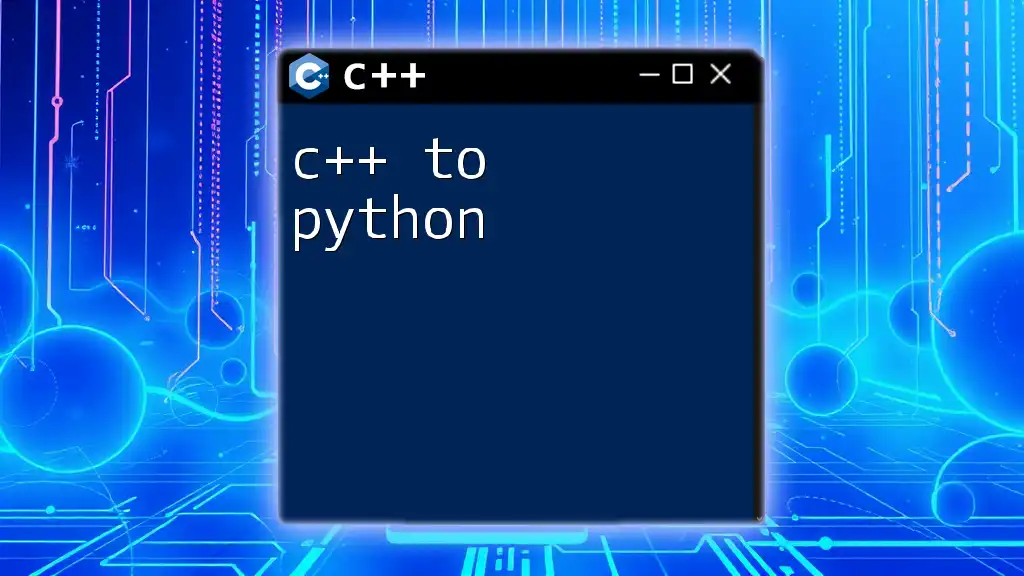
Manual Conversion Techniques
Understanding the Logic of the C++ Code
Before attempting to convert C++ code to Python, it's essential to fully understand the logic of the original C++ code. Spend time analyzing the code’s structure, especially focusing on functions, loops, and data flow.
Sample C++ Program:
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b;
}
int main() {
cout << add(5, 3);
return 0;
}
This C++ code defines a simple `add` function and it prints the result of adding two numbers.
Translating C++ Constructs to Python
When translating C++ constructs to Python, focus on the following aspects:
- Data Types: Python has more flexible data types. For instance, C++ arrays could be converted to Python lists.
- Control Structures: Control flow in C++ often translates directly to Python, but syntax will differ.
Example of A Control Statement:
C++ if statement:
if (number > 0) {
cout << "Positive";
}
Python equivalent:
if number > 0:
print("Positive")
Example: Converting C++ Functions to Python
When converting functions, maintain the logical flow while adapting the syntax.
C++ Function:
int multiply(int a, int b) {
return a * b;
}
Python Function:
def multiply(a, b):
return a * b
The translation retains the logic of multiplying two numbers while changing the syntax accordingly.
Handling C++ Specific Features
C++ offers features like the Standard Template Library (STL), which may not have direct counterparts in Python. For example, C++ vectors can be replaced with lists in Python.
C++ STL Usage:
#include <vector>
vector<int> numbers = {1, 2, 3};
Python Equivalent:
numbers = [1, 2, 3]
Understanding these fundamental differences is critical to a successful conversion.
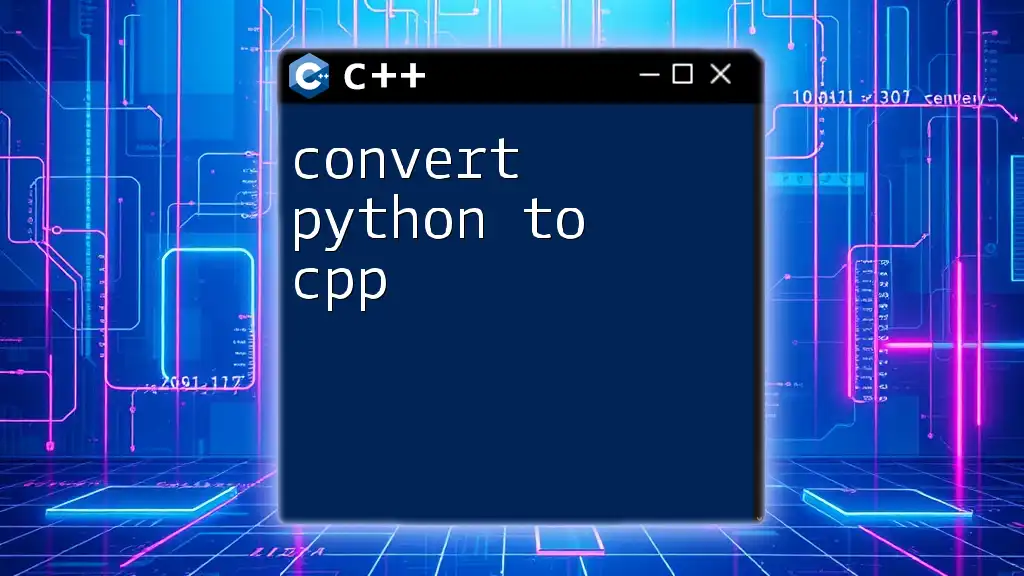
Challenges during Conversion
Common Pitfalls and How to Avoid Them
While convert C++ code to Python, you may encounter challenges. Frequent pitfalls include:
- Mismanaging Data Types: Forgetting that Python is dynamically typed can lead to errors in logic.
- Inefficient conversions: Sometimes the automated tools generate inefficient code, which can lead to performance issues.
Debugging Converted Code
After conversion, debugging is crucial. Use Python's built-in debugging tools like `pdb` or IDE features to pinpoint issues. Testing each part of the converted code thoroughly is vital to ensure accuracy.
Performance Considerations
After conversion, understanding speed and complexity differences is essential. C++ is generally faster due to compiled nature, while Python provides ease of use but can lead to slower performance. Benchmark your code to identify bottlenecks and optimize as necessary.
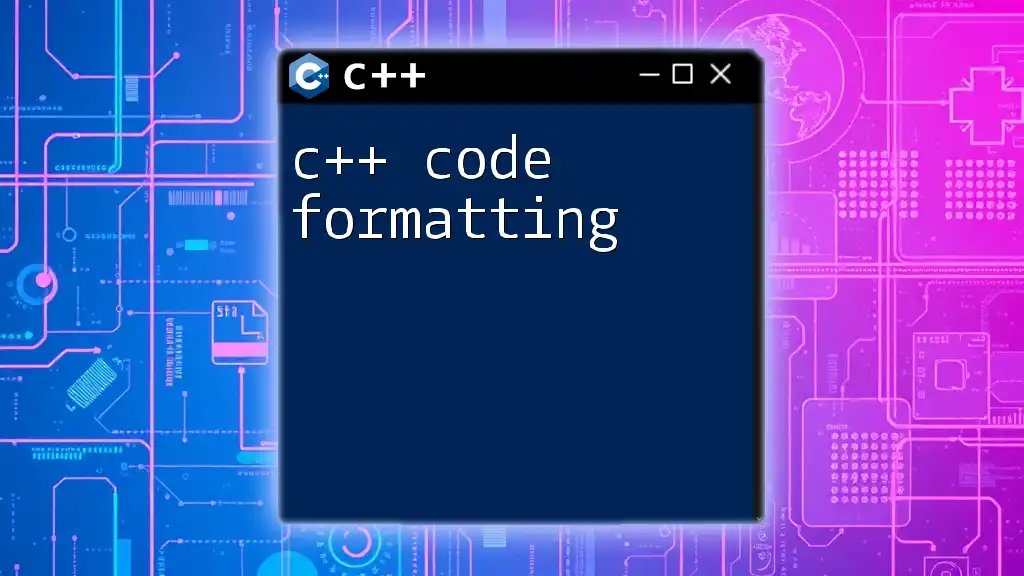
Conclusion
Converting C++ code to Python can be a meticulous process requiring an understanding of both languages' intricacies. While automated tools can simplify part of this transition, a strong grasp of the logic and constructs used in C++ is essential. By following systematic approaches and manually refining the code, you can ensure that the conversion maintains functionality and performance.
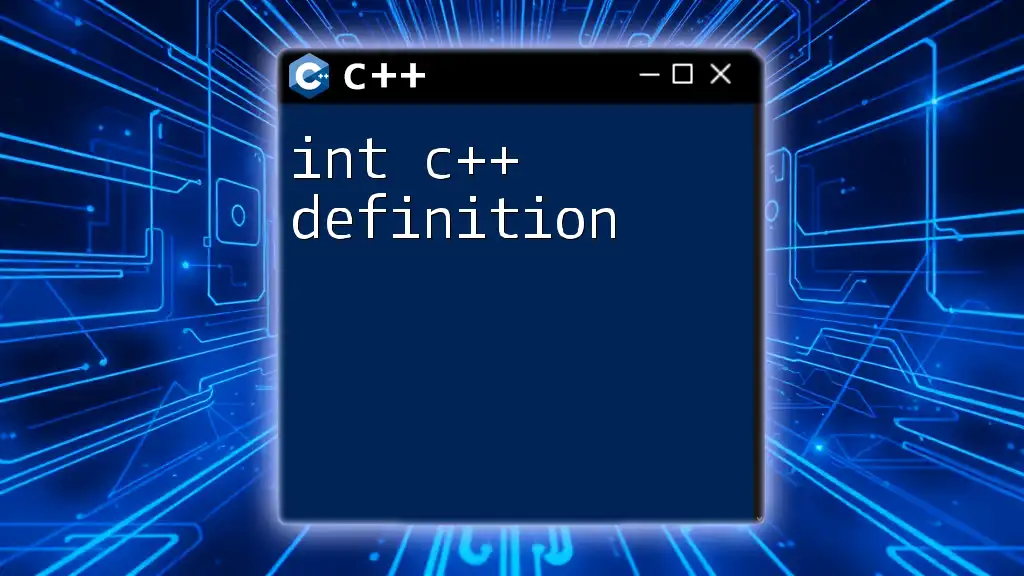
Call to Action
To truly master the art of conversion, we encourage you to practice by converting different snippets of C++ code to Python. The more you explore this process, the better you will become in navigating the differences between these two powerful programming languages.
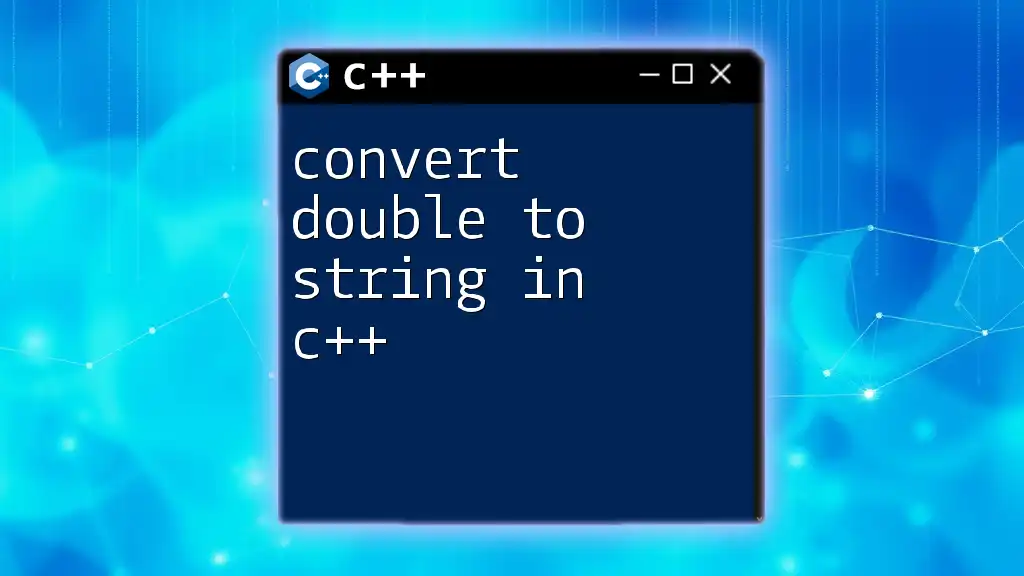
Additional Resources
For further assistance, check out some online C++ to Python converters, and don’t forget to look into books and tutorials dedicated to enhancing your skills in both languages.