In C++, you can convert a double to a string using the `std::to_string` function as shown in the following code snippet:
#include <iostream>
#include <string>
int main() {
double num = 3.14159;
std::string strNum = std::to_string(num);
std::cout << strNum << std::endl; // Output: 3.141590
return 0;
}
Understanding the Double Data Type in C++
The `double` data type in C++ is a widely used primitive type that represents floating-point numbers with double precision, allowing for increased accuracy in mathematical calculations. It is typically 64 bits in size, which grants it the ability to handle very large or very small numbers along with fractional values. Proprietarily, `double` can represent values ranging from approximately 2.2E-308 to 1.8E+308.
Despite its advantages, it’s important to recognize limitations associated with `double`. One major concern is precision issues. As floating-point numbers cannot always represent decimal fractions accurately, operations involving doubles can lead to rounding errors. Understanding these limitations is key to effectively utilizing `double` in your applications.
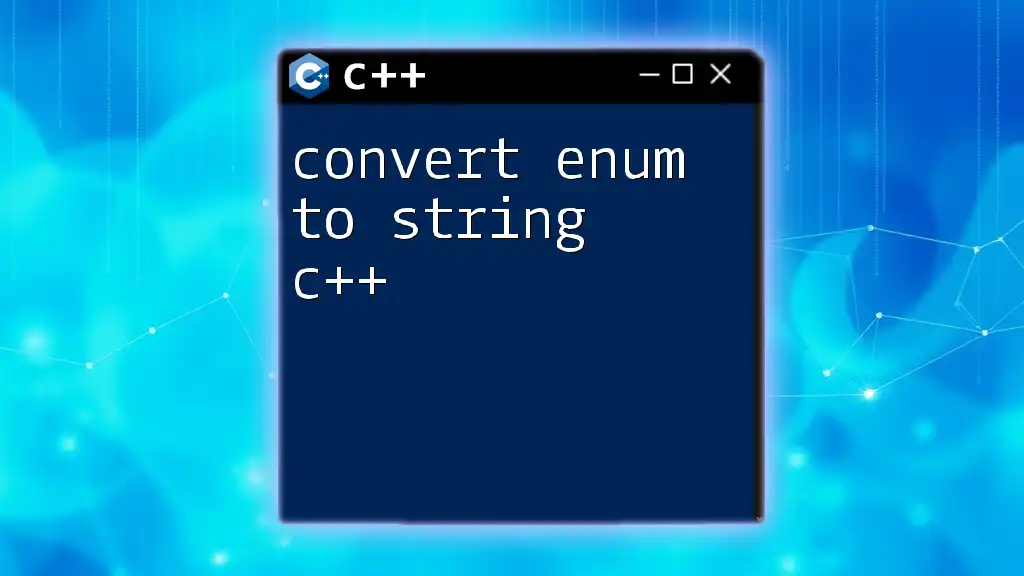
Why Convert Double to String in C++
Converting `double` to `string` in C++ is vital in numerous programming scenarios. Understanding when this conversion is necessary can greatly enhance your software functionality.
When is Conversion Necessary?
- Displaying Numbers: Most user interfaces require values to be displayed as strings. Whether it's showing results in a console or graphical interface, conversion is a must.
- Logging and Debugging: To capture numerical values in logs for troubleshooting or debugging, they need to be converted to strings.
- File I/O Operations: When writing numerical data to files (like CSVs or text files), it is often easier to manipulate them as strings.
Common Scenarios
Conversion can arise in a variety of common instances, such as:
- User Input/Output: Taking numerical input from users usually involves displaying prompts that utilize strings.
- Storing Data: If you need to store numerical values in databases or configurations, converting to strings is essential.
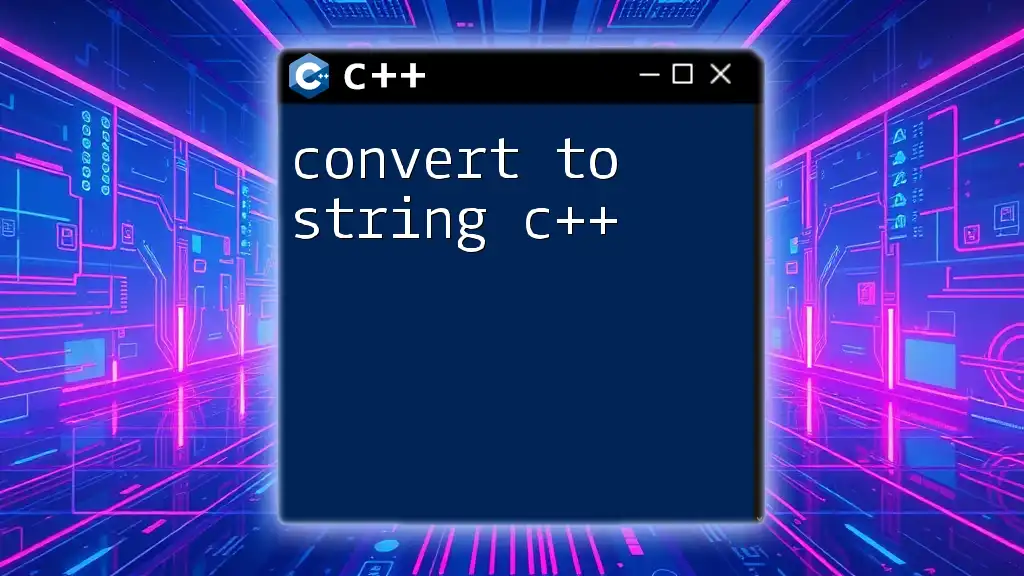
Methods to Convert Double to String in C++
Using the `std::to_string` Function
The `std::to_string` function is a part of the C++ Standard Library and provides a straightforward way to convert a `double` to a `string`.
Description of the Function This function has the signature `std::string to_string(double value)` and converts the provided double to its string representation.
Syntax and Example Code Here’s a basic example demonstrating how to use `std::to_string`:
double num = 3.14159;
std::string strNum = std::to_string(num);
In this code snippet, `num` is a double representing the mathematical constant pi, which is then converted to a string and stored in `strNum`.
Precision Control By default, `std::to_string` provides 6 decimal places of precision. If specific formatting is required, this method might not always meet your needs. To control output precision, consider alternatives.
Using `std::ostringstream`
Another powerful method for converting `double` to `string` is using the `std::ostringstream` class, which is part of the `<sstream>` library.
What is `std::ostringstream`? This class facilitates the use of string streams, which are versatile and allow for formatted output similar to standard C++ output operations.
Syntax and Example Code Here’s how you can use `std::ostringstream`:
#include <sstream>
double num = 3.14159;
std::ostringstream stream;
stream << num;
std::string strNum = stream.str();
In this example, we first create an `ostringstream` object called `stream`, then use the `<<` operator to convert the double to a string. The resultant string can be retrieved using `stream.str()`.
Custom Formatting Options This method allows you to use manipulators for custom formatting. For instance, if you want to display a precise number of digits, you can use:
#include <sstream>
#include <iomanip> // For std::setprecision
double num = 3.14159;
std::ostringstream stream;
stream << std::fixed << std::setprecision(2) << num;
std::string strNum = stream.str();
This way, you ensure that your output has exactly two decimal places.
Using `sprintf` and `snprintf`
Using `sprintf` (and its safer alternative, `snprintf`) is another viable option for converting `double` to `string`.
Overview of `sprintf` Functions These functions offer formatted output and allow for more control over the format of the resulting string.
Syntax and Example Code Here’s a basic example using `sprintf`:
char buffer[50];
double num = 3.14159;
sprintf(buffer, "%.2f", num);
std::string strNum(buffer);
In this code snippet, we create a buffer character array, format the double to two decimal places, and copy the output string into `strNum`.
Pros and Cons While `sprintf` and `snprintf` can be powerful, they come with caveats. Using `sprintf` without ensuring buffer size can lead to buffer overflow risks. Thus, employing `snprintf` is a safer choice as it limits the number of characters written to the buffer, reducing risk.
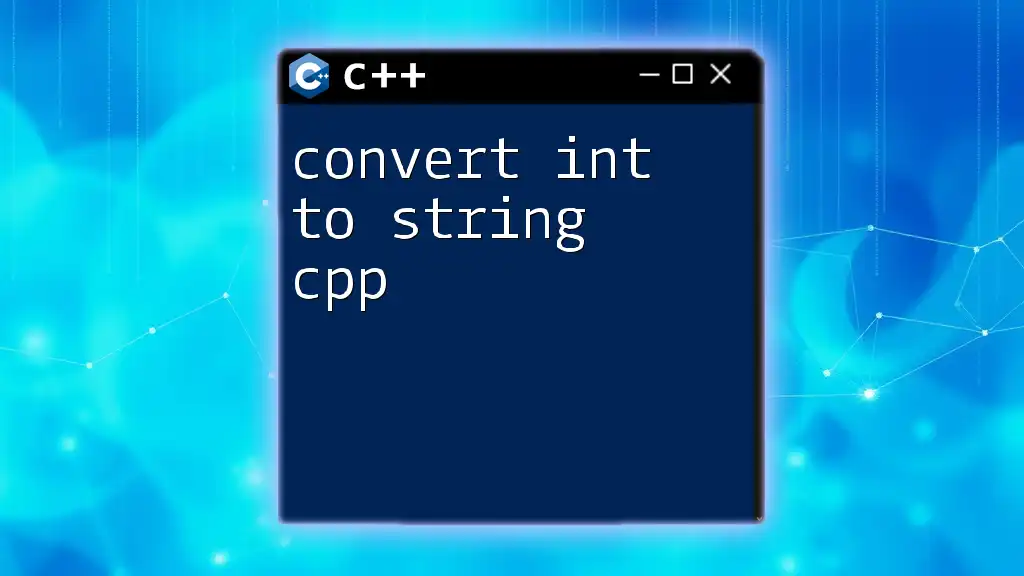
Best Practices for Converting Double to String
Choosing the Right Method
Deciding on which method to use depends on your specific programming context. For quick conversions without special formatting, `std::to_string` is the easiest. However, for more structured output, `std::ostringstream` or `sprintf` may be more suitable.
Managing Precision
When converting `double` to `string`, how you manage precision is critical. Be conscious of how you wish to format your output, and ensure the method you select meets your requirements for precision.
Error Handling
Always consider potential error handling scenarios, especially when dealing with conversion functions that require specific formatting.
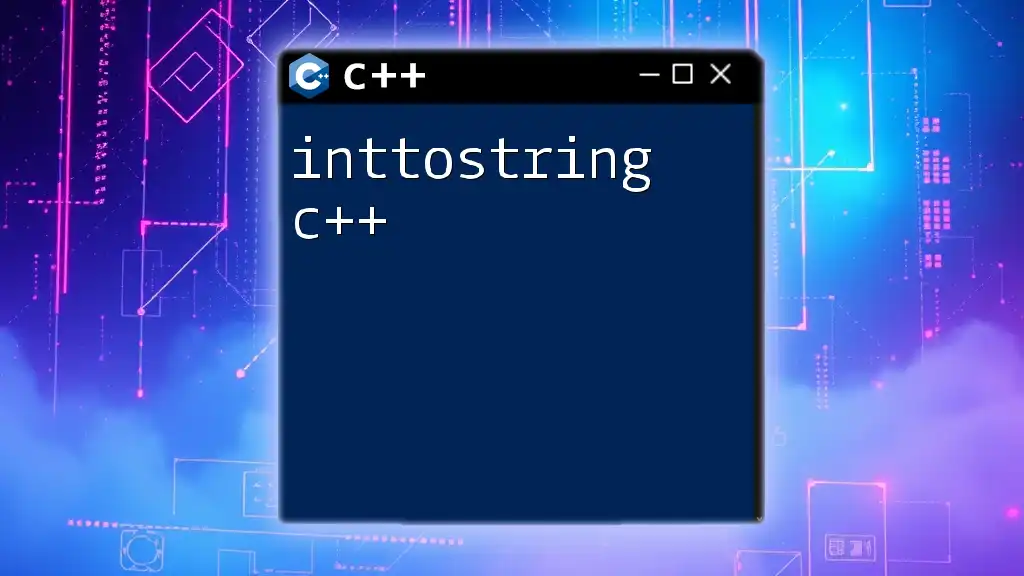
Conclusion
Understanding how to convert `double` to `string` in C++ is crucial for effective programming, allowing you to present numerical data accurately and efficiently. By exploring methods such as `std::to_string`, `std::ostringstream`, and `sprintf`, you can choose the correct tool for your specific needs, ensuring that your applications handle data type conversions smoothly.
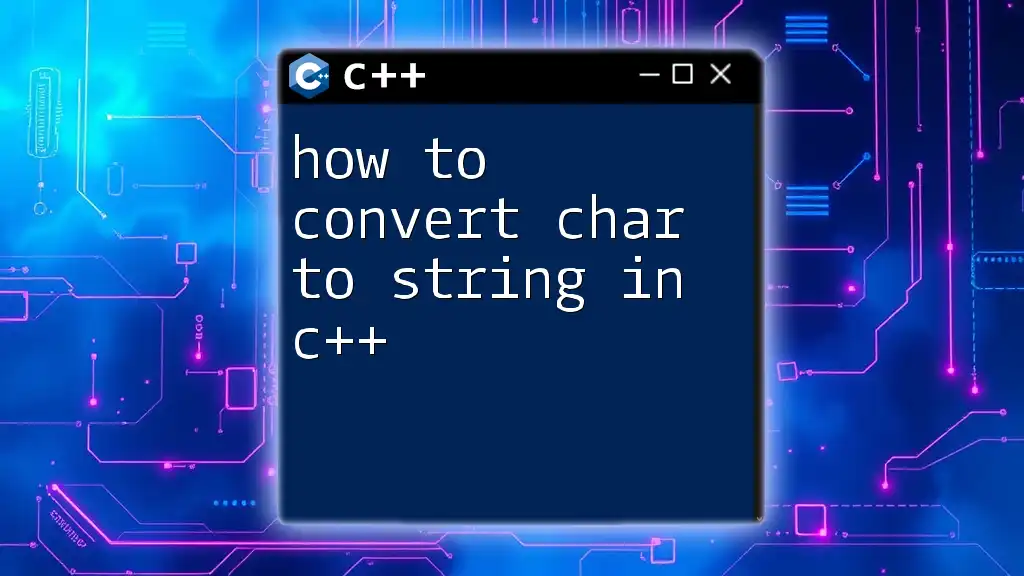
Frequently Asked Questions (FAQs)
Can I convert a string back to double in C++?
Yes, you can use `std::stod` for converting a `string` back to a `double`.
What happens to precision when converting double to string?
The precision may be altered based on the method used for conversion. For instance, `std::to_string` generally provides 6 decimal places by default.
Which method is fastest for large-scale conversions?
Performance can vary based on context. For high-speed operations, avoid stream methods; simply using `std::to_string` may suffice for most needs.
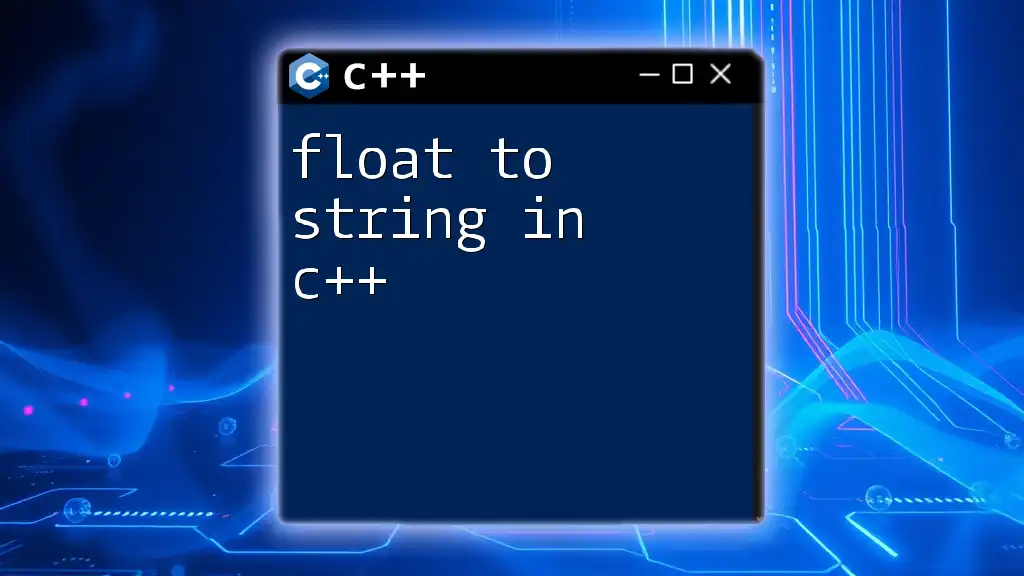
Further Reading/Resources
To deepen your understanding, consider visiting references like [cppreference.com](https://en.cppreference.com/) or exploring C++ programming books that extensively cover data type conversions.