In C++, you can compare two strings using the `==` operator to check for equality or the `compare()` method for more detailed comparisons.
Here's a code snippet to illustrate both methods:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
// Using == operator
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
// Using compare() method
if (str1.compare(str2) == 0) {
std::cout << "Strings are equal (compare method)." << std::endl;
} else {
std::cout << "Strings are not equal (compare method)." << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters that can represent text. The C++ Standard Library offers a `std::string` class which provides many convenient functionalities for string manipulation. It is important to distinguish between C-style strings (character arrays terminated by a null character) and C++ strings, which are objects that handle memory allocation and deallocation automatically.
Including the Necessary Libraries
To work with `std::string`, you must include the string header at the beginning of your program:
#include <string>
This library provides the necessary functions and definitions to use strings effectively in C++.
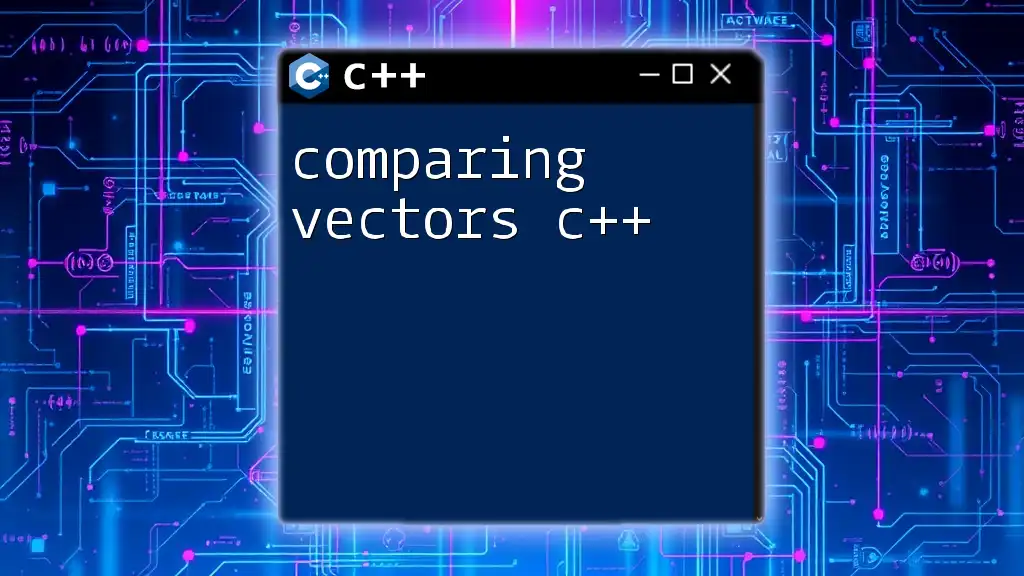
Basic String Comparison
Using the Equality Operator
The simplest way to compare two strings is by using the equality operator (`==`). This operator checks whether the strings are identical character by character.
Example: Comparing two strings for equality
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "Hello";
if (str1 == str2) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
return 0;
}
In this example, `str1` and `str2` are identical, so the output will confirm their equality.
Using the Relational Operators
C++ also allows the use of relational operators (`<`, `>`, `<=`, `>=`) to compare strings lexicographically. The comparison is based on the ASCII values of the characters.
Example: Lexicographical comparison of strings
#include <iostream>
#include <string>
int main() {
std::string str1 = "Apple";
std::string str2 = "Banana";
if (str1 < str2) {
std::cout << str1 << " is less than " << str2 << std::endl;
}
return 0;
}
In this case, `str1` is lexicographically less than `str2`, as "Apple" comes before "Banana".
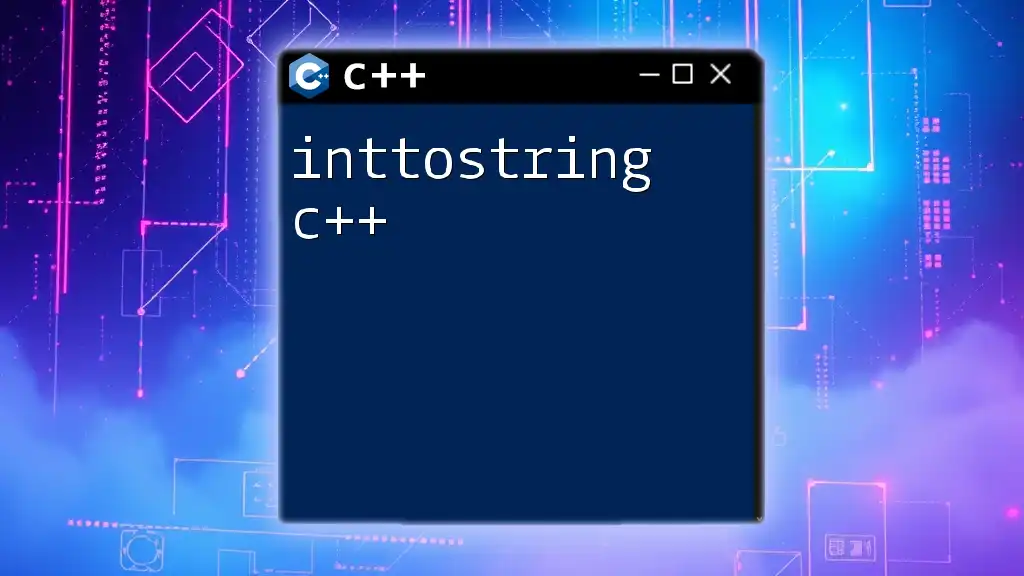
Case Sensitivity in String Comparison
Understanding Case Sensitivity
When comparing strings in C++, the comparison is case-sensitive, which means "hello" and "Hello" will not be considered equal. This can lead to unexpected results in string comparisons, especially when user input is involved.
Using `std::transform` for Case Insensitivity
If you want to perform a case-insensitive comparison, you can use the `std::transform` function to convert both strings to the same case before comparing them.
Example: Case-insensitive string comparison
#include <iostream>
#include <string>
#include <algorithm>
bool caseInsensitiveCompare(const std::string &str1, const std::string &str2) {
std::string a = str1, b = str2;
std::transform(a.begin(), a.end(), a.begin(), ::tolower);
std::transform(b.begin(), b.end(), b.begin(), ::tolower);
return a == b;
}
int main() {
std::string str1 = "Hello";
std::string str2 = "hello";
if (caseInsensitiveCompare(str1, str2)) {
std::cout << "Strings are equal (case insensitive)!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
return 0;
}
In this example, the function `caseInsensitiveCompare` ensures that both strings are compared in a case-insensitive manner.
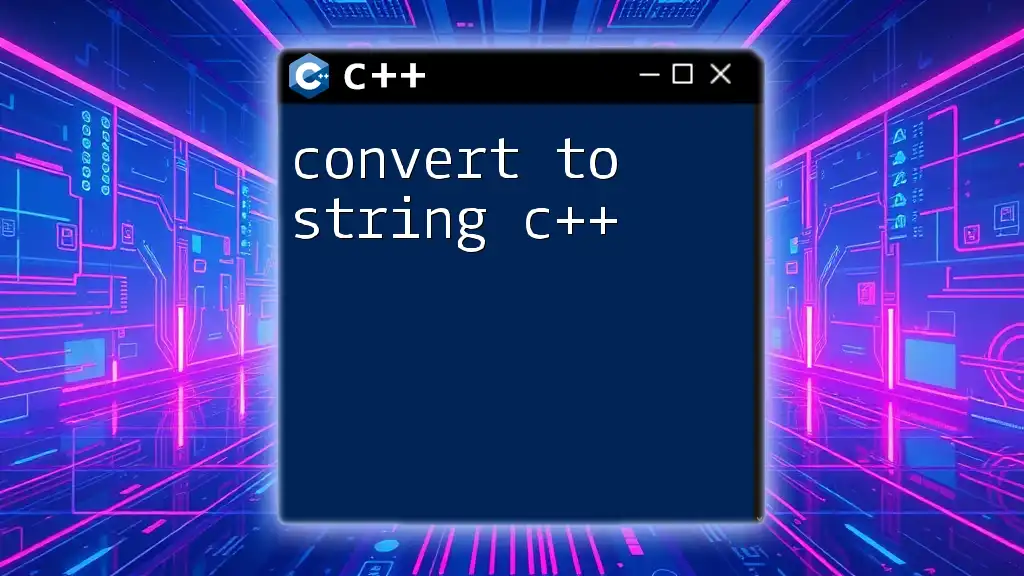
Comparing Substrings
Extracting Substrings
To compare part of a string, you can use the `substr()` method, which allows you to specify a starting index and a number of characters to include in the substring. This method is helpful when you want to compare only a specific part of a string.
Comparing Substrings
You can then proceed to compare these substrings just like you would compare full strings.
Example: Substring comparison
#include <iostream>
#include <string>
int main() {
std::string mainStr = "Hello World";
std::string subStr = mainStr.substr(0, 5); // "Hello"
if (mainStr.substr(0, 5) == subStr) {
std::cout << "The substring matches!" << std::endl;
}
return 0;
}
In this example, we extract the first five characters from `mainStr` and compare that substring to `subStr`. The comparison will be successful as they match.
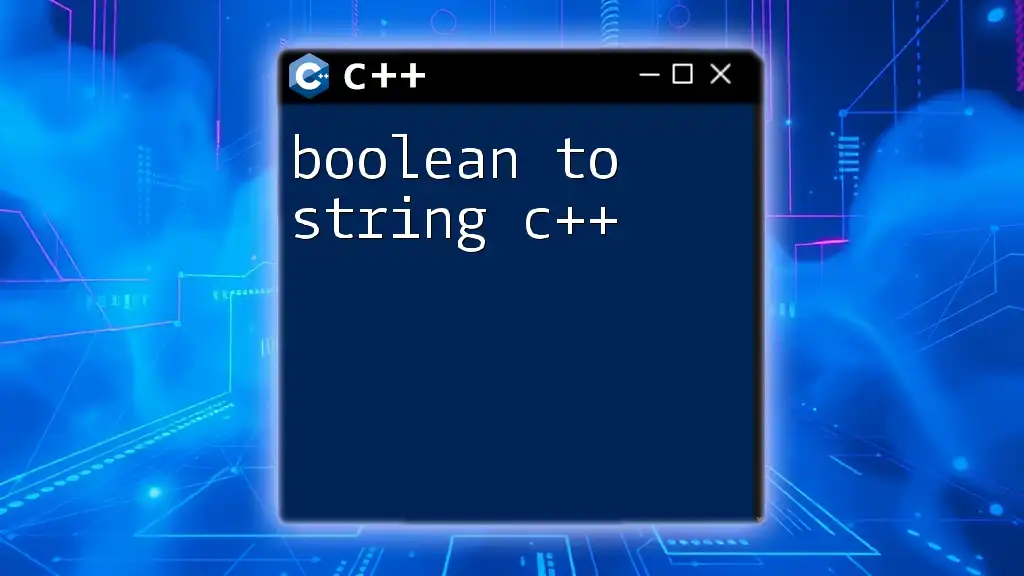
Performance Considerations
When to Use Efficient String Comparisons
In scenarios where performance is a significant concern (like dealing with large strings or frequent comparisons), it's critical to choose the appropriate method. Comparing strings using operators is generally efficient, but keep in mind the size of the strings being compared.
Best Practices for String Comparison in C++
- Avoid unnecessary copies: Use references (`const std::string &`) in function parameters to avoid copying strings.
- Check for early exits: If comparing two strings and one is shorter than the other, you can immediately determine they are not equal.
- Use `std::string::compare`: This function provides a lower-level control for string comparisons.
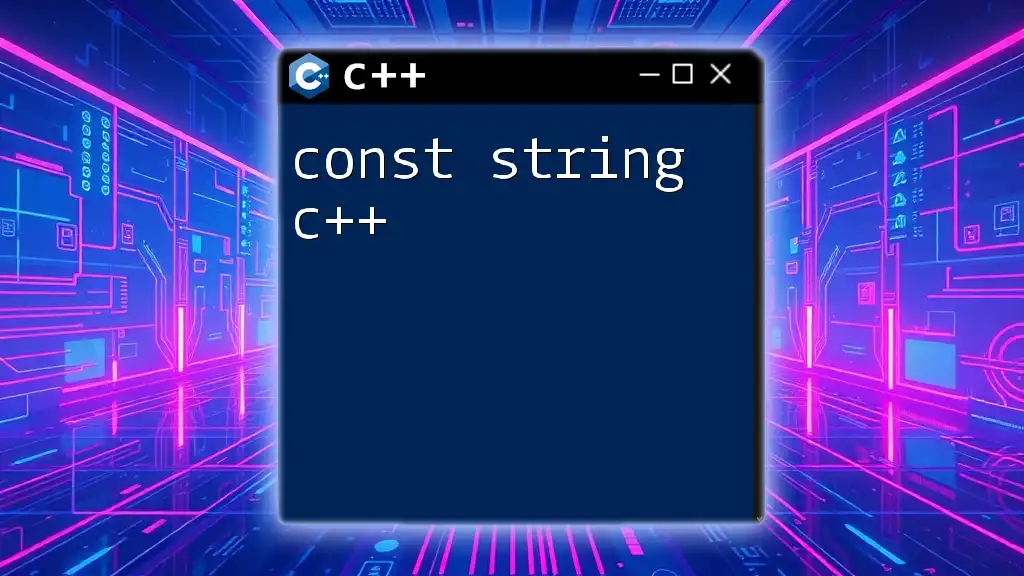
Common Pitfalls
Mistakes with String Comparisons
When comparing strings, some common mistakes may include:
- Using C-style strings (char arrays) without proper null-termination.
- Confusing `==` for pointer comparison instead of content comparison when using C-style strings.
Debugging Tips
To effectively debug string comparison issues:
- Print both strings before comparison to ensure they contain what you expect.
- Consider using logs or assertions to validate assumptions about string content.
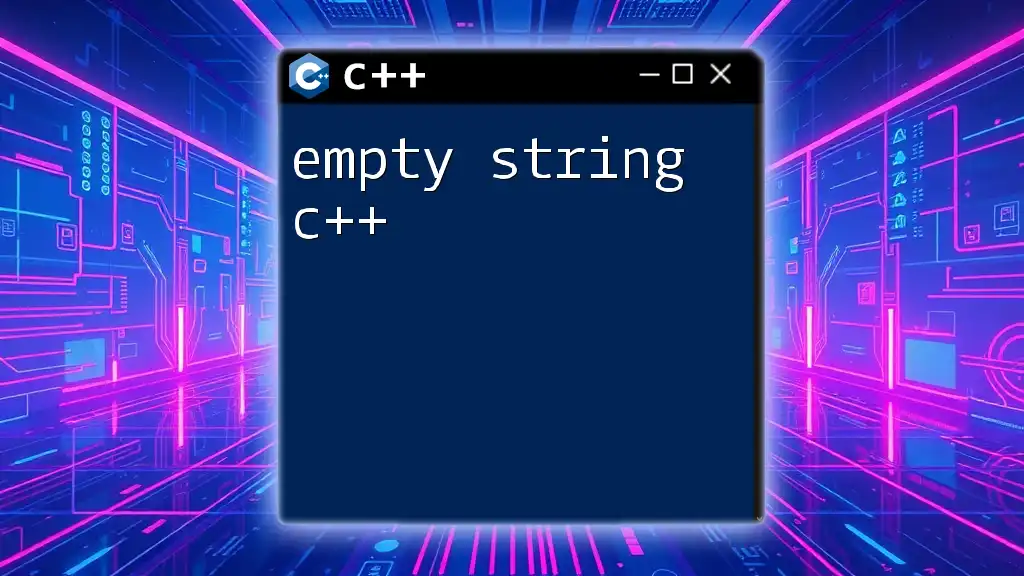
Conclusion
Mastering the art of comparing two strings in C++ is essential for robust application development. The equality operator and relational operators provide straightforward methods for comparison, while considerations for case sensitivity and substring extraction enrich the flexibility of your string manipulations. By adhering to best practices in performance and understanding common pitfalls, you can significantly enhance your programming efficiency in C++.