In C++, you can print output to the console using the `cout` stream along with the insertion operator `<<`. Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Output in C++
What is Output?
In programming, output refers to any data that is sent from a program to the user or to any other system. Printing is a crucial part of this process, as it allows us to communicate results, log data, or provide necessary feedback.
Common Use Cases for Printing
- Debugging Code: Printing helps developers understand the flow of execution and the state of variables at different points in time.
- Displaying Results to Users: Applications often present information to users, such as calculation results or status messages.
- Logging and Monitoring Application Behavior: Print statements can be captured in logs to analyze how an application behaves over time, which is useful for maintenance.
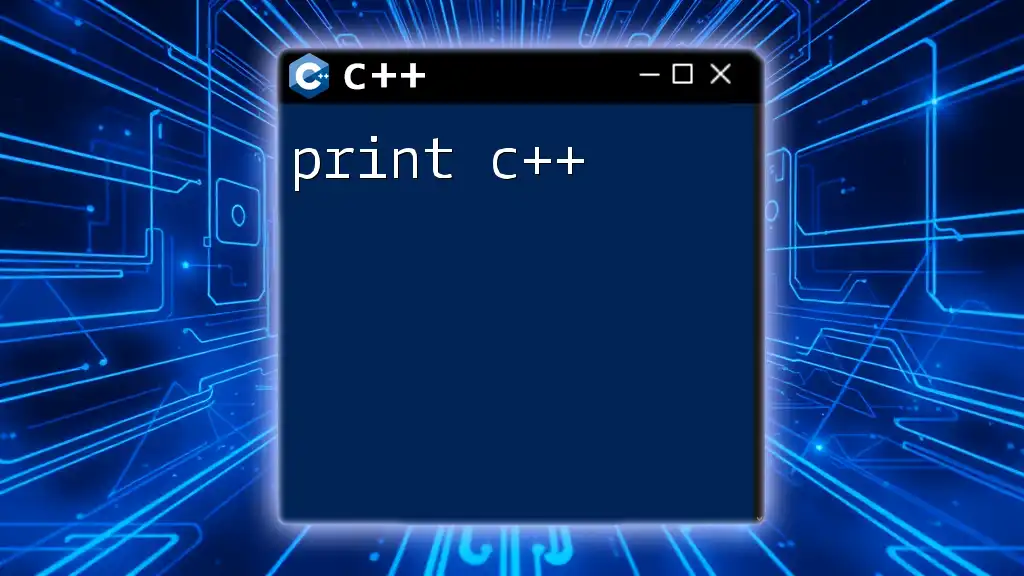
The `cout` Object
Introduction to `cout`
The `cout` (character output) is part of the C++ Standard Library, specifically in the `std` namespace. It enables sending data to the standard output device, which is usually the console.
Basic Syntax
The basic syntax for using `cout` involves the insertion operator `<<`, which directs data to be printed. Here’s how you can use it:
std::cout << "Hello, World!";
Printing Different Data Types
Integer
Printing integers is straightforward. You can display their values directly:
int value = 42;
std::cout << "The value is: " << value << std::endl;
In this example, `std::endl` is used to end the line and flush the output buffer.
Floating-Point Numbers
To print floating-point values, you can use `cout` in a similar manner:
float pi = 3.14;
std::cout << "Value of Pi: " << pi << std::endl;
It's essential to be aware of how floating-point values are represented in C++, as small inaccuracies can occur due to the nature of floating-point arithmetic.
Characters and Strings
Printing characters and strings can be done using `cout` as well:
char letter = 'A';
std::cout << "The first letter is: " << letter << std::endl;
std::string name = "Alice";
std::cout << "Hello, " << name << "!" << std::endl;
Here, we see how `cout` can concatenate different data types seamlessly.
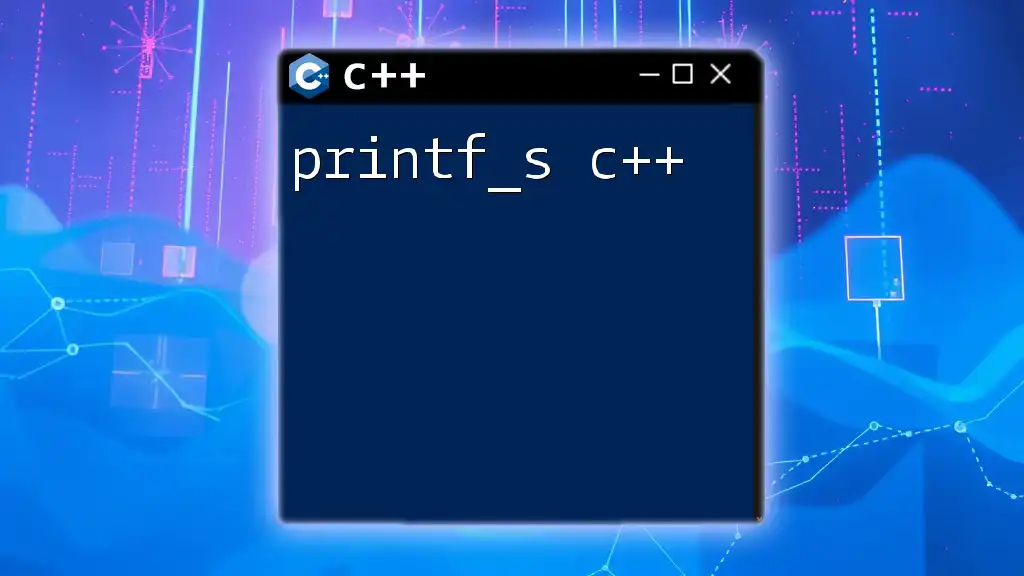
Manipulators in C++
What are Manipulators?
Manipulators are special functions used in C++ to format output. They make it easier to manipulate how data is displayed, providing control over aspects like alignment and precision.
Commonly Used Manipulators
`std::endl`
The `std::endl` manipulator is used to insert a newline character and flush the output buffer, ensuring that all output is displayed immediately:
std::cout << "Line one" << std::endl;
`std::fixed` and `std::setprecision`
These manipulators are valuable for controlling the number of decimal places when printing floating-point numbers:
#include <iomanip>
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
Using `std::fixed` ensures that the output is in fixed-point notation, while `std::setprecision(2)` limits the output to two decimal places.
Other Useful Manipulators
- `std::setw()`: Sets the width of the next output field. This is useful for aligning output.
std::cout << std::setw(10) << std::left << value << std::endl;
- `std::left`, `std::right`, `std::internal`: These manipulators control the alignment of output text within the designated field width.
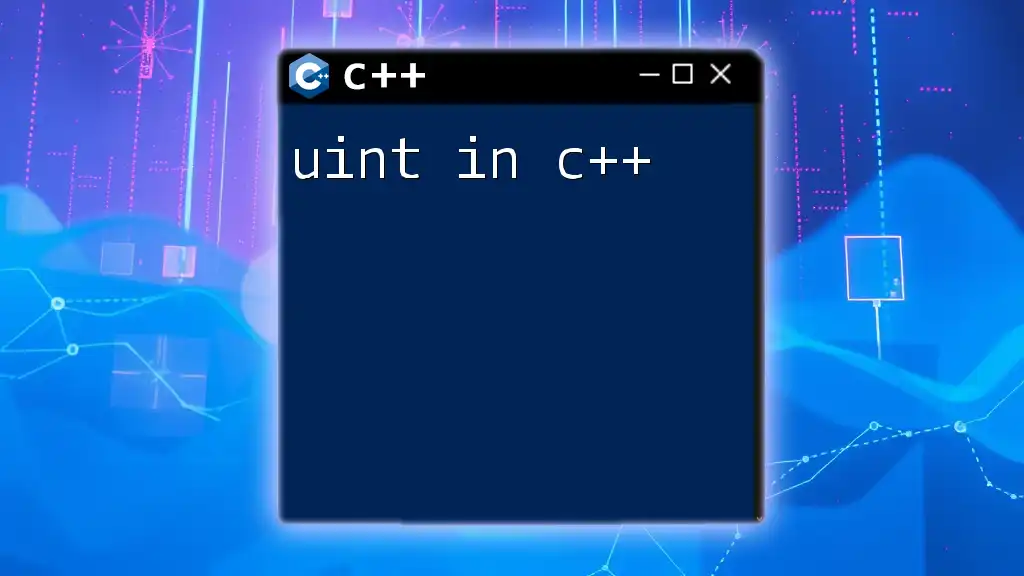
Error Handling with `cerr`
Introduction to `cerr`
`cerr` is another output stream in C++ designed specifically for error messages. Unlike `cout`, which can be buffered, `cerr` writes messages immediately to the output, making it ideal for logging errors.
Best Practices for Using `cerr`
When using `cerr`, it's essential to provide clear and concise messages that explain what went wrong. Here's an example:
std::cerr << "Error: Invalid input!" << std::endl;
Using `cerr` ensures that error messages are not lost or delayed, which is crucial for debugging.
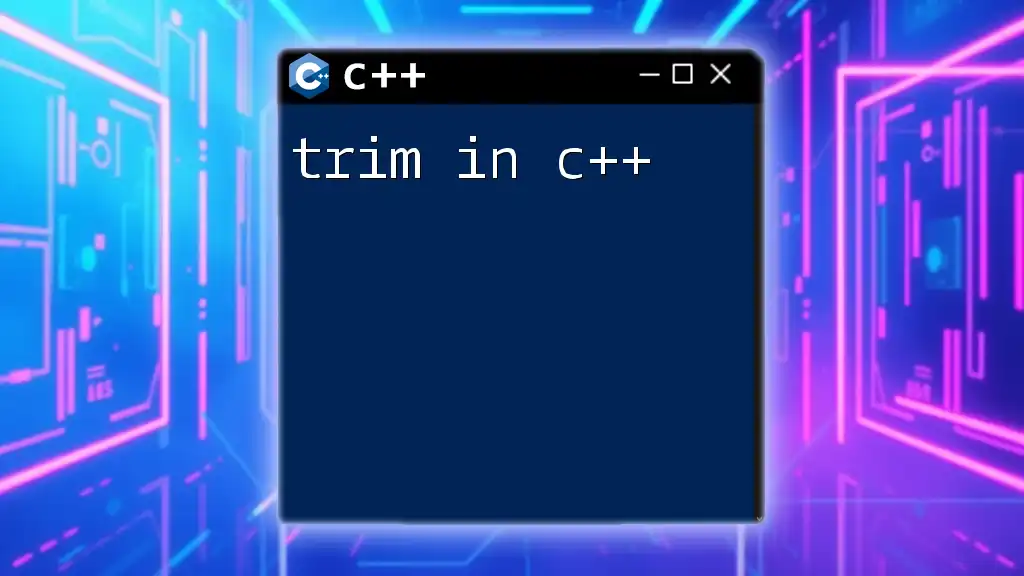
Formatting Output
Using `printf` Function
While C++ offers `cout`, you can also utilize `printf`, a feature inherited from C for formatted output. The syntax differs significantly:
printf("Value of Pi: %.2f\n", pi);
The formatting options in `printf` allow for dynamic and precise output control, which can be especially useful in cases where you require a specific format.
Stream Formatting with `ostringstream`
You can also format output using string streams, which allows building strings before outputting them:
#include <sstream>
std::ostringstream oss;
oss << "The value is: " << value;
std::cout << oss.str() << std::endl;
This method is useful for cases where you need to accumulate various pieces of output before displaying them.
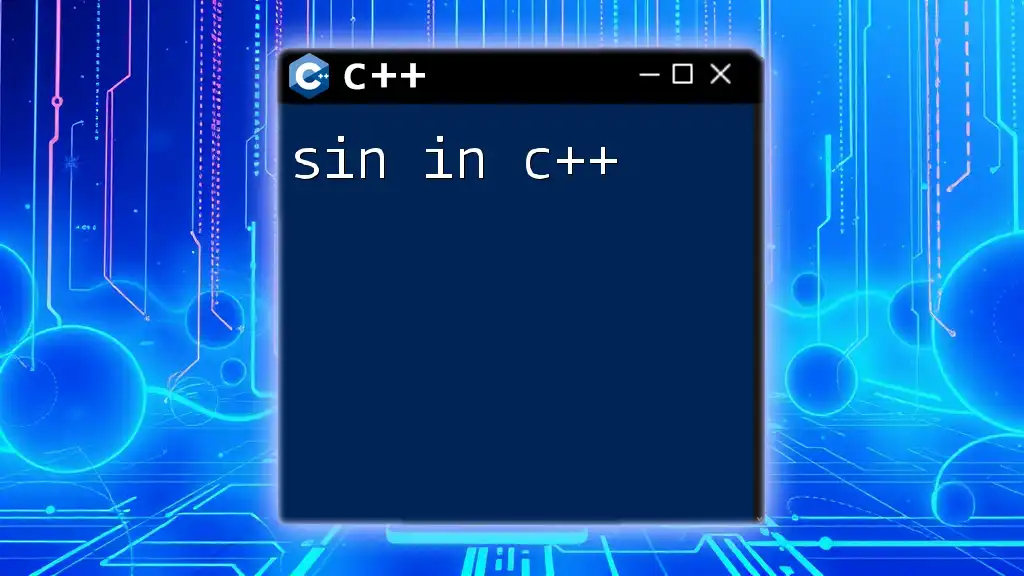
Printing Custom Objects
Overloading the `<<` Operator
In C++, you can extend the `cout` functionality by overloading the `<<` operator for your custom classes. This allows you to define how instances of your custom types should be printed:
class Point {
public:
int x, y;
friend std::ostream& operator<<(std::ostream& os, const Point& pt) {
os << "(" << pt.x << ", " << pt.y << ")";
return os;
}
};
By implementing this operator as a friend function, we allow `cout` to understand how to print `Point` objects, facilitating their output in a clean and user-friendly way.
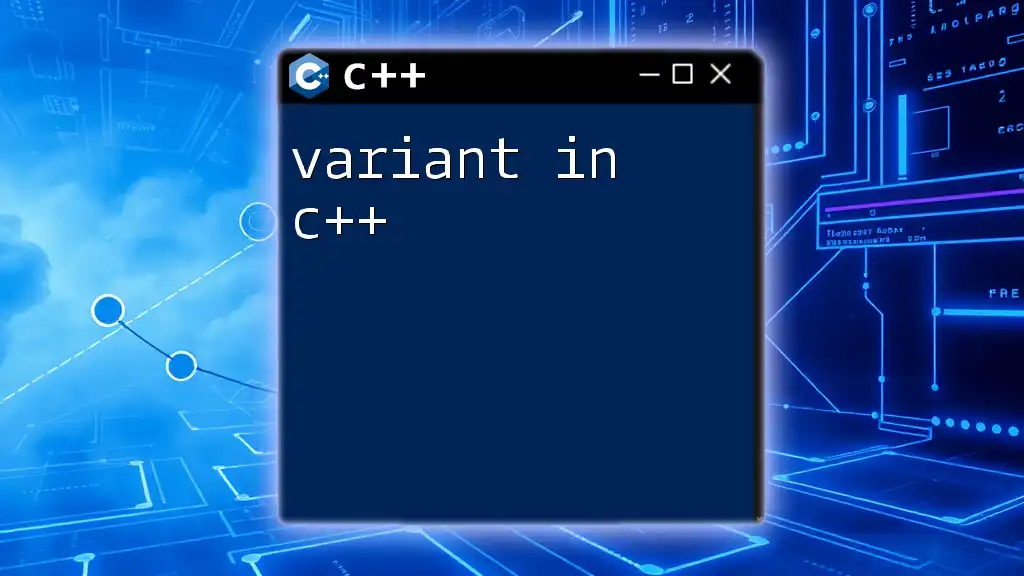
Conclusion
In summary, printing in C++ is a vital skill for any programmer, whether you are debugging code, displaying user information, or logging errors. The `cout` object, along with its manipulators and alternative output methods like `cerr` and `printf`, provides a flexible toolkit for managing output effectively.
By understanding the nuances of printing different data types and formats, as well as how to display custom objects, you can leverage these techniques to enhance your coding efficiency and improve user experiences. Embrace these practices, experiment with different methods, and you’ll quickly find the power of printing in C++.