APIs (Application Programming Interfaces) in C++ provide a set of tools and protocols that allow different software applications to communicate and interact with each other efficiently.
Here’s a simple code snippet showcasing how to use an API in C++ to retrieve data using the `cpr` library for making HTTP requests:
#include <cpr/cpr.h>
#include <iostream>
int main() {
auto response = cpr::Get(cpr::Url{"https://api.example.com/data"});
std::cout << response.text << std::endl;
return 0;
}
Make sure to link the `cpr` library in your project to use this code.
Understanding APIs in C++
What Are APIs?
An Application Programming Interface (API) is a set of rules and protocols for building and interacting with software applications. APIs are crucial in enabling different software systems to communicate, allowing developers to integrate functionalities from various sources seamlessly. In the realm of C++, APIs form the backbone of many libraries and frameworks, facilitating the use of pre-built functions without needing to reinvent the wheel.
Types of APIs
Library APIs
Library APIs provide a collection of functions and classes that developers can utilize without having to write code from scratch. In C++, the Standard Template Library (STL) is a prime example. It includes a variety of templates for data structures and algorithms, profoundly simplifying programming tasks such as sorting, searching, and managing data collections.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Web APIs
Web APIs are interfaces that allow developers to interact with remote servers over the Internet, usually using HTTP requests. RESTful APIs are widely used due to their simplicity and stateless nature. For instance, a C++ client can interact with a web-based weather service by sending a request to fetch current weather data.
Operating System APIs
Operating System APIs allow developers to perform low-level interactions with the operating system. For example, POSIX provides a standardized API for Unix-like operating systems, making it easier to write cross-platform applications.
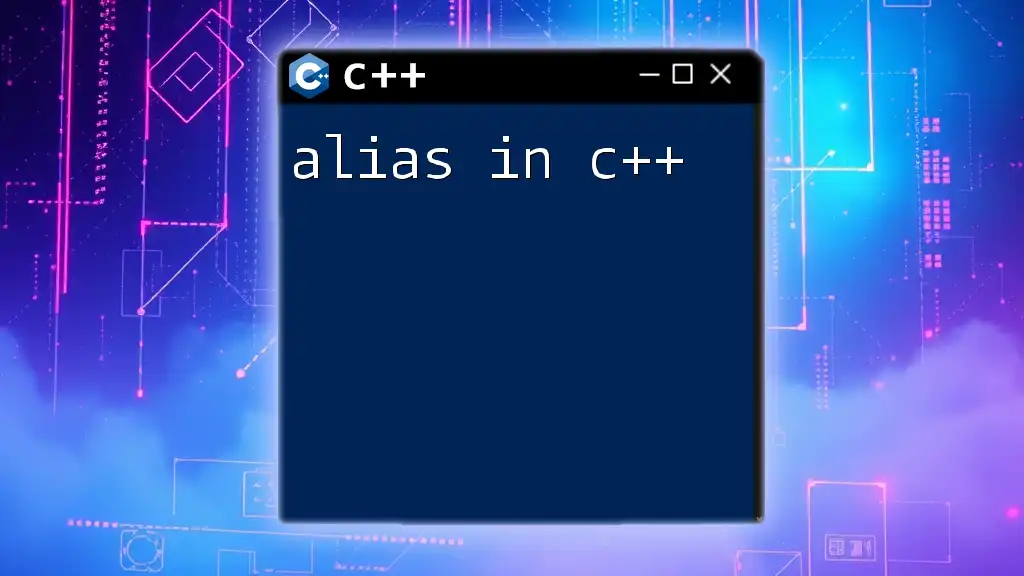
Building APIs in C++
Designing a C++ API
When designing a C++ API, adhering to certain principles is essential for ensuring usability and maintainability. Key strategies include encapsulation, clear interface definitions, and consideration for future scalability. A well-structured API should allow for easy integration without exposing complex internal workings.
Creating Functions and Classes
Functions as API Endpoints
Functions serve as the building blocks of APIs. They allow external developers to call specific functionalities. Here’s a simple example of creating a basic API function in C++:
#include <iostream>
void greet(const std::string& name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
Classes for Encapsulation
Classes are fundamental in C++ API design for encapsulating data and behavior. A simple class-based API could represent a geometric shape:
#include <iostream>
#include <cmath>
class Circle {
public:
Circle(double r) : radius(r) {}
double area() const {
return M_PI * radius * radius;
}
private:
double radius;
};
In this example, the `Circle` class encapsulates the radius and provides a method to calculate the area, illustrating how to expose functionality while hiding implementation details.
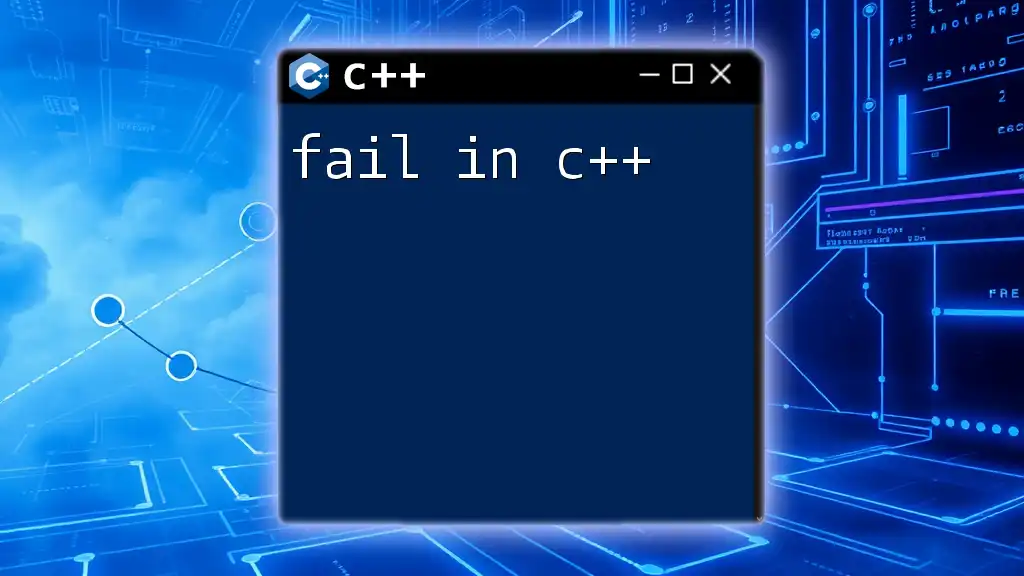
Consuming APIs in C++
Making API Calls
To interact with web APIs, you need to send HTTP requests. In C++, the libcurl library makes this task straightforward. Here’s how to make a simple GET request:
#include <iostream>
#include <curl/curl.h>
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
std::cout << readBuffer << std::endl;
return 0;
}
Handling Responses
Responses from web APIs often come in standard formats like JSON or XML. Using the nlohmann/json library simplifies JSON parsing. Here’s an example of how to parse a JSON response in C++:
#include <iostream>
#include <nlohmann/json.hpp>
void parseWeatherResponse(const std::string& response) {
auto jsonResponse = nlohmann::json::parse(response);
std::cout << "Weather in " << jsonResponse["name"] << ": " << jsonResponse["weather"][0]["description"] << std::endl;
}
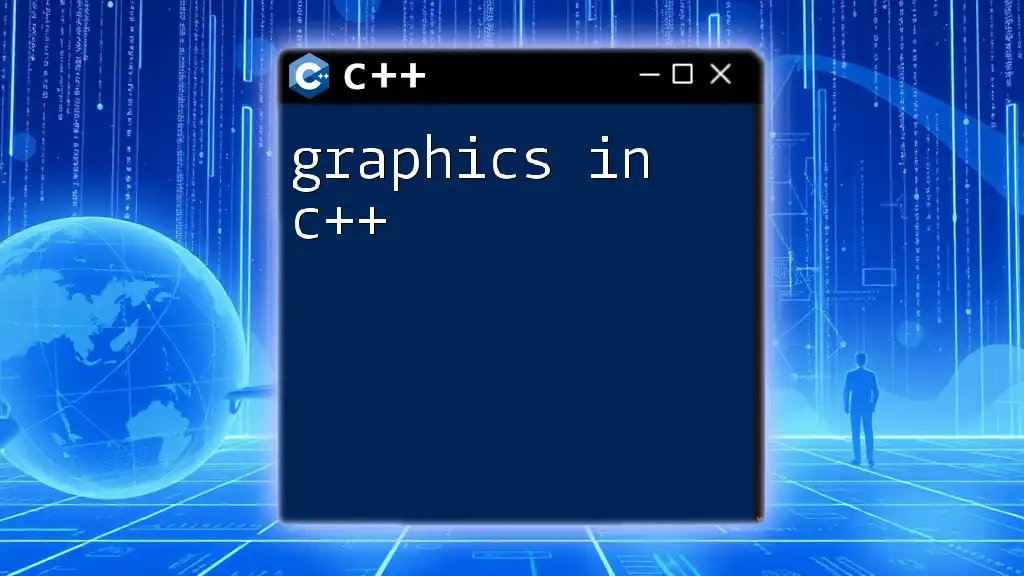
Error Handling in APIs
Importance of Robust Error Handling
When developing APIs, incorporating robust error handling mechanisms is vital. Common issues such as network failures, invalid responses, and timeouts can hinder functionality. C++ provides mechanisms like exceptions and error codes to manage these situations effectively.
Examples of Error Handling
To manage errors gracefully, you can use try-catch blocks in C++:
try {
// Attempt API call or parsing
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
Custom exception classes can also enhance your error-handling strategy, providing clearer insights upon failures.
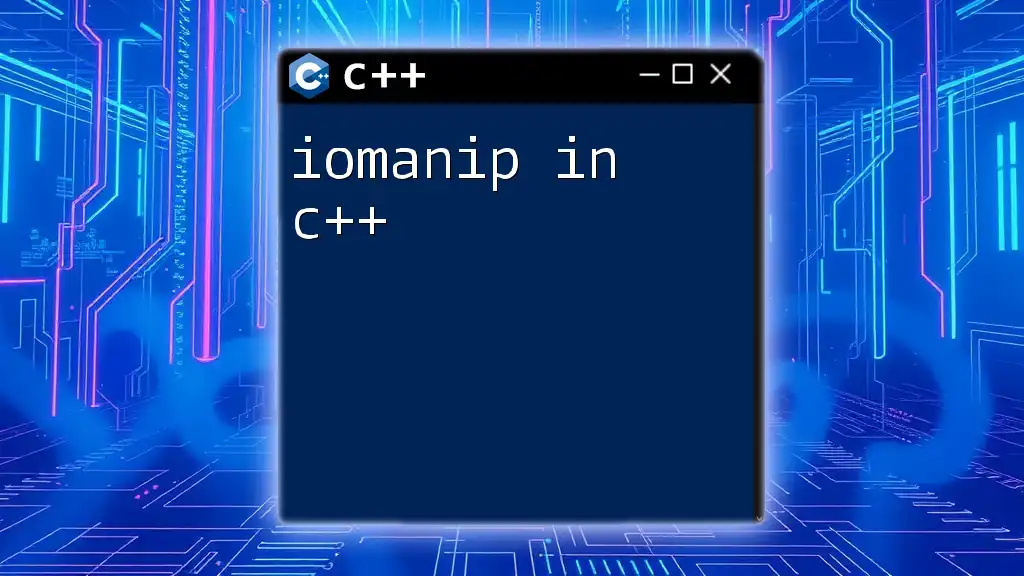
Best Practices for C++ APIs
Documentation and Comments
One of the crucial aspects of API development is documentation. It helps other developers understand how to use your API effectively. Consider tools like Doxygen or DocFX to generate comprehensive documentation from your code comments.
Versioning Your API
Effective API versioning ensures backward compatibility and allows you to introduce new features without breaking existing applications. Following semantic versioning conventions (MAJOR.MINOR.PATCH) can guide you in structuring your versions logically.
Security Considerations
API security must be a priority throughout development. Common vulnerabilities, such as SQL injection and cross-site scripting, must be addressed. Implementing authentication mechanisms like OAuth can also protect sensitive data.
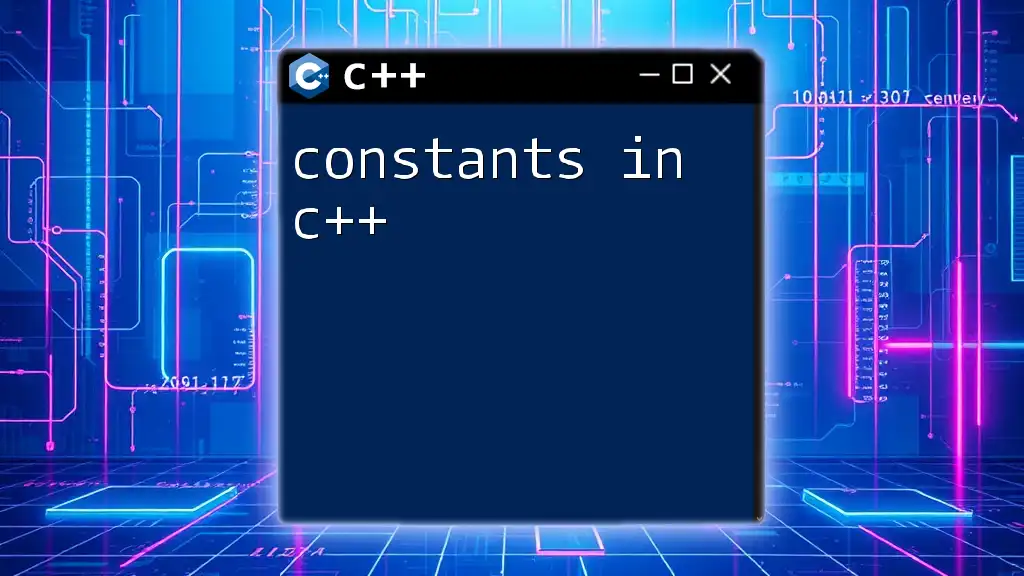
Conclusion
APIs in C++ play an integral role in modern software development, enabling efficient functionality integration and enhancing project scalability. By understanding the various types of APIs, learning how to build and consume them, and applying best practices, developers can maximize their productivity and unleash the full potential of C++ programming. As the landscape of API development continues to evolve, keeping abreast of new trends and techniques is crucial for any C++ developer.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Additional Resources
Books and Tutorials
To deepen your understanding, consider exploring recommended C++ programming books that delve into advanced topics, best practices, and API integration techniques.
Online Communities
Engaging with online forums and communities can provide valuable insights, allowing you to connect with other C++ API enthusiasts and expand your knowledge through shared experiences and collaboration.
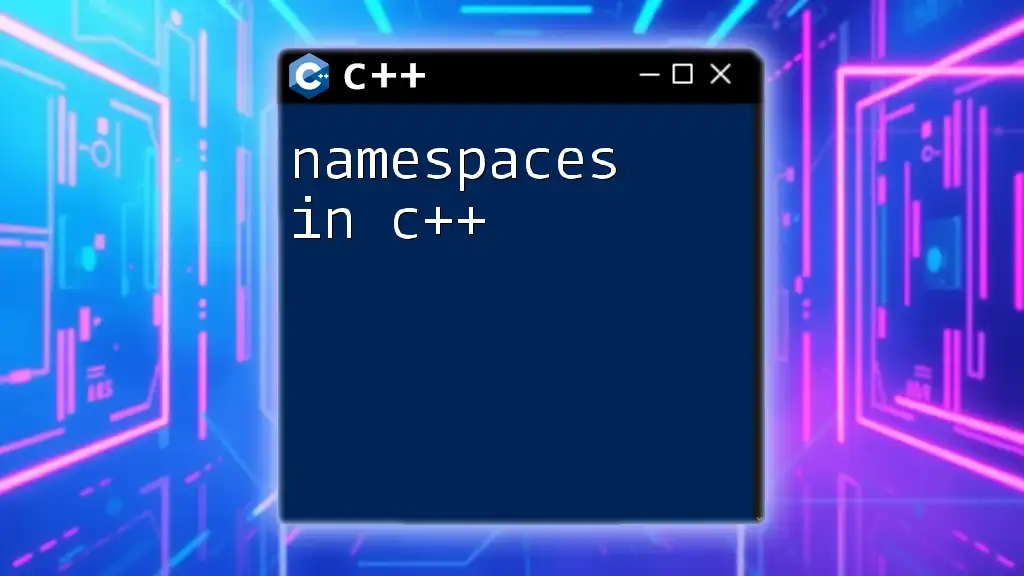
Call to Action
We invite you to share your experiences with APIs in C++. What challenges have you faced? What solutions have you implemented? Leave your thoughts and questions in the comments, and let's foster a community of learning together! Don’t forget to subscribe for more insights into mastering C++ programming.