The `puts` function in C++ is used to output a string to the console, automatically appending a newline character at the end.
#include <cstdio>
int main() {
puts("Hello, World!");
return 0;
}
What is `puts` in C++?
The `puts` function is a standard library function in C++ that belongs to the C programming language. It provides a way to output a string to the standard output (usually the console or terminal). Its primary distinction from other output functions like `cout` and `printf` lies in its simplicity and automatic handling of line breaks.
Syntax of `puts`
The syntax of the `puts` function is as follows:
int puts(const char *str);
- Parameters: `str` is a pointer to a null-terminated string that you want to print.
- Return Value: The function returns a non-negative value on success and a negative value if an error occurs.
To use `puts`, ensure you include the required header file at the beginning of your C++ program:
#include <cstdio>

How to Use `puts` in C++
Basic Usage of `puts`
Using `puts` is straightforward. Here’s a simple example demonstrating how to display a string:
#include <cstdio>
int main() {
puts("Hello, World!");
return 0;
}
In the example above, the `puts` function outputs "Hello, World!" to the console and automatically adds a newline character after the string. This behavior is a significant advantage when you need to print a string and start a new line without manually adding `\n`.
Example with Multiple Calls
You can also use `puts` multiple times in your program to display different strings. For example:
#include <cstdio>
int main() {
puts("First Line");
puts("Second Line");
return 0;
}
This code will output:
First Line
Second Line
Each string appears on a new line because of the automatic newline insertion by `puts`.

Advantages of Using `puts`
Simplicity and Ease of Use
The `puts` function is incredibly user-friendly. Unlike `cout` or `printf`, it requires minimal syntax. This makes it particularly beneficial for quick and straightforward debugging messages, where you simply want to check some output without worrying about formatting.
Performance Considerations
In terms of performance, `puts` is typically faster than `printf` and sometimes even faster than `cout`. This is because `puts` focuses solely on producing output, without the overhead of handling format specifiers or type safety. Thus, in performance-critical applications where simple string output is required, `puts` can be an optimal choice.

Limitations of `puts`
Limited Formatting Capability
While `puts` excels in simplicity, it lacks the formatting capabilities found in `printf`. If you need to display formatted strings or print variables combined with strings, you'll need to use `printf` or `cout`. Here’s how you would use `printf`:
#include <cstdio>
int main() {
int number = 42;
printf("The answer is: %d\n", number);
return 0;
}
In contrast, `puts` doesn’t support format specifiers, so it's mainly useful for basic output tasks.
UTF-8 and Character Encoding Issues
Another limitation of `puts` arises when dealing with character encodings, particularly non-ASCII characters. Always ensure that your strings are properly encoded to avoid unexpected output.
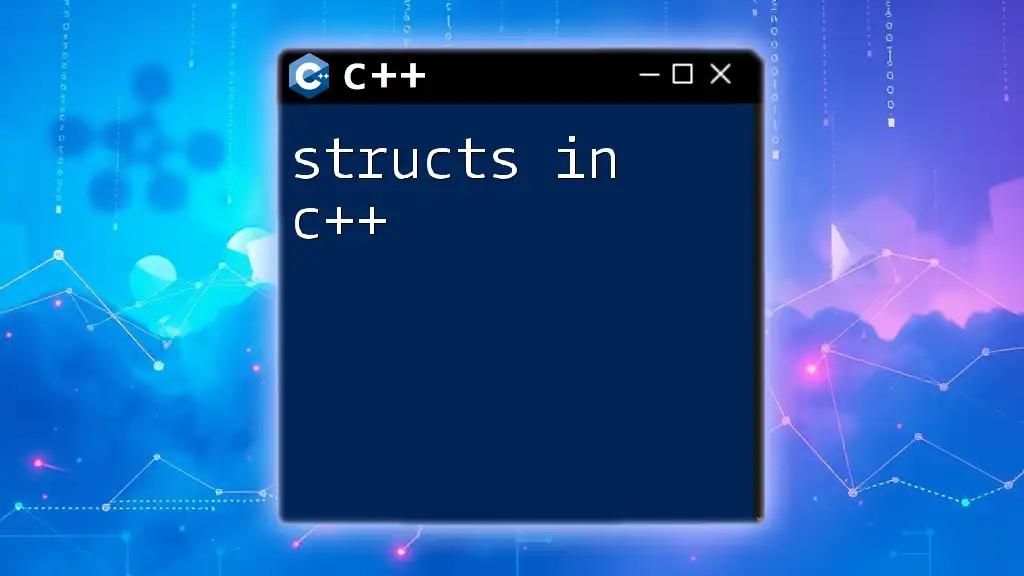
Common Mistakes to Avoid When Using `puts`
Not Including the Header File
One of the most common mistakes that can hinder compilation is forgetting to include the necessary header file. Always remember to add:
#include <cstdio>
Forgetting the Null-Terminator
C-style strings, which `puts` relies on, must be null-terminated. Failing to provide a null terminator will lead to undefined behavior. For example, this code is incorrect:
#include <cstdio>
int main() {
char str[] = {'H', 'e', 'l', 'l', 'o'}; // No null terminator
puts(str); // Undefined behavior
return 0;
}
In this case, `puts` may print garbage values or lead to a program crash. Always ensure that your strings are properly null-terminated.
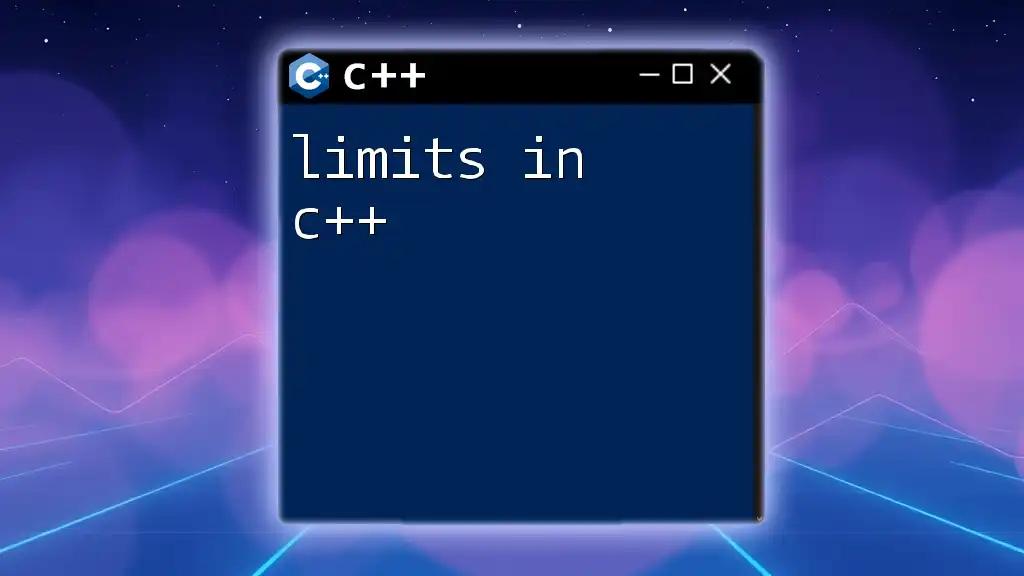
Alternatives to `puts` in C++
Using `cout` for Output
While `puts` is straightforward, `cout` offers much more flexibility with the advantage of being safer. For instance:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `cout` requires you to specify whether you want to add a new line (using `std::endl`) or not. `cout` is location- and type-safe, making it a preferred choice for complex applications.
Using `printf` for Formatted Output
If you need to output formatted text, `printf` is the better choice. This function allows you to include format specifiers, such as integers and floating-point numbers:
#include <cstdio>
int main() {
printf("The answer is: %d\n", 42);
return 0;
}
Using `printf`, you can easily format strings and mix different data types within the same output.

Conclusion
The `puts` function is a convenient way to display strings in C++, offering simplicity and ease of use for quick outputs. However, it is essential to be aware of its limitations in formatting and encoding. Understanding when to use `puts`, `cout`, and `printf` will help you make informed choices while developing in C++. Whether you're debugging or writing a full-scale application, knowing the right tool for output will enhance your coding experience.

Additional Resources
For more insights on output functions and their appropriate contexts in C++, consider exploring further reading and tutorials. Engage with coding exercises to practice using `puts` and experience the differences firsthand.
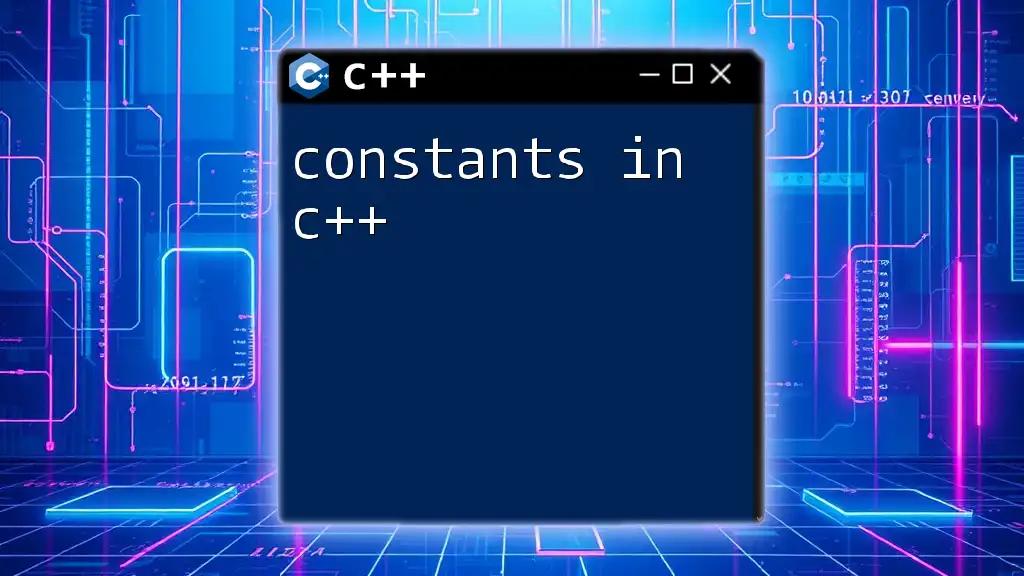
Call to Action
Feel free to share your experiences or any questions about using `puts` in C++. Your inquiries could help others in the community learn and explore this essential C++ functionality!