Structs in C++ are user-defined data types that group related variables under one name, allowing you to create complex data structures with multiple attributes.
Here's a simple code snippet demonstrating how to define and use a struct in C++:
#include <iostream>
struct Person {
std::string name;
int age;
};
int main() {
Person person1;
person1.name = "Alice";
person1.age = 30;
std::cout << "Name: " << person1.name << ", Age: " << person1.age << std::endl;
return 0;
}
Understanding Structs
Definition of Structs
Structs in C++ are essentially user-defined data types that allow you to combine different data items into a single unit. They can be compared to simple classes, with some key distinctions, mainly that structs have public members by default. This feature makes them an excellent choice for grouping related data together without the need for complex accessor methods.
Structs are particularly useful for representing a single entity that encompasses various attributes. For instance, a `Person` struct can hold multiple characteristics like name, age, and address.
Key Features of Structs
Public Members by Default
A fundamental aspect of structs in C++ is that their members are public by default, which means they can be accessed directly without the need for a public access specifier. This contrasts with classes, which have private members automatically.
Example:
struct Person {
std::string name; // Public by default
int age; // Public by default
};
In this example, both `name` and `age` can be accessed outside of the struct without any additional functions.
Data Aggregation
Structs are capable of aggregating different data types into a cohesive unit. This functionality allows for more organized and readable code. For example, consider the following struct that stores information about a book:
struct Book {
std::string title;
std::string author;
int year;
};
Here, the `Book` struct combines three different data types: two strings and an integer, effectively representing a single book entity.
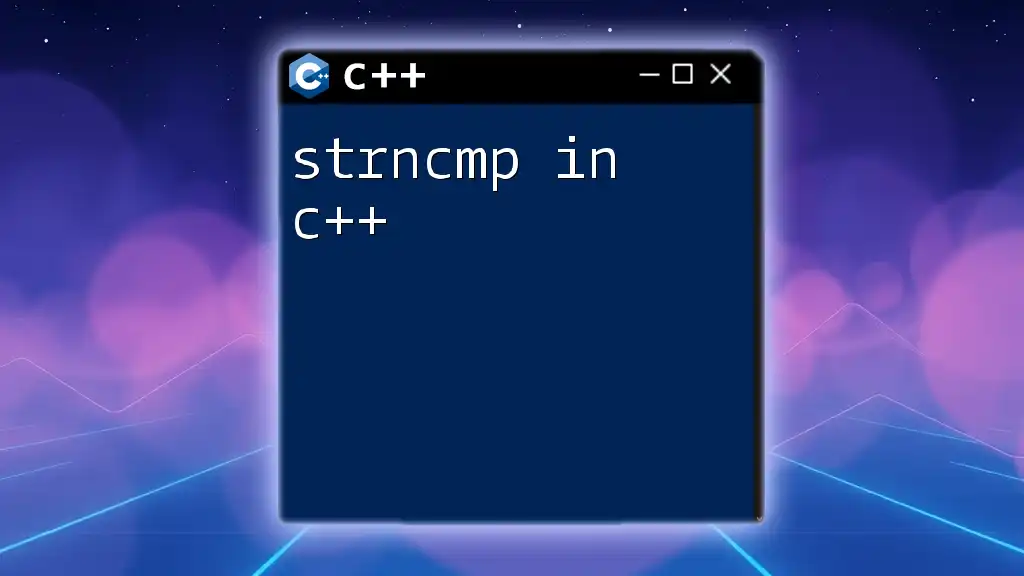
Creating and Using Structs
Defining a Struct
The syntax for defining a struct in C++ is straightforward. You use the `struct` keyword followed by the name of the struct, and enclosed within curly braces are the variables that represent the struct's data.
Here’s an example of how to declare a simple struct:
struct Book {
std::string title;
std::string author;
int year;
};
This declaration creates a new data type called `Book` with three members: `title`, `author`, and `year`.
Instantiating a Struct
Once a struct has been defined, you can create instances of it. You can initialize a struct at the time of creation, making it easy to populate data.
Example:
Book myBook = {"1984", "George Orwell", 1949};
In this line, we create a `Book` object named `myBook` and initialize it with values directly within the curly braces.
Accessing Struct Members
To access the members of a struct, you simply use the dot operator (`.`). This operator provides a clear and intuitive way to manipulate the data contained within the struct.
For instance, to print the title of `myBook`, you can do the following:
std::cout << myBook.title; // Outputs: 1984
This operation directly retrieves the `title` member from the `myBook` instance.
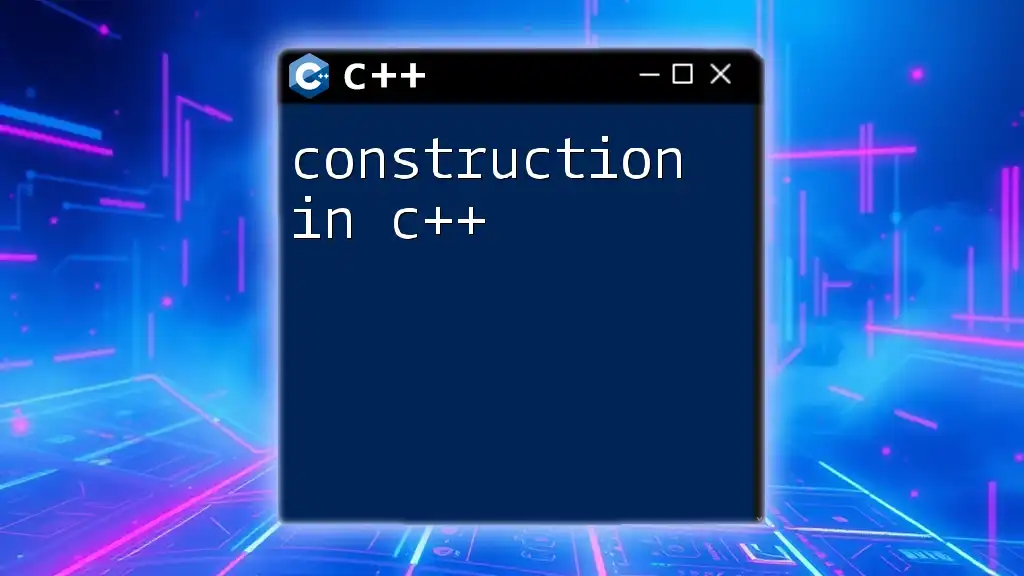
Advanced Struct Concepts
Structs with Functions
Another interesting feature of structs in C++ is the ability to include member functions, just like you would in a class. This allows you to encapsulate functionality within the struct, making it even more versatile.
struct Rectangle {
int width, height;
int area() {
return width * height;
}
};
In this example, the `Rectangle` struct has a method called `area()` that calculates the area based on the struct's dimensions provided via its members.
Nested Structs
Structs can also contain other structs, allowing for more sophisticated data organizations. This feature is particularly useful for modeling complex relationships.
struct Address {
std::string city;
std::string state;
};
struct Person {
std::string name;
Address address; // Nested struct
};
In this case, the `Person` struct has an `address` member that is itself a struct, demonstrating how to create nested levels of structured data.
Structs and Pointers
Using pointers with structs can enhance memory management by allowing for dynamic allocation. This approach is beneficial when you need to work with large amounts of structured data.
Person* p = new Person();
p->name = "Alice"; // Using pointer to set name
By utilizing a pointer, you are able to create an instance of `Person` on the heap, which gives you flexibility with memory management, particularly useful in larger applications.
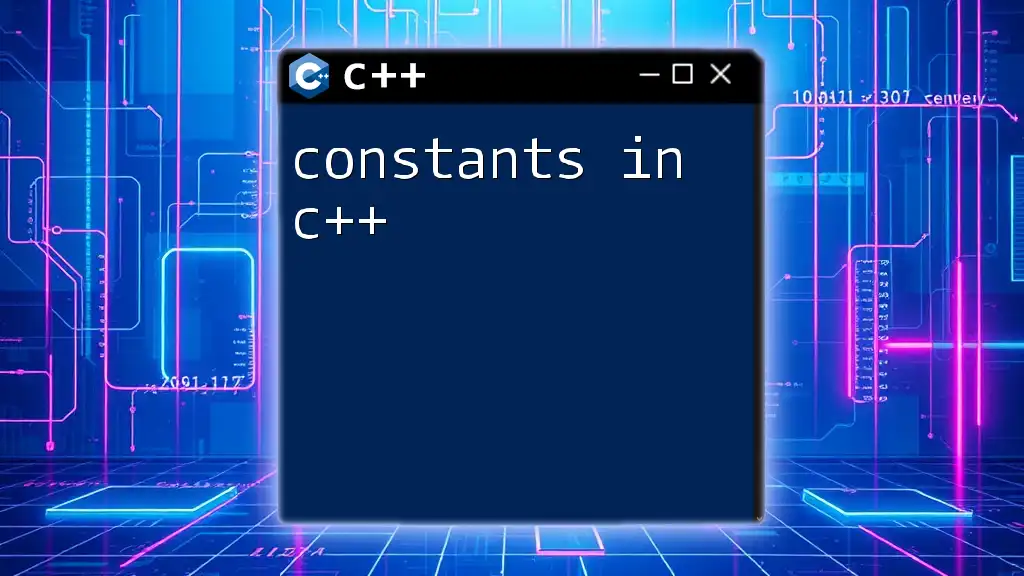
Common Use Cases for Structs
Data Transfer Objects (DTOs)
Structs in C++ are often employed for Data Transfer Objects (DTOs), which make passing data between methods or functions straightforward. They simplify the code, making it cleaner and easier to understand.
Representing Complex Data Types
Structs are ideal for representing complex data structures in real-world applications. Here are a couple of relatable examples:
-
Bank Account: You can design a `BankAccount` struct that contains fields for `accountNumber`, `balance`, and methods for `deposit`, and `withdraw`.
-
Vehicle Specification: A `Vehicle` struct could include fields like `make`, `model`, and `year`, encapsulating essential data about a vehicle.
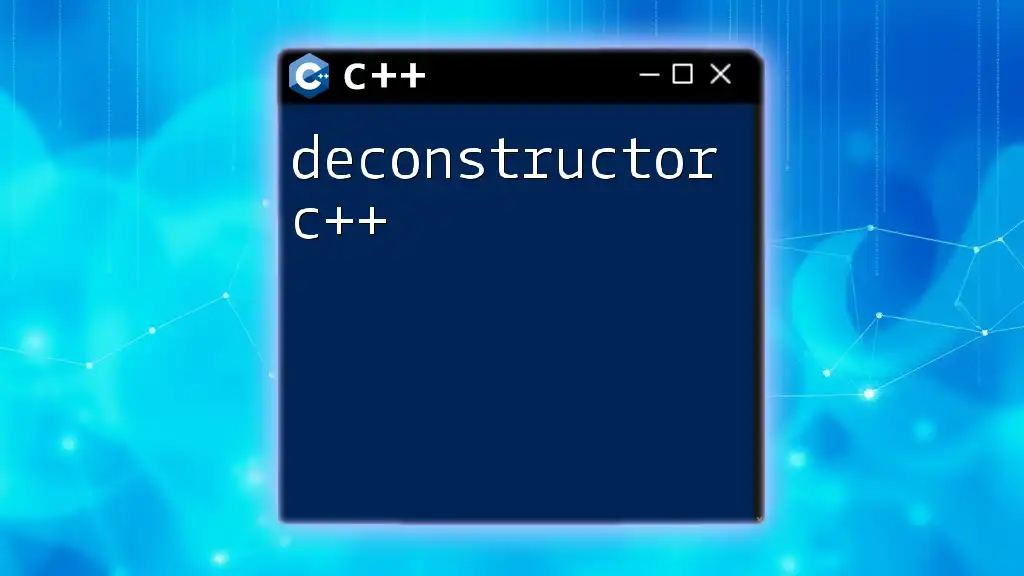
Conclusion
Structs in C++ are a powerful concept that offers simplicity and flexibility in data management. Their ability to aggregate data types, support member functions, and nest other structs makes them versatile for various applications. By understanding how to create and manipulate structs, you can significantly enhance your programming capabilities.
Continue exploring structs in your coding projects, implement them in real scenarios, and witness how they can streamline your data handling processes.
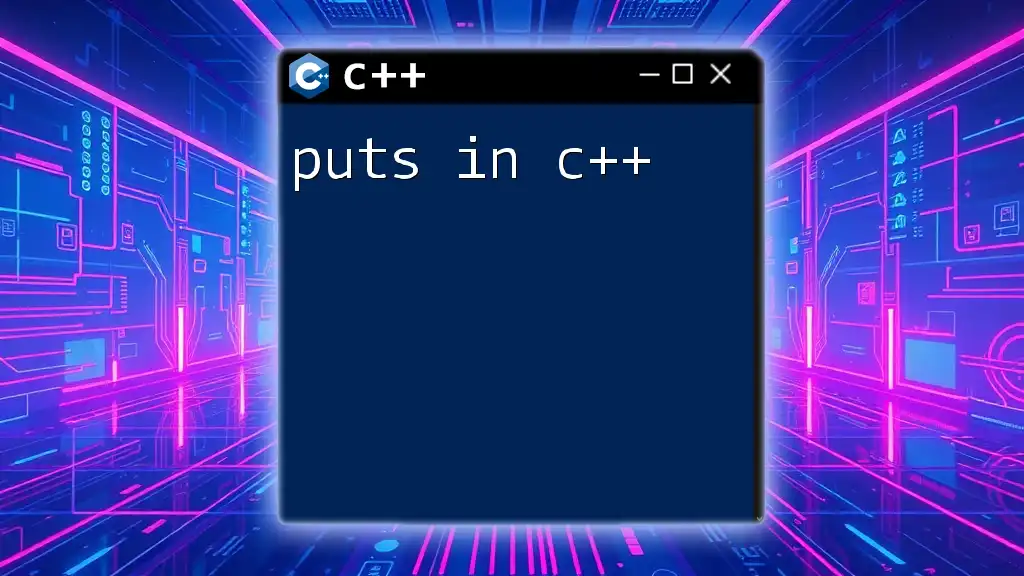
Resources
To deepen your understanding of structs and expand your C++ knowledge, consider checking out the following resources:
- C++ Programming Language by Bjarne Stroustrup
- Online C++ reference sites
- Interactive coding tutorials and courses focused on C++ structures and design.