In C++, an alias is a convenient way to create an alternate name for an existing type, using the `using` keyword or the `typedef` keyword to improve code readability and maintainability.
Here's a simple example using the `using` keyword:
using Integer = int; // Integer is now an alias for int
And here’s an example using `typedef`:
typedef int Integer; // Integer is also an alias for int
What is an Alias in C++?
In the context of programming, an alias refers to a secondary name that you can use to refer to a data type. This concept is particularly beneficial in the world of C++ where types can get complex, and better code readability is paramount. Aliasing helps developers avoid repeating verbose type definitions, which enhances both clarity and maintainability of the code.
An alias effectively allows you to define a new name for an existing type, making your code more intuitive and easier to manage. Understanding aliases is crucial for every C++ programmer aiming to write cleaner and more maintainable code.
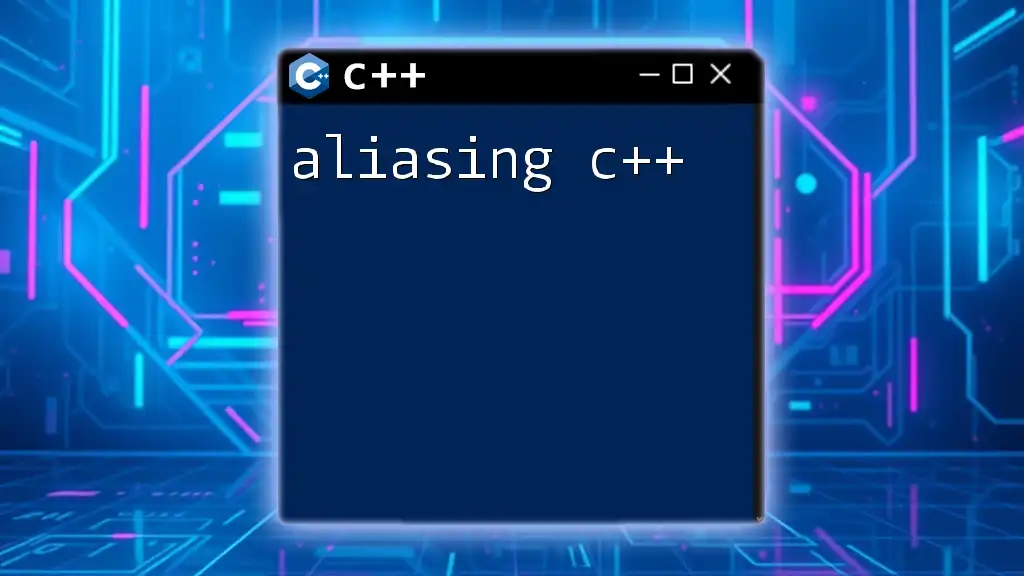
Types of Aliases in C++
Using typedef
One of the traditional methods to create an alias in C++ is through the `typedef` keyword. This keyword allows you to create a new name for an existing type, which can simplify code significantly.
Code Example: Using typedef to Create an Alias
typedef unsigned long ulong;
ulong number = 1000; // Using ulong instead of unsigned long
In this example, instead of writing `unsigned long` every time, you can simply use `ulong`. This not only makes your code neater but also reduces the likelihood of errors due to repetitive typing. The benefits of using `typedef` are clear—it leads to cleaner code and simpler type management.
Using typedef with Structures and Classes
Aliases become even more valuable when dealing with custom types such as structures and classes. By using `typedef`, you can create more meaningful and context-specific names for your types.
Code Example: Typedef for Structs
struct Person {
std::string name;
int age;
};
typedef Person User;
User user1; // More intuitive than struct Person user1;
In this scenario, `User` serves as a more understandable alias for `Person`. This can greatly enhance the readability of your code, especially for those who may not be as familiar with the underlying data structures.
Using the `using` Keyword
In modern C++, the `using` directive has emerged as a more versatile and readable alternative to `typedef`. It allows for the creation of type aliases that can often be easier to understand, especially when dealing with complex types.
Code Example: Using for Type Definitions
using IntPtr = int*;
IntPtr ptr = nullptr; // Using IntPtr instead of int*
With the `using` keyword, you get cleaner syntax that can be especially useful in the case of complex types, such as pointers or function types. When coding, the primary advantage of `using` is that it promotes code readability, making it less intimidating for new developers.
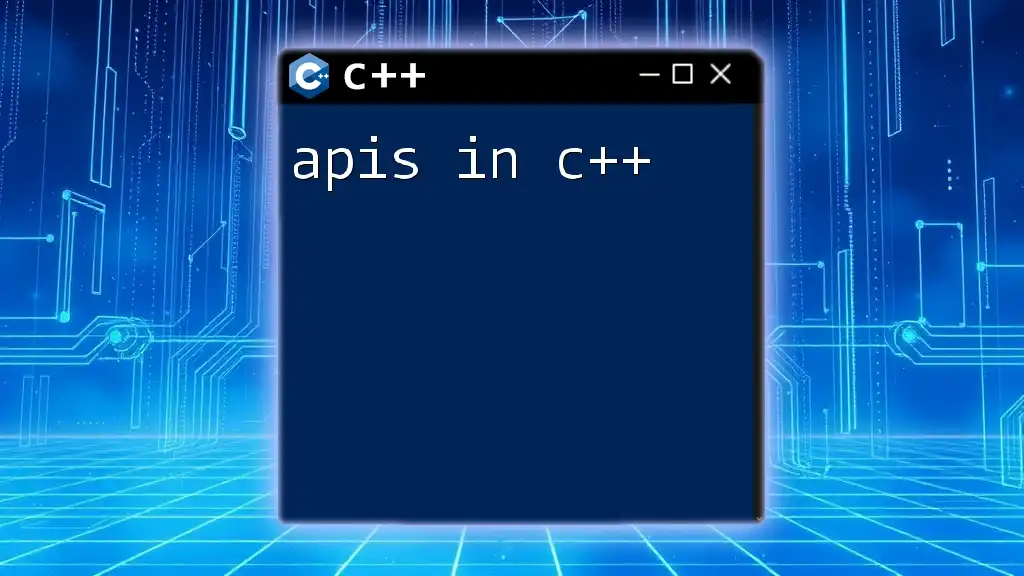
Advantages of Aliases
Improved Code Readability
One of the primary advantages of using aliases in C++ is the improved readability of your code. Well-placed aliases can make your intentions much clearer. For instance, instead of using cumbersome and complex type names throughout your code, consider employing aliases that succinctly describe what each type represents.
A trivial comparison reinforces this point. Consider the following:
unsigned long long age;
versus using an alias:
using Years = unsigned long long;
Years age;
The latter version instantly conveys that `age` is meant to represent a duration in years, enhancing understanding for anyone reading the code.
Simplification of Complex Types
Aliases can dramatically reduce the complexity of type declarations. This is particularly advantageous when it comes to function pointers or heavily templated types.
Code Example: Alias for Function Pointers
using FuncPtr = void(*)(int);
This alias, `FuncPtr`, abstracts away the complexity of function pointer syntax. Now, when you want to declare a function pointer, you can simply use `FuncPtr` instead of the more convoluted syntax.
Facilitating Code Maintenance
Another significant benefit of using aliases is their ability to facilitate easier code maintenance over time. For instance, if a type needs to be changed (e.g., switching from `unsigned long` to `uint64_t`), using aliases means you only need to update the alias definition itself rather than every instance throughout your codebase.
For example, in multiple files or sections of your codebase, if you used `typedef unsigned long ulong;` and later decided to change it to `typedef uint64_t ulong;`, the change will immediately reflect everywhere `ulong` is used. This reduces errors and improves code quality.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Practical Applications of Aliases
In API Development
Aliases play a crucial role in API development, where clarity and ease of use are vital for ensuring good developer experience. For example, if you're designing a library, you can use aliases to create cleaner interfaces.
Code Example: API Alias Use
using Callback = void(*)(int, const std::string&);
In this instance, `Callback` provides a clear signal to users about what type of function is expected, fostering a more intuitive interaction with the API.
In Template Programming
Aliases can hugely enhance code clarity in template programming. As templates can often involve complex type definitions, using aliases can simplify how we represent these types.
Code Example: Template with Aliases
template<typename T>
using Vec = std::vector<T>;
Vec<int> myInts; // Now myInts is a std::vector<int>
This simplification leads to more maintainable and readable template code, making it easier for developers to grasp what types are being dealt with.
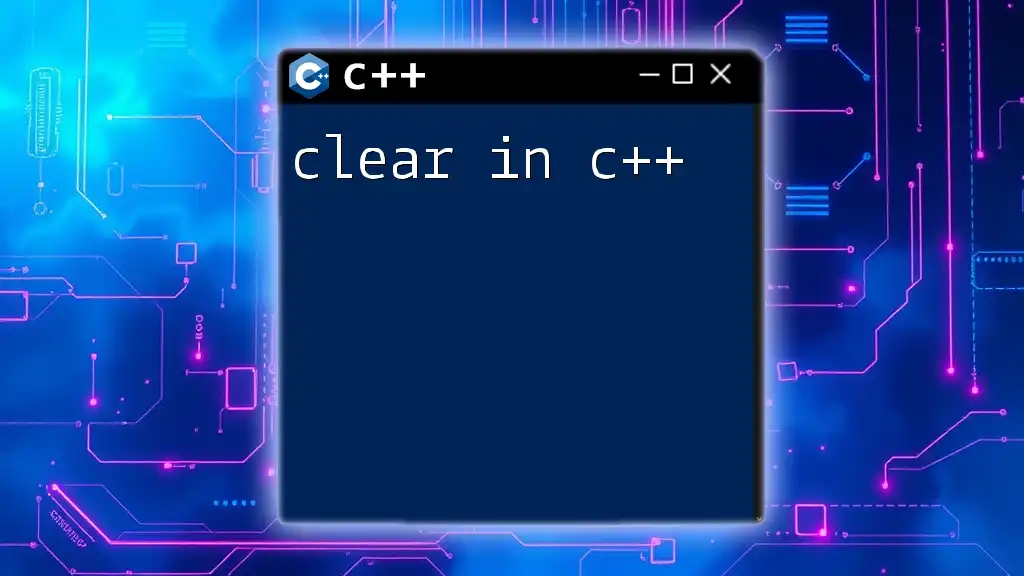
Common Mistakes and How to Avoid Them
Despite their benefits, aliases can lead to misunderstandings if not used carefully. Common pitfalls include overusing aliases, which can lead to confusion. When aliases are too abstract or loosely tied to their original type, they can become a source of ambiguity. Best practices include keeping your aliases descriptive and relevant to their purpose in the code.
Misusing aliases and potential pitfalls
Some developers may abuse aliasing by creating aliases for every type, no matter how trivial, resulting in a codebase filled with cryptic names that obfuscate the underlying types. As a guideline, use aliases when:
- The original type is cumbersome or complex.
- The alias conveys a meaningful context that improves understanding.
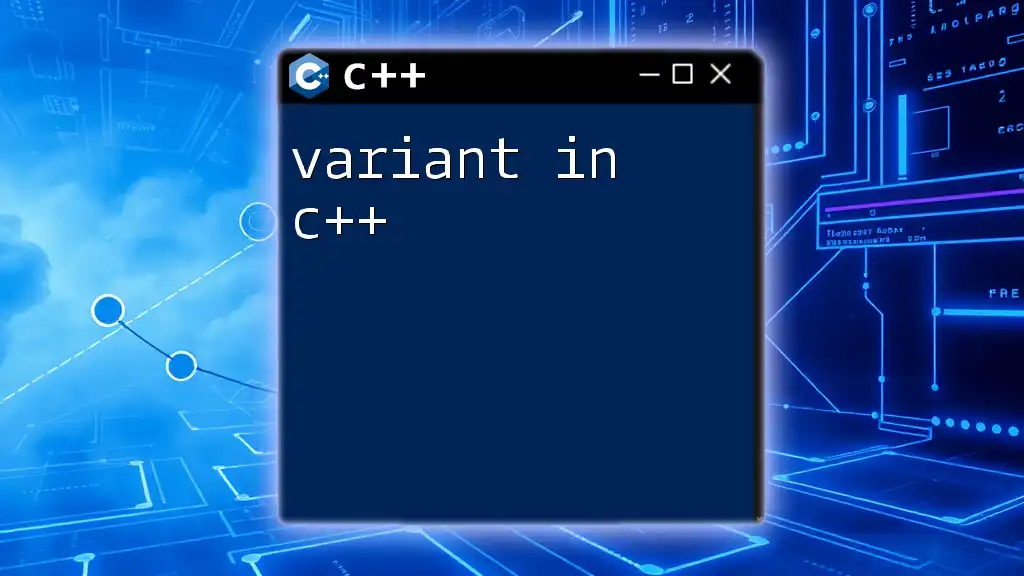
Conclusion
In conclusion, understanding the concept of alias in C++ is essential for any programmer seeking to create clean, maintainable, and easily readable code. By employing `typedef` and `using`, you can enhance both the clarity and simplicity of your code. The practical applications of aliases, from API development to template programming, showcase their versatility and the significant improvements they can bring to code management.
Encouragement is in order—try integrating aliases in your next C++ project, and experience firsthand how they can streamline your coding practices.
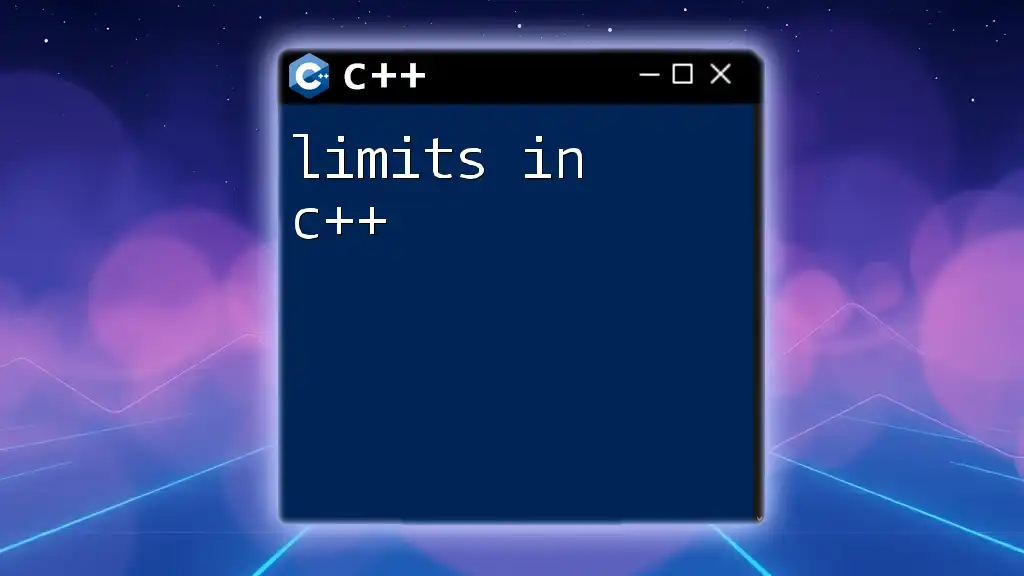
Additional Resources
For further reading and deeper insights into aliases in C++, consider exploring articles, documentation, and online forums dedicated to advanced C++ programming. These resources can provide valuable perspectives and enhance your understanding of effective coding strategies.
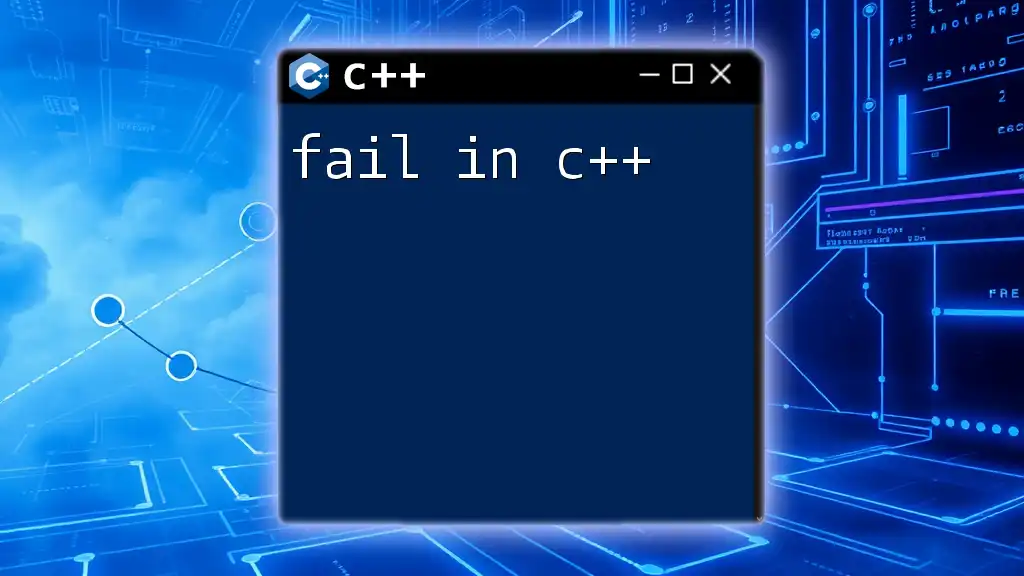
FAQ Section
What is the difference between typedef and using in C++?
The main difference between `typedef` and `using` lies in their syntax and usability. `typedef` has been part of C++ for a longer time, while `using` is considered more modern and flexible. Notably, `using` is often easier to read, especially for complex types and templates.
Can aliases be used with template types?
Yes! Aliases can be particularly beneficial in template programming, allowing for more readable and manageable code. The `using` directive shines in this area, enabling developers to define clear and concise type aliases for templates.
Are there performance implications with using aliases?
Generally, aliases do not impact performance in C++. They are merely alternate names for types and do not add any runtime overhead. However, they do improve code readability and maintainability, making your code easier to work with and less error-prone.