An ArrayList in C++ can be implemented using the `std::vector` class from the Standard Template Library (STL) for dynamic array functionality that allows resizing and easy manipulation of elements.
Here's a simple code snippet demonstrating how to use an `std::vector` as an ArrayList:
#include <iostream>
#include <vector>
int main() {
std::vector<int> arrayList; // Creating an ArrayList
// Adding elements
arrayList.push_back(10);
arrayList.push_back(20);
arrayList.push_back(30);
// Accessing elements
for (int i = 0; i < arrayList.size(); i++) {
std::cout << arrayList[i] << " ";
}
return 0;
}
What is an ArrayList in C++?
An ArrayList in C++ is a dynamic array structure that allows for flexible resizing and easy manipulation of data elements. Unlike standard arrays in C++, which have a fixed length, an ArrayList offers the advantage of growing and shrinking in size as needed. This dynamic behavior makes the ArrayList a useful tool in scenarios where the exact number of data elements cannot be predetermined.
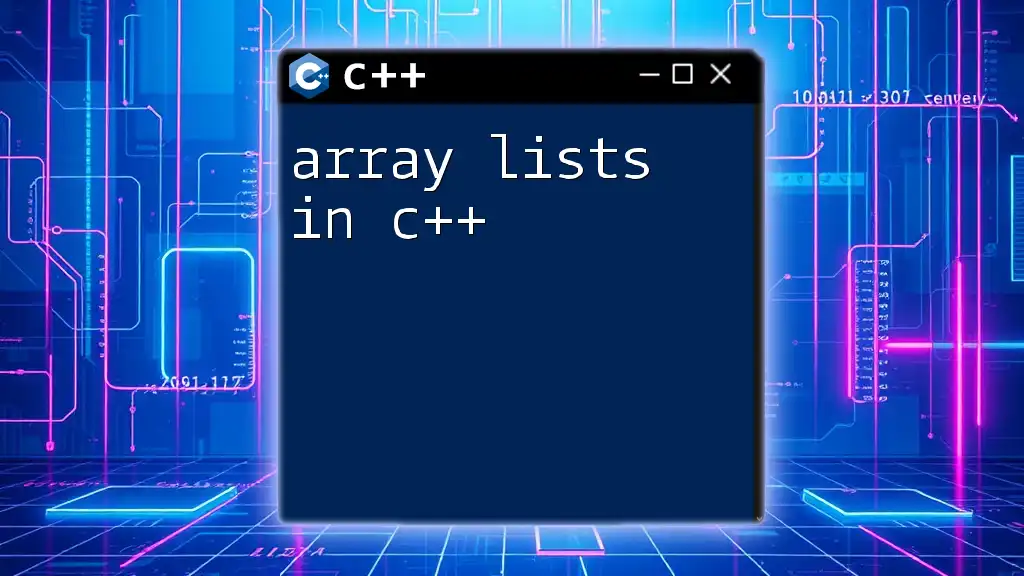
Why Use ArrayList in C++?
Using an ArrayList provides several advantages:
- Dynamic Sizing: The ArrayList can automatically expand or contract according to the number of elements added or removed.
- Ease of Insertion and Deletion: Adding or removing elements can be done efficiently without the need to allocate new memory every time.
- Familiar Syntax: The interface can be similar to that of standard arrays, maintaining readability.
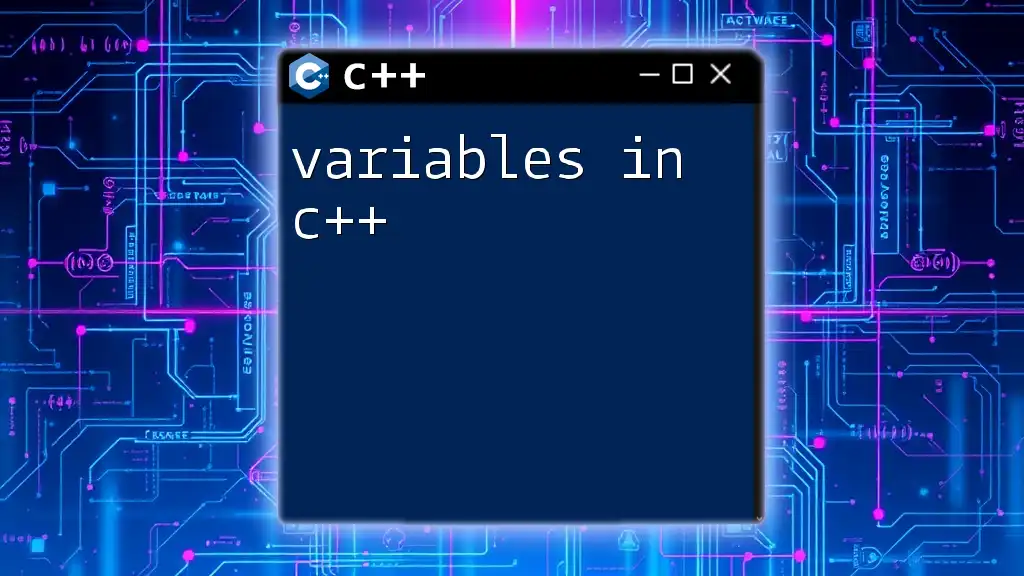
Understanding C++ Standard Library Containers
In C++, the Standard Library offers a variety of containers that can be used to manage collections of data. The most commonly used ones include:
- Vectors: Implemented as `std::vector`, this container is a dynamic array that automatically resizes itself as elements are added or removed.
- Lists: Implemented as `std::list`, this is a doubly linked list that allows for efficient insertions and deletions.
C++ ArrayList vs. C++ Vector
While the ArrayList is a custom implementation, `std::vector` is a pre-built solution provided by the Standard Library. Here are some key differences:
- Performance: `std::vector` is highly optimized for performance and often includes sophisticated memory management.
- Functionality: Vectors come with a complete set of functionalities, such as range checks and iterators, that simplify usage.
When using dynamic arrays or lists, it is generally advisable to use `std::vector` due to its robustness unless specific use cases necessitate a custom ArrayList.
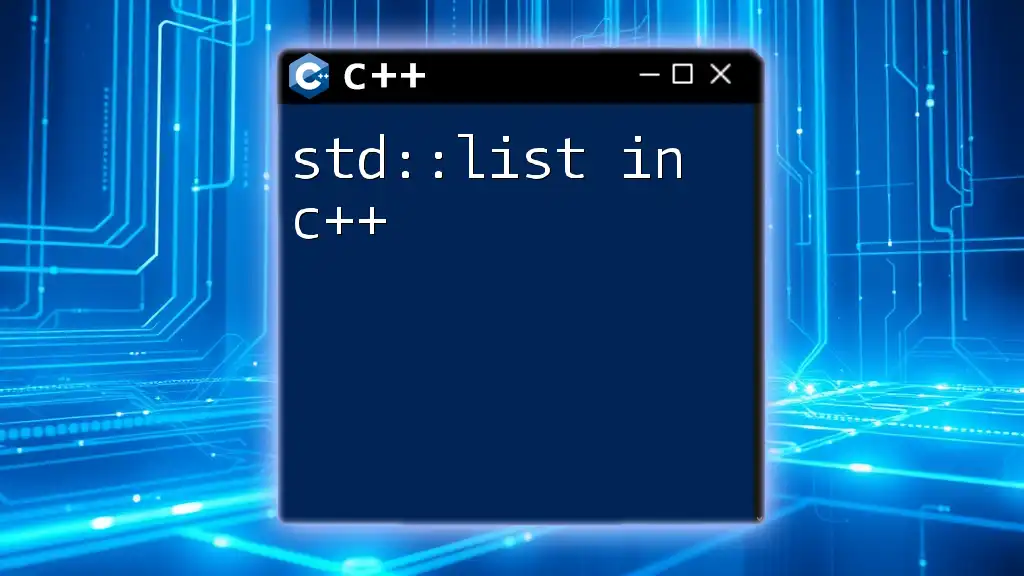
Implementing ArrayList in C++
Creating your own ArrayList involves building a class that manages an array and its operations.
Basic Structure of the Class
The core functionalities of the ArrayList will include methods for adding, removing, and accessing elements.
Member Variables
You will need the following member variables in your ArrayList class:
- Size: Current number of elements stored in the ArrayList.
- Capacity: Maximum number of elements the array can hold before resizing.
- Pointer to the underlying array: A dynamic array to store the list’s elements.
Constructor and Destructor
The constructor is responsible for initializing the underlying array, while the destructor frees the allocated memory.
template<typename T>
class ArrayList {
private:
T* arr;
size_t size;
size_t capacity;
public:
ArrayList() : size(0), capacity(10) {
arr = new T[capacity];
}
~ArrayList() {
delete[] arr;
}
};
Adding and Removing Elements
To effectively utilize the ArrayList, you must implement methods to add and remove elements.
Implementing the `add` Method
This method checks if the array needs to be resized before adding a new element.
void add(const T& value) {
if (size >= capacity) {
resize();
}
arr[size++] = value;
}
void resize() {
capacity *= 2;
T* newArr = new T[capacity];
for (size_t i = 0; i < size; i++) {
newArr[i] = arr[i];
}
delete[] arr;
arr = newArr;
}
Implementing the `remove` Method
The `remove` method allows you to delete an element by shifting subsequent elements to fill the gap.
void remove(size_t index) {
if (index < size) {
for (size_t i = index; i < size - 1; i++) {
arr[i] = arr[i + 1];
}
size--;
}
}
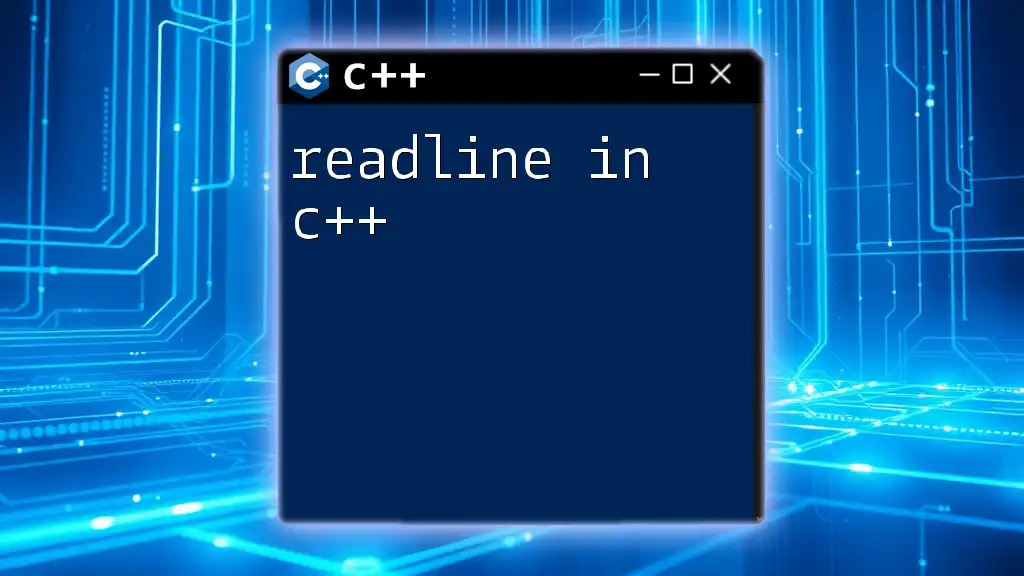
Accessing Elements
To retrieve elements from the ArrayList, implement the `get` method, which includes bounds checking to prevent accessing invalid indices.
T get(size_t index) const {
if (index < size) {
return arr[index];
}
throw std::out_of_range("Index out of range");
}
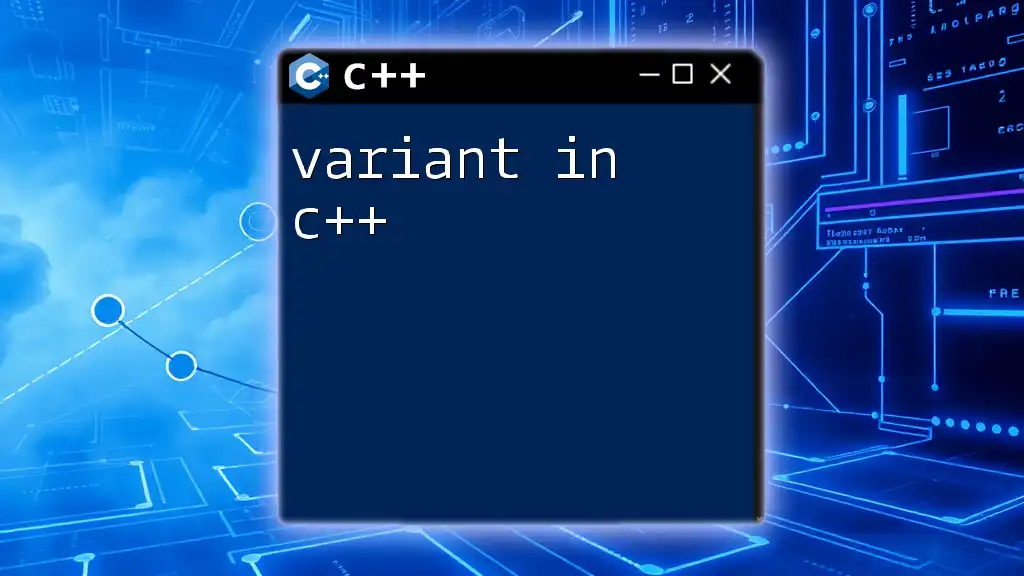
Iterating Through the ArrayList
To make your ArrayList more usable, implement an iterator. This allows users to iterate through elements using standard formatting.
class Iterator {
private:
ArrayList& arrayList;
size_t currentIndex;
public:
Iterator(ArrayList& list) : arrayList(list), currentIndex(0) {}
T& operator*() {
return arrayList.arr[currentIndex];
}
Iterator& operator++() {
currentIndex++;
return *this;
}
bool operator!=(const Iterator& other) {
return currentIndex != other.currentIndex;
}
};
Iterator begin() { return Iterator(*this); }
Iterator end() { return Iterator(*this, size); }
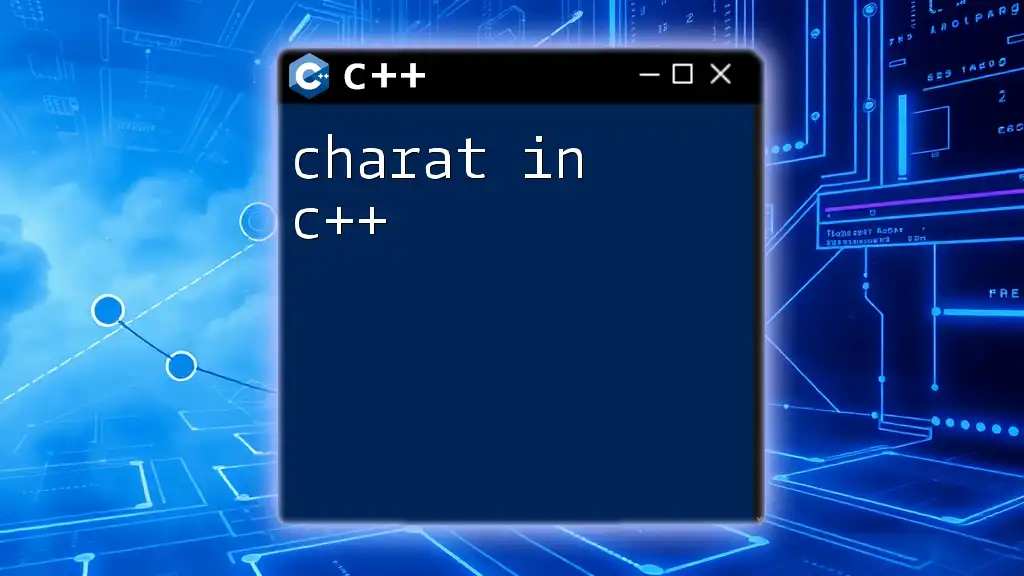
Practical Applications of ArrayList in C++
The ArrayList can serve various real-world applications:
- Dynamic Storage: When you cannot ascertain the number of elements ahead of time, an ArrayList serves as a flexible storage option.
- Buffer Management: It can be used as a buffer where elements keep coming in, and you may want to process or discard them dynamically.
When considering performance, although an ArrayList is sufficient for many applications, using `std::vector` is often more efficient due to optimization by the C++ Standard Library.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Common Pitfalls and How to Avoid Them
While implementing an ArrayList can be straightforward, there are common challenges to consider.
Memory Management Issues
One of the primary concerns is managing memory correctly. Be conscious of deep vs. shallow copies. A shallow copy could lead to multiple references to the same dynamic array, resulting in undefined behavior when one is deleted.
Dynamic Resizing Concerns
Dynamic resizing can lead to performance bottlenecks, especially if done frequently. It is wise to increase the capacity exponentially (e.g., doubling) rather than linearly to minimize the number of resizing operations.
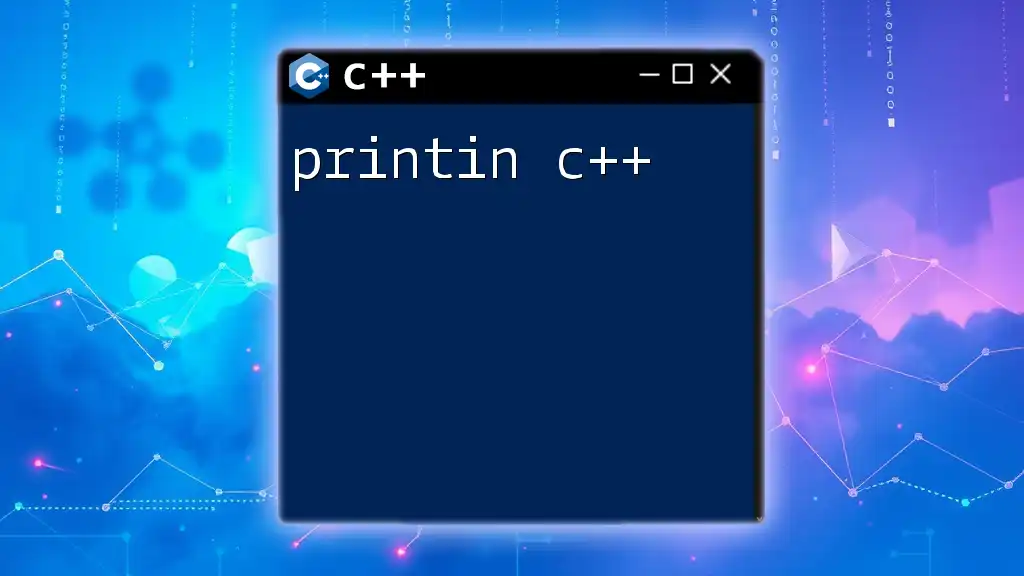
Conclusion
In summary, the ArrayList in C++ provides a powerful way to manage dynamic arrays of data without the constraints of fixed sizes. Whether you choose to implement your own ArrayList or utilize `std::vector` depends on your application’s specific needs. For most situations, relying on the Standard Library is advisable unless special circumstances justify building a custom solution.
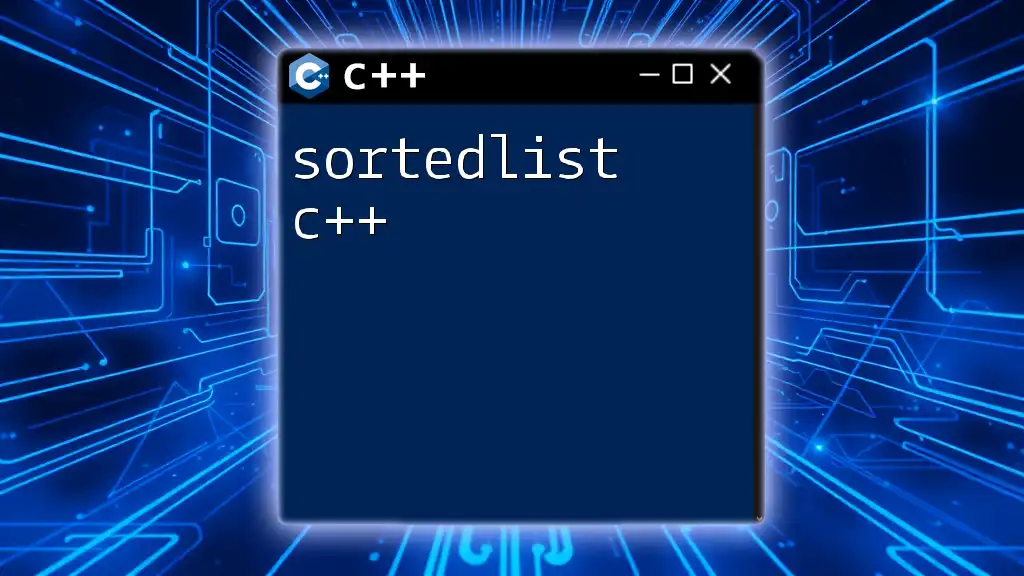
Related Topics
For further exploration, consider learning about other C++ data structures, such as `std::list` and `std::deque`. Each has its unique benefits and use cases, providing various options for effective data management in C++.