In C++, graphics can be implemented using libraries like SFML or SDL to create visual applications and games efficiently. Here's a simple example using SFML to create a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear(sf::Color::Black);
window.display();
}
return 0;
}
Understanding Graphics Libraries
Graphics libraries are essential tools for developers looking to create visually appealing applications. They provide ready-made functions and classes that handle complex rendering tasks, saving time and effort.
Popular Graphics Libraries for C++
SFML (Simple and Fast Multimedia Library)
SFML is a popular choice for C++ graphics due to its simplicity and ease of use. It provides a high-level interface for 2D graphics, while also supporting audio, network, and input handling.
To get started with SFML, you can download it from the official website and follow the installation instructions relevant to your operating system. A simple code snippet to create a basic SFML window is as follows:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Graphics");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
SDL (Simple DirectMedia Layer)
SDL is another robust graphics library that allows developers to create cross-platform applications with access to graphics, audio, and input devices. Setting up SDL involves downloading the library and linking it to your project.
Below is a simple example of an SDL program that opens a window:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("SDL Graphics", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, 0);
SDL_Event event;
bool running = true;
while (running) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
running = false;
}
}
}
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
OpenGL
OpenGL is a widely-used graphics API for rendering 2D and 3D graphics. It's a low-level graphics library that gives developers an immense amount of control over rendering. Integration with C++ can be done through libraries such as GLEW and GLFW.
Here’s a basic OpenGL program that sets up a window and renders a colored triangle:
#include <GL/glew.h>
#include <GLFW/glfw3.h>
// Vertex data for a triangle
GLfloat vertices[] = {
0.0f, 0.5f, 0.0f,
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f
};
int main() {
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL Graphics", NULL, NULL);
glfwMakeContextCurrent(window);
glewInit();
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
// Drawing the triangle
glEnableClientState(GL_VERTEX_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, vertices);
glDrawArrays(GL_TRIANGLES, 0, 3);
glDisableClientState(GL_VERTEX_ARRAY);
glfwSwapBuffers();
glfwPollEvents();
}
glfwTerminate();
return 0;
}
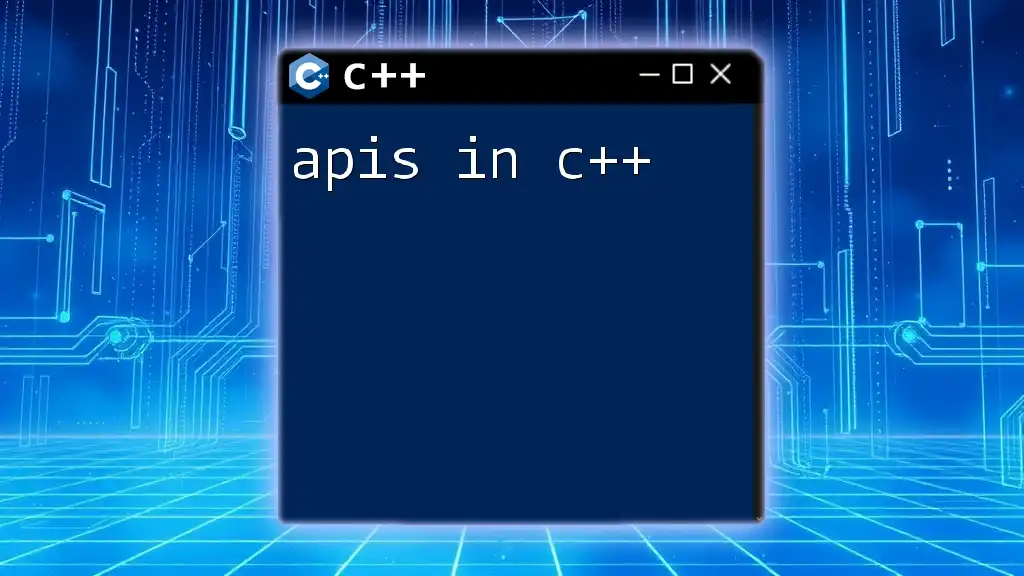
Setting Up Your C++ Graphics Environment
Choosing the right Integrated Development Environment (IDE) can greatly enhance your development experience. Recommended IDEs for C++ graphics programming include Visual Studio, Code::Blocks, and CLion.
Installing necessary libraries is crucial for getting started with graphics in C++. Here's a quick guide on how to do that:
- SFML: You can install SFML using package managers like vcpkg or manually by downloading it from the official website.
- SDL: Similar to SFML, SDL can be installed through package managers or by obtaining it directly from their website.
- OpenGL: OpenGL is often included with modern graphics drivers. For a full experience, consider using GLEW to manage OpenGL extensions, and GLFW for window and context management.
# Example installation commands for **Linux**
sudo apt-get install libsfml-dev
sudo apt-get install libsdl2-dev
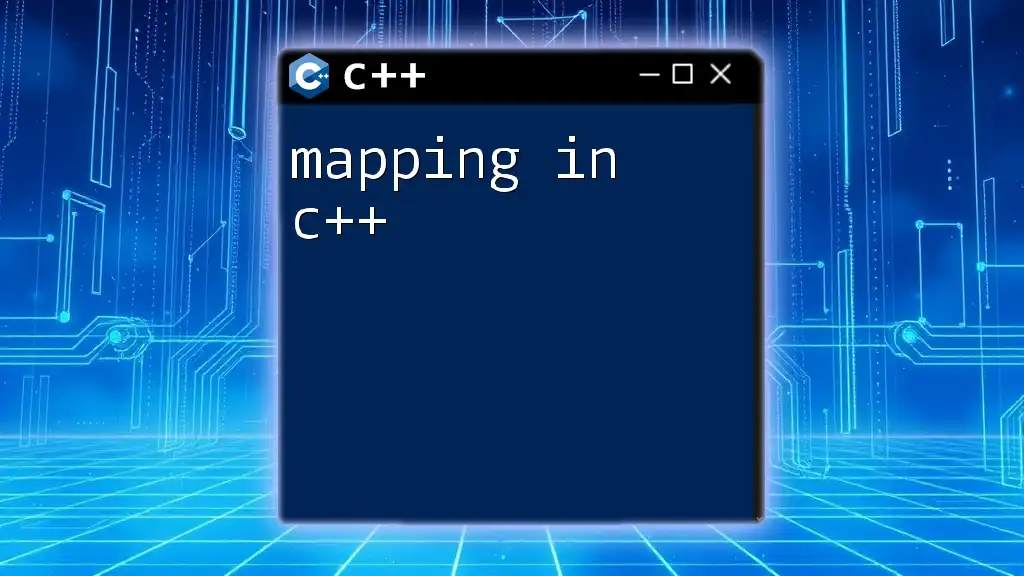
Basic Concepts in C++ Graphics
Understanding Coordinate Systems
In graphics programming, understanding coordinate systems is fundamental. Most libraries adopt a Cartesian coordinate system, where the origin (0,0) is typically at the top-left corner of the window or viewport. Positive X values extend to the right, and positive Y values descend downward.
Drawing Shapes
2D Shapes
Creating basic 2D shapes like circles and rectangles is straightforward in graphics libraries. Using SFML, you can easily draw a circle by initializing a `sf::CircleShape`:
sf::CircleShape circle(50); // Radius of 50
circle.setFillColor(sf::Color::Green);
circle.setPosition(100, 100);
window.draw(circle);
3D Shapes
3D graphics involve additional complexity. Basic shapes like cubes can be created by defining their vertices and rendering them using modern OpenGL techniques. A simple cube setup in OpenGL requires defining a vertex array and using transformation matrices to position and manipulate it.
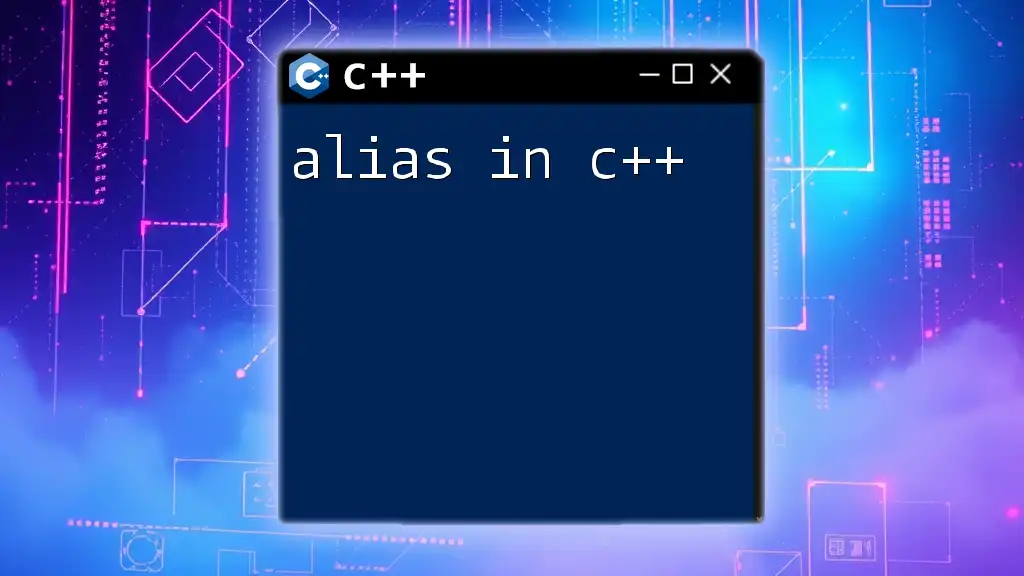
Creating Graphics Applications
Designing a Basic Graphics Application
When creating graphics applications, structuring your code is vital. A typical application involves initializing the graphics library, creating a main loop, and handling events. Here’s a simple animation loop in SFML:
sf::Clock clock;
while (window.isOpen()) {
sf::Time deltaTime = clock.restart();
// Update objects based on deltaTime
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Draw your objects here
window.display();
}
Handling User Input
Interactivity is crucial for graphics applications. Handling keyboard and mouse input allows users to interact with your application dynamically. Here’s an example of keyboard event handling in SDL:
while (SDL_PollEvent(&event)) {
if (event.type == SDL_KEYDOWN) {
switch (event.key.keysym.sym) {
case SDLK_ESCAPE:
running = false; // Exit the application
break;
}
}
}
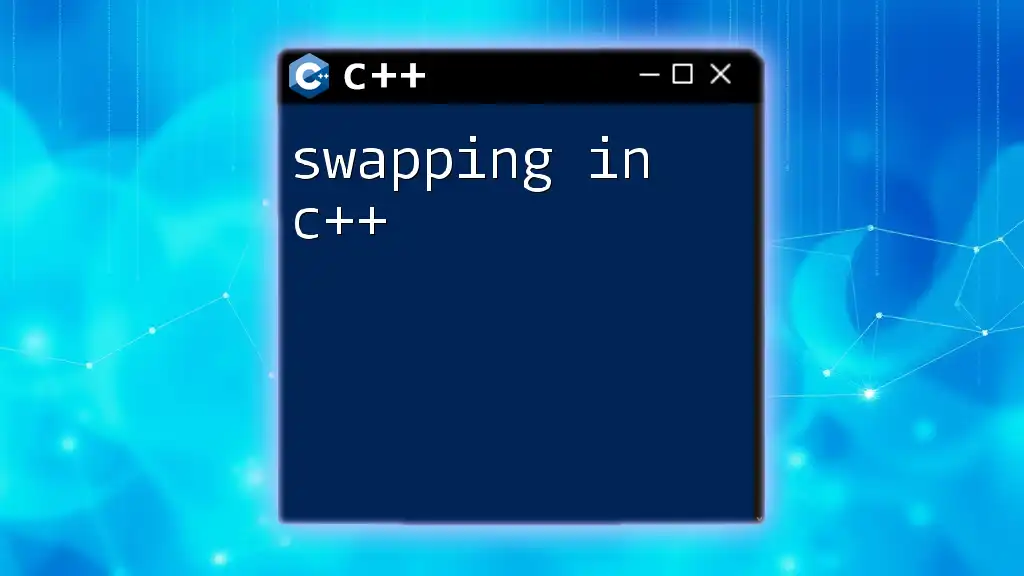
Working with Textures and Images
Loading and Displaying Textures
Textures add richness to your graphics, allowing you to use images instead of plain colors. In SFML, loading and displaying a texture is simple:
sf::Texture texture;
if (!texture.loadFromFile("image.png")) {
// Handle error
}
sf::Sprite sprite(texture);
window.draw(sprite);
Animations with Sprites
Sprites facilitate sprite-based animations by changing their visuals over time. This can be done by cycling through a set of textures:
std::vector<sf::Texture> frames;
frames.push_back(texture1);
frames.push_back(texture2);
// ...
sprite.setTexture(frames[currentFrame]);
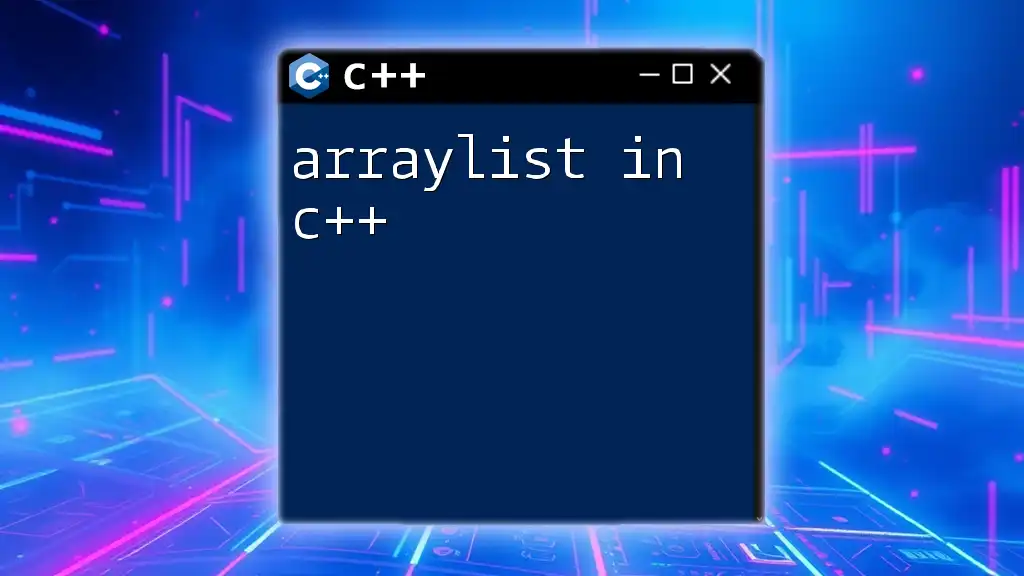
Advanced Graphics Techniques
Exploring 2D Lighting and Shadowing
Adding lighting and shadowing effects can elevate your 2D graphics. One method involves drawing semi-transparent shapes over regions to create shadow effects visually.
Introduction to 3D Graphics
Transitioning from 2D to 3D graphics involves understanding concepts like depth, perspective, and camera manipulation. A basic introduction to 3D involves learning about the rendering pipeline and how to manage 3D models.
Shader Programming
Shaders are programs that run on the GPU, allowing for custom rendering effects. A fundamental vertex and fragment shader might look like this:
// Vertex Shader
#version 330 core
layout(location = 0) in vec3 position;
void main() {
gl_Position = vec4(position, 1.0);
}
// Fragment Shader
#version 330 core
out vec4 color;
void main() {
color = vec4(1.0, 0.5, 0.0, 1.0); // Orange color
}
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Performance Optimization in Graphics Programming
Common Performance Bottlenecks
Understanding performance issues is essential when developing graphics applications. Common bottlenecks include high draw calls, CPU-GPU synchronization, and inefficient memory use.
Techniques to Optimize Code
To optimize your graphics code, consider batching draw calls, utilizing object pooling, and minimizing state changes. A simple performance improvement technique in OpenGL is to use buffer objects to store vertex data:
GLuint VBO;
glGenBuffers(1, &VBO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
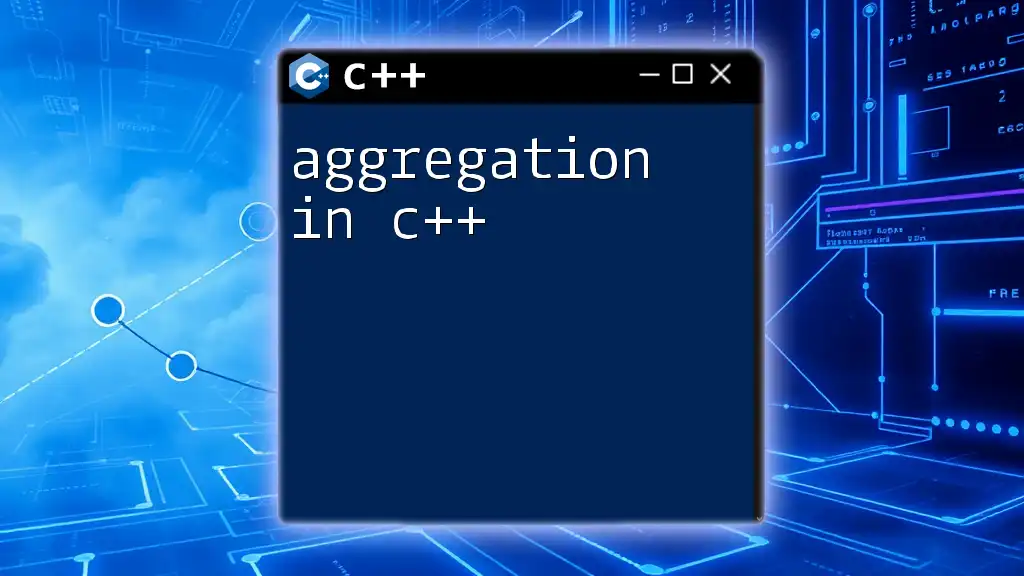
Conclusion
As you delve deeper into graphics in C++, you will discover a world of possibilities. With powerful libraries and various techniques at your disposal, enhancing your applications with stunning visuals is within reach. The future of graphics programming is bright, and as technologies evolve, continuous learning is essential. Expand your skills with various resources such as online courses, books, and community forums dedicated to graphics programming.
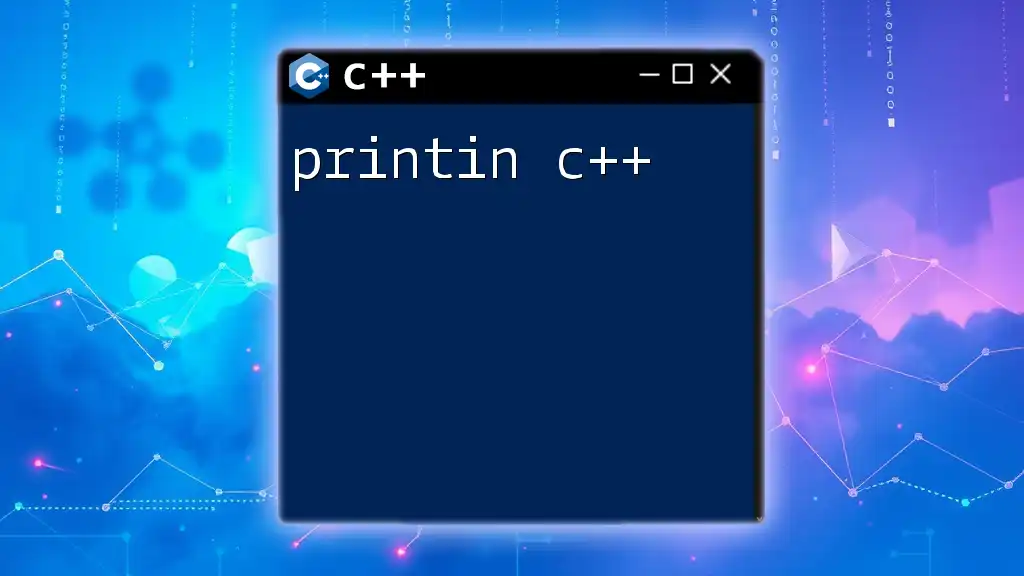
Call to Action
Join our community to enhance your skills in C++ graphics! Sign up for our courses, participate in discussions, and keep yourself updated with the latest trends in graphics programming. Your journey in graphics begins now!