"Practical C++ focuses on applying core C++ principles and commands to solve real-world problems efficiently and effectively."
Here's a simple code snippet showcasing how to create a basic function and use it in C++:
#include <iostream>
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 3);
std::cout << "The sum is: " << result << std::endl;
return 0;
}
Setting Up Your C++ Environment
To begin your journey with practical C++, it’s crucial to set up your development environment effectively. The setup process involves a few key steps, starting with the installation of a C++ compiler.
Installing a C++ Compiler
A compiler is a program that converts your C++ code into executable files. Some of the most popular compilers include:
- GCC (GNU Compiler Collection): Widely used in Unix/Linux environments.
- Clang: Known for its speed and performance, ideal for macOS systems.
- MSVC (Microsoft Visual C++): The go-to compiler for Windows users, integrated into Visual Studio.
To install GCC on a Linux distribution, you can use:
sudo apt install g++
For Windows, you can use MinGW or install Visual Studio, which comes with MSVC.
Choosing an Integrated Development Environment (IDE)
An IDE provides you with tools to write, debug, and compile your code. Here are some popular options:
- Visual Studio: Highly recommended for its powerful features and user-friendly interface. The Community Edition is free to use.
- Code::Blocks: A simple and lightweight option, highly configurable.
- CLion: A paid IDE by JetBrains that offers excellent features for large projects.
To set up Visual Studio for C++:
- Download the installer from the official website.
- During installation, select the "Desktop development with C++" workload.
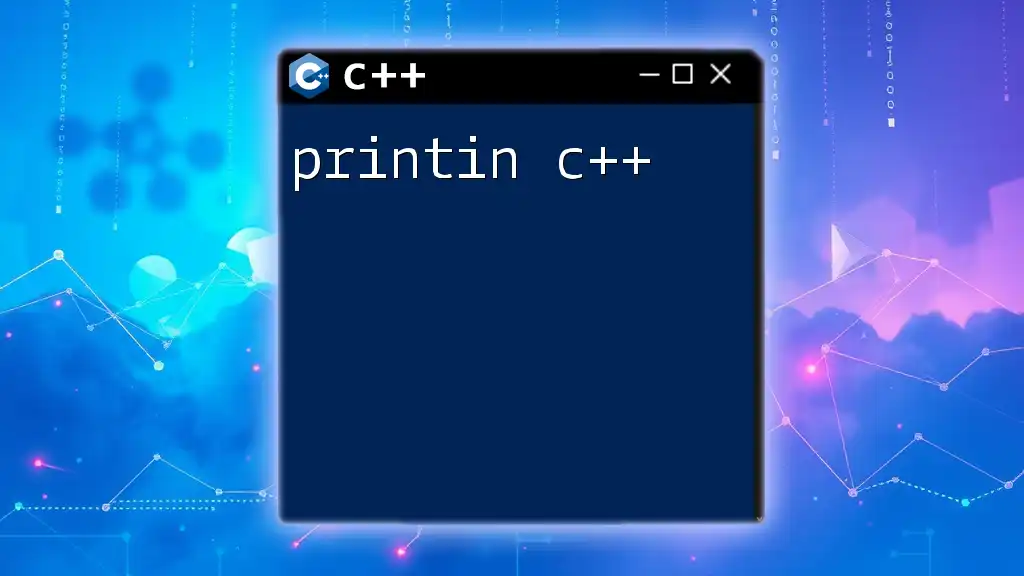
Understanding Basic Syntax and Structure
The syntax of C++ is crucial for writing efficient code. Understanding the foundational elements sets the stage for more complex concepts.
Basic C++ Program Structure
Every C++ program has a basic structure. Here’s a simple example with the classic “Hello, World!” program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `#include <iostream>` is a directive that includes the standard input-output stream library. The `main()` function is the entry point of your program.
Data Types and Variables
C++ has several fundamental data types that you should know:
- int: Represents integers.
- float: Represents floating-point numbers.
- char: Represents single characters.
- string: Represents a sequence of characters (from the `<string>` library).
Declaring and initializing variables is straightforward:
int age = 30;
float salary = 75000.50;
char initial = 'A';
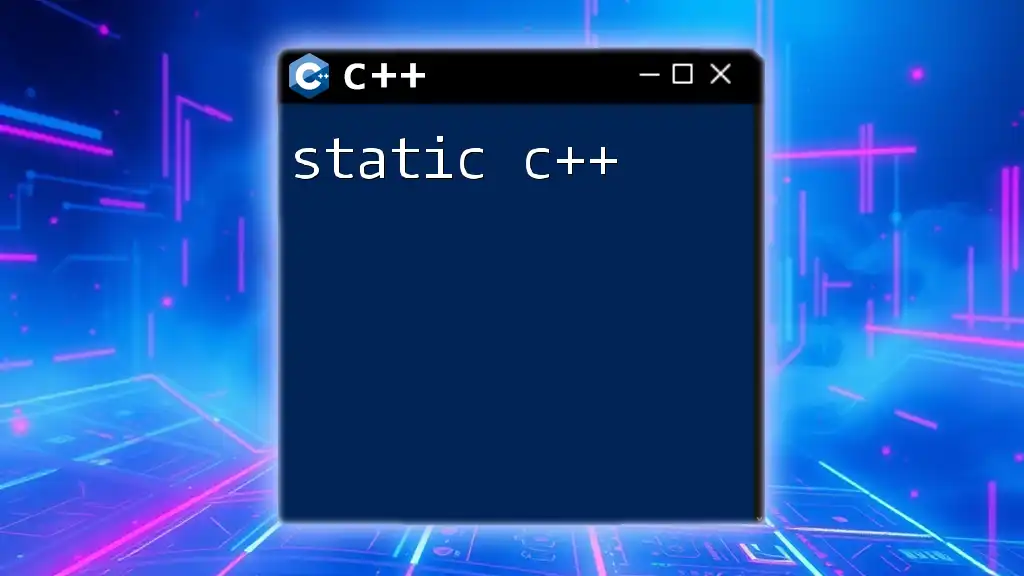
Control Structures
Control structures dictate the flow of your program. They allow you to execute certain blocks of code based on specific conditions.
Conditional Statements
The common types of conditional statements include `if`, `else if`, and `else`. Here’s an example of using conditional statements to check if someone is an adult:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Loops and Iteration
Loops are used to repeat a block of code multiple times. Here are the three main types of loops:
-
For Loop: Best for situations where the number of iterations is known.
int numbers[] = {1, 2, 3, 4, 5}; for (int i = 0; i < 5; i++) { std::cout << numbers[i] << " "; }
-
While Loop: Continues until a condition becomes false.
-
Do-While Loop: Ensures the loop is executed at least once.
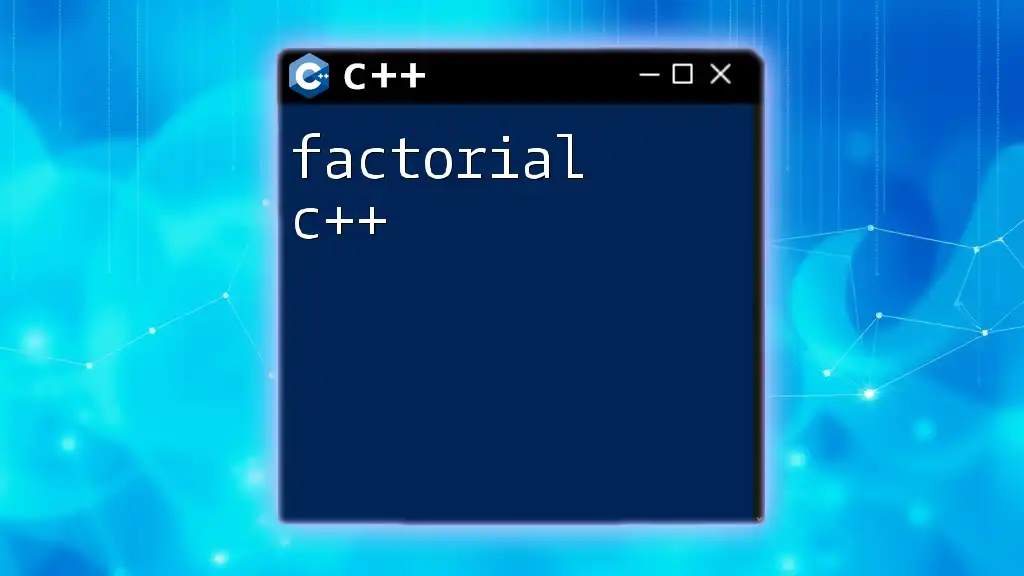
Functions in C++
Functions help organize your code and promote reuse. They allow you to encapsulate logic and call it whenever needed.
Defining and Calling Functions
A function can take parameters and return values. Here’s how you define a simple add function:
int add(int a, int b) {
return a + b;
}
To call the function, you can do:
int result = add(5, 3);
std::cout << "The sum is: " << result << std::endl;
Function Overloading
C++ supports function overloading, which means you can have multiple functions with the same name but different parameters. Here’s an example with an overloaded `add` function:
double add(double a, double b) {
return a + b;
}
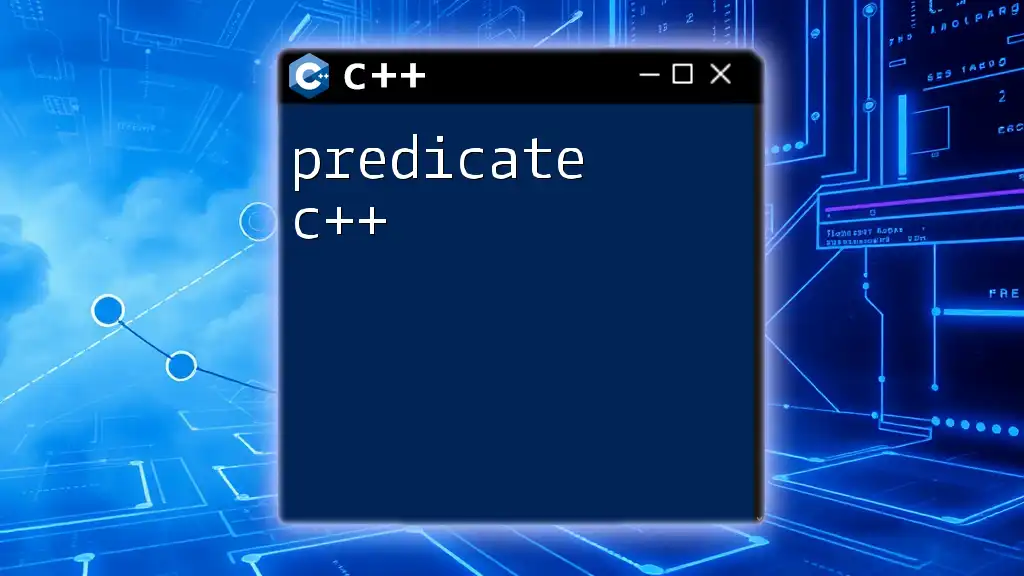
Object-Oriented Programming Concepts
C++ is an object-oriented programming language, allowing developers to create programs based on real-world entities.
Classes and Objects
A class serves as a blueprint for creating objects. Here's a simple example:
class Car {
public:
void drive() {
std::cout << "Driving" << std::endl;
}
};
Car myCar;
myCar.drive();
In this example, `Car` is a class with a method `drive()`. You create an object `myCar` from the class and call its method.
Inheritance and Polymorphism
Inheritance allows you to create new classes based on existing ones. For instance:
class Vehicle {
public:
void start() {}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Ring Ring!" << std::endl;
}
};
In this case, `Bike` inherits from `Vehicle`, allowing it to use methods from the base class while also having its own specific methods.
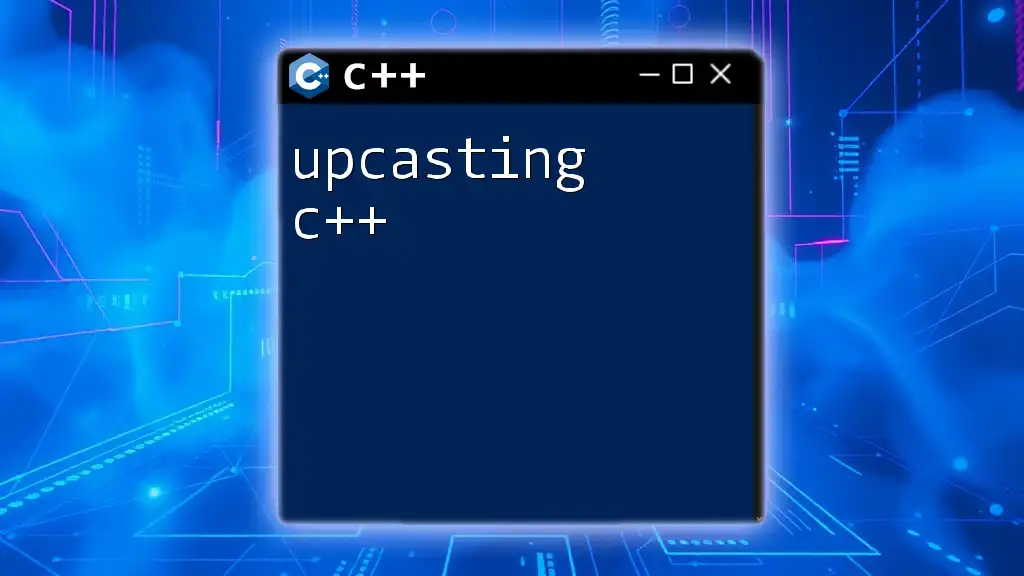
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful set of C++ template classes that provide general-purpose classes and functions.
Introduction to STL
Understanding STL is vital for writing efficient C++ code, as it includes data structures and algorithms that you can use directly in your applications.
Common STL Containers
Some of the most common containers in STL are:
-
Vectors: Dynamic arrays that can resize themselves.
Example of creating and using a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
- Maps: Key-value pair data structure ideal for associative arrays.
#include <map>
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
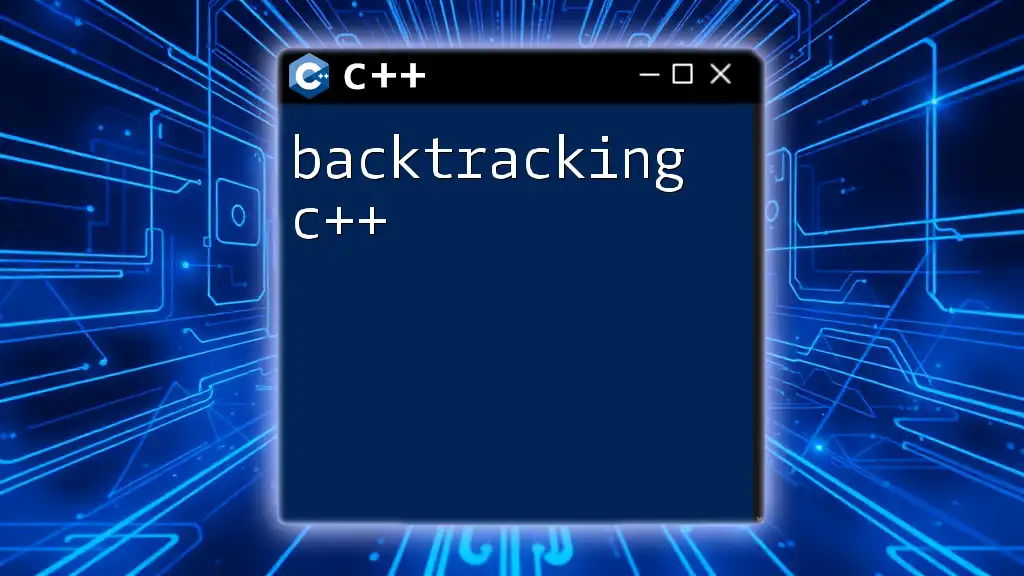
Error Handling in C++
Proper error handling can prevent unexpected crashes in your program. C++ provides exceptions for managing error scenarios efficiently.
Understanding Exceptions
Using exceptions allows you to handle errors without complicating your program's flow. Here's a basic `try-catch` block:
try {
throw std::runtime_error("Something went wrong!");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
In this case, if an exception is thrown, it’s caught in the `catch` block, and the program can handle it gracefully.
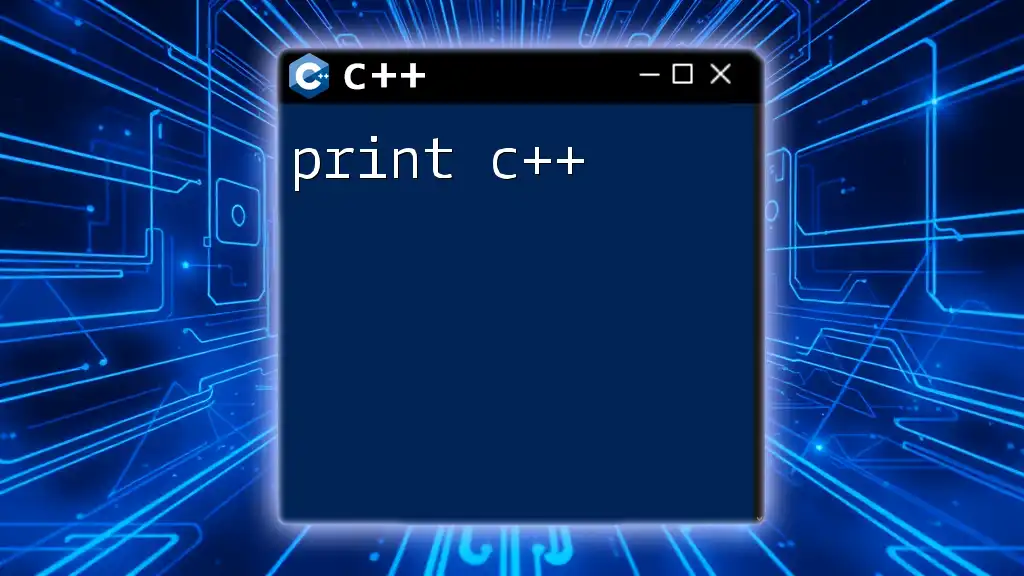
Practical Applications of C++
Applying what you've learned about practical C++ can bring your knowledge to life, allowing you to build useful applications.
Building Simple Applications
Start with small projects, such as a console-based calculator. A simple calculator program can incorporate functions, control structures, and user input.
Working with Files
C++ allows you to read from and write to files, enabling persistent data storage. Here’s a code snippet for file handling:
#include <fstream>
std::ofstream outfile("example.txt");
outfile << "Hello, File!";
outfile.close();
In this example, we create an output file stream, write to a file named `example.txt`, and then close the file to ensure the data is saved properly.
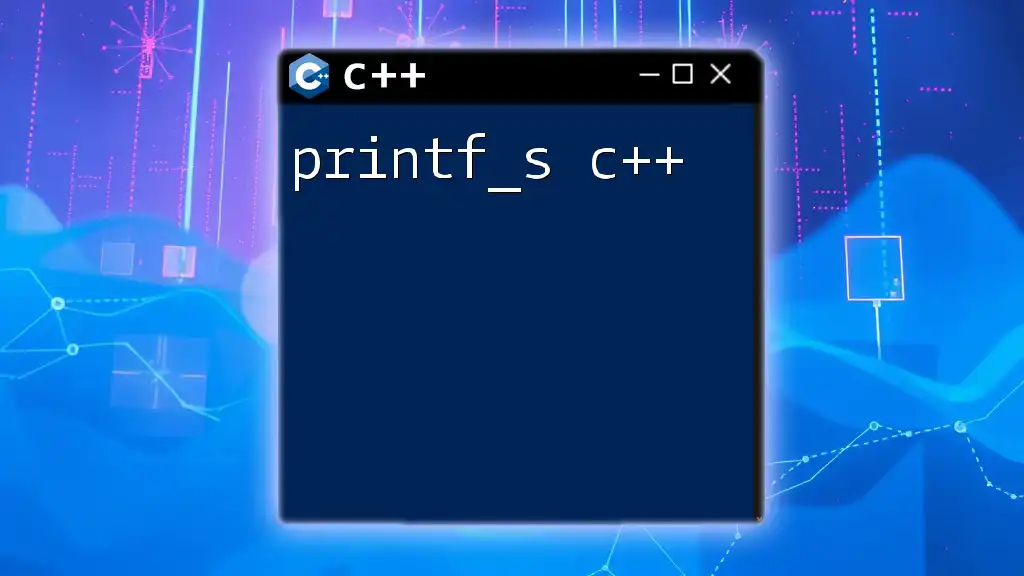
Conclusion
Throughout this guide on practical C++, we've traversed various essential topics, from installation to advanced concepts like object-oriented programming and STL. Each section is geared towards helping you understand and apply C++ effectively. Remember that continuous practice is vital when learning to code.
As you continue your C++ journey, consider exploring further resources, such as books and online courses, to deepen your knowledge. Engage in coding challenges and collaborative projects to solidify your skills in practical C++.