A `multimap` in C++ is a type of associative container that allows storing multiple values for the same key, enabling efficient retrieval and organization of pairs.
#include <iostream>
#include <map>
int main() {
std::multimap<int, std::string> myMultimap;
myMultimap.insert({1, "Apple"});
myMultimap.insert({1, "Avocado"});
myMultimap.insert({2, "Banana"});
for (const auto &pair : myMultimap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Key Features of C++ Multimap
Definition and Characteristics
A multimap in C++ is a type of associative container that allows for the storage of key-value pairs, where multiple keys can have the same value. This supports duplicate keys, making it an essential choice when the relationship between keys and values is many-to-many.
The defining characteristics of a multimap include:
-
Allows Duplicate Keys: Unlike a standard map, which enforces unique keys, a multimap can hold multiple entries with the same key. This feature makes it highly versatile for applications requiring grouped data.
-
Ordered Storage: The elements in a multimap are always stored in a sorted order based on the key. This enables efficient searching and retrieval. By default, the ordering is determined using the `operator<`.
-
Automatic Memory Management: Multimap manages memory automatically, providing conveniences such as dynamic resizing and element destruction.
Syntax of Multimap
To declare a multimap, the syntax is straightforward. It can be defined as follows:
#include <map>
std::multimap<key_type, value_type> multimap_name;
Here, `key_type` represents the data type of the key, while `value_type` denotes the data type of the value.
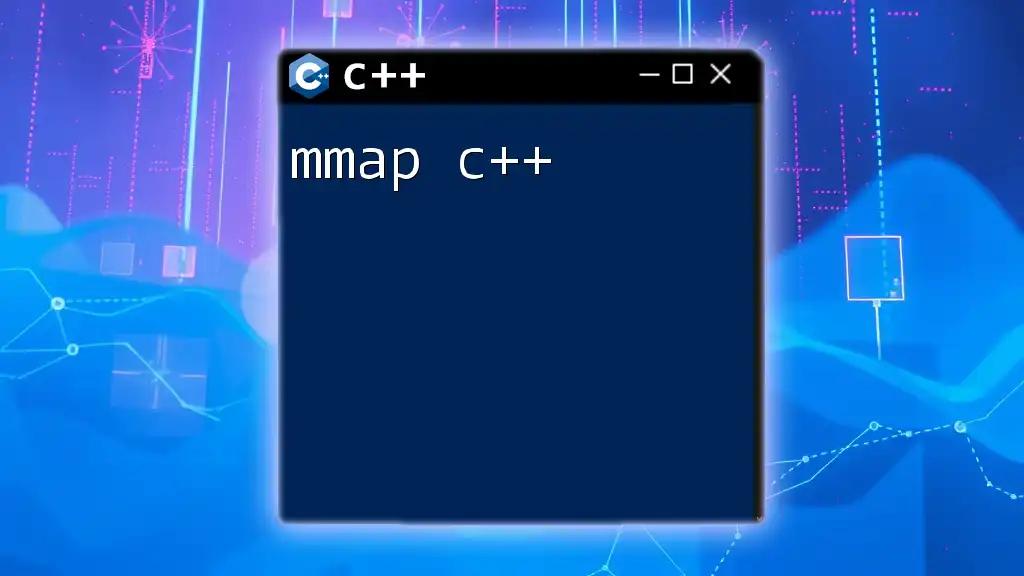
Creating and Initializing a Multimap
Default Constructor
You can declare an empty multimap without any initial elements:
std::multimap<int, std::string> myMultimap;
This initializes `myMultimap` as an empty multimap, ready for insertion.
Inserting Elements
To add elements to a multimap, use the `insert()` function:
myMultimap.insert(std::make_pair(1, "Apple"));
myMultimap.insert(std::make_pair(1, "Avocado"));
With this, you have successfully inserted two entries with the same key (`1`), indicating that both values correspond to the same key.
Initialization with List
Multimaps can also be initialized using an initializer list, which makes the code cleaner and more concise:
std::multimap<int, std::string> myMultimap{
{1, "Apple"},
{2, "Banana"},
{1, "Avocado"}
};
This way, you can define multiple key-value pairs right at the moment of creation.

Accessing Elements in a Multimap
Finding Elements
To search for elements, you can utilize the `find()` and `equal_range()` functions.
Using `find()`, for instance:
auto it = myMultimap.find(1);
if (it != myMultimap.end()) {
std::cout << "Found: " << it->second << std::endl;
}
The `equal_range()` function is particularly useful for retrieving all entries with the same key, yielding a range of iterators:
auto range = myMultimap.equal_range(1);
for (auto i = range.first; i != range.second; ++i) {
std::cout << "Found: " << i->second << std::endl;
}
Iterating Over Multimap
There are multiple approaches to iterate through a multimap. Here’s a simple method using a range-based for loop:
for (const auto& pair : myMultimap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This loop will print each key-value pair, showcasing both the keys and the associated values stored in the multimap.

Modifying Elements in a Multimap
Inserting New Elements
Further entries can be added to an existing multimap, even for duplicate keys. Just call the `insert()` function as described earlier.
Removing Elements
When it comes to removing elements, you can use the `erase()` function. If you want to remove all pairs linked to a particular key, simply do so as follows:
myMultimap.erase(1); // Removes all pairs with key 1
This operation efficiently clears the data associated with that key without the need for extensive searching.

Multimap Use Cases
When to Use Multimap
A multimap is best suited for situations where you need to associate one key with multiple values. Common use cases include:
- Grouping data: If you have records that can belong to several categories.
- Counting occurrences: Useful for tallying items where repeat occurrences are common, like in voting systems or survey responses.
Real-World Applications
Multimaps can be employed in various fields, such as:
- Database records: Group items based on a common attribute, facilitating efficient data retrieval.
- Frequency analysis: In data science, you may analyze how many times different categories appear in a data set.
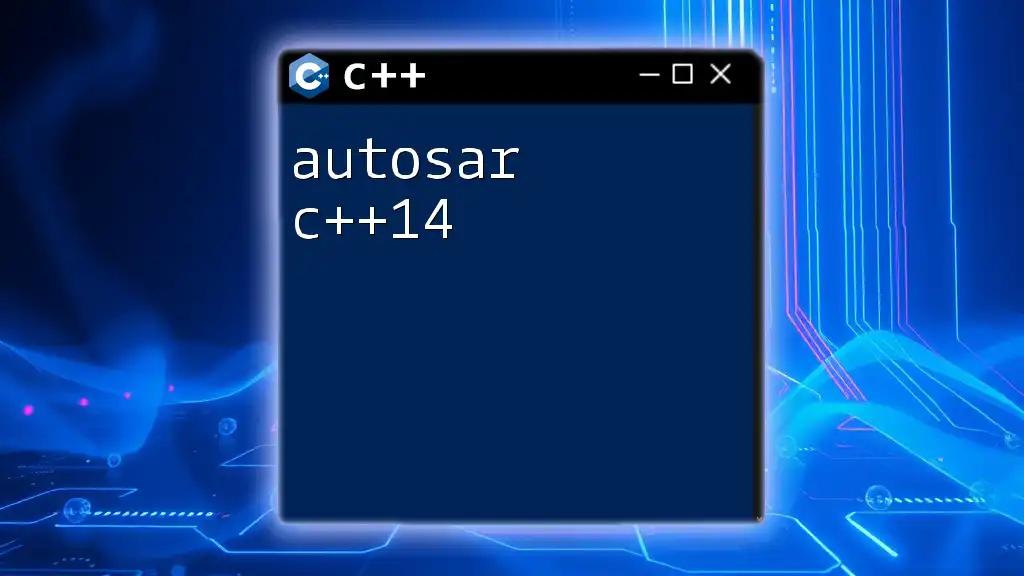
Performance Considerations
Time Complexity
The time complexity for operations in a multimap is generally logarithmic for insertions, deletions, and searches. This efficiency makes multimaps an excellent choice when performance is critical, especially with larger datasets.
Memory Management
Multimap’s ability to manage memory automatically reduces the cognitive load on developers. However, understanding when to utilize custom allocators can help optimize memory usage further for extensive applications.

Conclusion
A multimap in C++ is a powerful and flexible data structure that can effectively handle scenarios where duplicate keys are essential. Its sorted nature, combined with efficient insertion and retrieval, positions it as a great choice for various applications. Experimenting with multimaps is encouraged to fully understand their capabilities and benefits in real-world scenarios.

Additional Resources
For further study and deeper understanding of multimaps in C++, consider exploring the official C++ documentation or enrolling in advanced C++ programming courses that offer practical exercises.
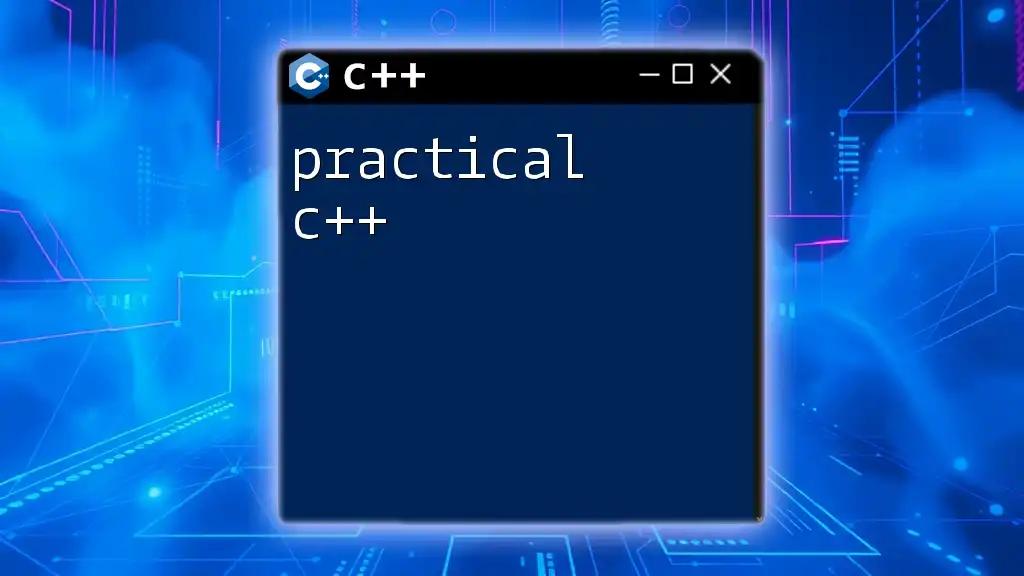
Call to Action
Feel free to share your experiences or questions regarding the use of multimaps in C++. Engaging with a larger community can provide valuable insights and help refine your skills in this powerful programming language.