A mutex (short for "mutual exclusion") in C++ is a synchronization primitive used to manage access to shared resources by multiple threads, ensuring that only one thread can access the resource at a time.
Here’s a simple code snippet demonstrating how to use a mutex in C++:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void printThreadId(int id) {
mtx.lock();
std::cout << "Thread ID: " << id << std::endl;
mtx.unlock();
}
int main() {
std::thread threads[5];
for (int i = 0; i < 5; ++i) {
threads[i] = std::thread(printThreadId, i);
}
for (auto& th : threads) {
th.join();
}
return 0;
}
Understanding Mutex
What is a Mutex?
A mutex (short for mutual exclusion) is a synchronization primitive used in multithreaded programming to prevent concurrent threads from accessing shared resources simultaneously. The primary purpose of a mutex is to ensure that only one thread can access a resource at any one time, thus protecting the resource from potential conflicts and ensuring consistency.
Without the protection of a mutex, issues can arise known as race conditions. A race condition occurs when two or more threads attempt to read from and write to the same resource at the same time, leading to unpredictable results. For instance, if two threads increment the same variable simultaneously without synchronization, the final value may not reflect the intended number of increments.
How Mutex Works
A mutex operates through a mechanism of locking and unlocking. When a thread wants to access a protected resource, it must first lock the mutex associated with that resource. If the mutex is already locked by another thread, the requesting thread will have to wait until the mutex is unlocked. This ensures mutual exclusion, a fundamental principle in concurrency, where only one thread can have access to the resource at any one time.
Visualizing this behavior helps in understanding how thread interactions can either lead to race conditions or be safely managed through mutexes. When the mutex is locked, other threads are blocked from entering the critical section until the mutex is unlocked, thereby safeguarding shared resources.
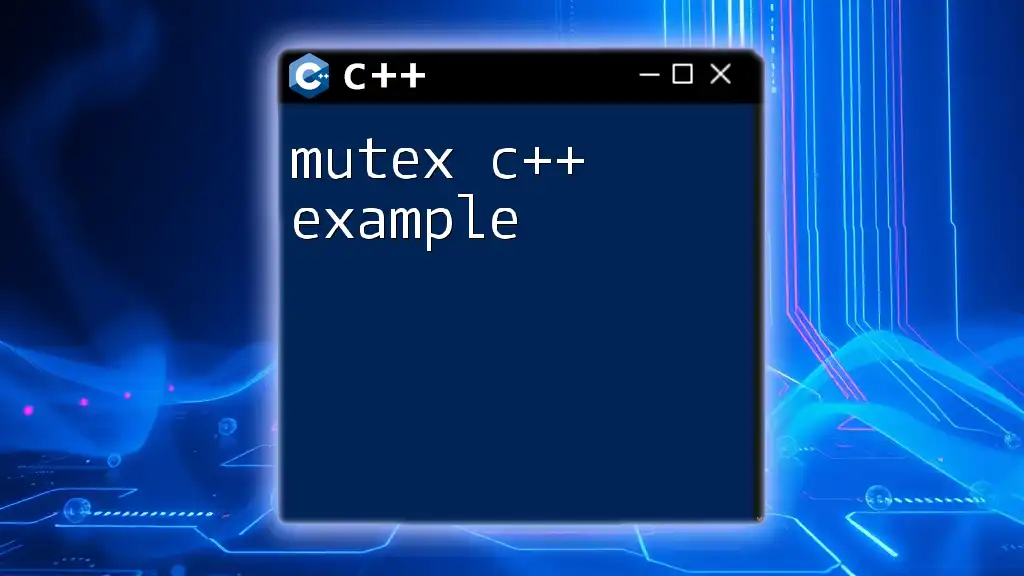
Using Mutex in C++
Including Necessary Header Files
To use mutexes in C++, you need to include the `<mutex>` header, along with `<thread>` and `<iostream>` for concurrent programming functionalities. Here’s how you can do it:
#include <iostream>
#include <thread>
#include <mutex>
Creating and Initializing a Mutex
Creating a mutex is straightforward. You simply declare a mutex object as follows:
std::mutex myMutex;
This line initializes your mutex, which can then be used to control access to shared resources among multiple threads.
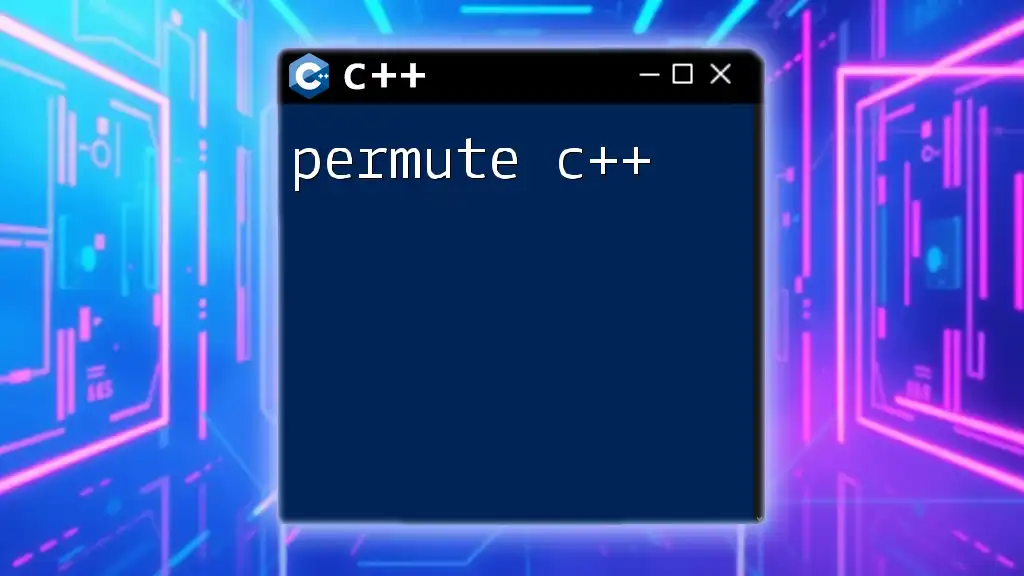
Locking and Unlocking a Mutex
Manual Locking
To manually control access to resources, you can use the `lock()` method of the mutex object when a thread needs to access the resource. After the thread is done, it should use the `unlock()` method to release the mutex. Here’s an example:
void safeIncrement(int &count) {
myMutex.lock();
++count; // Critical section
myMutex.unlock();
}
In this example, the critical section is the increment operation on the `count` variable. Locking is essential here to ensure that no two threads increment `count` at the same time.
Scoped Locking
A safer alternative to manual locking is to use `std::lock_guard`. This RAII (Resource Acquisition Is Initialization) style object locks the mutex upon creation and automatically unlocks it when the lock_guard goes out of scope, providing better exception safety. Here’s how it looks:
void safeIncrement(int &count) {
std::lock_guard<std::mutex> guard(myMutex);
++count; // Critical section is automatically protected
}
With `std::lock_guard`, you don’t need to worry about forgetting to unlock the mutex, which can lead to deadlocks.
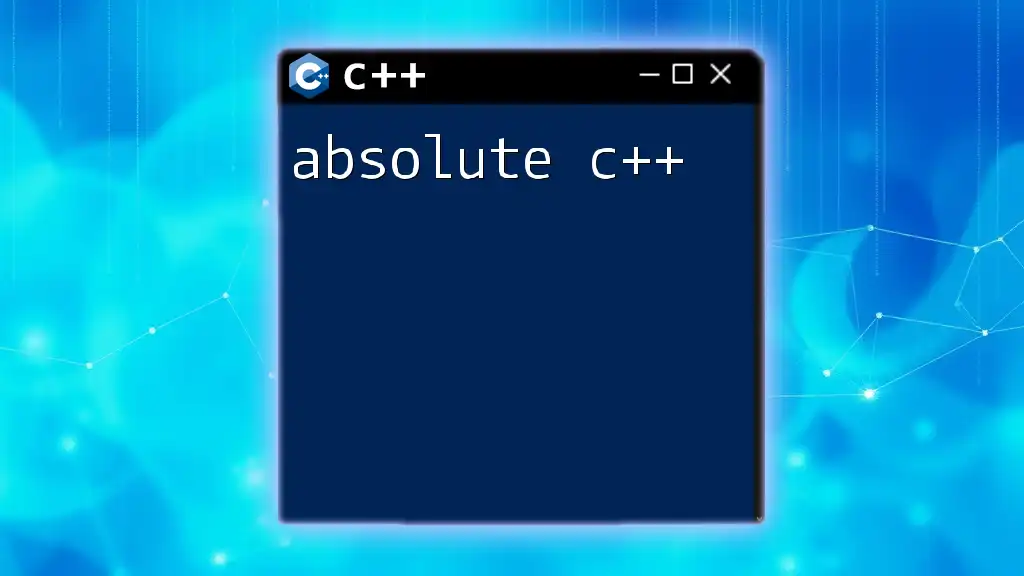
Advanced Mutex Techniques
Using `std::unique_lock`
While `std::lock_guard` is great for simple scenarios, `std::unique_lock` offers additional features such as deferred locking and timed locking. This flexibility allows you to manually manage the locking process and even use it with condition variables. Here’s an example:
void safeIncrement(int &count) {
std::unique_lock<std::mutex> lock(myMutex); // Locking while allowing more flexibility
// Perform actions...
lock.unlock(); // Unlock when done
}
With `std::unique_lock`, you gain more control over when and how the mutex is locked and unlocked.
Deadlocks and How to Avoid Them
Deadlocks can occur when two or more threads are waiting for each other to release locks. This situation leads to a standstill where no progress can be made. To prevent deadlocks:
- Always acquire locks in a consistent order across threads.
- Use timeouts or try-lock mechanisms to avoid indefinite waiting.
Consider the following example that illustrates a potential deadlock scenario:
std::mutex mutexA, mutexB;
void threadFunction1() {
std::lock_guard<std::mutex> guardA(mutexA);
std::this_thread::sleep_for(std::chrono::milliseconds(100)); // Emulate processing time
std::lock_guard<std::mutex> guardB(mutexB); // Could cause a deadlock if another thread has mutexB
}
void threadFunction2() {
std::lock_guard<std::mutex> guardB(mutexB);
std::this_thread::sleep_for(std::chrono::milliseconds(100)); // Emulate processing time
std::lock_guard<std::mutex> guardA(mutexA); // Could cause a deadlock if another thread has mutexA
}
In this situation, if both threads execute simultaneously, a deadlock can occur. To avoid this, use a consistent locking order across all threads.
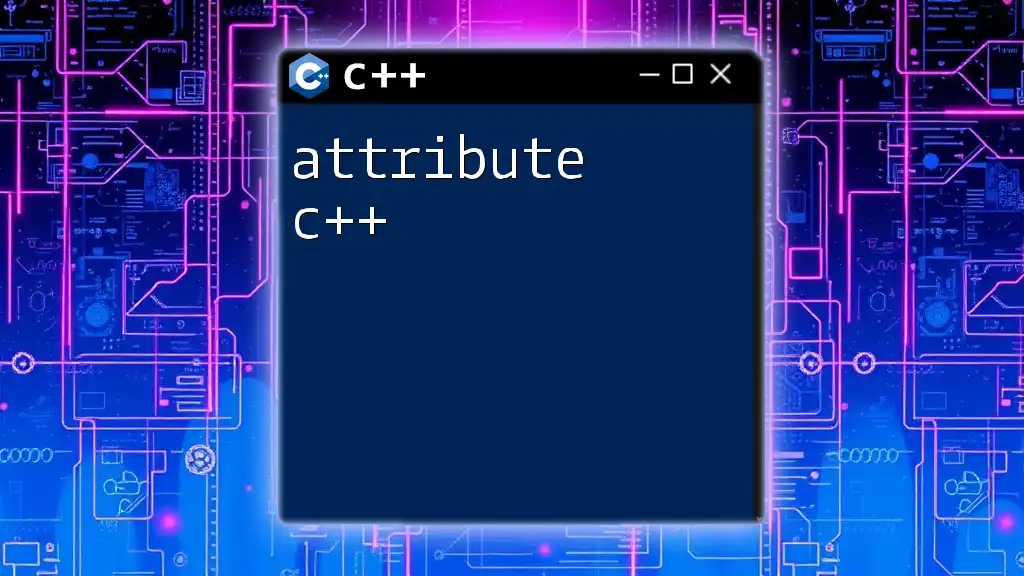
Best Practices for Using Mutexes
Keep Locks Short
To optimize performance, it is critical to keep the locked sections of code as short as possible. This minimizes contention between threads, enhancing throughput. Lock only the necessary operations, allowing other threads swift access when not in the critical section. For instance:
void optimizedIncrement(int &count) {
{
std::lock_guard<std::mutex> guard(myMutex);
// Critical section
++count;
} // Mutex automatically released here
// Other non-critical operations
}
Avoid Nested Locks
Nested locks can complicate code and increase the likelihood of deadlocks. Aim to structure your code to avoid nested locking instances. If nested locks are unavoidable, consider using a single lock for multiple resources when possible. Here’s an example illustrating better code structure:
void combinedFunction() {
std::unique_lock<std::mutex> lockA(mutexA);
// Do work that requires mutexA
// Instead of locking mutexB here, refactor the logic to minimize locking
lockA.unlock();
}
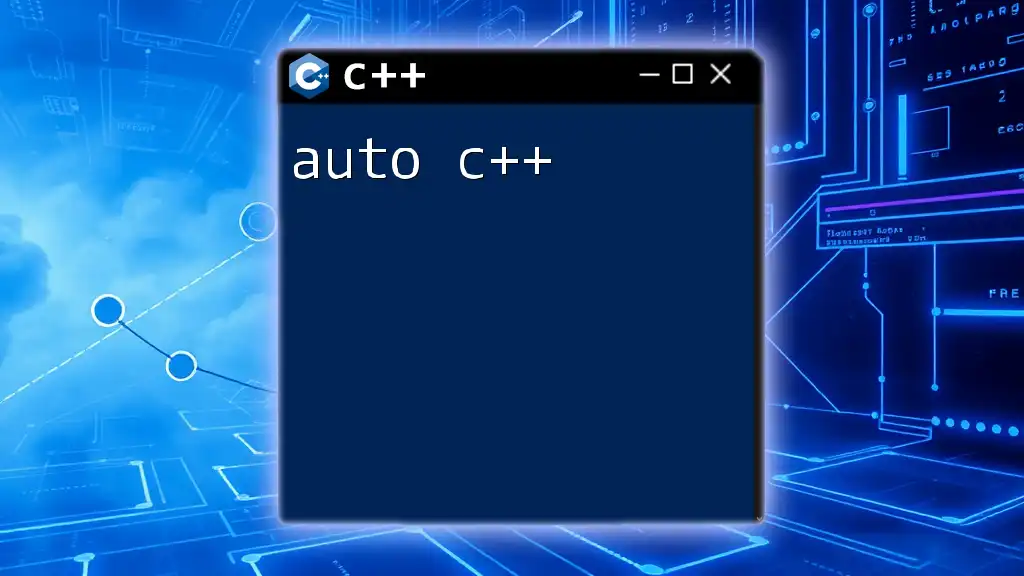
Alternative Synchronization Mechanisms
Reader-Writer Locks
In scenarios where a resource can be read by multiple threads but modified by only one, reader-writer locks may be more efficient than mutexes. These locks allow concurrent read access while ensuring exclusive write access.
Condition Variables
Condition variables are useful for signaling between threads. They work in conjunction with mutexes to allow threads to wait for certain conditions to be met. Here's a simple snippet demonstrating their usage:
std::condition_variable condVar;
std::mutex condMutex;
void producer() {
std::unique_lock<std::mutex> lock(condMutex);
// Produce an item, then notify consumer
condVar.notify_one();
}
void consumer() {
std::unique_lock<std::mutex> lock(condMutex);
condVar.wait(lock); // Wait for notification
// Consume the item
}
In this code, the consumer waits for a notification from the producer, ensuring coordination between threads.
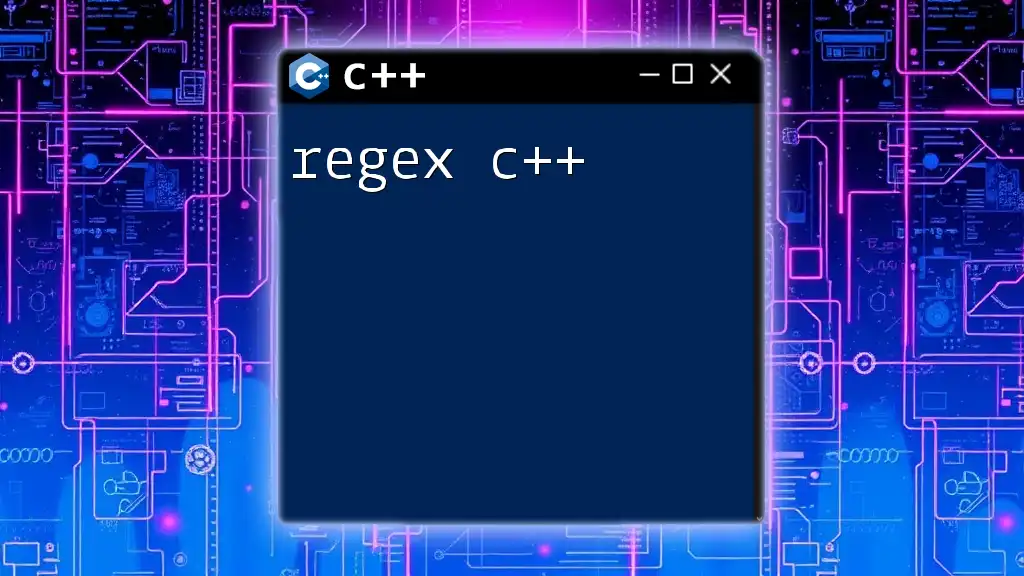
Conclusion
Mutexes are essential in C++ for synchronizing access to shared resources in multithreaded applications. By understanding how to properly implement and utilize mutexes, developers can effectively prevent race conditions and improve code safety. By consistently applying best practices, employing advanced techniques like scoped and unique locks, and exploring other synchronization mechanisms, programmers can elevate their concurrent programming skills and build robust applications for performance and reliability.