Linux C++ refers to the use of the C++ programming language for developing software applications in a Linux environment, allowing developers to leverage its efficiency and low-level system access.
Here's a simple code snippet that demonstrates a "Hello World" program in C++ for Linux:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
History of C++ and Linux
C++ has a rich history, emerging in the early 1980s as an enhancement of the C programming language. Its introduction by Bjarne Stroustrup was driven by the need for a language that provided both high-level abstractions and low-level control over system resources. Over the decades, C++ has become a backbone for many software projects, especially within Linux systems.
Linux, created by Linus Torvalds in 1991, is one of the most prominent operating systems that supports C++. The synergy between C++ and Linux has given rise to numerous successful projects, such as the KDE desktop environment and the popular game development frameworks. Understanding the relationship between C++ and Linux is essential for any programmer looking to excel on this platform.
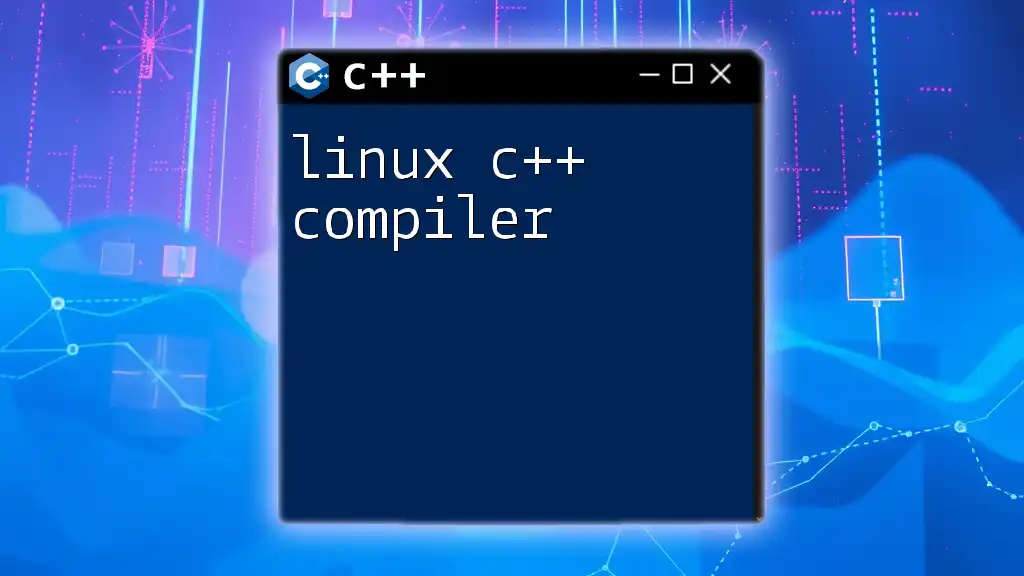
Setting Up Your C++ Development Environment on Linux
Choosing a Linux Distribution
When starting with Linux C++, the first step is selecting a suitable Linux distribution. Popular options include:
- Ubuntu: Known for its user-friendly interface and extensive documentation, which is great for beginners.
- Fedora: Offers cutting-edge features and packages, making it a favorite among developers who wish to stay up to date.
- Arch: A more advanced option that allows for extreme customization, ideal for users who want total control over their environment.
Each distribution has its own strengths and weaknesses, so choose one that aligns with your development needs.
Installing Essential Tools
Installing a C++ Compiler: A crucial step in setting up your environment is installing a C++ compiler. GCC (GNU Compiler Collection) is the most commonly used C++ compiler on Linux systems. To install GCC on Ubuntu, for example, you would run:
sudo apt update
sudo apt install g++
Once installed, you can verify the installation by checking the version:
g++ --version
Code Example: Compiling a Basic Program
Create a simple C++ file, `hello.cpp`, containing:
#include <iostream>
int main() {
std::cout << "Hello, Linux!" << std::endl;
return 0;
}
Compile the program using:
g++ hello.cpp -o hello
Run it with:
./hello
Using Make and CMake: Once you've installed a compiler, managing larger C++ projects becomes essential. Make is a build automation tool that simplifies this process. Here's a simple Makefile for the `hello` program:
all: hello
hello: hello.o
g++ -o hello hello.o
hello.o: hello.cpp
g++ -c hello.cpp
clean:
rm -f hello hello.o
To compile using this Makefile, simply execute the command:
make
IDEs and Text Editors: While command-line tools are powerful, having a good Integrated Development Environment (IDE) or text editor can enhance your productivity. Recommended IDEs include Code::Blocks for its simplicity and CLion for its advanced features. If you prefer a lightweight experience, text editors like Vim, Emacs, or Visual Studio Code are excellent alternatives.
Writing Your First C++ Program on Linux
Creating Your C++ File
Once your environment is set up, it's time to write your first program. You can create a C++ file using your preferred text editor. For a quick command-line approach, you can use `nano` or `vim`:
nano calculator.cpp
Once in the editor, you can write your code.
Compiling and Running Your Program
After writing your C++ code, it’s time to compile and run it. This can be done using the same commands we went through earlier with `g++`. Make sure you are in the correct directory where your `calculator.cpp` file resides.
Example Project: Building a Simple Calculator
Here’s a simple calculator program demonstrating basic operations:
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter operation (+, -, *, /): ";
std::cin >> operation;
std::cout << "Enter second number: ";
std::cin >> num2;
switch(operation) {
case '+':
std::cout << "Result: " << num1 + num2 << std::endl;
break;
case '-':
std::cout << "Result: " << num1 - num2 << std::endl;
break;
case '*':
std::cout << "Result: " << num1 * num2 << std::endl;
break;
case '/':
if(num2 != 0)
std::cout << "Result: " << num1 / num2 << std::endl;
else
std::cout << "Division by zero!" << std::endl;
break;
default:
std::cout << "Invalid operation!" << std::endl;
}
return 0;
}
Compile and run your program, and enjoy testing its functionalities!
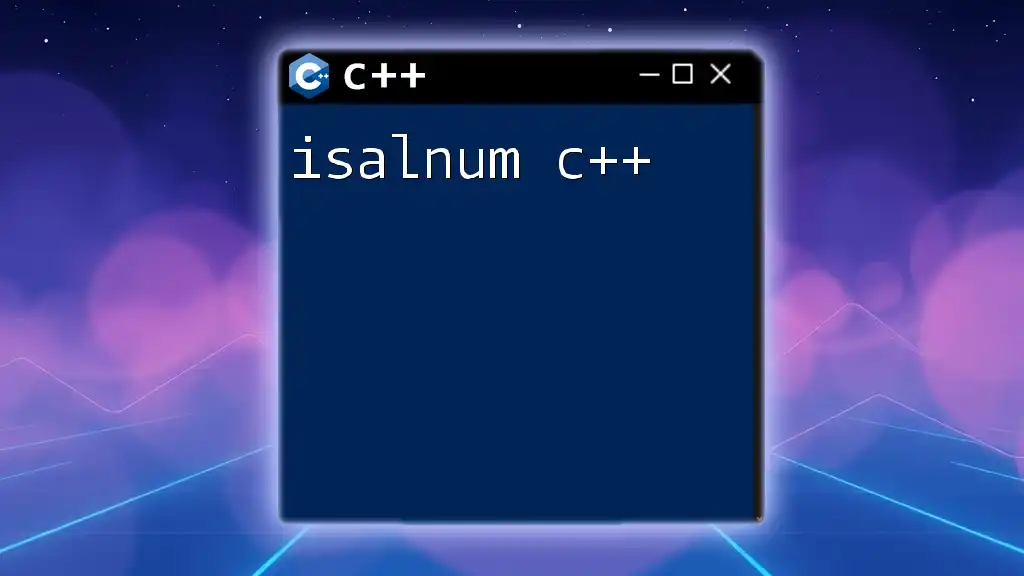
Advanced C++ Concepts in Linux
Object-Oriented Programming in C++
C++ allows programmers to utilize Object-Oriented Programming (OOP) principles, which are crucial for building scalable and maintainable software. The fundamental OOP concepts include:
- Classes: Blueprints for creating objects (data structures).
- Inheritance: Mechanism to create a new class from an existing class.
- Polymorphism: Ability to present the same interface for different data types.
By using classes, you can encapsulate data and functions that operate on that data, leading to more organized and modular code.
Utilizing Standard Template Library (STL)
The Standard Template Library (STL) is a powerful set of C++ template classes to provide general-purpose classes and functions. Vectors, lists, and maps are some of the major components in STL that simplify data handling significantly.
Code Example: Using a Vector
Here’s an example demonstrating the use of a vector to store integers:
#include <iostream>
#include <vector>
int main(){
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this code snippet, we create a vector of integers and use a range-based for loop to print its elements.
Multithreading and Concurrency in C++
As software becomes increasingly complex, managing multiple tasks simultaneously is essential. C++ provides the `<thread>` library that allows developers to create multi-threaded applications with ease.
Example of a Simple Multithreaded Program
Here’s a basic example of defining and executing a thread:
#include <iostream>
#include <thread>
void function1() {
std::cout << "Thread 1 executing." << std::endl;
}
int main() {
std::thread t1(function1);
t1.join(); // Wait for the thread to finish
return 0;
}
In this example, we define a simple function that will run in a separate thread. Using `join()`, the main thread waits for `t1` to complete before proceeding.
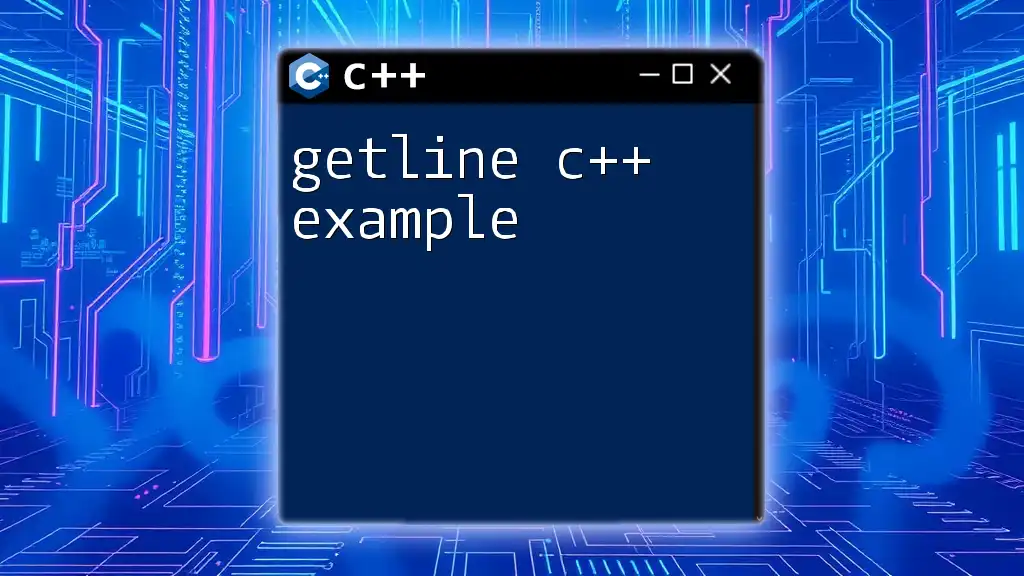
Debugging C++ Programs on Linux
Using gdb (GNU Debugger)
Debugging is an essential skill for programmers. gdb (GNU Debugger) allows you to inspect what is happening inside your program while it executes or what it was doing at the moment it crashed. To use gdb, compile your program with debugging symbols:
g++ -g your_program.cpp -o your_program
Launch gdb with your executable:
gdb ./your_program
You can set breakpoints, inspect variables, and step through your program line by line.
Valgrind for Memory Management
Memory management is a critical aspect of C++ programming. Valgrind is a tool that helps find memory leaks and memory-related errors. To install Valgrind on Ubuntu, run:
sudo apt install valgrind
Run your program with Valgrind to analyze memory usage:
valgrind --leak-check=full ./your_program
This will report any memory leaks or improper memory access, helping you maintain clean code.
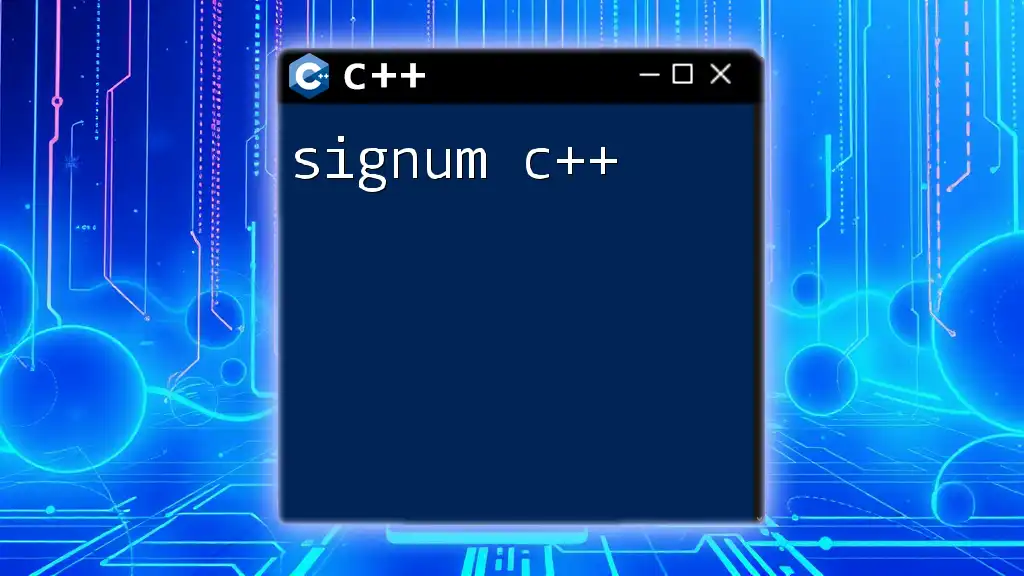
Conclusion
Exploring Linux C++ opens up a world of possibilities for software development. From setting up your environment and writing your first programs to delving into advanced concepts and debugging, everything contributes to your growth as a proficient C++ programmer on Linux systems. Embrace practice and experimentation, as these experiences will greatly enhance your coding journey.
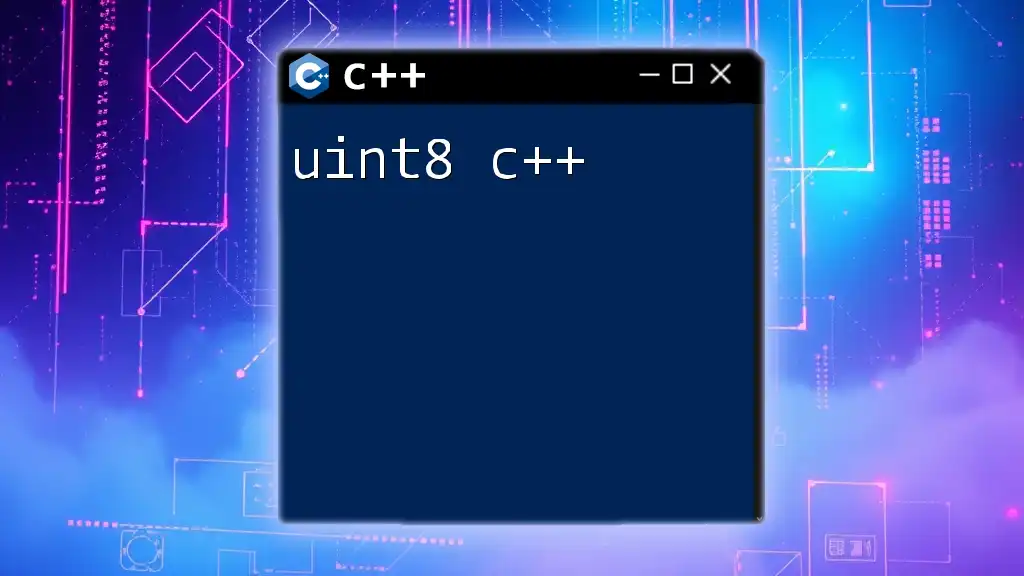
Additional Resources
When you're ready to further your knowledge, check out recommended C++ books and tutorials. Engaging with online communities and forums can also provide valuable support and insights from fellow C++ and Linux enthusiasts. Happy coding!